Using the Recruit CRM API to Get Contacts in Python
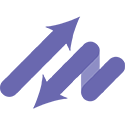
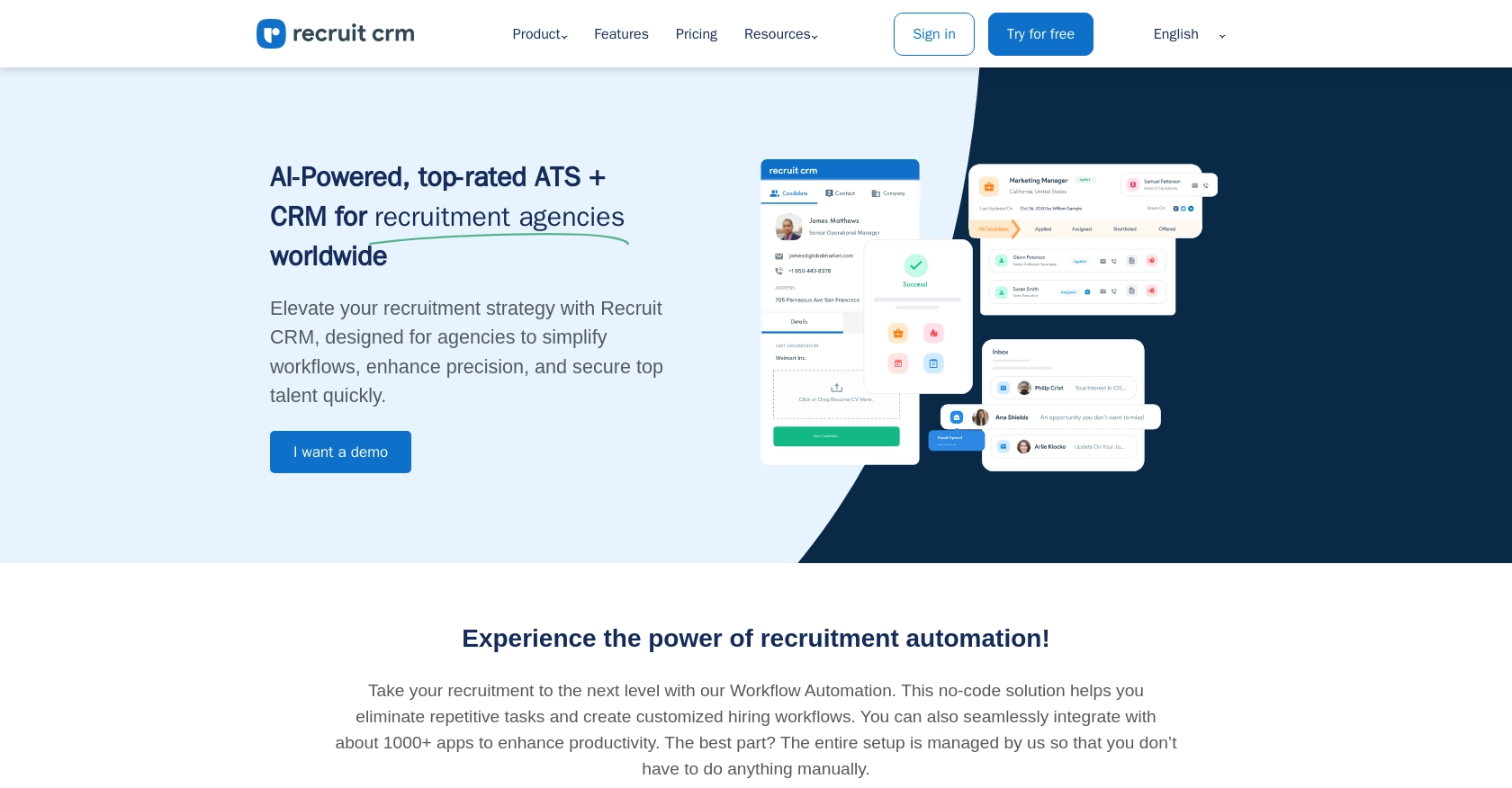
Introduction to Recruit CRM
Recruit CRM is a powerful recruitment software designed to enhance the efficiency of recruitment businesses by providing a comprehensive suite of tools for managing candidates, contacts, companies, and jobs. Its robust API allows developers to customize and extend the platform's capabilities, making it an ideal choice for businesses looking to streamline their recruitment processes.
Connecting with the Recruit CRM API can enable developers to automate various tasks, such as retrieving and managing contact information. For example, a developer might use the API to fetch contact details and integrate them into a custom dashboard, providing recruiters with real-time access to candidate information.
Setting Up Your Recruit CRM Test Account
Before you can start interacting with the Recruit CRM API, you'll need to set up a Recruit CRM account. This process involves obtaining an API token, which is essential for authenticating your API requests.
Creating a Recruit CRM Account
If you don't already have a Recruit CRM account, you can sign up for one on the Recruit CRM website. The platform offers a Business Plan that is required to access the API features. Follow the instructions to create your account and log in.
Generating Your Recruit CRM API Token
Once your account is set up, you'll need to generate an API token to authenticate your requests. Follow these steps to obtain your API token:
- Log in to your Recruit CRM account.
- Navigate to Admin Setting and then to Account Management.
- Locate the section for API Tokens and generate a new token.
- Copy the API token and store it securely. This token will be used in your API requests to authenticate them.
Remember, your API token carries significant privileges, so ensure it is kept secure and not exposed in publicly accessible areas.
Understanding Recruit CRM API Authentication
The Recruit CRM API uses API tokens for authentication. All API requests must be made over HTTPS, and requests without proper authentication will fail. Here is an example of how to include the API token in your request headers:
headers = {
"Authorization": "Bearer Your_API_Token",
"Accept": "application/json"
}
Replace Your_API_Token
with the token you generated earlier. This header setup is crucial for successfully interacting with the Recruit CRM API.
For more detailed information, you can refer to the Recruit CRM API documentation.
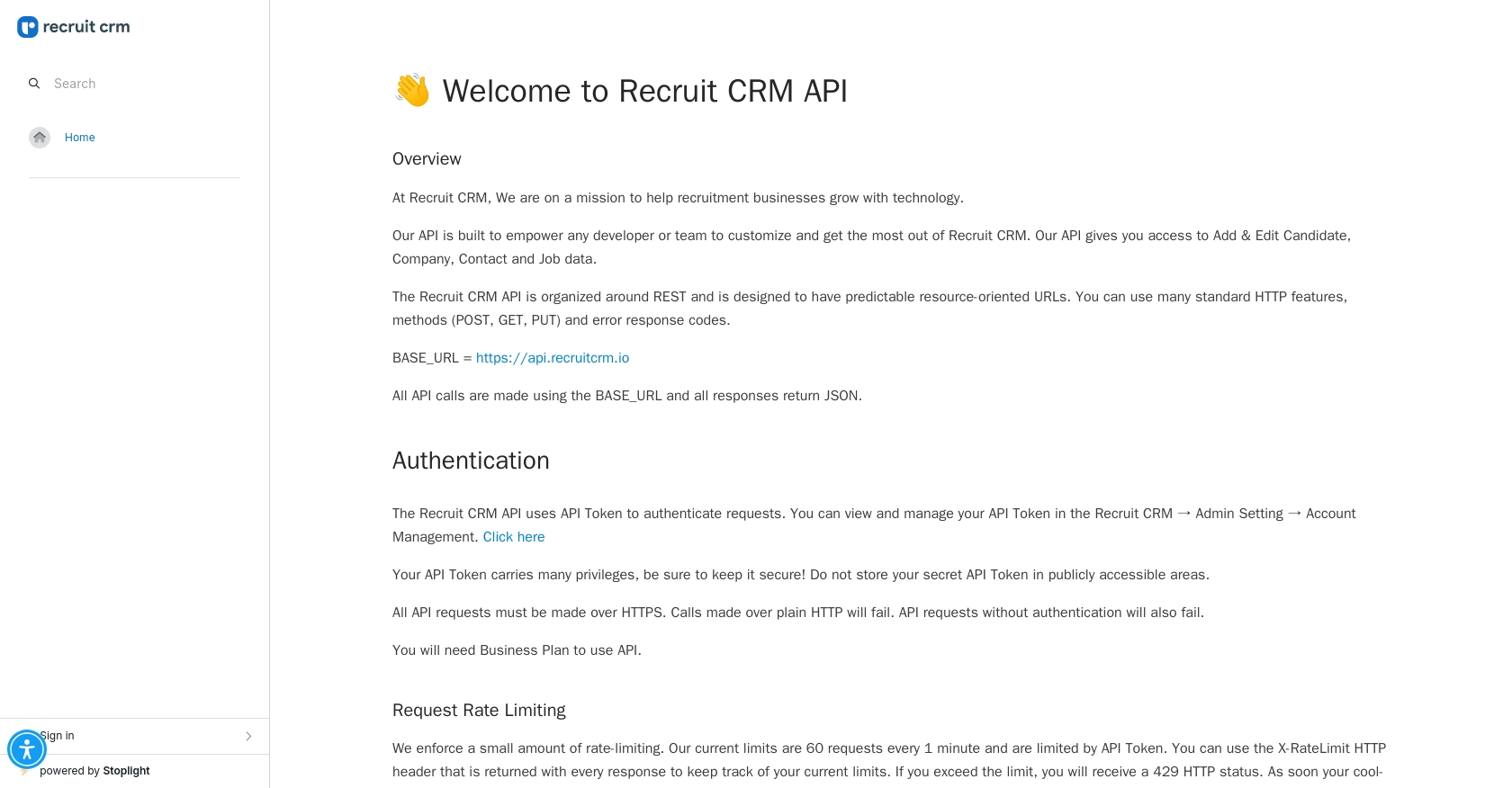
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Recruit CRM Using Python
To interact with the Recruit CRM API and retrieve contact information, you'll need to use Python to make HTTP requests. This section will guide you through setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Recruit CRM API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the requests
library, which simplifies making HTTP requests in Python. You can install it using pip:
pip install requests
Writing Python Code to Fetch Contacts from Recruit CRM
Now that your environment is ready, you can write a Python script to fetch contacts from Recruit CRM. Create a file named get_recruitcrm_contacts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.recruitcrm.io/v1/contacts"
headers = {
"Authorization": "Bearer Your_API_Token",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the contacts and print their information
for contact in data.get("contacts", []):
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code}")
Replace Your_API_Token
with the API token you generated earlier. This script sends a GET request to the Recruit CRM API to fetch contact information and prints the details if the request is successful.
Running Your Python Script and Verifying Results
Execute your script from the terminal or command line:
python get_recruitcrm_contacts.py
If successful, the script will output the contact details retrieved from Recruit CRM. You can verify the results by checking the contacts in your Recruit CRM account.
Handling Errors and Understanding Recruit CRM API Error Codes
When working with the Recruit CRM API, it's crucial to handle errors gracefully. The API returns standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful, and the contacts were retrieved.
- 401 Unauthorized: The API token is missing or invalid.
- 429 Too Many Requests: You've exceeded the rate limit of 60 requests per minute.
Ensure your application checks the response status code and handles errors appropriately, such as retrying after a cooldown period if you hit the rate limit.
For more detailed information on error handling, refer to the Recruit CRM API documentation.
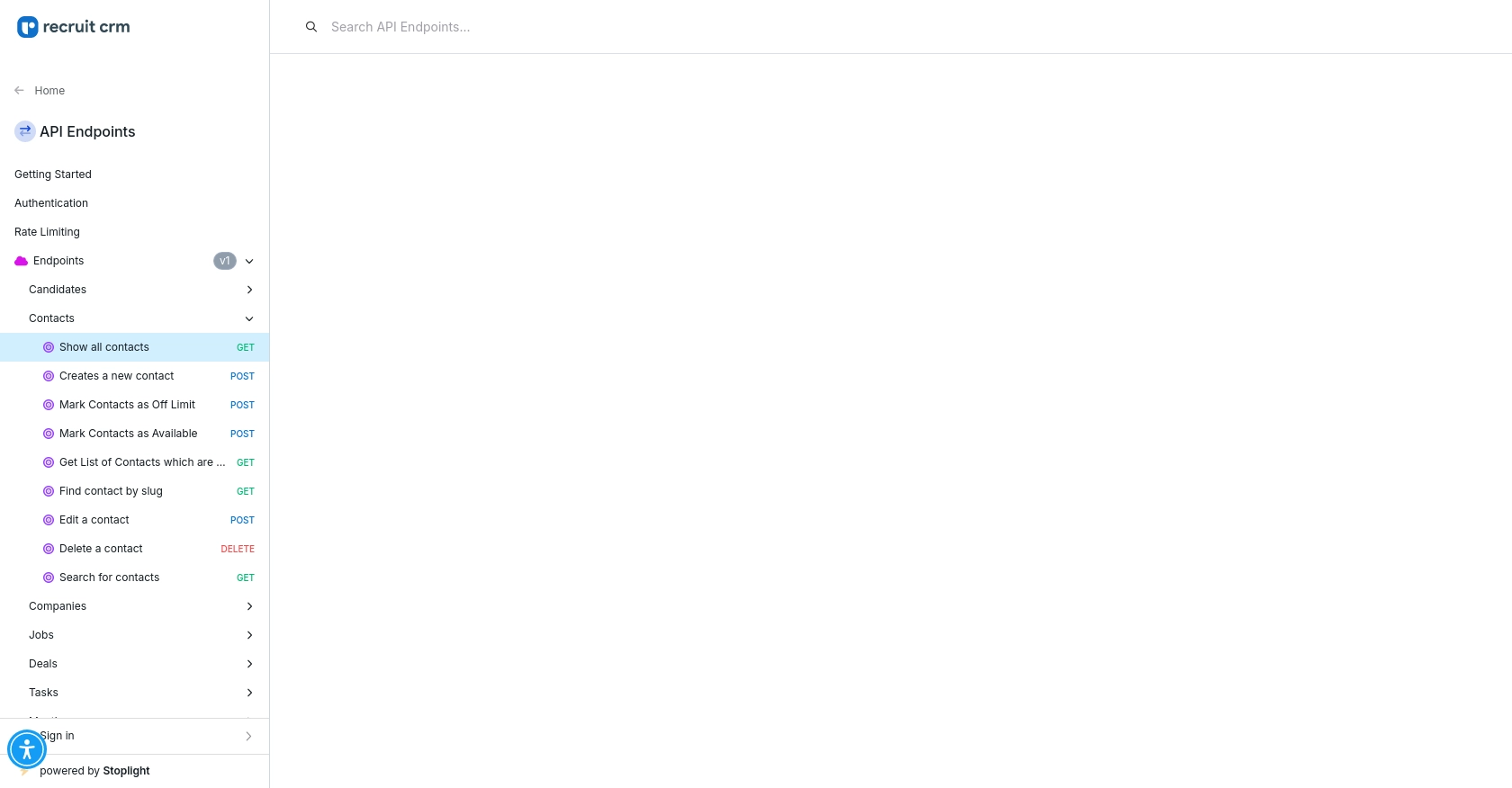
Best Practices for Using the Recruit CRM API
When integrating with the Recruit CRM API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Secure API Tokens: Always keep your API tokens secure and never expose them in publicly accessible areas. Consider using environment variables or secure vaults to store sensitive information.
- Handle Rate Limiting: Be mindful of the Recruit CRM API's rate limit of 60 requests per minute. Implement logic to handle the
429 Too Many Requests
status by pausing requests and retrying after the cooldown period. - Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications and systems.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Recruit CRM. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate.
Read More
Ready to get started?