How to Create or Update People with the Affinity API in PHP
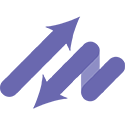
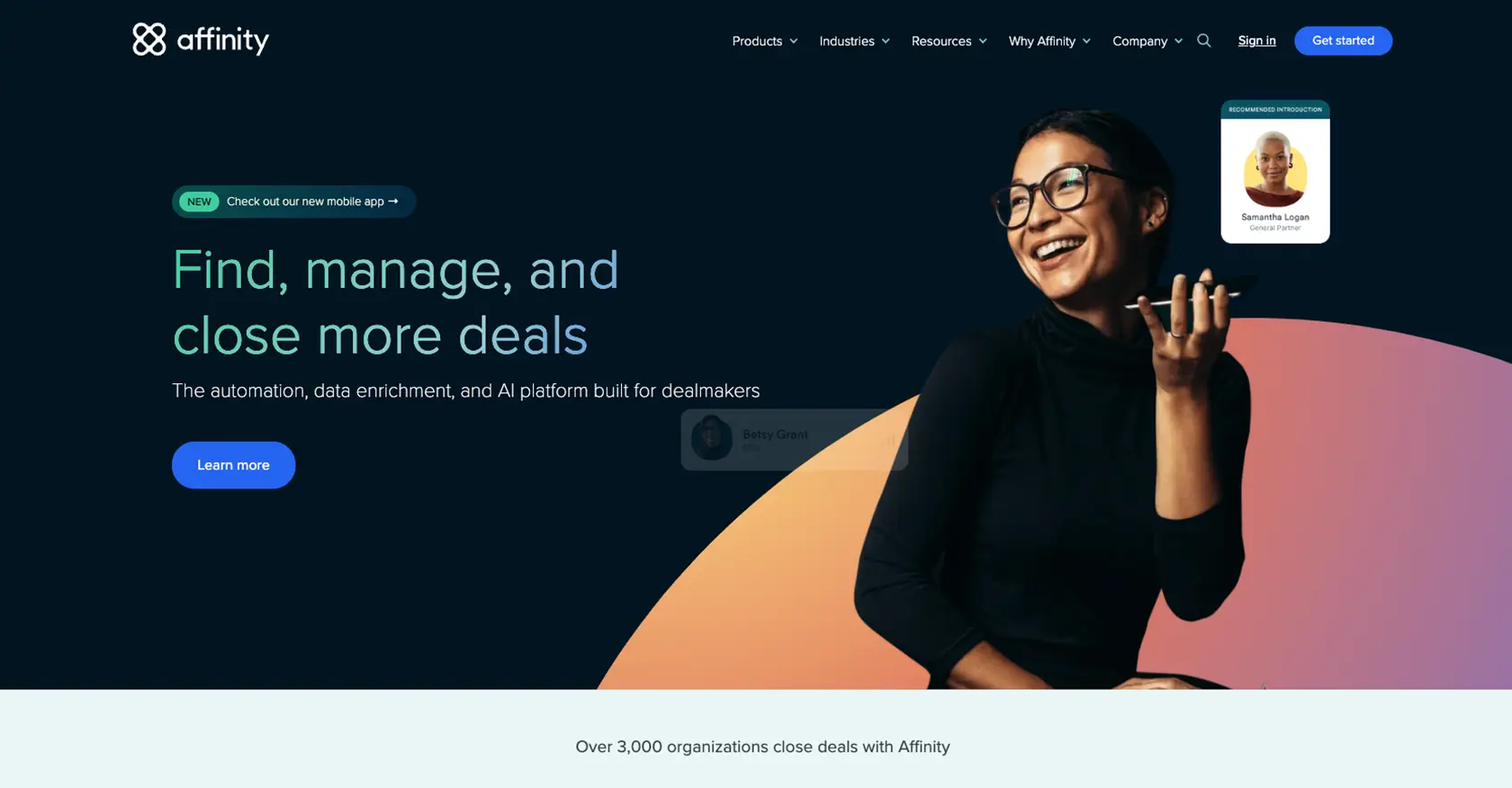
Introduction to Affinity API for PHP Developers
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. By providing tools to track interactions, manage contacts, and analyze relationship strengths, Affinity empowers organizations to build and maintain meaningful connections.
Integrating with the Affinity API allows developers to automate and enhance their CRM processes. For example, you can create or update contact information directly from your application, ensuring that your Affinity database is always up-to-date. This integration is particularly useful for businesses that need to manage large volumes of contacts efficiently.
In this article, we will explore how to use PHP to interact with the Affinity API, focusing on creating and updating people records. This guide will provide step-by-step instructions to help you seamlessly integrate Affinity's capabilities into your PHP applications.
Setting Up Your Affinity API Test Account
Before you begin integrating with the Affinity API using PHP, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Affinity provides a straightforward process to obtain an API key, which is necessary for authenticating your requests.
Creating an Affinity Account
If you don't already have an Affinity account, you can sign up for one on the Affinity website. Follow the registration process to create your account. Once your account is set up, you can access the API settings to generate your API key.
Generating an API Key for Affinity
To interact with the Affinity API, you'll need an API key. Follow these steps to generate one:
- Log in to your Affinity account.
- Navigate to the Settings panel, accessible from the left sidebar.
- Locate the API Key section.
- Click on Generate API Key to create a new key.
- Copy the generated API key and store it securely, as you will need it for authentication in your PHP application.
For more detailed instructions, you can refer to the Affinity API documentation.
Understanding Affinity API Authentication
The Affinity API uses HTTP Basic Auth for authentication. You will provide your API key as the password, leaving the username field empty. This method ensures secure access to the API endpoints.
// Example of setting up authentication in PHP
$apiKey = 'your_api_key_here';
$options = [
'http' => [
'header' => "Authorization: Basic " . base64_encode(":$apiKey")
]
];
$context = stream_context_create($options);
By following these steps, you will have your Affinity test account ready, allowing you to proceed with making API calls to create or update people records using PHP.
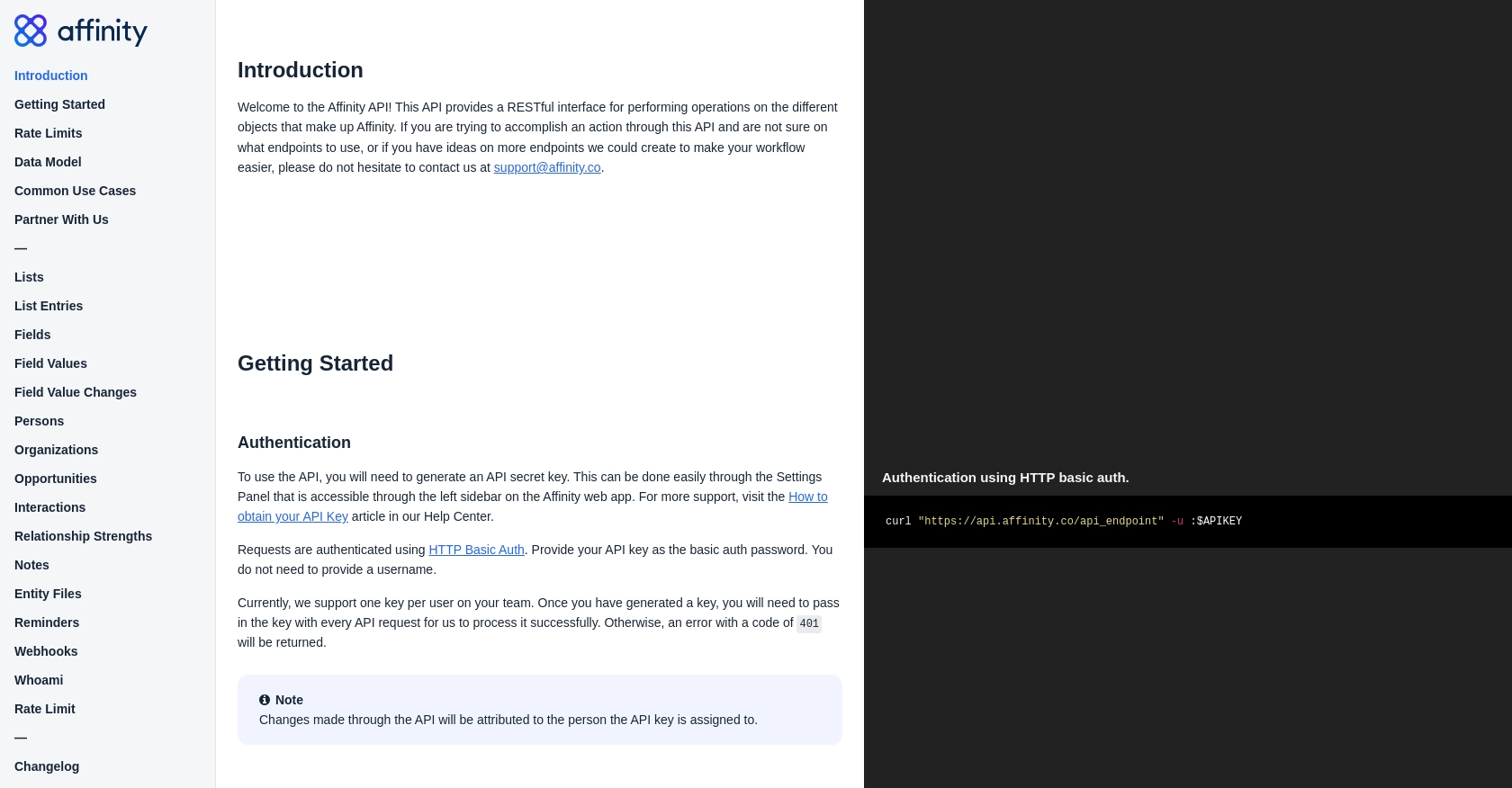
sbb-itb-96038d7
Making API Calls to Create or Update People with Affinity API in PHP
To effectively interact with the Affinity API using PHP, you need to understand how to make API calls for creating or updating people records. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling responses.
Setting Up PHP Environment for Affinity API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- cURL extension enabled
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Writing PHP Code to Create or Update People in Affinity
Below is an example of how to create or update a person using the Affinity API in PHP:
$apiKey = 'your_api_key_here';
$personId = '12345'; // Use null if creating a new person
$url = $personId ? "https://api.affinity.co/persons/$personId" : "https://api.affinity.co/persons";
$method = $personId ? 'PUT' : 'POST';
$data = [
'first_name' => 'John',
'last_name' => 'Doe',
'emails' => ['john.doe@example.com']
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => $method,
CURLOPT_HTTPHEADER => [
"Authorization: Basic " . base64_encode(":$apiKey"),
"Content-Type: application/json"
],
CURLOPT_POSTFIELDS => json_encode($data)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
if ($response === false) {
echo "Error: " . curl_error($ch);
} else {
$responseData = json_decode($response, true);
if (isset($responseData['id'])) {
echo "Person " . ($personId ? "updated" : "created") . " successfully with ID: " . $responseData['id'];
} else {
echo "Failed to " . ($personId ? "update" : "create") . " person.";
}
}
Handling API Responses and Errors
After making the API call, it's crucial to handle the response correctly. Affinity API returns JSON responses, which you can decode and process in PHP. Pay attention to the HTTP status codes:
- 200 - Success
- 401 - Unauthorized (check your API key)
- 404 - Not Found (invalid endpoint or person ID)
- 422 - Unprocessable Entity (check your data format)
- 429 - Too Many Requests (rate limit exceeded)
For more details on error codes, refer to the Affinity API documentation.
Verifying Success in Affinity Dashboard
Once you successfully create or update a person, verify the changes in your Affinity dashboard. This ensures that your API integration is functioning as expected.
By following these steps, you can efficiently manage people records in Affinity using PHP, enhancing your CRM processes and maintaining accurate contact data.
Conclusion and Best Practices for Affinity API Integration in PHP
Integrating the Affinity API into your PHP applications can significantly enhance your CRM processes by automating the management of contact data. By following the steps outlined in this guide, you can efficiently create and update people records, ensuring your Affinity database remains accurate and up-to-date.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Affinity's rate limits, which allow up to 900 requests per user per minute. Implement error handling for
429 Too Many Requests
responses and consider implementing exponential backoff for retries. - Data Validation: Ensure that the data you send to the API is correctly formatted and validated to avoid
422 Unprocessable Entity
errors. - Monitor API Usage: Regularly monitor your API usage and responses to identify any issues or areas for optimization.
Enhance Your Integration with Endgrate
While integrating with the Affinity API can streamline your CRM processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Affinity.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your users. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
Ready to get started?