How to Get Records with the Postgres API in PHP
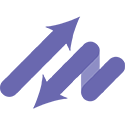
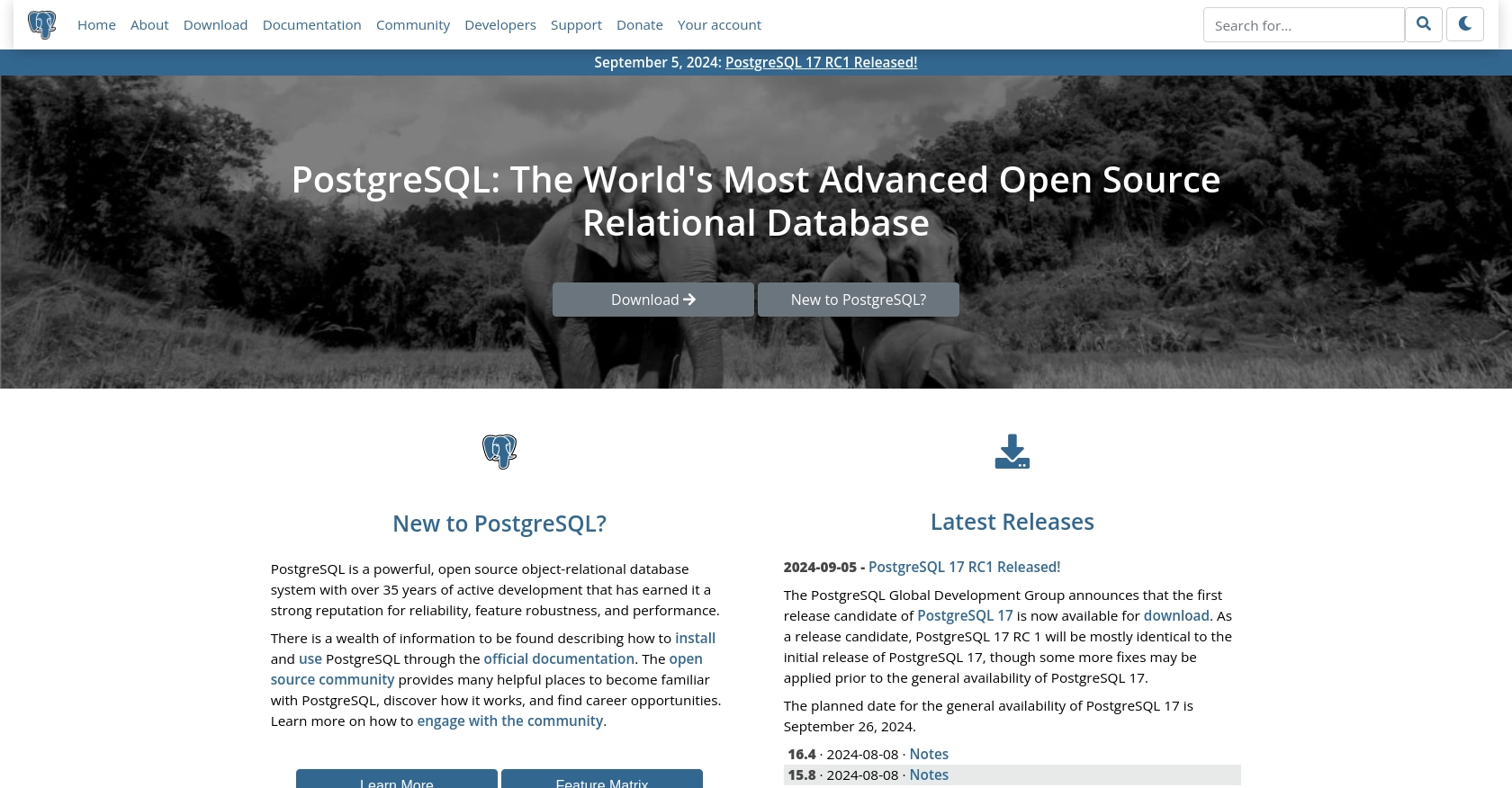
Introduction to PostgreSQL API Integration
PostgreSQL, often referred to as Postgres, is a powerful, open-source relational database management system known for its robustness, scalability, and extensive feature set. It supports advanced data types and performance optimization features, making it a popular choice for developers and businesses seeking a reliable database solution.
Integrating with the PostgreSQL API allows developers to interact programmatically with their databases, enabling automated data retrieval and manipulation. For example, a developer might use the PostgreSQL API to fetch records from a database to display on a web application, facilitating real-time data updates and enhancing user experience.
Setting Up a Test Environment for PostgreSQL API Integration
Before diving into PostgreSQL API integration, it's crucial to set up a test environment. This allows developers to experiment and test their code without affecting the production database. PostgreSQL offers several ways to create a sandbox environment, ensuring a safe space for development and testing.
Installing PostgreSQL Locally
To begin, you can install PostgreSQL on your local machine. This setup provides a controlled environment where you can create and manage databases without external dependencies. Follow these steps to install PostgreSQL:
- Visit the PostgreSQL download page and choose the appropriate version for your operating system.
- Follow the installation instructions provided for your platform.
- Once installed, use the PostgreSQL command-line tool,
psql
, to create a new database for testing purposes.
Creating a PostgreSQL Sandbox Database
After installing PostgreSQL, you can create a sandbox database to test your API interactions. Use the following command in the psql
tool to create a new database:
CREATE DATABASE test_db;
This command creates a new database named test_db
, which you can use for testing API calls and data manipulation.
Setting Up Authentication for PostgreSQL
PostgreSQL uses a custom authentication method to secure database access. To set up authentication, follow these steps:
- Edit the
pg_hba.conf
file, located in the PostgreSQL data directory, to configure client authentication. - Add an entry to allow local connections using a password, such as:
# TYPE DATABASE USER ADDRESS METHOD
local all all md5
- Restart the PostgreSQL server to apply changes.
- Create a new user with a password for API access using the following command:
CREATE USER api_user WITH PASSWORD 'securepassword';
Ensure that the user has the necessary permissions to access the test database:
GRANT ALL PRIVILEGES ON DATABASE test_db TO api_user;
Connecting PHP to PostgreSQL
With the sandbox environment ready, you can now connect your PHP application to the PostgreSQL database. Ensure you have the necessary PHP extensions installed, such as pdo_pgsql
or pg_connect
, to facilitate the connection.
Here's a sample PHP code snippet to connect to the PostgreSQL database:
<?php
$host = 'localhost';
$db = 'test_db';
$user = 'api_user';
$pass = 'securepassword';
$dsn = "pgsql:host=$host;dbname=$db";
try {
$pdo = new PDO($dsn, $user, $pass, [PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION]);
echo "Connected to PostgreSQL successfully!";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
This code establishes a connection to the PostgreSQL database using the specified credentials. Ensure that the database and user details match those set up in your sandbox environment.
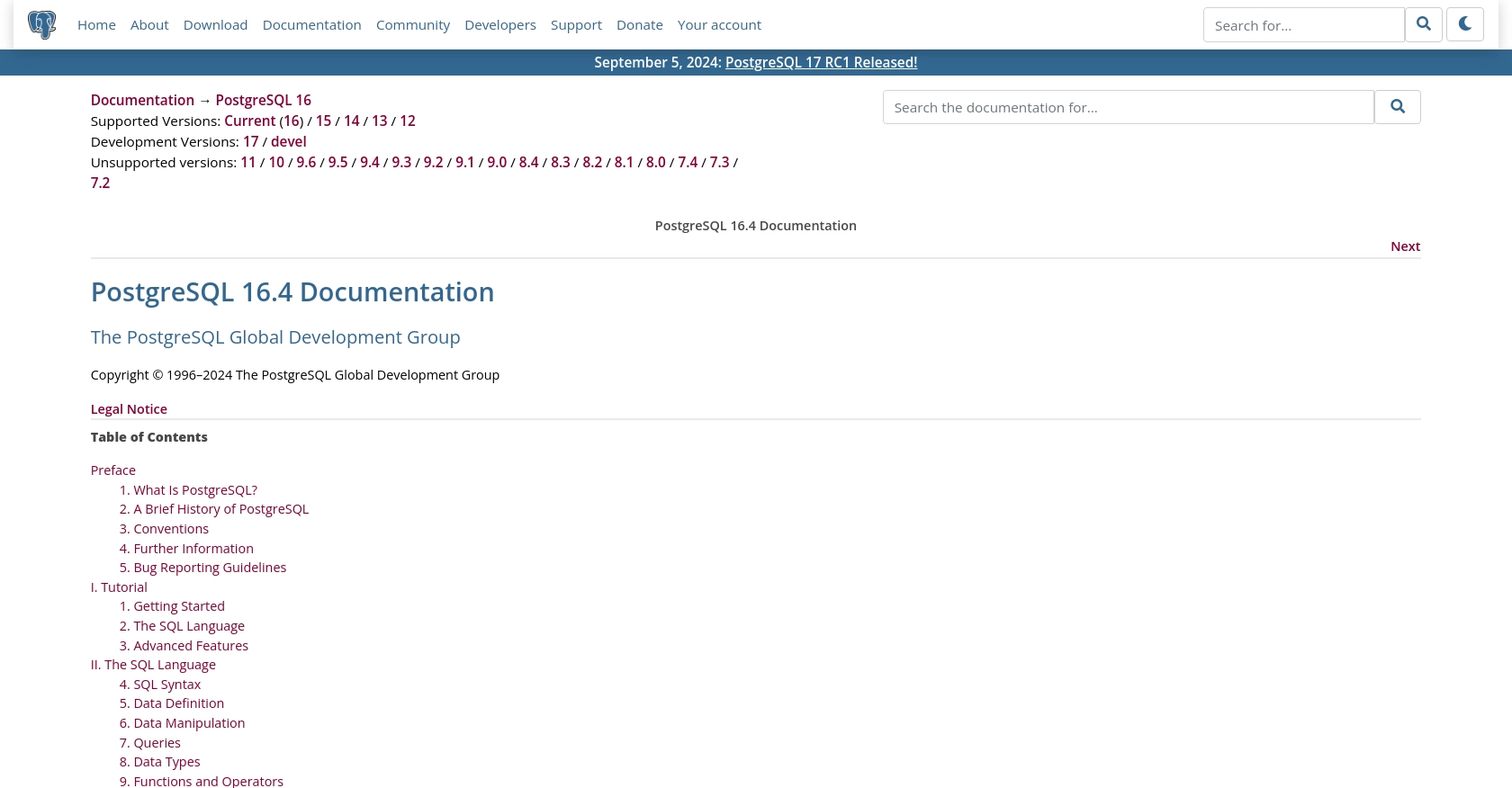
sbb-itb-96038d7
Making API Calls to Retrieve Records from PostgreSQL Using PHP
Once your PostgreSQL environment is set up and connected to your PHP application, you can proceed to make API calls to retrieve records. This section will guide you through the process of querying the PostgreSQL database using PHP, ensuring you can efficiently access and manipulate your data.
Prerequisites for PHP and PostgreSQL Integration
Before making API calls, ensure you have the following prerequisites in place:
- PHP version 7.4 or higher installed on your server.
- The
pdo_pgsql
extension enabled in your PHP configuration. - A PostgreSQL database with sample data for testing purposes.
Writing PHP Code to Fetch Records from PostgreSQL
To retrieve records from your PostgreSQL database, you can use a simple SQL query within your PHP script. Below is a sample code snippet to fetch data:
<?php
$host = 'localhost';
$db = 'test_db';
$user = 'api_user';
$pass = 'securepassword';
$dsn = "pgsql:host=$host;dbname=$db";
try {
$pdo = new PDO($dsn, $user, $pass, [PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION]);
// Define the SQL query to fetch records
$sql = 'SELECT * FROM your_table_name';
// Execute the query
$stmt = $pdo->query($sql);
// Fetch the records
$records = $stmt->fetchAll(PDO::FETCH_ASSOC);
// Display the records
foreach ($records as $record) {
echo 'Record: ' . implode(', ', $record) . "<br>";
}
} catch (PDOException $e) {
echo "Error fetching records: " . $e->getMessage();
}
?>
Replace your_table_name
with the actual name of the table you wish to query. This code connects to the PostgreSQL database, executes a SELECT query, and fetches all records from the specified table.
Verifying Successful API Calls and Handling Errors
After executing the PHP script, verify that the records are correctly retrieved by checking the output. If the query is successful, you should see the records displayed in your browser or console.
In case of errors, the script will catch exceptions and display an error message. Common issues might include incorrect database credentials or syntax errors in the SQL query. Ensure that your database connection details are accurate and that your SQL query is correctly formatted.
Best Practices for Efficient API Calls to PostgreSQL
- Use prepared statements to prevent SQL injection attacks and enhance security.
- Limit the number of records fetched by using SQL clauses like
LIMIT
andOFFSET
to improve performance. - Regularly update your PHP and PostgreSQL versions to benefit from the latest features and security patches.
Conclusion and Best Practices for PostgreSQL API Integration in PHP
Integrating with the PostgreSQL API using PHP provides developers with a robust solution for managing and retrieving data efficiently. By following the steps outlined in this guide, you can set up a secure and effective connection to your PostgreSQL database, enabling seamless data interactions.
Best Practices for Secure and Efficient PostgreSQL API Usage
- Secure Credentials: Always store database credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Implement logic to handle potential rate limits by queuing requests or using exponential backoff strategies.
- Optimize Queries: Use indexing and query optimization techniques to enhance performance and reduce load times.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with PostgreSQL can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing integrations, allowing you to focus on your core product development. By leveraging Endgrate's unified API, you can simplify your integration processes, reduce development time, and provide a seamless experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today. Save time and resources by outsourcing integrations and focusing on what truly matters—your product's innovation and growth.
Read More
Ready to get started?