Using the Zoho Books API to Create or Update Sales Orders in PHP
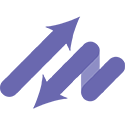
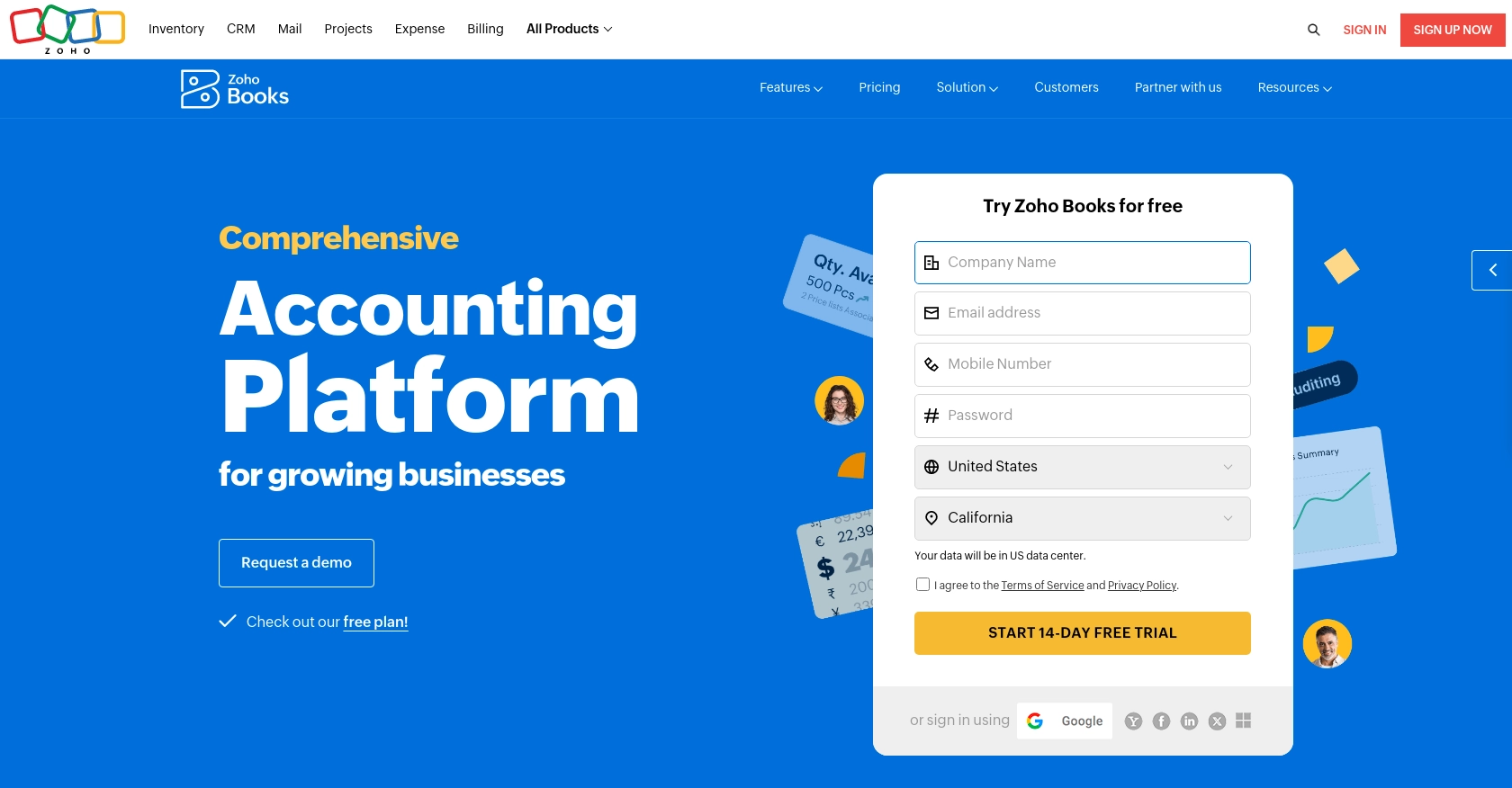
Introduction to Zoho Books API for Sales Order Management
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses of all sizes. It offers a wide range of features, including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to automate and manage sales orders efficiently. By leveraging the API, developers can create or update sales orders directly from their applications, ensuring seamless synchronization of sales data. For example, a developer might want to automate the creation of sales orders from an e-commerce platform, reducing manual data entry and minimizing errors.
In this article, we will explore how to use the Zoho Books API to create or update sales orders using PHP, providing step-by-step guidance to help you integrate this functionality into your applications.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start integrating with the Zoho Books API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Zoho Books offers a free trial that you can use to access the API and test your integration.
Creating a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial account.
- Follow the on-screen instructions to complete your account setup. Once your account is created, you will have access to the Zoho Books dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for secure API authentication. Follow these steps to register your application and obtain the necessary credentials:
- Go to the Zoho Developer Console.
- Click on "Add Client ID" to register your application.
- Fill in the required details, such as the client name, redirect URI, and authorized domains.
- Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating OAuth Tokens for Zoho Books API Access
With your Client ID and Client Secret, you can now generate OAuth tokens to access the Zoho Books API:
- Redirect users to the following authorization URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified redirect URI with a code parameter.
- Exchange the code for an access token by making a POST request to:
- Store the access token securely. It will be used in the Authorization header for API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.salesorders.CREATE,ZohoBooks.salesorders.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Now that your Zoho Books account is set up and authenticated, you are ready to start making API calls to create or update sales orders using PHP.
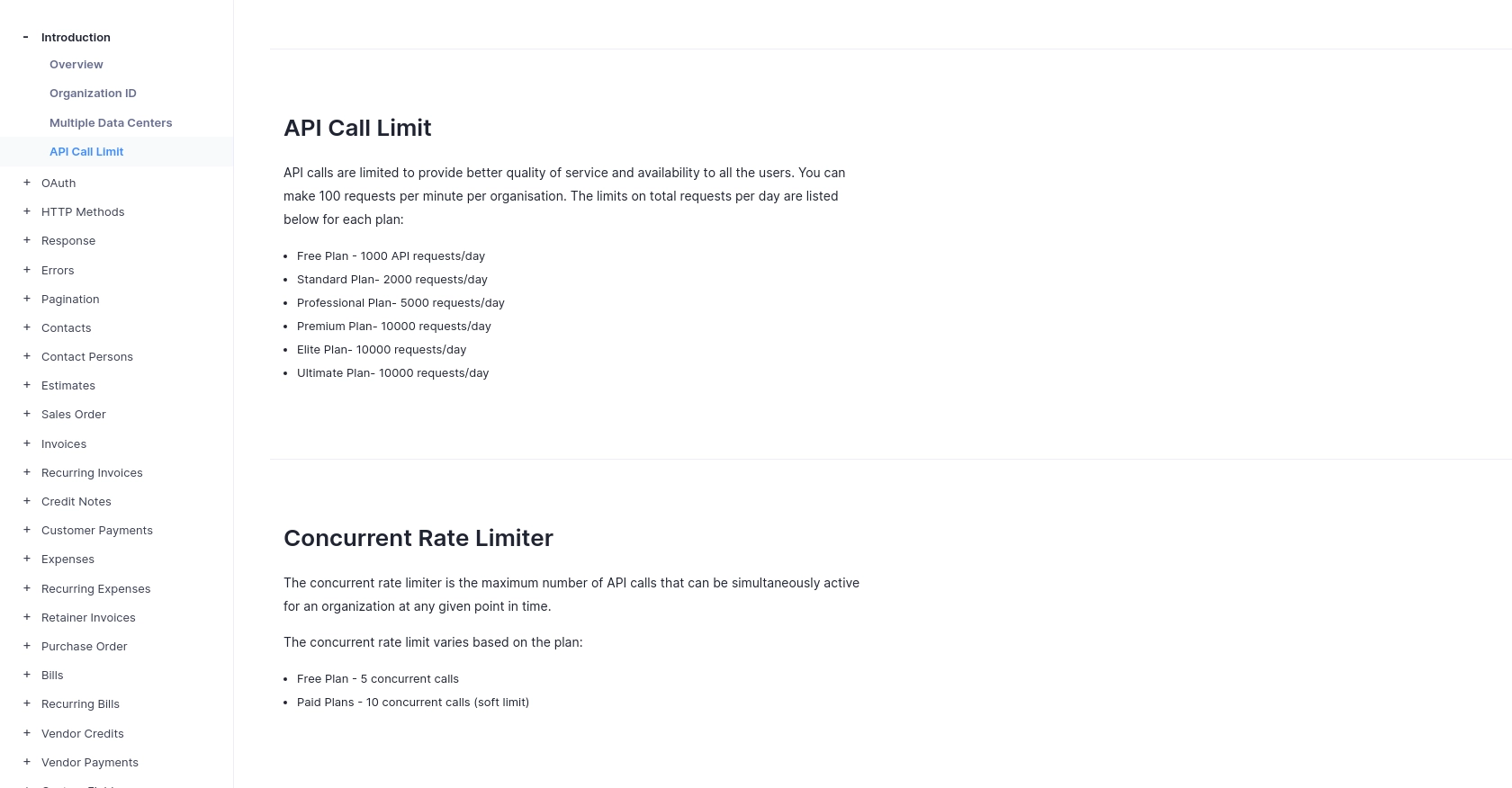
sbb-itb-96038d7
Making API Calls to Zoho Books for Sales Order Management Using PHP
To interact with the Zoho Books API for creating or updating sales orders, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the API.
Setting Up Your PHP Environment for Zoho Books API Integration
Before you start coding, ensure that your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled to make HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
.
Installing Required PHP Libraries for Zoho Books API
To simplify HTTP requests, you can use the Guzzle library. Install it via Composer:
composer require guzzlehttp/guzzle
Creating a Sales Order in Zoho Books Using PHP
Now, let's write a PHP script to create a sales order in Zoho Books. Replace placeholders with your actual data and credentials.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://www.zohoapis.com/books/v3/salesorders', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'customer_id' => '460000000017138',
'currency_id' => '460000000000097',
'line_items' => [
[
'item_id' => '460000000017088',
'quantity' => 10,
'rate' => 100
]
]
]
]);
echo $response->getBody();
This script sends a POST request to the Zoho Books API to create a sales order. Ensure you replace YOUR_ACCESS_TOKEN
with your actual access token.
Updating a Sales Order in Zoho Books Using PHP
To update an existing sales order, modify the script to use a PUT request. Here's an example:
$response = $client->put('https://www.zohoapis.com/books/v3/salesorders/{salesorder_id}', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
],
'json' => [
'line_items' => [
[
'line_item_id' => '460000000039131',
'quantity' => 20,
'rate' => 90
]
]
]
]);
echo $response->getBody();
Replace {salesorder_id}
with the ID of the sales order you wish to update. This script updates the quantity and rate of a line item in the sales order.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. Check the status code and handle any errors:
if ($response->getStatusCode() == 200) {
echo "Success: " . $response->getBody();
} else {
echo "Error: " . $response->getStatusCode() . " - " . $response->getBody();
}
Zoho Books uses standard HTTP status codes to indicate success or failure. Refer to the Zoho Books API documentation for detailed error codes and their meanings.
With these steps, you can efficiently manage sales orders in Zoho Books using PHP. Be sure to test thoroughly in your sandbox environment before deploying to production.
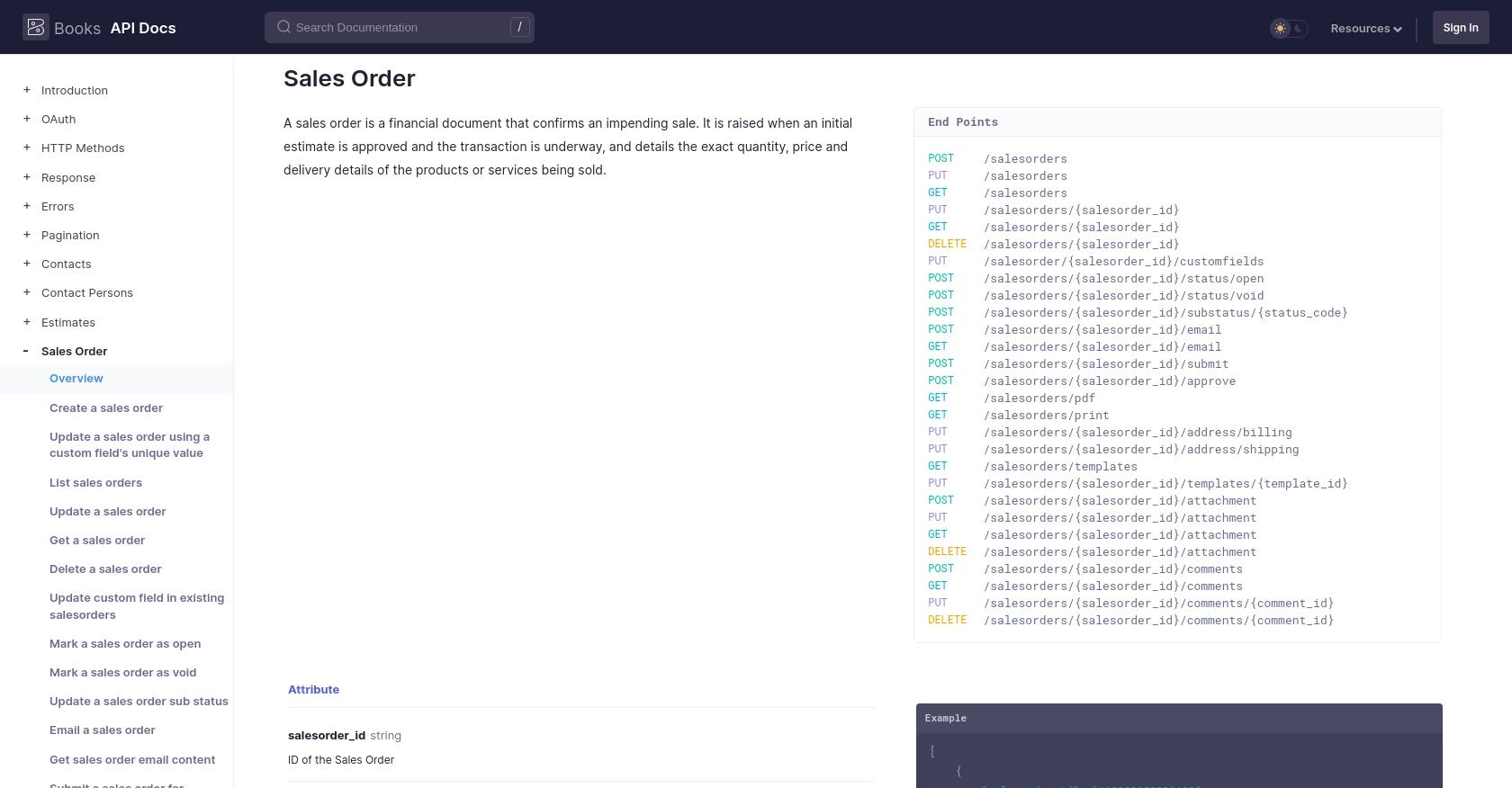
Conclusion and Best Practices for Zoho Books API Integration in PHP
Integrating with the Zoho Books API to manage sales orders using PHP can significantly enhance your business operations by automating and streamlining processes. By following the steps outlined in this article, you can efficiently create and update sales orders, ensuring that your sales data is always synchronized and accurate.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Zoho Books API documentation.
- Manage Error Responses: Always check the HTTP status codes and handle errors appropriately. Refer to the Zoho Books API error documentation for guidance on handling specific error codes.
- Optimize Data Handling: Ensure that data fields are standardized and transformed as needed to maintain consistency across your systems.
Leverage Endgrate for Simplified Integration Management
If managing multiple integrations becomes overwhelming, consider using Endgrate to streamline your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Zoho Books, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can enhance your integration strategy and improve operational efficiency.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/sales-order/#overview
Ready to get started?