Using the Pendo API to Get Page Views in PHP
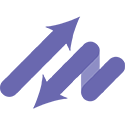
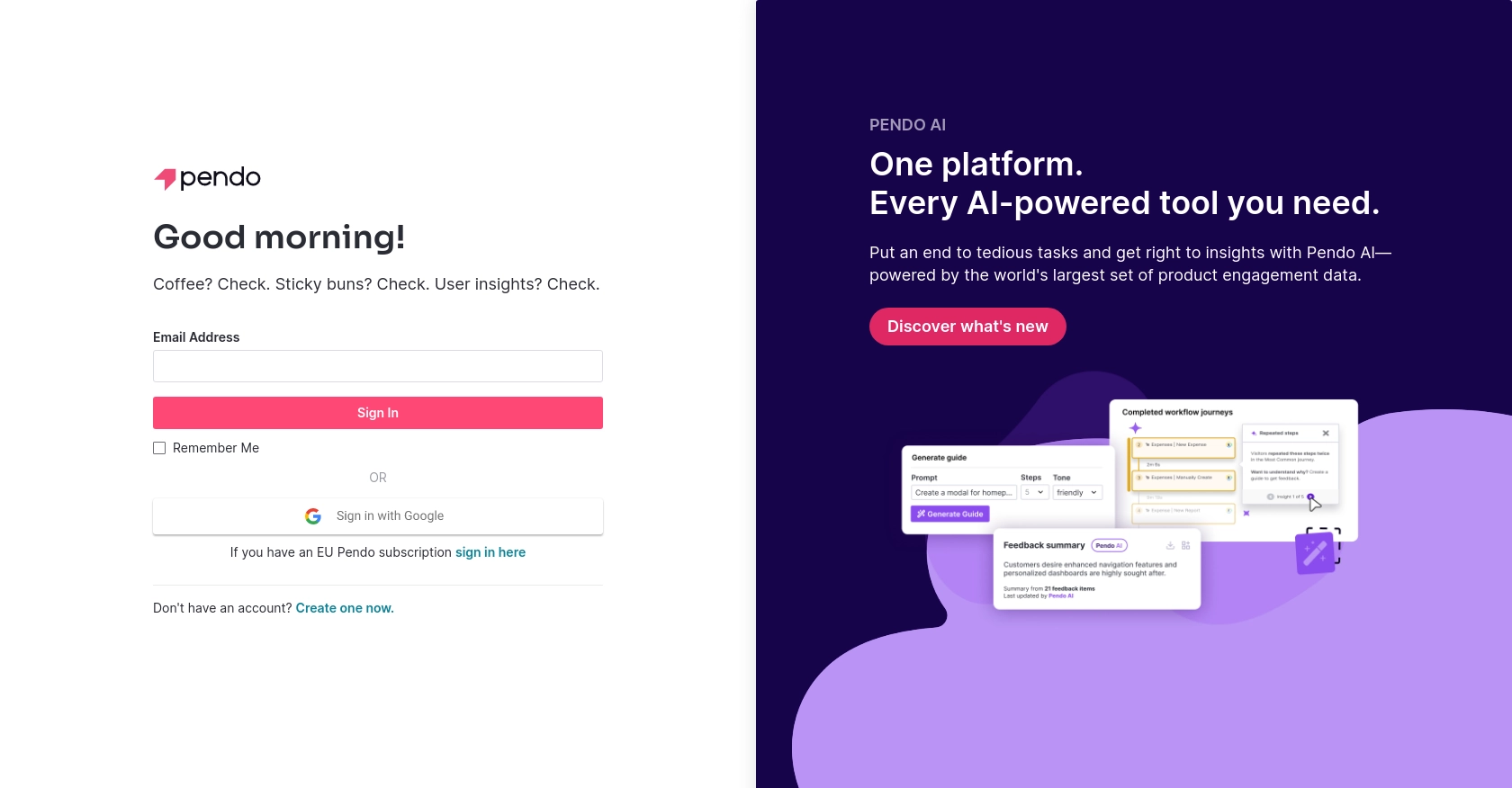
Introduction to Pendo API
Pendo is a powerful platform that helps businesses understand and guide user behavior within their applications. By providing insights into user interactions, Pendo enables companies to enhance user experiences, drive product adoption, and increase customer satisfaction.
Integrating with the Pendo API allows developers to access valuable analytics data, such as page views, which can be crucial for understanding user engagement. For example, a developer might use the Pendo API to retrieve page view data and analyze which features are most popular among users, helping to inform product development and marketing strategies.
Setting Up Your Pendo Test Account for API Access
Before you can start using the Pendo API to retrieve page view data, you'll need to set up a Pendo account. This will allow you to generate the necessary API key for authentication. Follow these steps to get started:
Step 1: Sign Up for a Pendo Account
If you don't already have a Pendo account, visit the Pendo website and sign up for a free trial or demo account. This will give you access to the platform's features and allow you to explore its capabilities.
Step 2: Accessing the Pendo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps:
- Log in to your Pendo account.
- Navigate to the settings section of your dashboard.
- Locate the API settings and generate a new API key.
- Copy the API key and store it securely, as you'll need it for making API calls.
Step 3: Understanding Pendo API Authentication
The Pendo API uses API key-based authentication. This means you'll need to include the API key in the headers of your HTTP requests to authenticate and access the data. Ensure that your API key is kept confidential and not exposed in client-side code.
With your Pendo account and API key ready, you can now proceed to make API calls to retrieve page view data using PHP. In the next section, we'll explore how to set up your PHP environment and make the necessary API calls.
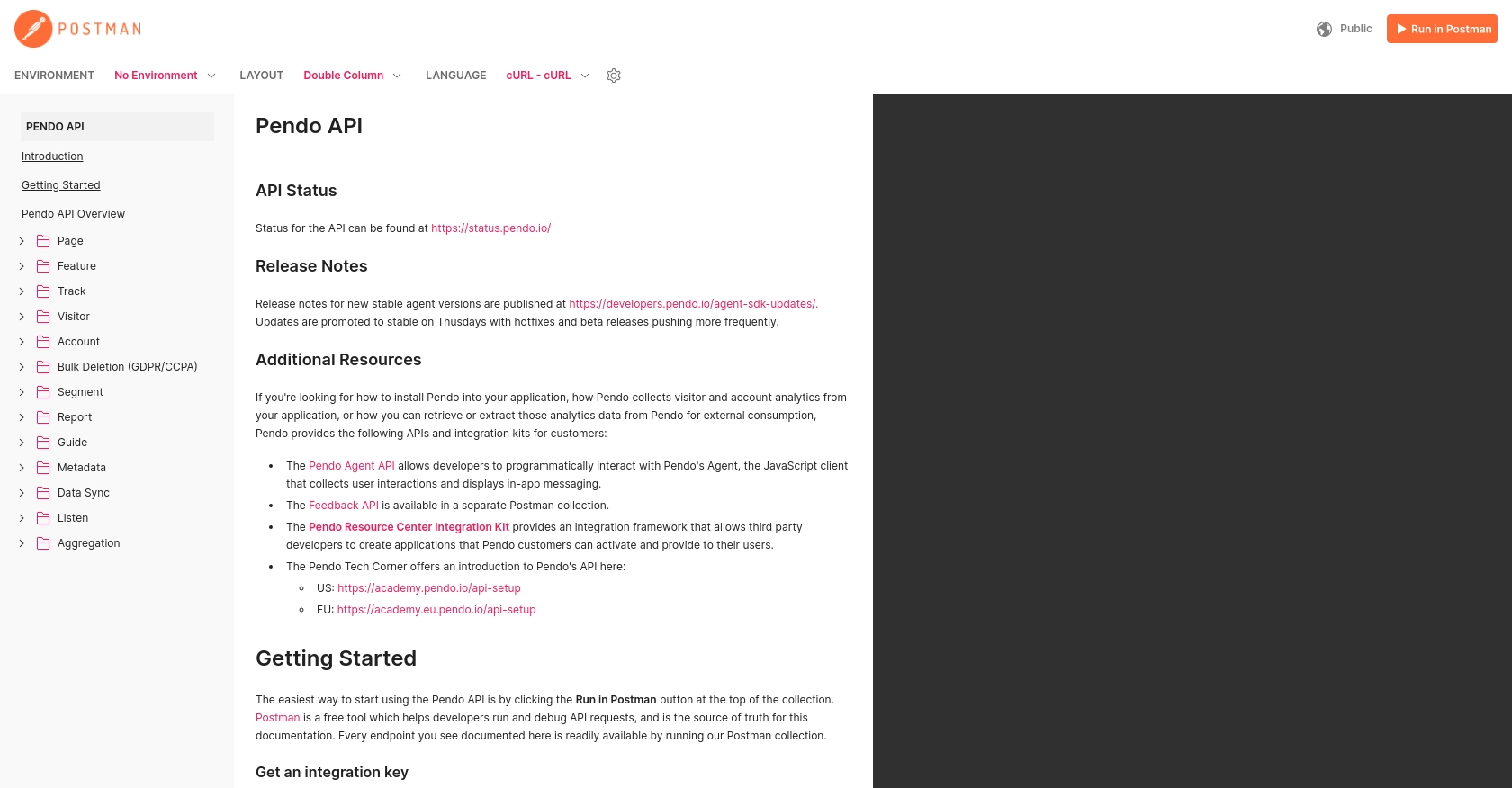
sbb-itb-96038d7
Making API Calls to Retrieve Pendo Page Views Using PHP
To interact with the Pendo API and retrieve page view data, you'll need to set up your PHP environment and make HTTP requests. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for Pendo API Integration
Before making API calls, ensure your development environment is ready. You'll need:
- PHP version 7.4 or higher
- Composer for dependency management
To install Composer, follow the instructions on the Composer website.
Installing Required PHP Dependencies
You'll need the Guzzle HTTP client to make HTTP requests. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Pendo Page Views
With your environment set up, you can now write the PHP code to call the Pendo API. Create a file named get_pendo_page_views.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://engageapi.pendo.io/api/v1/pageViews';
$headers = [
'Authorization' => 'api_key YOUR_API_KEY',
'Content-Type' => 'application/json'
];
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Check if the request was successful
if ($response->getStatusCode() == 200) {
$data = json_decode($response->getBody(), true);
// Output the page view data
foreach ($data as $pageView) {
echo 'Page ID: ' . $pageView['id'] . ' - Views: ' . $pageView['views'] . "\n";
}
} else {
echo 'Failed to retrieve page views. Status code: ' . $response->getStatusCode();
}
Replace YOUR_API_KEY
with the API key you generated earlier. This code initializes a Guzzle client, sets the API endpoint and headers, and makes a GET request to retrieve page view data. If successful, it outputs the page ID and view count.
Running the PHP Script and Verifying Results
Run the script from the command line using:
php get_pendo_page_views.php
You should see the page view data printed in the terminal. Verify the data by checking your Pendo dashboard to ensure it matches the retrieved information.
Handling Errors and Understanding Pendo API Response Codes
When making API calls, it's crucial to handle potential errors. The Pendo API may return various HTTP status codes indicating success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your API key.
- 404 Not Found: The requested resource doesn't exist.
- 500 Internal Server Error: An error occurred on the server.
Implement error handling in your code to manage these scenarios gracefully and ensure robust integration.
Conclusion: Best Practices for Using the Pendo API in PHP
Integrating with the Pendo API to retrieve page view data in PHP can significantly enhance your ability to analyze user engagement and improve product development strategies. By following the steps outlined in this guide, you can efficiently set up your PHP environment, authenticate API requests, and handle responses effectively.
Storing Pendo API Credentials Securely
It's crucial to store your API key securely to prevent unauthorized access. Consider using environment variables or secure vaults to manage sensitive information, ensuring that your API key is not exposed in your codebase.
Handling Pendo API Rate Limits
While interacting with the Pendo API, be mindful of any rate limits that may apply. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay or queuing requests to prevent exceeding limits.
Transforming and Standardizing Pendo Data
When retrieving data from the Pendo API, consider transforming and standardizing the data fields to fit your application's needs. This can involve mapping Pendo's data structure to your internal models or aggregating data for more comprehensive analysis.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, Endgrate offers a unified API solution. By leveraging Endgrate, you can save time and resources, focusing on your core product while outsourcing complex integrations. Endgrate's intuitive platform allows you to build once for each use case, providing a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?