Using the Salesloft API to Get Tasks in Javascript
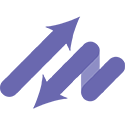
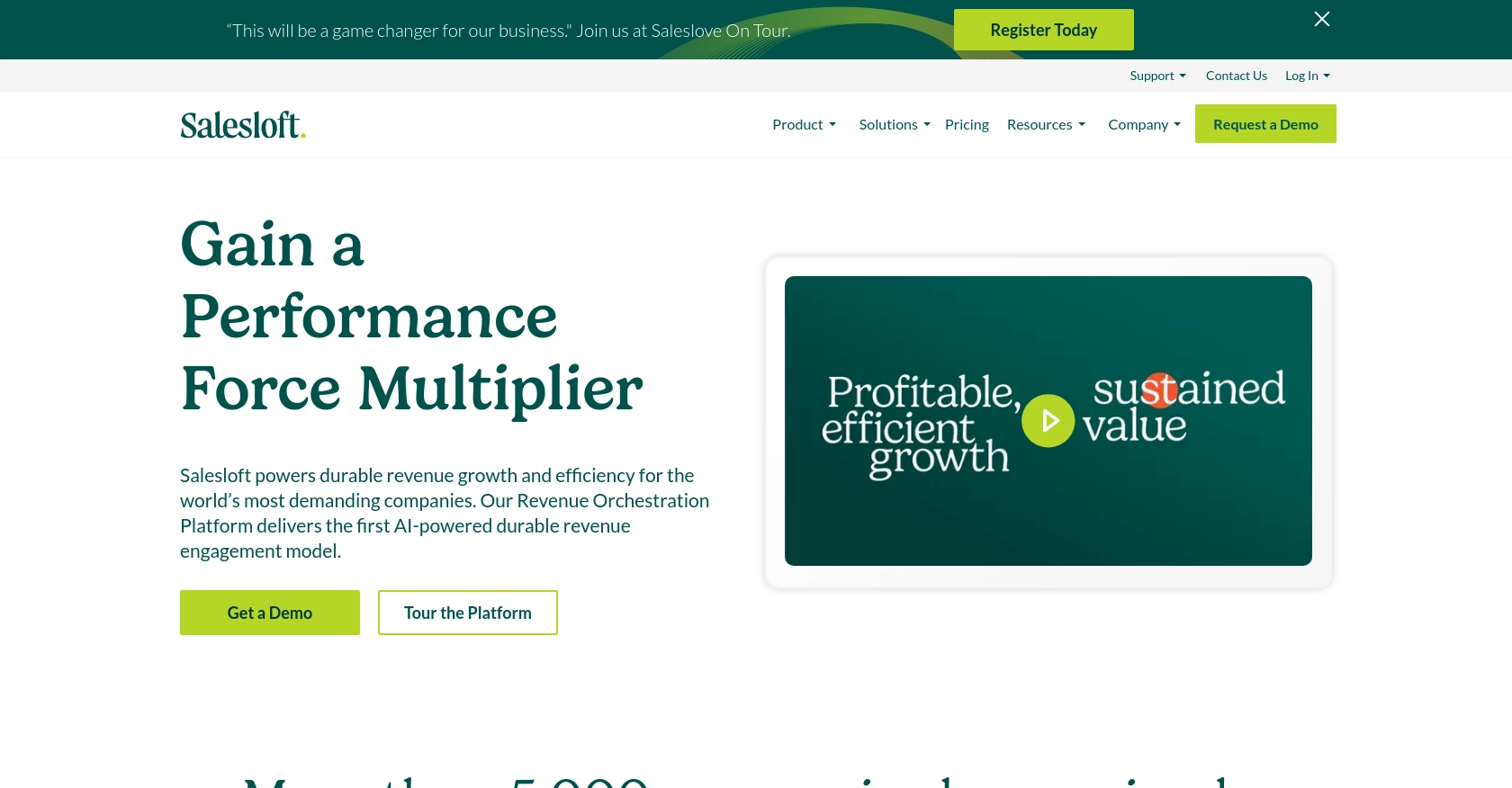
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform designed to enhance the productivity and efficiency of sales teams. It offers a suite of tools that streamline communication, automate workflows, and provide valuable insights into sales processes.
Integrating with the Salesloft API allows developers to access and manage sales tasks programmatically, enabling seamless integration with other systems and applications. For example, a developer might use the Salesloft API to retrieve tasks and automate follow-up actions, ensuring that no sales opportunity is missed.
Setting Up Your Salesloft Test Account for API Integration
Before diving into the Salesloft API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment for developers to test their integrations.
Creating a Salesloft Sandbox Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This trial account will give you access to the necessary features to test API integrations.
- Visit the Salesloft website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access the Salesloft dashboard.
Creating a Salesloft OAuth App for API Access
To interact with the Salesloft API, you'll need to create an OAuth application. This will provide you with the necessary credentials to authenticate API requests.
- Navigate to your Salesloft account settings.
- Go to Your Applications and select OAuth Applications.
- Click on Create New to start a new OAuth application.
- Fill in the required fields, such as the application name and description, and click Save.
- After saving, you will receive your Client ID and Client Secret. Make sure to store these securely as they are needed for authentication.
Obtaining Authorization Code and Access Tokens
With your OAuth app set up, you can now obtain the authorization code and access tokens needed to make API calls.
- Direct users to the following URL, replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
with your app's details: - Once the user authorizes the app, they will be redirected to your specified URI with a
code
parameter. - Exchange this code for an access token by making a POST request to the token endpoint:
- Store the returned
access_token
andrefresh_token
securely for future API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With these steps completed, your Salesloft test account is ready for API integration, allowing you to explore and interact with Salesloft's powerful features programmatically.
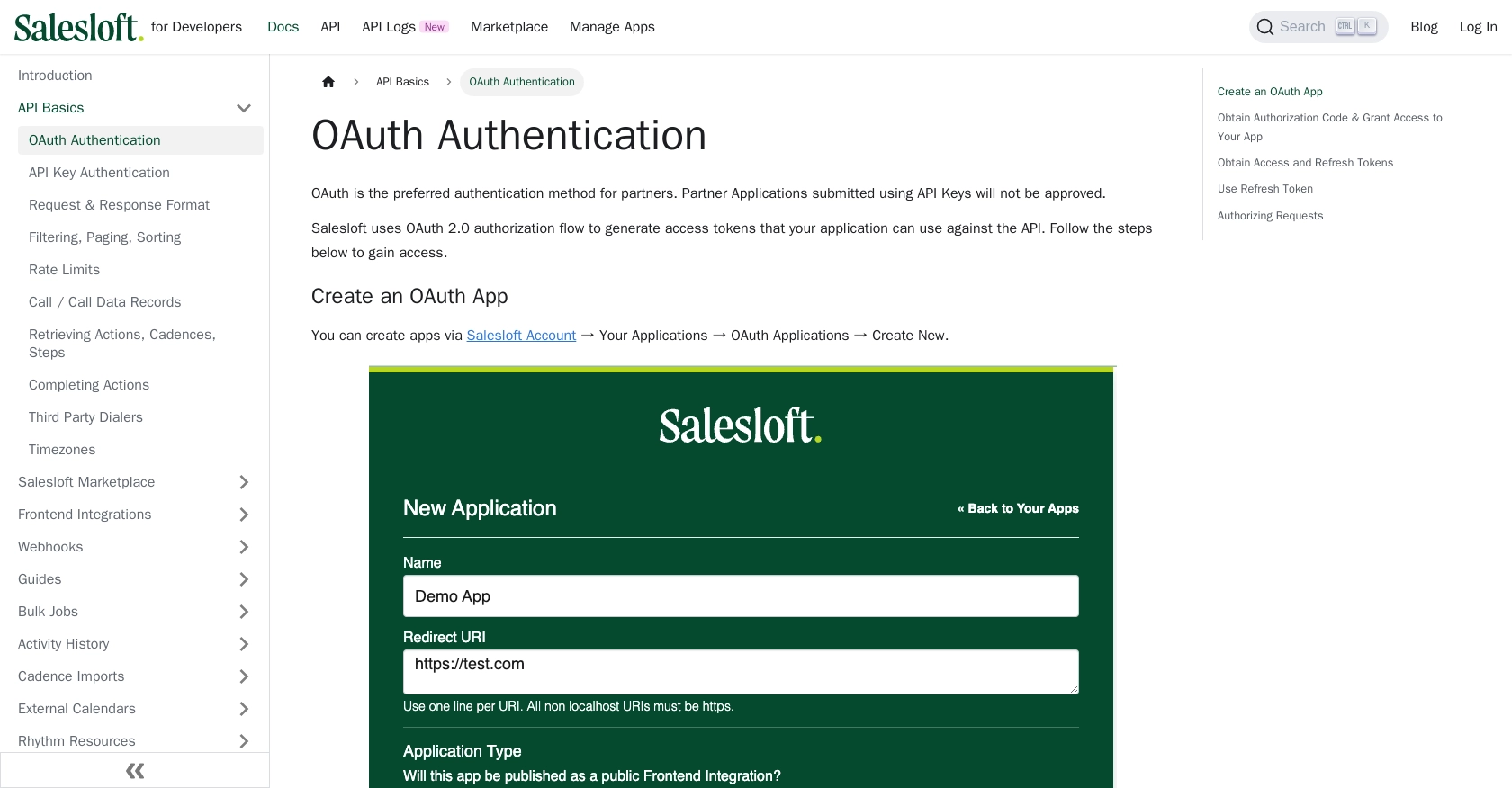
sbb-itb-96038d7
Making API Calls to Retrieve Salesloft Tasks Using JavaScript
To interact with the Salesloft API and retrieve tasks, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Salesloft API Integration
Before you begin, ensure that you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side scripting and API interactions.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions.
Installing Required Dependencies for Salesloft API Calls
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Tasks from Salesloft API
Create a new JavaScript file named getSalesloftTasks.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.salesloft.com/v2/tasks';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to fetch tasks
async function fetchTasks() {
try {
const response = await axios.get(endpoint, { headers });
const tasks = response.data.data;
// Display the tasks
tasks.forEach(task => {
console.log(`Task ID: ${task.id}, Subject: ${task.subject}, Due Date: ${task.due_date}`);
});
} catch (error) {
console.error('Error fetching tasks:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchTasks();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup.
Running the JavaScript Code to Retrieve Salesloft Tasks
Execute the script using Node.js:
node getSalesloftTasks.js
You should see a list of tasks printed in the console, each with its ID, subject, and due date.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the response status code. A status code of 200 indicates success. If an error occurs, the code will log the error message, which can help in troubleshooting.
Refer to the Salesloft API documentation for more details on error codes and handling.
Checking Results in Salesloft Sandbox
After running the script, verify the retrieved tasks by logging into your Salesloft sandbox account. Ensure that the tasks displayed in the console match those in your sandbox environment.
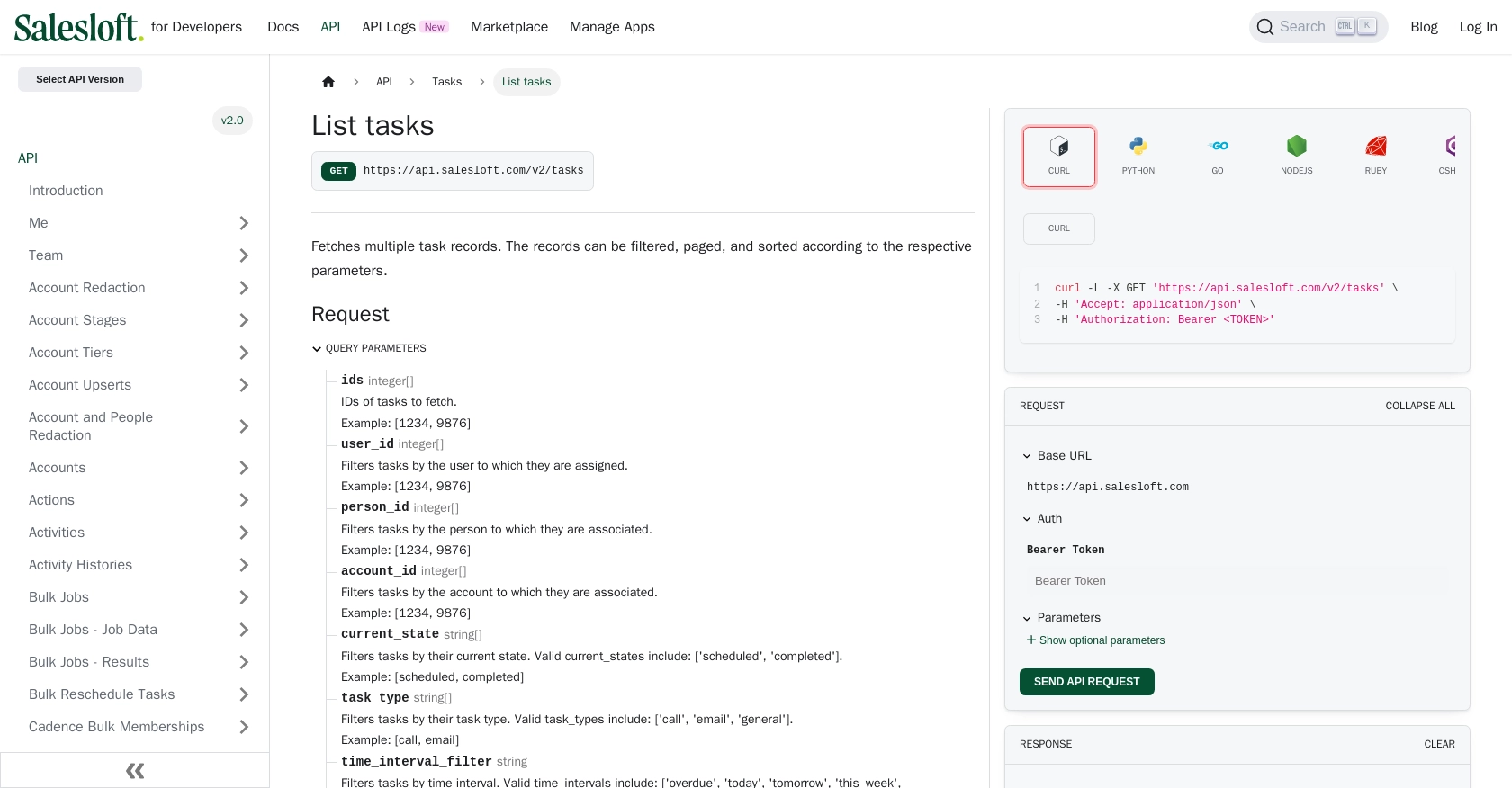
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using JavaScript provides a powerful way to automate and manage sales tasks efficiently. By following the steps outlined in this guide, you can seamlessly connect to Salesloft, retrieve tasks, and enhance your sales processes.
Best Practices for Storing Salesloft API Credentials
- Always store your
access_token
andrefresh_token
securely. Consider using environment variables or a secure vault to manage sensitive information. - Regularly refresh your tokens to maintain uninterrupted access to the API.
Handling Salesloft API Rate Limits
Salesloft imposes a rate limit of 600 cost per minute. To avoid hitting this limit:
- Implement efficient paging and filtering to minimize the number of API calls.
- Monitor the
x-ratelimit-remaining-minute
header to track your usage. - Consider batching requests where possible to reduce the number of calls.
For more details, refer to the Salesloft API rate limits documentation.
Transforming and Standardizing Salesloft Data
- Ensure that data retrieved from Salesloft is transformed to fit your application's data model.
- Standardize fields such as dates and task types to maintain consistency across your systems.
Enhancing Your Integration Strategy with Endgrate
While integrating with Salesloft directly is beneficial, using a tool like Endgrate can further streamline your integration efforts. Endgrate allows you to manage multiple integrations through a single API endpoint, saving time and resources.
By leveraging Endgrate, you can focus on your core product while outsourcing complex integration tasks. This approach not only enhances your integration capabilities but also provides a seamless experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/tasks-index/
Ready to get started?