How to Get Users with the Intercom API in PHP
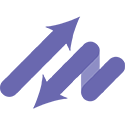
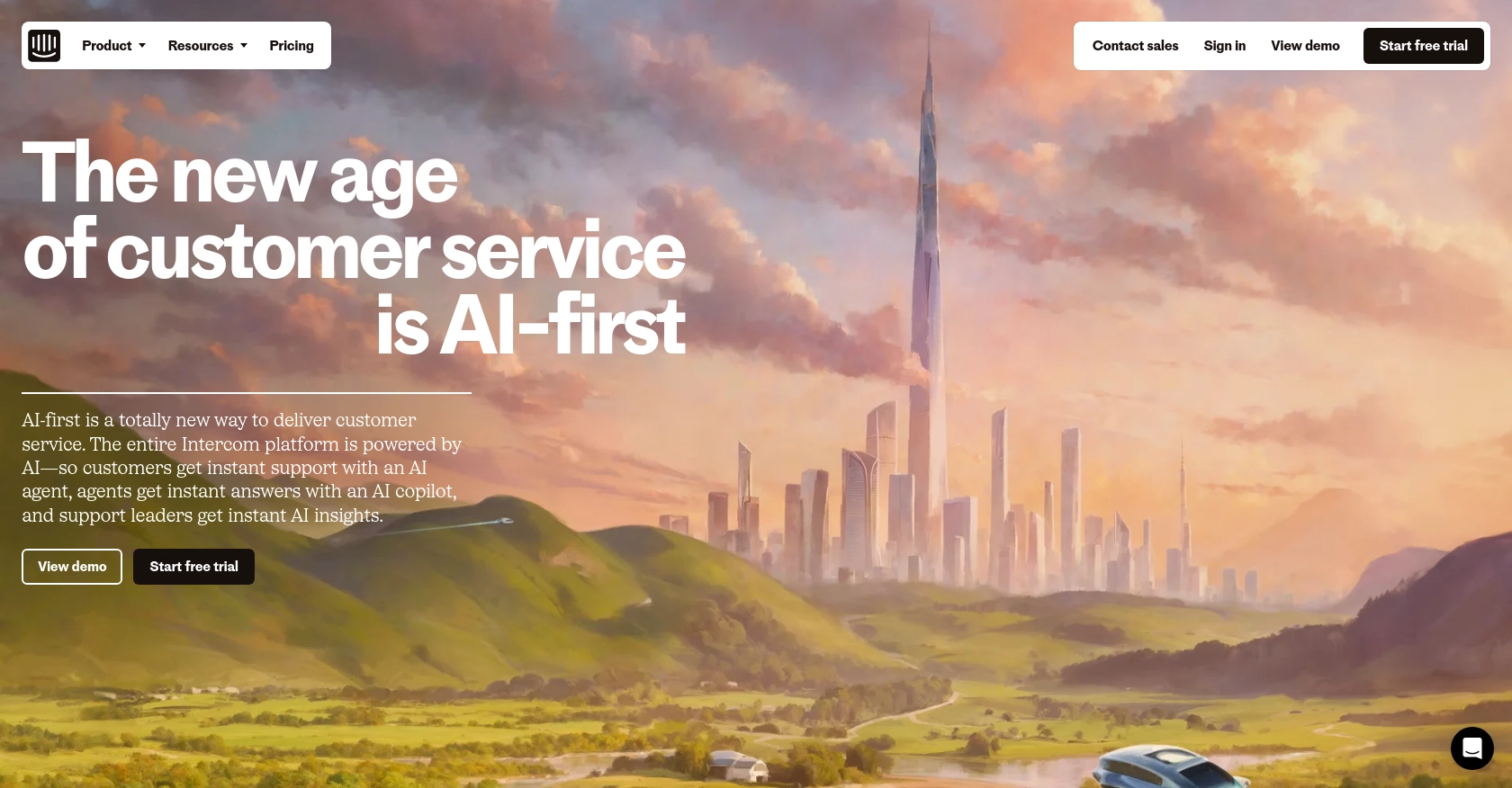
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for live chat, email marketing, and customer feedback, making it an essential tool for enhancing customer relationships.
Developers may want to integrate with Intercom's API to streamline customer data management and automate communication workflows. For example, using the Intercom API, a developer can retrieve user information to personalize interactions or trigger automated messages based on user behavior.
This article will guide you through the process of using PHP to interact with the Intercom API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access and manage user information within the Intercom platform using PHP.
Setting Up Your Intercom Test or Sandbox Account
Before you can start integrating with the Intercom API using PHP, you'll need to set up a test or sandbox account. This will allow you to safely test your API interactions without affecting live data.
Creating an Intercom Developer Workspace
To begin, you'll need to create a development workspace on Intercom. This workspace will serve as your testing environment.
- Visit the Intercom Developer Hub and sign up for a free account.
- Once registered, navigate to the Developer Hub and create a new app. Make sure to select the option to use OAuth for authentication.
- Fill in the required information, including your app's name and description.
Configuring OAuth for Intercom API Access
Intercom uses OAuth for authentication, which allows your app to access user data securely. Follow these steps to configure OAuth:
- In your app's settings, locate the "Authentication" section and enable OAuth.
- Provide the necessary redirect URLs. These URLs will be used to redirect users after they authorize your app. Ensure they are HTTPS.
- Select the permissions your app requires. For retrieving user data, ensure you have the "Read and list users and companies" scope enabled.
Generating Client ID and Client Secret
To authenticate API requests, you'll need a Client ID and Client Secret:
- In the Developer Hub, navigate to your app's "Basic Information" page.
- Locate your Client ID and Client Secret. These credentials will be used to obtain an access token.
With your Intercom test account set up and OAuth configured, you're ready to start making API calls to retrieve user data using PHP. In the next section, we'll walk through the process of making these API calls.
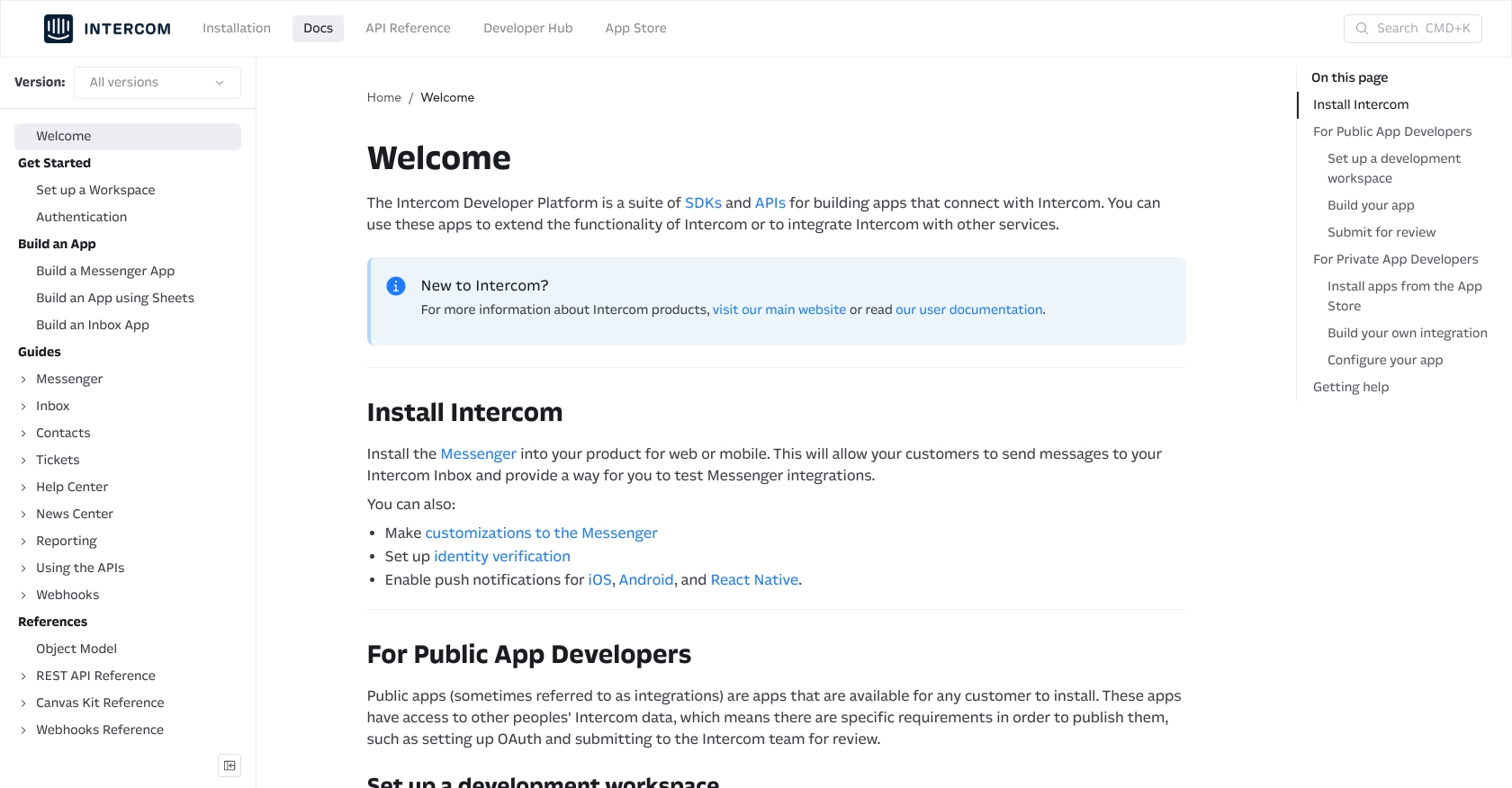
sbb-itb-96038d7
Making API Calls to Retrieve Users with Intercom API in PHP
Now that your Intercom development workspace is set up and OAuth is configured, it's time to make API calls to retrieve user data using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to interact with the Intercom API, and handling potential errors.
Setting Up Your PHP Environment for Intercom API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Ensure you have PHP 7.4 or later installed on your machine.
- Install Composer, the PHP package manager, if you haven't already.
- Use Composer to install the Guzzle HTTP client, which will be used to make HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Users from Intercom API
With your environment set up, you can now write the PHP code to retrieve user data from the Intercom API:
<?php
require_once('vendor/autoload.php');
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://api.intercom.io/users', [
'headers' => [
'Intercom-Version' => '2.11',
'accept' => 'application/json',
'authorization' => 'Bearer <ACCESS_TOKEN>',
],
]);
echo $response->getBody();
?>
Replace <ACCESS_TOKEN>
with the access token obtained during the OAuth setup. This code initializes the Guzzle HTTP client, sets the necessary headers, and sends a GET request to the Intercom API to retrieve user data.
Verifying API Call Success and Handling Errors
After running the PHP script, you should see the user data returned from the Intercom API. To verify the success of the API call, check the response status code:
<?php
if ($response->getStatusCode() === 200) {
echo "API call successful!";
} else {
echo "Failed to retrieve users. Status code: " . $response->getStatusCode();
}
?>
Handle potential errors by checking the status code and implementing error handling logic. Common error codes include 401 for unauthorized access and 404 for not found. Refer to the Intercom API documentation for more details on error codes.
Testing and Debugging Your Intercom API Integration
Test your integration thoroughly in the development workspace to ensure it works as expected. Use logging and debugging tools to troubleshoot any issues that arise during the API interaction.
By following these steps, you can efficiently retrieve user data from the Intercom API using PHP, enabling you to enhance your application's customer interaction capabilities.
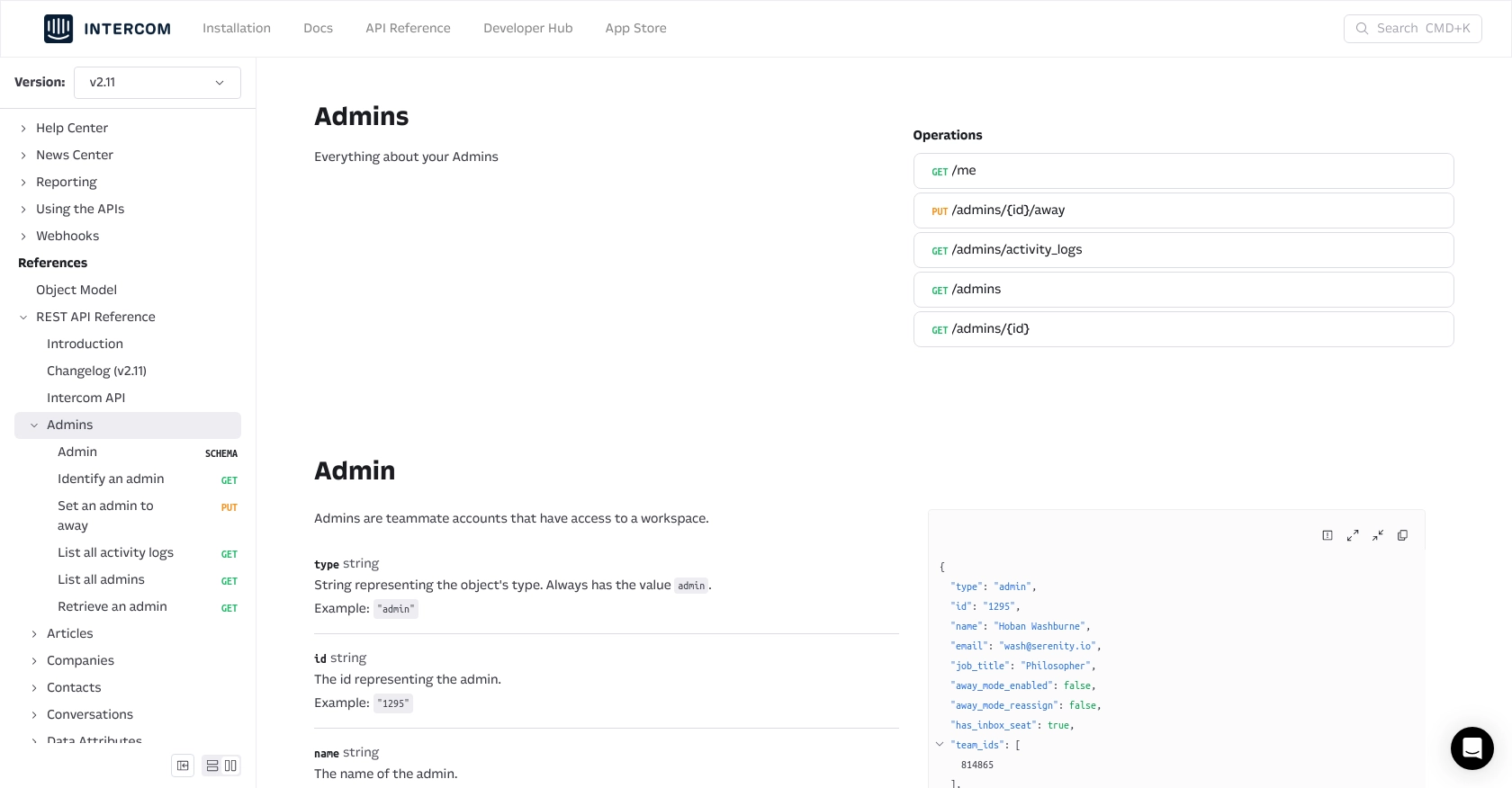
Conclusion and Best Practices for Intercom API Integration in PHP
Integrating with the Intercom API using PHP allows developers to efficiently manage user data and automate communication workflows, enhancing customer engagement. By following the steps outlined in this guide, you can set up a robust integration that leverages Intercom's powerful features.
Best Practices for Secure and Efficient Intercom API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and Access Tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Intercom's API may impose rate limits. Implement logic to handle rate limits gracefully, such as retrying requests after a delay. Refer to the Intercom API documentation for specific rate limit details.
- Data Standardization: Ensure that data retrieved from Intercom is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage potential API errors, such as unauthorized access or resource not found, to ensure a smooth user experience.
Enhance Your Integration Strategy with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Consider leveraging Endgrate to streamline your integration efforts. With Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations, simplifying maintenance.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?