Using the Clickup API to Create Teams in Javascript
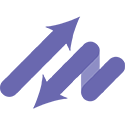
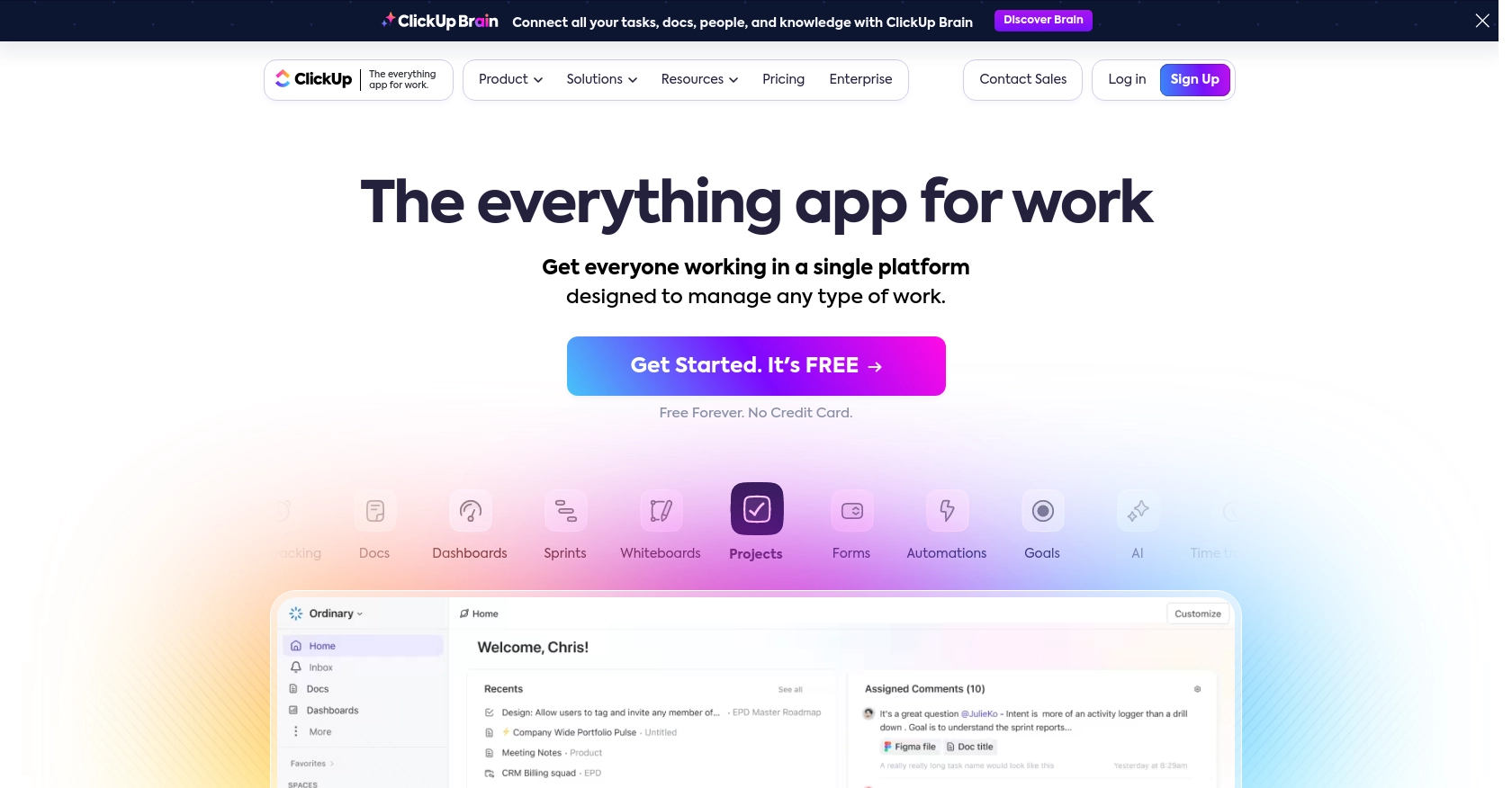
Introduction to ClickUp API Integration
ClickUp is a versatile project management platform that offers a wide range of features to help teams collaborate and manage tasks efficiently. With its customizable interface and robust functionality, ClickUp is a popular choice for businesses looking to streamline their workflow and improve productivity.
Developers may want to integrate with ClickUp's API to automate and enhance their project management processes. For example, using the ClickUp API, you can create teams programmatically, allowing for dynamic team management and assignment of tasks within your workspace.
This article will guide you through using JavaScript to interact with the ClickUp API, specifically focusing on creating teams. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your ClickUp Test Account and OAuth Authentication
Before you can start using the ClickUp API to create teams, you'll need to set up a ClickUp account and configure OAuth authentication. This process ensures secure access to ClickUp's resources and allows your application to interact with the API on behalf of users.
Creating a ClickUp Account
If you don't already have a ClickUp account, you can easily sign up for a free account on the ClickUp website. Follow the on-screen instructions to complete the registration process. Once your account is set up, you'll be ready to proceed with the API integration.
Generating a Personal API Token
For personal use or testing purposes, you can generate a personal API token. This token will be used to authenticate your API requests.
- Log into your ClickUp account.
- In ClickUp 3.0, click your avatar in the upper-right corner, select Settings, and scroll down to click Apps in the sidebar.
- Under API Token, click Generate.
- Copy and save your personal API token securely, as you'll need it for API requests.
Setting Up OAuth for Application Development
If you're developing an application for others to use, you'll need to implement OAuth authentication. This allows users to authorize your app to access their ClickUp resources.
- Log into ClickUp and click on your avatar in the lower-left corner, then select Integrations.
- Click on ClickUp API and then Create an App.
- Provide a name for your app and a redirect URL. The redirect URL is where users will be sent after authorizing your app.
- Once your app is created, you'll receive a
client_id
andclient_secret
. Keep these credentials secure.
Retrieving an Authorization Code
To connect a user's ClickUp account to your app, you'll need to obtain an authorization code.
- Share the following URL with the user, replacing
{client_id}
and{redirect_uri}
with your app's details: - Once the user authorizes your app, they will be redirected to your specified redirect URL with an authorization code.
https://app.clickup.com/api?client_id={client_id}&redirect_uri={redirect_uri}
Requesting an Access Token
After obtaining the authorization code, you can request an access token to authenticate API requests.
- Use the Get Access Token endpoint with the parameters
client_id
,client_secret
, andcode
to obtain the access token. - Include this token in the Authorization header of your API requests.
For more detailed information on OAuth authentication, refer to the ClickUp Authentication Documentation.
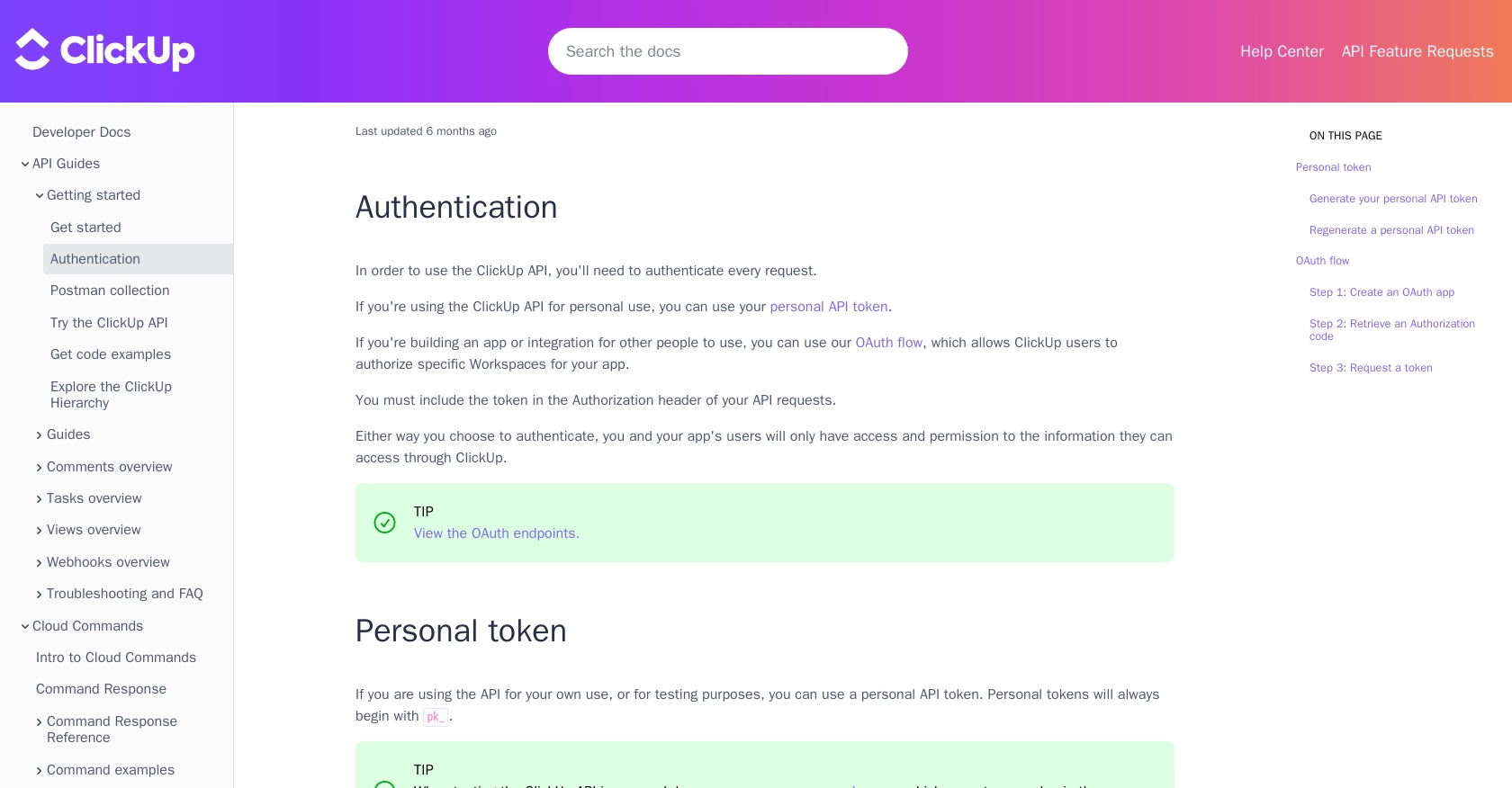
sbb-itb-96038d7
Making API Calls to Create Teams in ClickUp Using JavaScript
Once you have set up OAuth authentication, you can proceed to make API calls to create teams in ClickUp using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment
To interact with the ClickUp API, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the Axios library to handle HTTP requests.
- Open your terminal and navigate to your project directory.
- Initialize a new Node.js project by running:
- Install Axios by running:
npm init -y
npm install axios
Writing JavaScript Code to Create a Team in ClickUp
With your environment ready, you can now write the JavaScript code to create a team in ClickUp. The following code demonstrates how to make a POST request to the ClickUp API to create a team.
const axios = require('axios');
// Replace with your actual access token
const accessToken = 'Your_Access_Token';
// Define the API endpoint and request headers
const endpoint = 'https://api.clickup.com/api/v2/team/{team_id}/group';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
};
// Define the team data
const teamData = {
name: 'New Team Name',
members: [123456, 987654] // Replace with actual member IDs
};
// Make the POST request to create the team
axios.post(endpoint, teamData, { headers })
.then(response => {
console.log('Team Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Team:', error.response ? error.response.data : error.message);
});
Replace Your_Access_Token
with the access token obtained during the OAuth process. Also, update the team_id
and members
array with the appropriate values for your workspace.
Verifying the API Request and Handling Errors
After running the code, you should see a success message in the console if the team is created successfully. You can verify the creation by checking your ClickUp workspace for the new team.
In case of errors, the code includes error handling to log the error message. Common issues might include invalid tokens or incorrect team data. Ensure your access token is valid and the member IDs exist in your workspace.
For more details on the API call, refer to the ClickUp API Documentation.
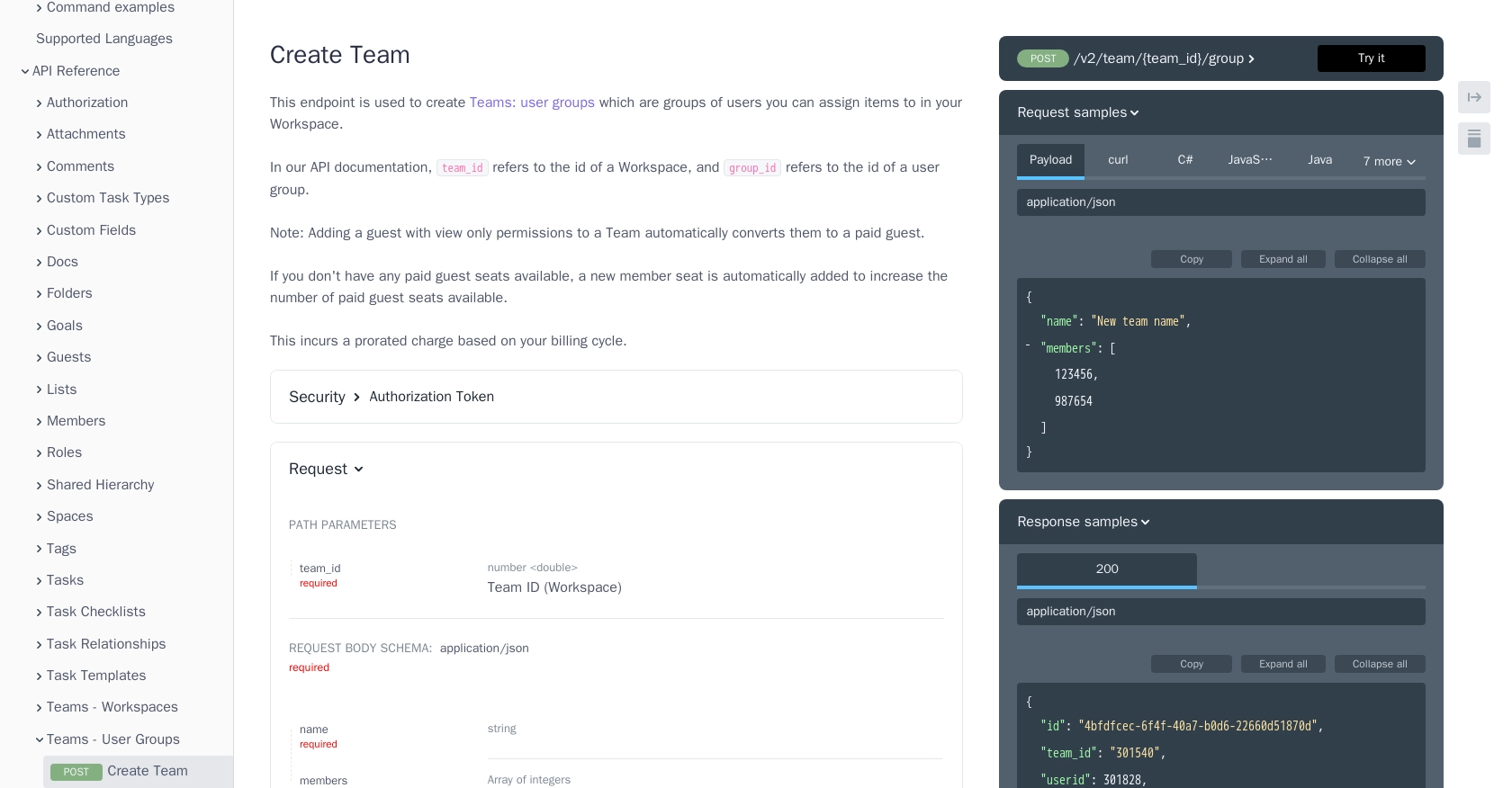
Conclusion and Best Practices for Using ClickUp API with JavaScript
Integrating with the ClickUp API to create teams using JavaScript can significantly enhance your project management capabilities by automating team management and task assignments. By following the steps outlined in this article, you can efficiently set up OAuth authentication, make API calls, and handle responses to create teams programmatically.
Best Practices for Secure and Efficient ClickUp API Integration
- Secure Storage of Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information. - Handling Rate Limits: Be mindful of ClickUp's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when sending and receiving data from the API. This helps maintain data integrity across different systems and applications.
- Error Handling: Implement comprehensive error handling to manage API response errors effectively. Log errors for troubleshooting and provide user-friendly messages when issues occur.
Streamlining Integrations with Endgrate
While integrating with ClickUp's API can be powerful, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including ClickUp. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?