Using the Xero API to Get Items (with Javascript examples)
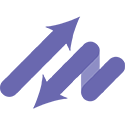
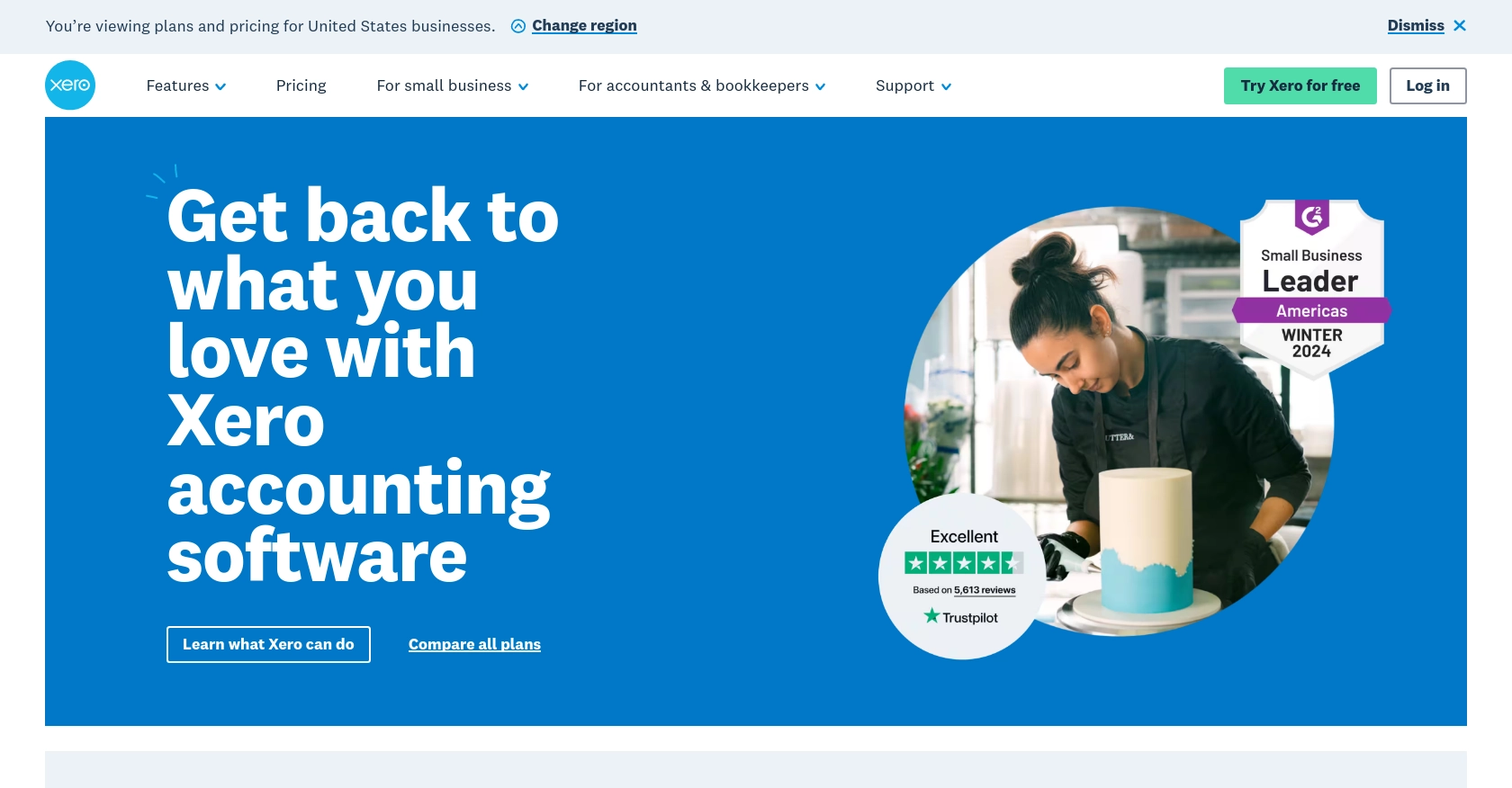
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, bank reconciliation, and financial reporting, Xero provides a comprehensive solution for businesses looking to streamline their accounting processes.
Developers may want to integrate with Xero's API to automate financial tasks and enhance business operations. For example, using the Xero API, a developer can retrieve a list of inventory items, enabling seamless inventory management and synchronization with other business systems.
This article will guide you through using JavaScript to interact with the Xero API, specifically focusing on retrieving items from the inventory. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Xero Sandbox Account for API Integration
Before diving into the Xero API, you need to set up a sandbox account. This will allow you to test API calls without affecting real data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To start, you'll need a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your developer account.
Accessing the Xero Demo Company
After setting up your developer account, you can access the demo company:
- Navigate to the My Apps section in the Xero Developer Portal.
- Select Try the Demo Company to access a pre-configured sandbox environment.
Creating a Xero App for OAuth 2.0 Authentication
To interact with the Xero API, you'll need to create an app to obtain OAuth 2.0 credentials:
- In the My Apps section, click on Create App.
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where you can receive authorization codes.
- Once created, note down the Client ID and Client Secret.
For more information on OAuth 2.0 authentication, refer to the Xero OAuth 2.0 Overview.
Configuring OAuth 2.0 Scopes
Scopes define the level of access your app has to the Xero API. To retrieve items, ensure you have the necessary scopes:
- Navigate to the Scopes section of your app settings.
- Select the scopes related to inventory and accounting, such as
accounting.transactions
andaccounting.settings
.
For detailed information on scopes, visit the Xero Scopes Documentation.
Generating OAuth 2.0 Tokens
With your app set up, you can now generate tokens to authenticate API requests:
- Use the standard OAuth 2.0 authorization code flow to obtain an access token.
- Exchange the authorization code for an access token and refresh token.
Refer to the Xero OAuth 2.0 Auth Flow for detailed steps.
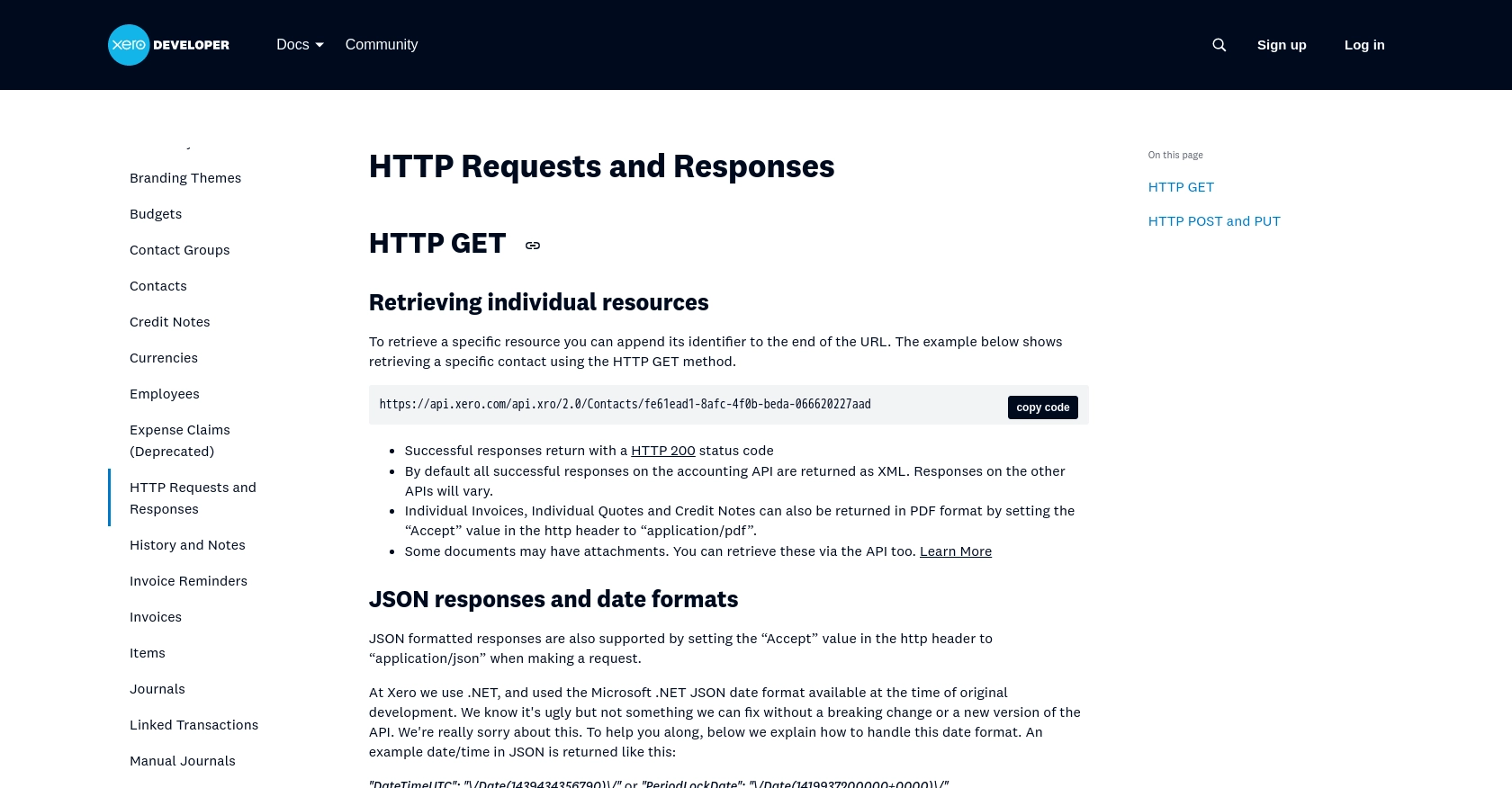
sbb-itb-96038d7
Making API Calls to Retrieve Items from Xero Using JavaScript
With your Xero app set up and OAuth 2.0 tokens generated, you can now proceed to make API calls to retrieve items from Xero's inventory. This section will guide you through the process using JavaScript, ensuring you understand each step and can handle responses effectively.
Prerequisites for Xero API Integration with JavaScript
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A text editor or IDE for writing JavaScript code, such as Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Items from Xero
Create a new JavaScript file named getXeroItems.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Items';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to fetch items from Xero
async function fetchXeroItems() {
try {
const response = await axios.get(endpoint, { headers });
const items = response.data.Items;
console.log('Retrieved Items:', items);
} catch (error) {
console.error('Error fetching items:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch items
fetchXeroItems();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authentication process.
Running the JavaScript Code and Verifying the Output
To execute the code and retrieve items from Xero, run the following command in your terminal:
node getXeroItems.js
If the request is successful, you should see a list of items from your Xero demo company printed in the console. Verify the retrieved items by cross-referencing them with the inventory in your Xero sandbox account.
Handling Errors and Understanding Xero API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common Xero API error codes include:
- 400 Bad Request: The request was invalid. Check your request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You don't have permission to access the resource. Check your OAuth scopes.
- 429 Too Many Requests: You've hit the rate limit. Implement rate limiting strategies.
For more detailed information on error codes, refer to the Xero API Requests and Responses Documentation.
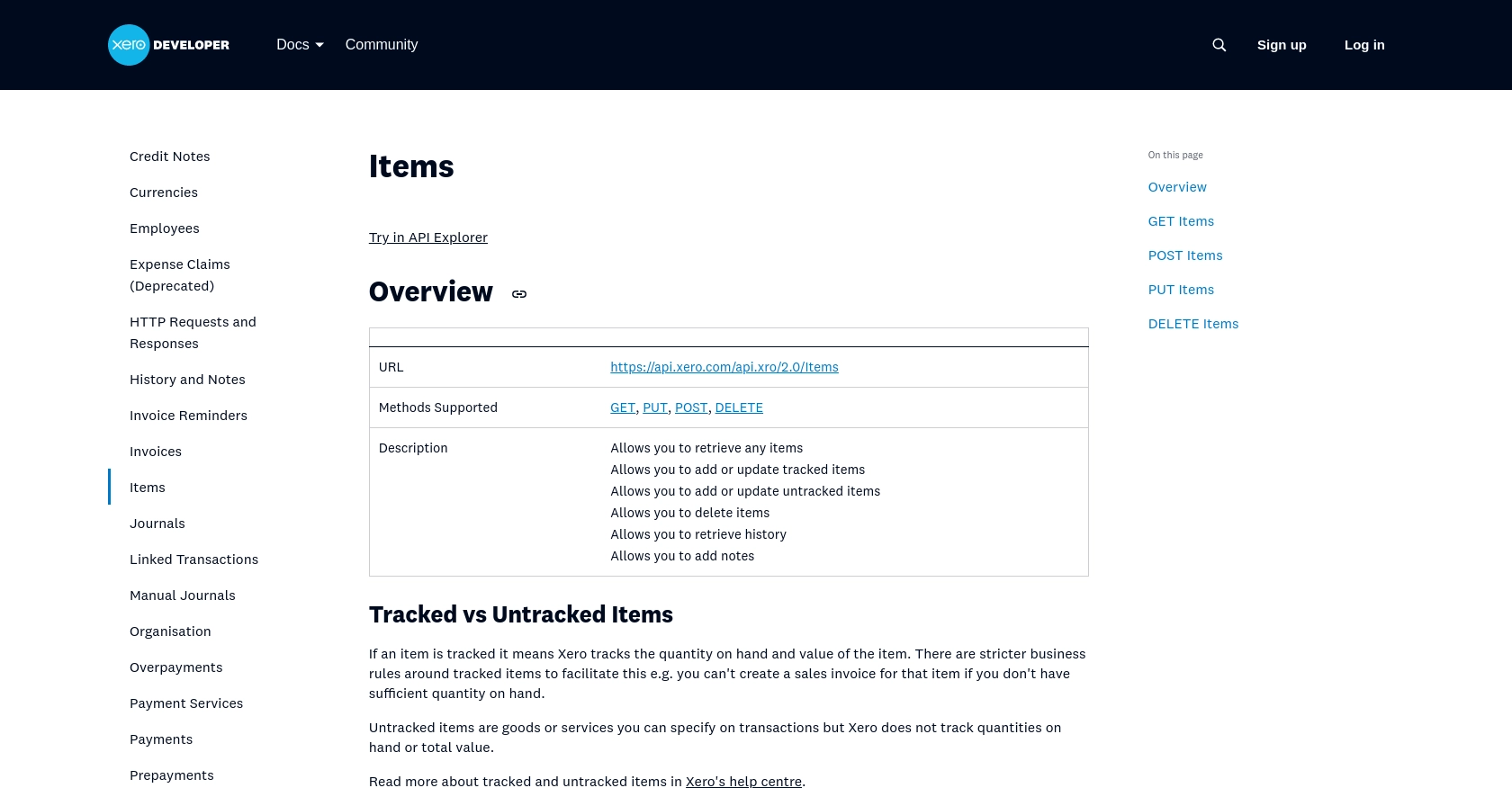
Conclusion and Best Practices for Xero API Integration with JavaScript
Integrating with the Xero API using JavaScript provides a powerful way to automate and enhance your business's financial operations. By following the steps outlined in this guide, you can efficiently retrieve inventory items and seamlessly integrate them into your existing systems.
Best Practices for Secure and Efficient Xero API Usage
- Secure Storage of Credentials: Always store your OAuth 2.0 credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Implementing Rate Limiting: Xero imposes rate limits on API requests. To avoid hitting these limits, implement strategies such as exponential backoff and request queuing. For more details, refer to the Xero OAuth 2.0 API Limits.
- Data Transformation and Standardization: Ensure that the data retrieved from Xero is transformed and standardized to fit your application's requirements. This helps maintain consistency across different systems.
Streamlining Integrations with Endgrate
While building integrations with Xero can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Xero. This allows you to focus on your core product while outsourcing the integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/items
Ready to get started?