How to Get Payments with the Younium API in PHP
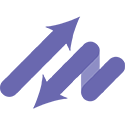
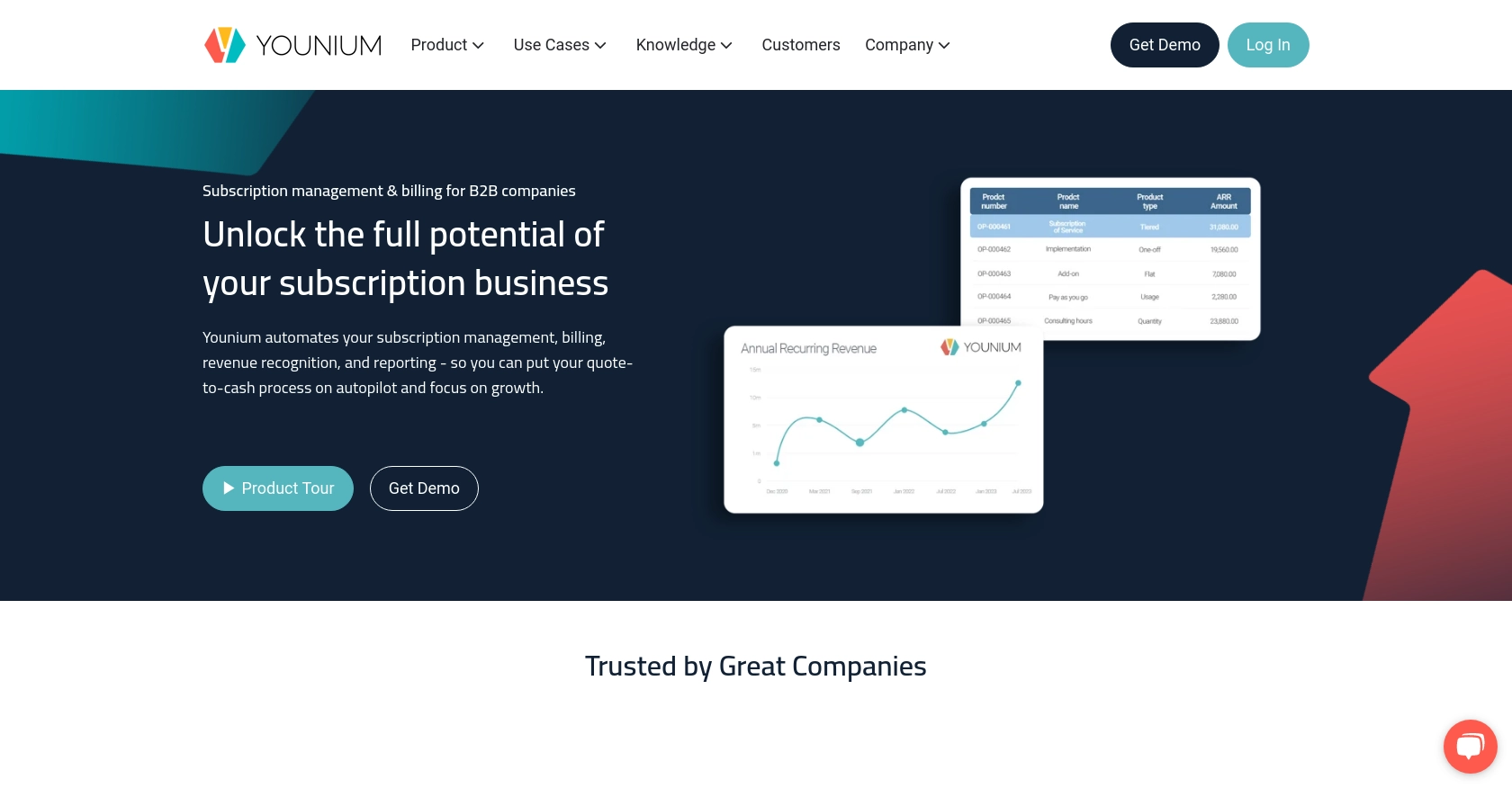
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform that empowers businesses to streamline their billing and subscription processes. With features designed to handle complex billing scenarios, Younium is a preferred choice for B2B SaaS companies looking to automate and optimize their financial operations.
Integrating with the Younium API allows developers to access and manage payment data efficiently. For example, a developer might use the Younium API to retrieve payment information and integrate it into a custom financial dashboard, providing real-time insights into revenue streams.
Setting Up Your Younium Test/Sandbox Account
Before you can start integrating with the Younium API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your production data. Follow the steps below to get started.
Create a Younium Sandbox Account
If you don't already have a Younium account, visit the Younium website and sign up for a sandbox account. This will give you access to a test environment where you can perform API operations without impacting live data.
Generate API Token and Client Credentials
Once your sandbox account is set up, you'll need to generate an API token and client credentials to authenticate your API requests. Follow these steps:
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select Privacy & Security from the dropdown menu.
- Navigate to the Personal Tokens section on the left panel and click Generate Token.
- Provide a relevant description for your token and click Create.
- Copy the generated Client ID and Secret Key. These credentials will not be visible again, so ensure you store them securely.
Acquire a JWT Access Token
With your client credentials ready, you can now acquire a JWT access token. This token is necessary for authenticating your API requests. Here's how to do it:
// Set the API endpoint for the sandbox environment
$url = 'https://api.sandbox.younium.com/auth/token';
// Prepare the request headers and body
$headers = [
'Content-Type: application/json'
];
$body = json_encode([
'clientId' => 'Your_Client_ID',
'secret' => 'Your_Secret_Key'
]);
// Initialize a cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and capture the response
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Decode the JSON response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. If successful, the response will include an accessToken
that you will use for subsequent API calls.
For more details on authentication, refer to the Younium Developer Documentation.
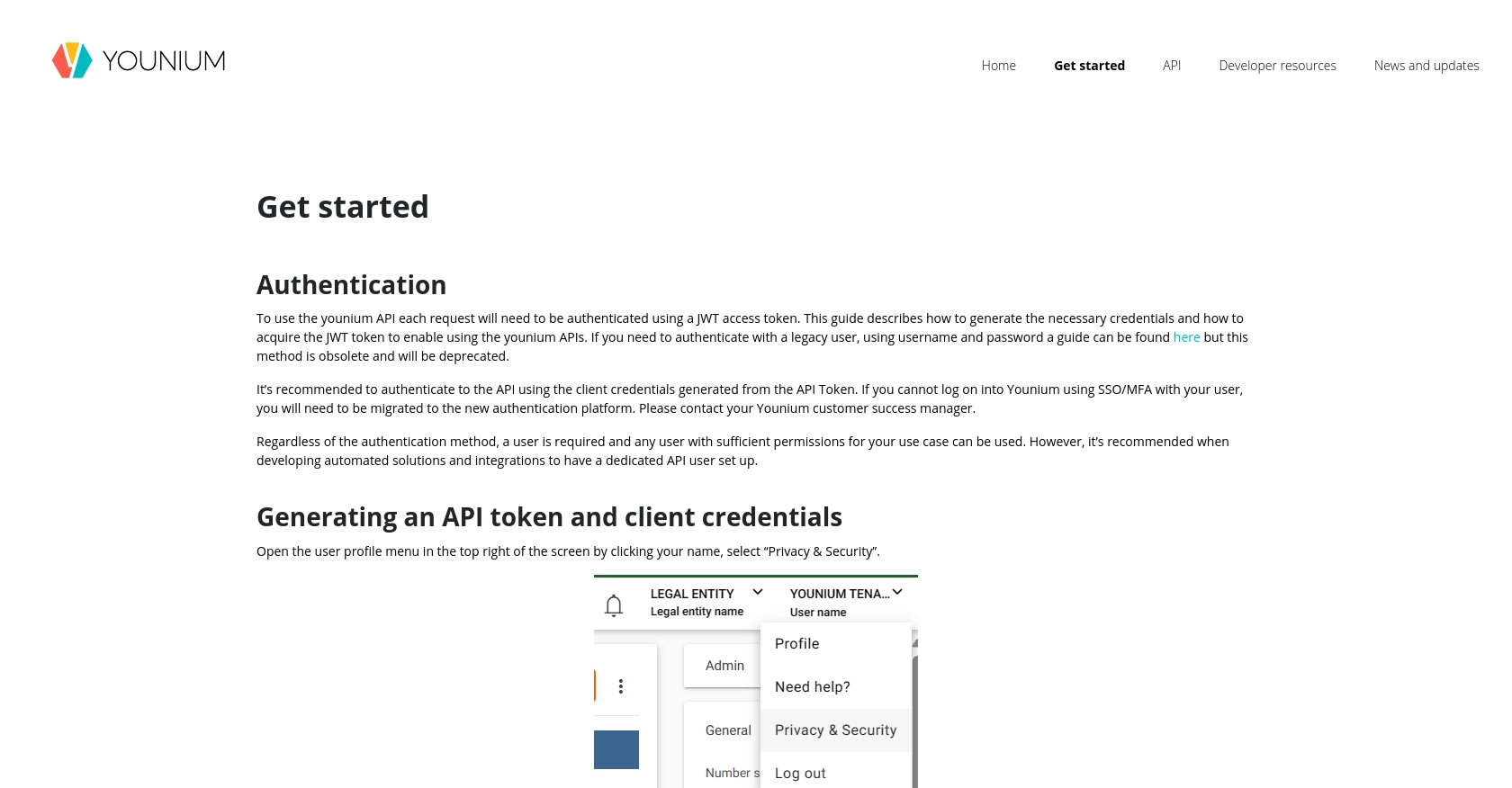
sbb-itb-96038d7
Making API Calls to Retrieve Payments with Younium in PHP
Now that you have your JWT access token, you can proceed to make API calls to the Younium platform. In this section, we'll guide you through the process of retrieving payment data using PHP. This is crucial for integrating payment information into your applications, providing insights into financial transactions.
Prerequisites for PHP Integration with Younium API
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- cURL extension enabled in your PHP setup.
Installing Required PHP Dependencies
To interact with the Younium API, you'll need to use the cURL library, which is typically included with PHP. Ensure it's enabled in your php.ini
file:
extension=curl
Example Code to Retrieve Payments from Younium API
Below is a sample PHP script to retrieve payment data from the Younium API:
// Set the API endpoint for retrieving payments
$url = 'https://api.sandbox.younium.com/payments';
// Prepare the request headers
$headers = [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json',
'api-version: 2.1'
];
// Initialize a cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and capture the response
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
}
// Close the cURL session
curl_close($ch);
// Decode the JSON response
$data = json_decode($response, true);
// Output the payment data
foreach ($data['data'] as $payment) {
echo 'Payment ID: ' . $payment['id'] . "\n";
echo 'Amount: ' . $payment['totalAmount'] . "\n";
echo 'Status: ' . $payment['status'] . "\n";
echo "\n";
}
Replace $accessToken
with the access token obtained earlier. This script sends a GET request to the Younium API to fetch payment data, which is then parsed and displayed.
Verifying Successful API Requests in Younium Sandbox
After running the script, verify the retrieved payment data by checking your Younium sandbox account. Ensure the data matches the expected results, confirming that the API call was successful.
Handling Errors and Common Error Codes
When making API calls, it's essential to handle potential errors. The Younium API may return the following error codes:
- 400 Bad Request: Indicates a malformed request. Check your request parameters and headers.
- 401 Unauthorized: Indicates an expired or invalid access token. Ensure your token is correct and not expired.
- 403 Forbidden: Indicates insufficient permissions or incorrect legal entity. Verify your permissions and legal entity settings.
For more detailed error handling, refer to the Younium API Documentation.
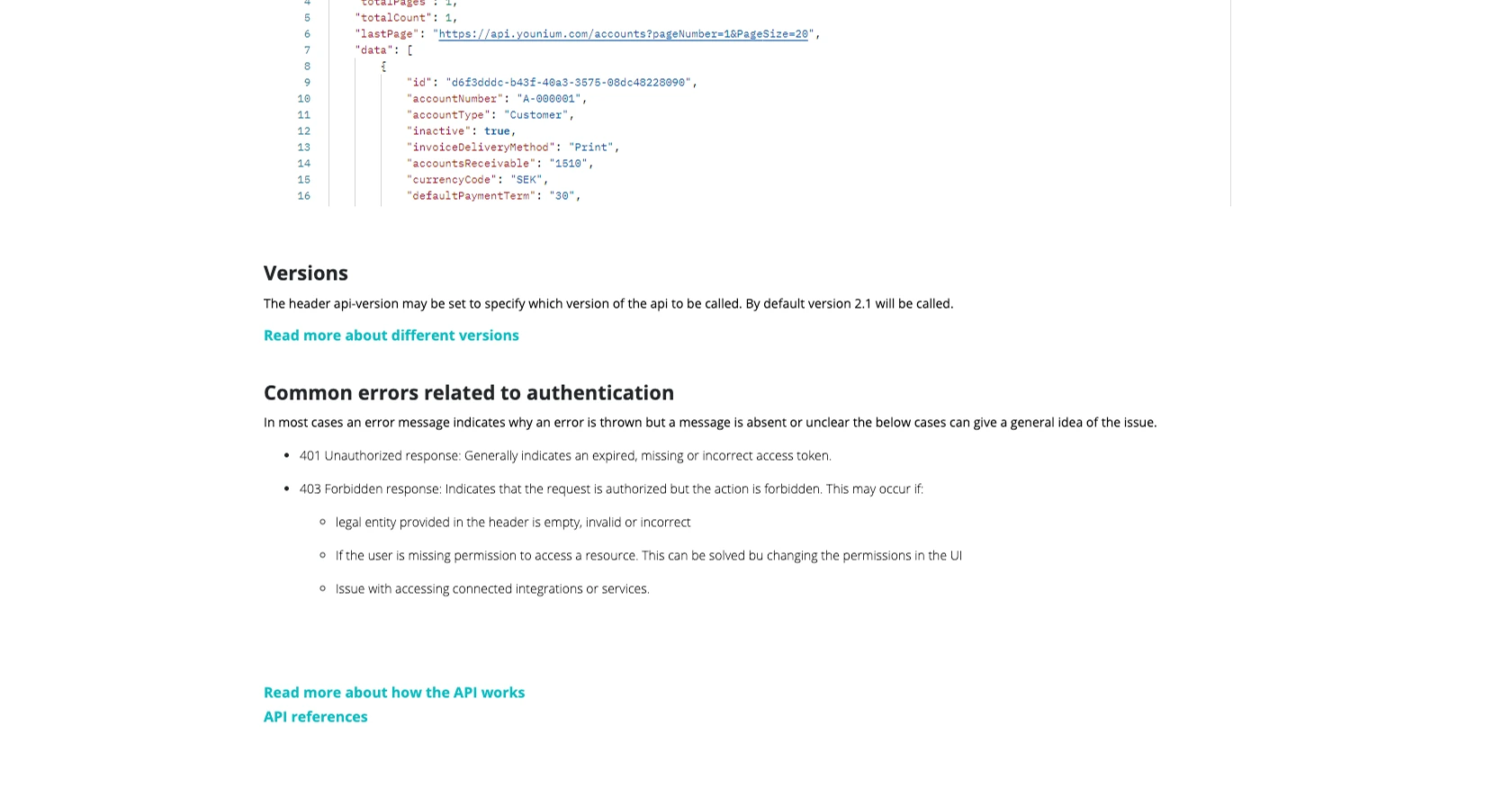
Conclusion and Best Practices for Younium API Integration in PHP
Integrating with the Younium API using PHP allows developers to efficiently manage payment data, enhancing financial insights and operational efficiency. By following the steps outlined in this guide, you can successfully authenticate and retrieve payment information from Younium's platform.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Store your Client ID, Secret Key, and JWT access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Younium API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the payment data retrieved from Younium is transformed and standardized to fit your application's data model, facilitating seamless integration.
- Regular Token Renewal: Since the JWT access token is valid for 24 hours, implement a mechanism to refresh the token automatically to maintain uninterrupted API access.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Younium. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration experience by visiting Endgrate's website.
By adhering to these best practices and utilizing tools like Endgrate, you can ensure a robust and efficient integration with the Younium API, driving value and insights for your business.
Read More
Ready to get started?