Using the Teamleader API to Get Companies (with Python examples)
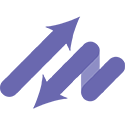
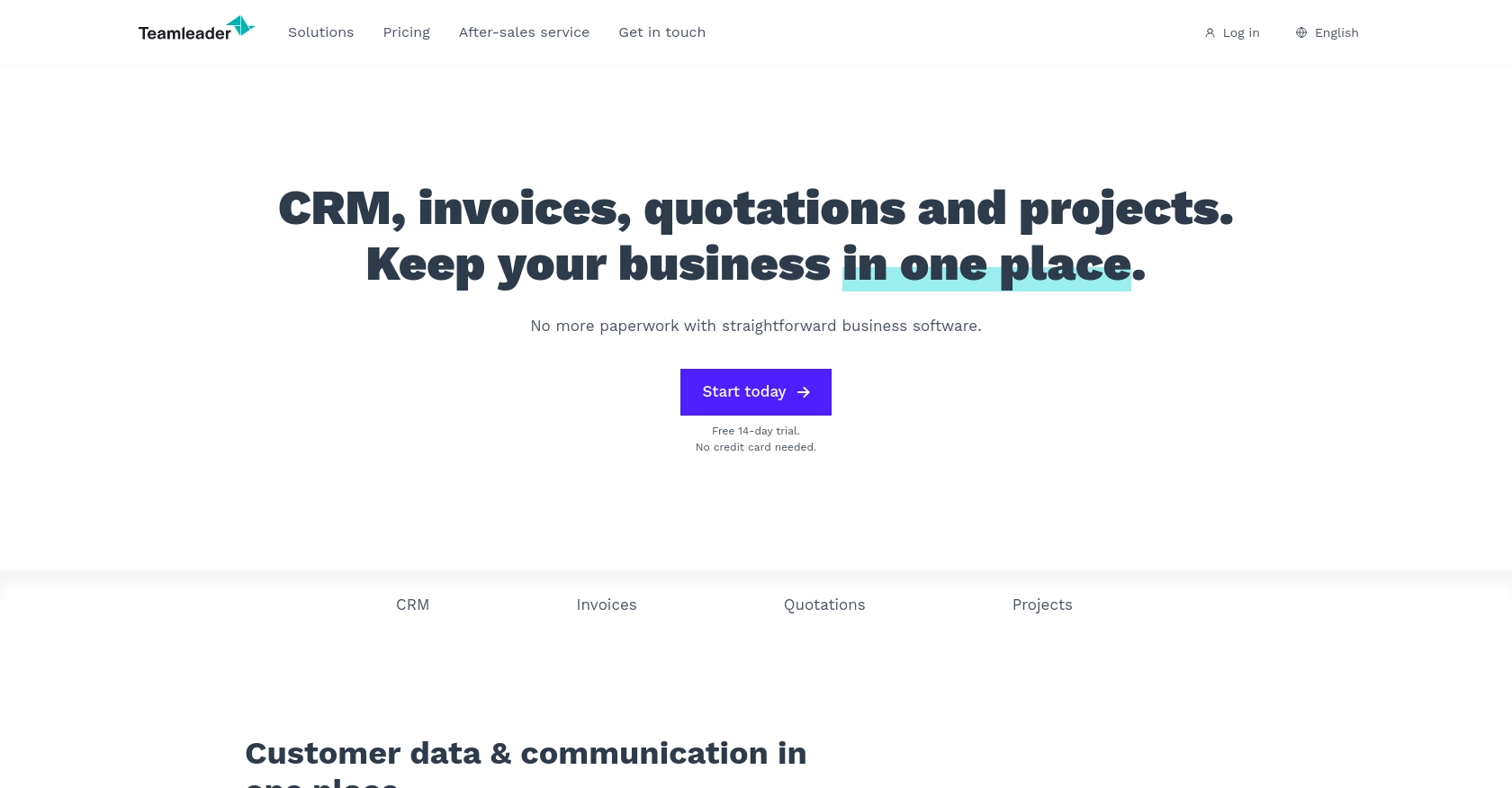
Introduction to Teamleader API
Teamleader is a versatile business management software designed to streamline operations for small and medium-sized enterprises. It offers a comprehensive suite of tools for CRM, project management, and invoicing, making it an essential platform for businesses looking to enhance productivity and efficiency.
Integrating with the Teamleader API allows developers to access and manage company data seamlessly. For example, you might want to retrieve a list of companies from Teamleader to synchronize with another CRM system or to generate detailed business reports. This integration can significantly enhance data consistency and operational workflows.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start interacting with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. This will give you access to the necessary features to test API interactions.
- Visit the Teamleader website and click on the sign-up button.
- Follow the on-screen instructions to create your account.
- Once your account is created, log in to access the dashboard.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication. Follow these steps to create an app and obtain the credentials needed for API access:
- Navigate to the developer section in your Teamleader account.
- Click on "Create a new app" to start the process.
- Fill in the required details such as app name, description, and redirect URI.
- After creating the app, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate your API requests:
- Use the OAuth 2.0 authorization flow to obtain an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint.
import requests
# Replace with your client ID and client secret
client_id = 'your_client_id'
client_secret = 'your_client_secret'
redirect_uri = 'your_redirect_uri'
authorization_code = 'your_authorization_code'
# Token endpoint
token_url = 'https://app.teamleader.eu/oauth2/access_token'
# Request payload
payload = {
'grant_type': 'authorization_code',
'client_id': client_id,
'client_secret': client_secret,
'redirect_uri': redirect_uri,
'code': authorization_code
}
# Make the POST request to get the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get('access_token')
print(f'Access Token: {access_token}')
Replace the placeholders with your actual client ID, client secret, redirect URI, and authorization code. The response will include an access token, which you'll use in subsequent API requests.
With your Teamleader account set up and access tokens ready, you're now prepared to make API calls to retrieve company data.
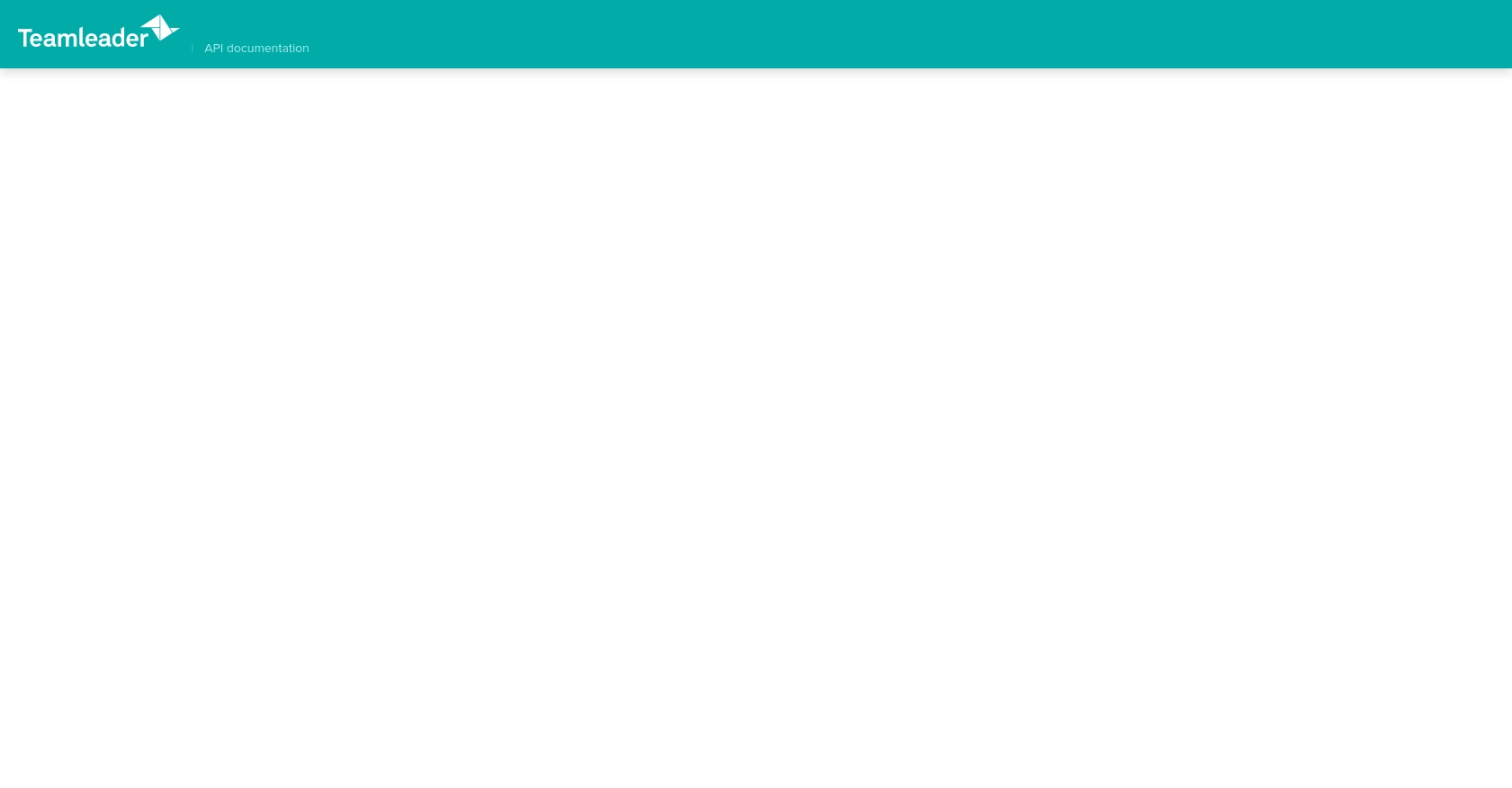
sbb-itb-96038d7
Making API Calls to Retrieve Companies Using Teamleader API with Python
With your Teamleader account and access tokens ready, you can now proceed to make API calls to retrieve company data. This section will guide you through the process of setting up your Python environment and executing the necessary API requests.
Setting Up Your Python Environment for Teamleader API Integration
Before making API calls, ensure you have the correct version of Python and necessary dependencies installed on your machine:
- Python 3.11.1
- The Python package installer
pip
Install the requests
library, which is essential for making HTTP requests:
pip install requests
Executing the API Call to Retrieve Companies from Teamleader
Now, let's write a Python script to retrieve a list of companies from Teamleader:
import requests
# Set the API endpoint for retrieving companies
endpoint = "https://api.teamleader.eu/companies.list"
# Set the request headers with the access token
headers = {
"Authorization": "Bearer your_access_token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
companies = response.json()
for company in companies:
print(company)
else:
print(f"Failed to retrieve companies: {response.status_code} - {response.text}")
Replace your_access_token
with the access token obtained earlier. This script sends a GET request to the Teamleader API endpoint for companies, using the access token for authentication. If successful, it prints the list of companies.
Verifying API Call Success and Handling Errors
After running the script, you should see the list of companies printed in your terminal. To verify the success of the API call, cross-check the returned data with the companies listed in your Teamleader sandbox account.
In case of errors, the script will output the status code and error message. Common error codes include:
- 401 Unauthorized: Check if your access token is correct and not expired.
- 403 Forbidden: Ensure your app has the necessary permissions to access company data.
- 500 Internal Server Error: This might be an issue on Teamleader's end; try again later.
For more detailed error handling, refer to the official Teamleader API documentation.
Conclusion and Best Practices for Using Teamleader API
Integrating with the Teamleader API to retrieve company data can significantly enhance your business operations by ensuring data consistency and improving workflow efficiency. By following the steps outlined in this guide, you can seamlessly connect to Teamleader and leverage its powerful features for your applications.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handling Rate Limits: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Teamleader is transformed and standardized to match your application's data model. This will facilitate smoother data integration and reporting.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Teamleader can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Teamleader.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. Endgrate's intuitive integration experience ensures that you build once for each use case, reducing the need for multiple integrations.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?