Using the Mailchimp API to Get Members in PHP
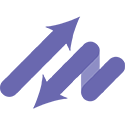
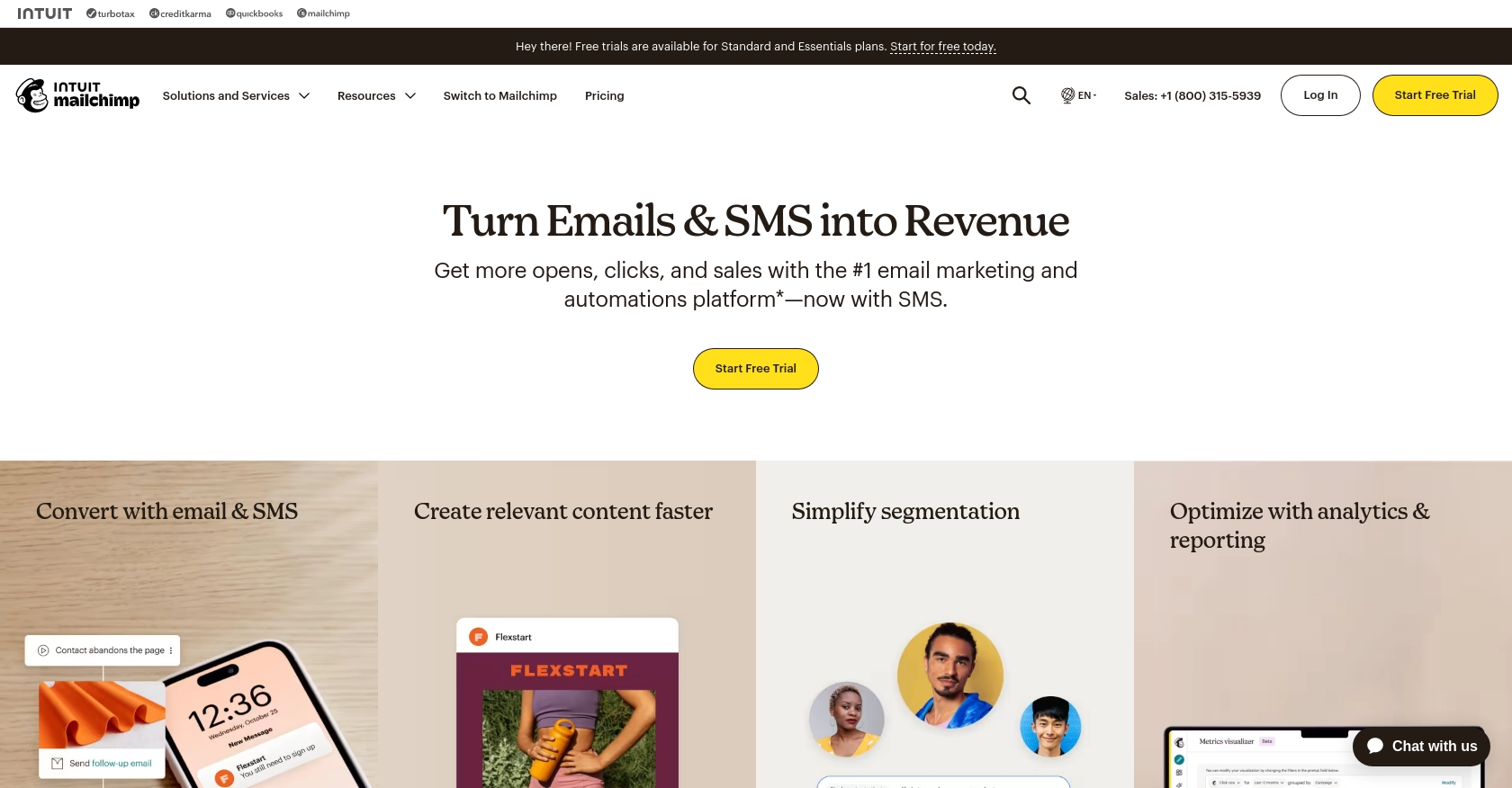
Introduction to Mailchimp API Integration
Mailchimp is a powerful marketing automation platform that enables businesses to create, send, and analyze email and ad campaigns. Known for its user-friendly interface and robust features, Mailchimp is a go-to solution for marketers looking to engage with their audience effectively.
Developers may want to integrate with Mailchimp's API to access and manage audience data, automate marketing workflows, and synchronize email activity with their applications. For example, a developer might use the Mailchimp API to retrieve a list of members from a specific audience, allowing for personalized marketing strategies based on user behavior and preferences.
This article will guide you through using PHP to interact with the Mailchimp API, focusing on retrieving member information from your Mailchimp lists. By following this tutorial, you'll learn how to efficiently connect to Mailchimp, authenticate requests, and handle data using PHP.
Setting Up Your Mailchimp Test Account for API Integration
Before diving into the Mailchimp API, it's essential to set up a test account to safely experiment with API calls without affecting your live data. Mailchimp offers a straightforward process to create an account and generate the necessary credentials for API access.
Create a Mailchimp Account
If you don't already have a Mailchimp account, follow these steps to create one:
- Visit the Mailchimp website and click on "Sign Up Free."
- Fill in the required details, such as your email, username, and password, and follow the prompts to complete the registration process.
- Once your account is set up, log in to access the Mailchimp dashboard.
Generate an API Key for Mailchimp
To interact with the Mailchimp API, you'll need an API key. Here's how to generate one:
- Navigate to your account settings by clicking on your profile icon and selecting "Account."
- Go to the "Extras" dropdown menu and select "API keys."
- Click on "Create A Key" to generate a new API key. Give it a descriptive name for easy identification.
- Copy the generated API key and store it securely, as you will need it for authentication in your PHP code.
For more details, refer to the Mailchimp API Quick Start Guide.
Understanding Mailchimp OAuth Authentication
While API keys provide a simple way to authenticate, Mailchimp also supports OAuth 2 for applications that require access on behalf of other users. This method is recommended for integrations that involve multiple Mailchimp accounts.
For OAuth setup, you'll need to register your application with Mailchimp and follow the OAuth flow to obtain access tokens. Detailed steps can be found in the Mailchimp OAuth Documentation.
Mailchimp API Rate Limits
Mailchimp imposes certain limits on API requests to ensure fair usage. The Marketing API allows up to 10 simultaneous connections. Exceeding this limit will result in a 429 error. It's crucial to design your application to handle these limits gracefully.
For more information on rate limits, visit the Mailchimp API Limits Documentation.
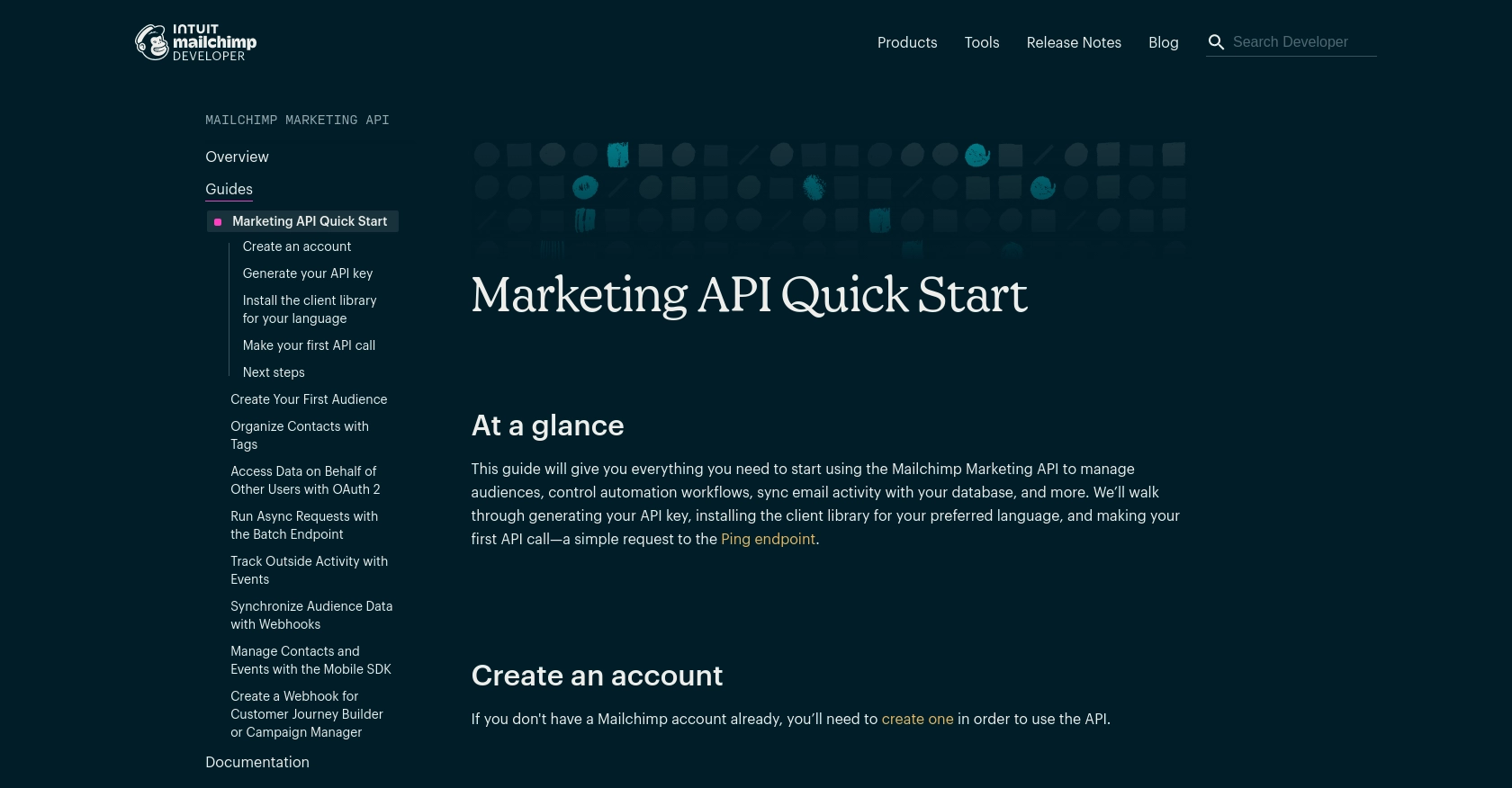
sbb-itb-96038d7
How to Make API Calls to Retrieve Mailchimp Members Using PHP
To interact with the Mailchimp API and retrieve member information, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling the responses.
Setting Up Your PHP Environment for Mailchimp API Integration
Before you begin, ensure that your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled to make HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Libraries for Mailchimp API
To simplify API interactions, you can use the Guzzle HTTP client, a popular PHP library for making HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Members from Mailchimp Lists
With your environment set up, you can now write the PHP code to retrieve members from a Mailchimp list. Below is a sample script:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$apiKey = 'YOUR_API_KEY';
$listId = 'YOUR_LIST_ID';
$serverPrefix = 'YOUR_SERVER_PREFIX'; // e.g., us19
$client = new Client([
'base_uri' => "https://{$serverPrefix}.api.mailchimp.com/3.0/"
]);
$response = $client->request('GET', "lists/{$listId}/members", [
'auth' => ['anystring', $apiKey]
]);
if ($response->getStatusCode() === 200) {
$members = json_decode($response->getBody(), true);
foreach ($members['members'] as $member) {
echo "Email: " . $member['email_address'] . "\n";
}
} else {
echo "Failed to retrieve members. Status code: " . $response->getStatusCode();
}
Replace YOUR_API_KEY
, YOUR_LIST_ID
, and YOUR_SERVER_PREFIX
with your actual Mailchimp API key, list ID, and server prefix.
Understanding the Mailchimp API Response
Upon successful execution, the script will output the email addresses of the members in the specified list. The response from the Mailchimp API includes detailed information about each member, which you can use to tailor your marketing strategies.
Handling Errors and Mailchimp API Error Codes
It's crucial to handle potential errors when making API calls. The Mailchimp API may return various error codes, such as 401 for unauthorized access or 404 if the list ID is incorrect. Implement error handling to manage these scenarios gracefully.
For more detailed error information, refer to the Mailchimp API Documentation.
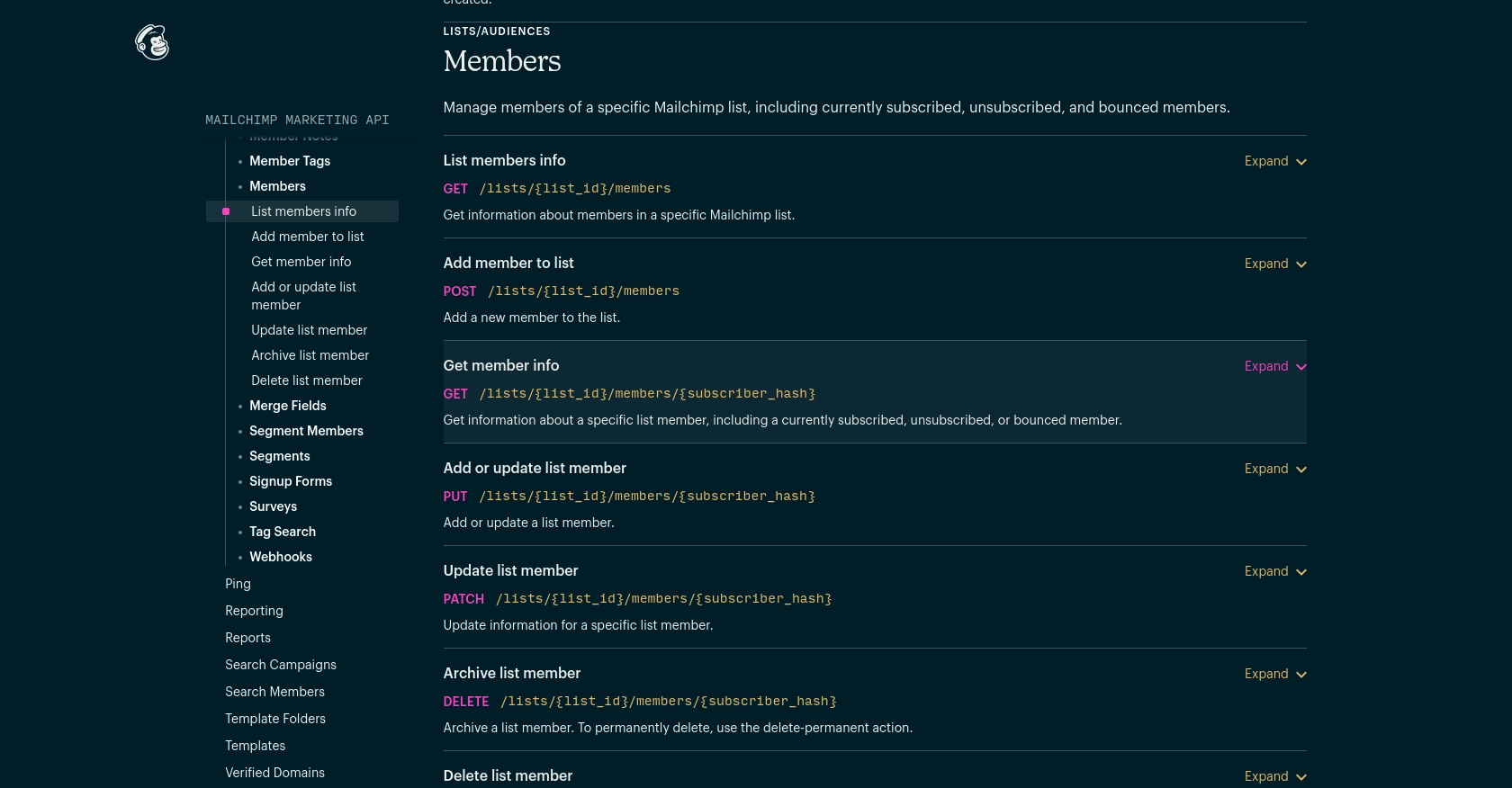
Conclusion and Best Practices for Mailchimp API Integration
Integrating with the Mailchimp API using PHP allows developers to efficiently manage audience data and automate marketing workflows. By following the steps outlined in this guide, you can retrieve member information from your Mailchimp lists and leverage this data to enhance your marketing strategies.
Best Practices for Secure and Efficient Mailchimp API Usage
- Secure Storage of API Credentials: Always store your Mailchimp API key securely, similar to how you would handle passwords. Avoid hardcoding it in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Be mindful of Mailchimp's API rate limits, which allow up to 10 simultaneous connections. Implement logic to handle 429 errors gracefully and consider caching frequently accessed data to reduce API calls.
- Error Handling: Implement robust error handling to manage various API error codes, such as 401 for unauthorized access. This ensures your application can respond appropriately to issues.
- Data Standardization: When retrieving member data, consider standardizing and transforming fields to align with your application's data structure, ensuring consistency across platforms.
Streamlining Integrations with Endgrate
For developers looking to simplify the integration process across multiple platforms, Endgrate offers a unified API solution. By using Endgrate, you can save time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover how companies like yours have successfully scaled their integrations.
Read More
Ready to get started?