How to Create Task with the Wrike API in PHP
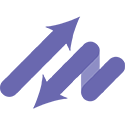
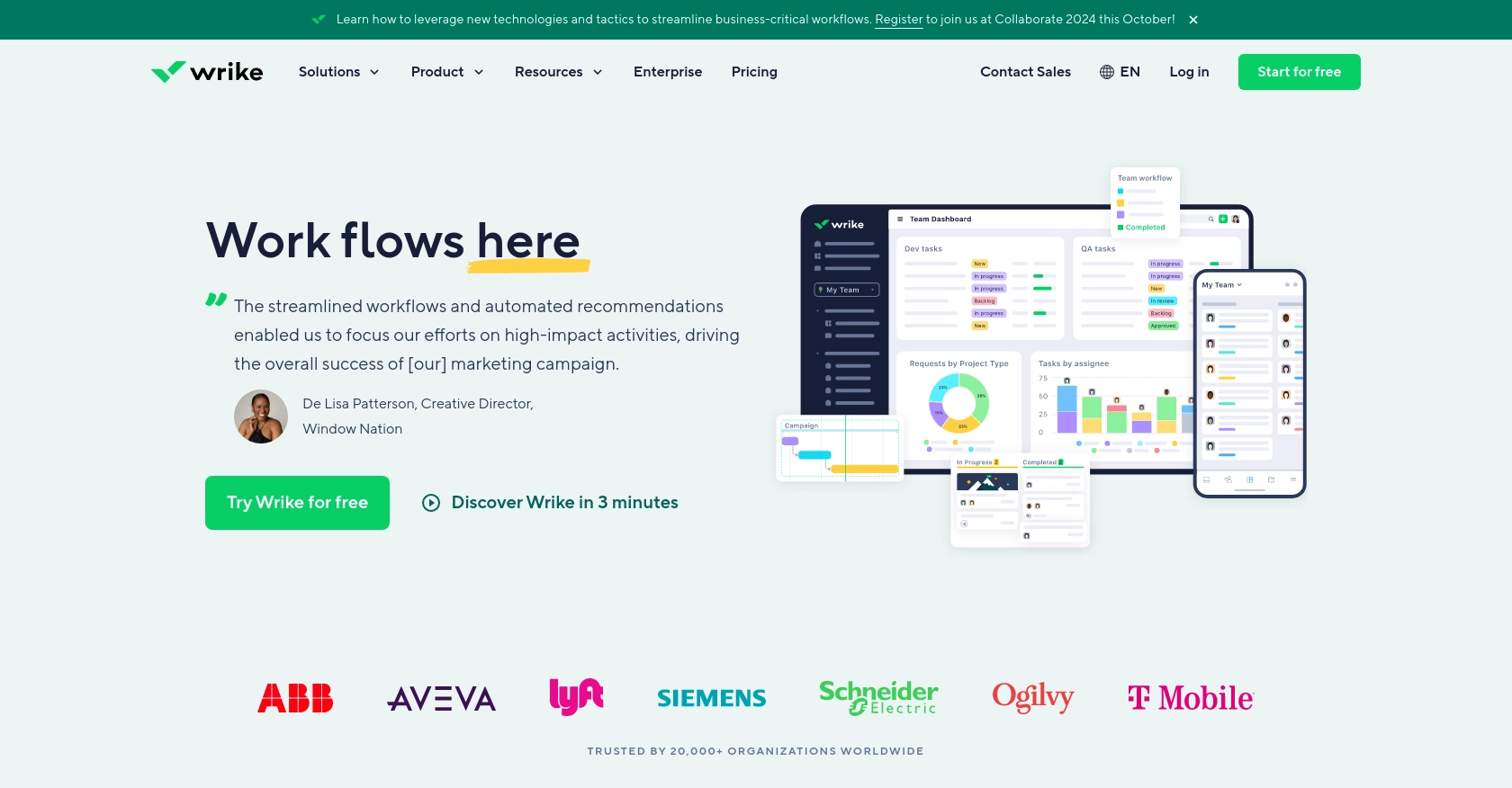
Introduction to Wrike API Integration
Wrike is a robust project management and collaboration platform that empowers teams to streamline their workflows and enhance productivity. With features like task management, time tracking, and resource allocation, Wrike is an essential tool for businesses aiming to optimize their project execution.
For developers, integrating with the Wrike API offers the opportunity to automate and customize task management processes. By creating tasks programmatically, you can enhance efficiency and ensure seamless integration with other systems. For example, you might automate the creation of tasks in Wrike based on events in another application, such as a new customer inquiry in a CRM system.
Setting Up Your Wrike Test/Sandbox Account for API Integration
Before diving into the integration process, it's crucial to set up a Wrike test or sandbox account. This environment allows you to experiment with the API without affecting your live data, ensuring a safe and controlled development process.
Creating a Wrike Sandbox Account
To begin, you'll need to create a Wrike account if you haven't already. Wrike offers a free trial that you can use as a sandbox environment:
- Visit the Wrike website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Wrike account to access the dashboard.
Setting Up OAuth 2.0 Authentication for Wrike API
Wrike uses OAuth 2.0 for authentication, which requires you to create an application within your Wrike account to obtain the necessary credentials:
- Navigate to the Apps & Integrations section in your Wrike dashboard.
- Click on Create New App and provide the required details, such as the app name and description.
- Once the app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure, as they are essential for authenticating API requests.
Generating Access Tokens for Wrike API Requests
With your app set up, you can now generate access tokens to authenticate your API requests:
- Direct users to the Wrike authorization URL, including your Client ID and redirect URI.
- Upon user authorization, Wrike will redirect to your specified URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Wrike's token endpoint, including your Client ID, Client Secret, and authorization code.
For detailed steps, refer to the Wrike OAuth 2.0 documentation.
With your sandbox account and OAuth 2.0 authentication set up, you're ready to start integrating with the Wrike API and creating tasks programmatically.
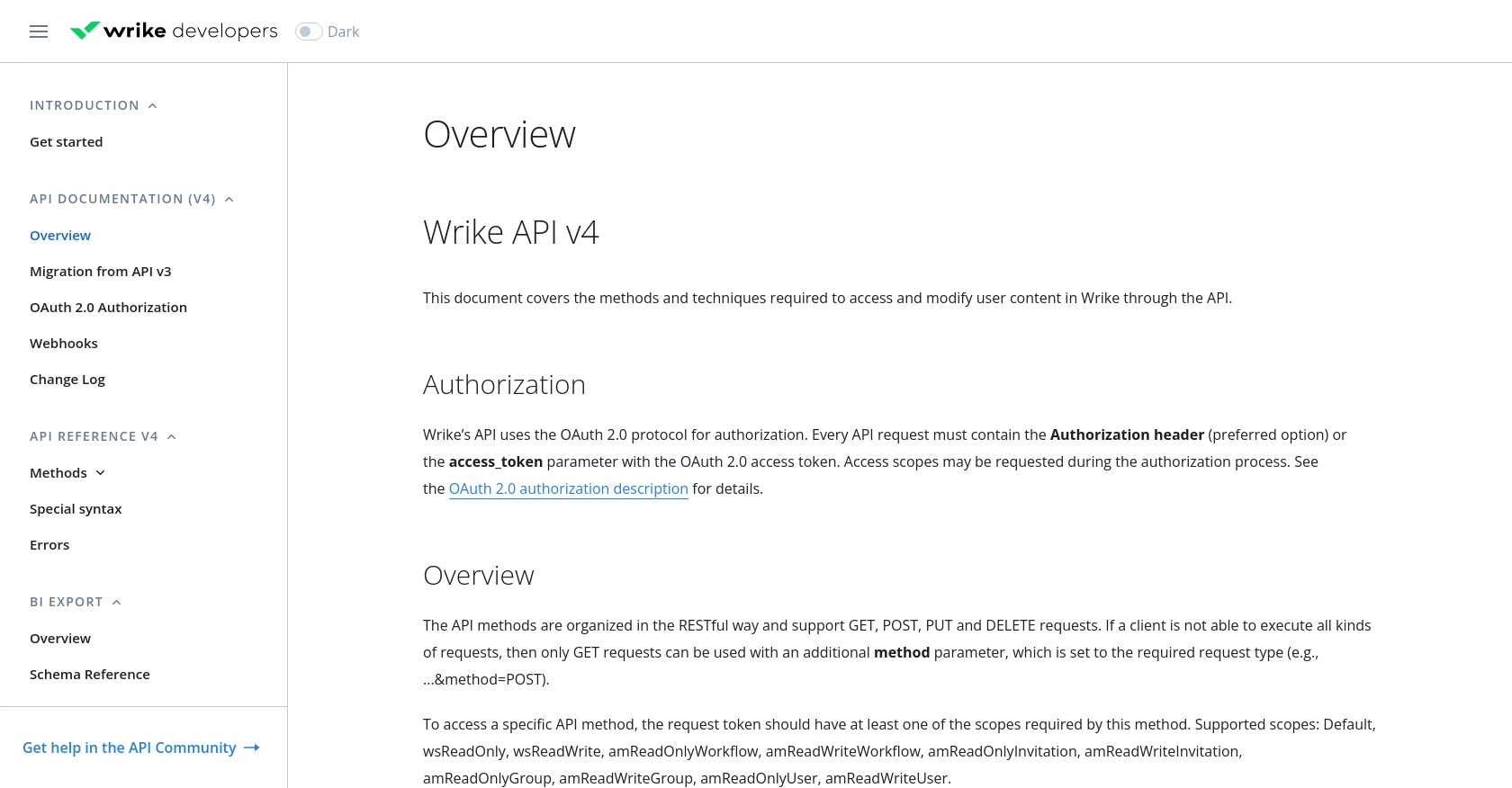
sbb-itb-96038d7
Making API Calls to Create Tasks in Wrike Using PHP
To interact with the Wrike API and create tasks programmatically, you'll use PHP to send HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Wrike API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Install PHP 7.4 or later on your system.
- Ensure you have
cURL
enabled, as it is required to send HTTP requests. - Optionally, use Composer to manage dependencies, though it's not mandatory for this tutorial.
Writing PHP Code to Create a Task in Wrike
With your environment ready, you can now write the PHP code to create a task in Wrike. Follow these steps:
<?php
// Set your access token and folder ID
$accessToken = 'Your_Access_Token';
$folderId = 'Your_Folder_ID';
// Define the API endpoint
$url = "https://www.wrike.com/api/v4/folders/$folderId/tasks";
// Set the task data
$data = [
'title' => 'New Task Title',
'description' => 'Task description goes here',
'status' => 'Active'
];
// Initialize cURL
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$responseData = json_decode($response, true);
echo 'Task Created: ' . $responseData['data'][0]['title'];
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
and Your_Folder_ID
with your actual Wrike access token and folder ID.
Verifying the Task Creation in Wrike
After running the PHP script, you should verify that the task was successfully created:
- Log in to your Wrike account and navigate to the specified folder.
- Check for the new task with the title you specified in the script.
Handling Errors and Wrike API Response Codes
When making API calls, it's crucial to handle potential errors. The Wrike API may return various HTTP status codes indicating success or failure:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
For more detailed error handling, refer to the Wrike API error documentation.
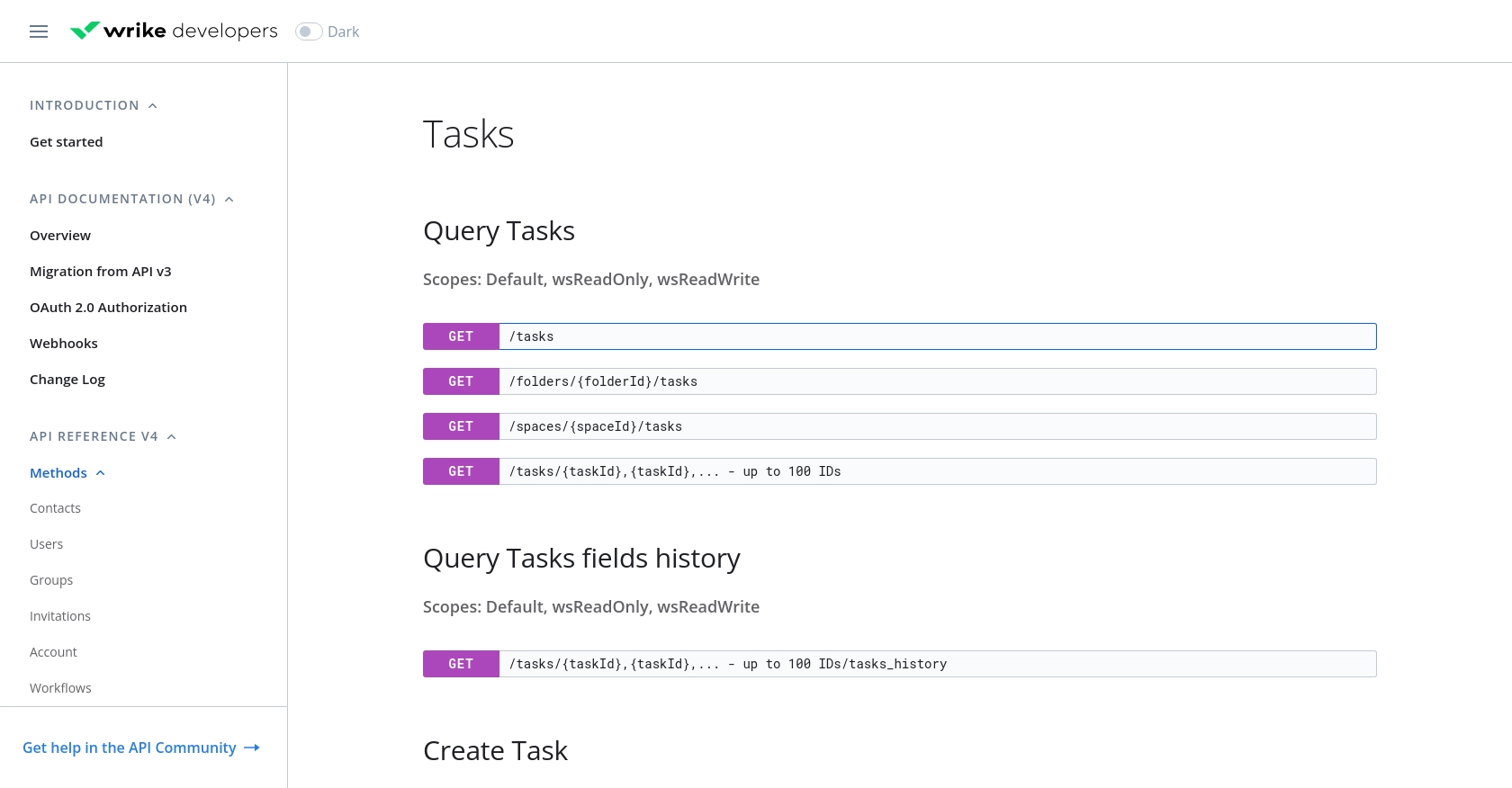
Best Practices for Wrike API Integration in PHP
When integrating with the Wrike API using PHP, it's essential to follow best practices to ensure secure and efficient interactions. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Wrike's API may impose rate limits to prevent abuse. Be sure to implement logic to handle HTTP 429 Too Many Requests responses by pausing requests and retrying after a specified interval. For more details, refer to the Wrike API documentation.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized to match your application's requirements. This includes converting date formats, normalizing text fields, and mapping custom fields appropriately.
Enhancing Your Integration Strategy with Endgrate
While integrating with the Wrike API can significantly enhance your task management processes, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a difference.
Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Wrike. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integration development and maintenance to Endgrate.
- Build Once, Deploy Everywhere: Create a single integration for each use case and apply it across multiple platforms without redundant development efforts.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience, reducing friction and improving satisfaction.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?