Using the Teamwork CRM API to Get Users in PHP
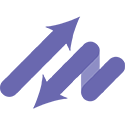
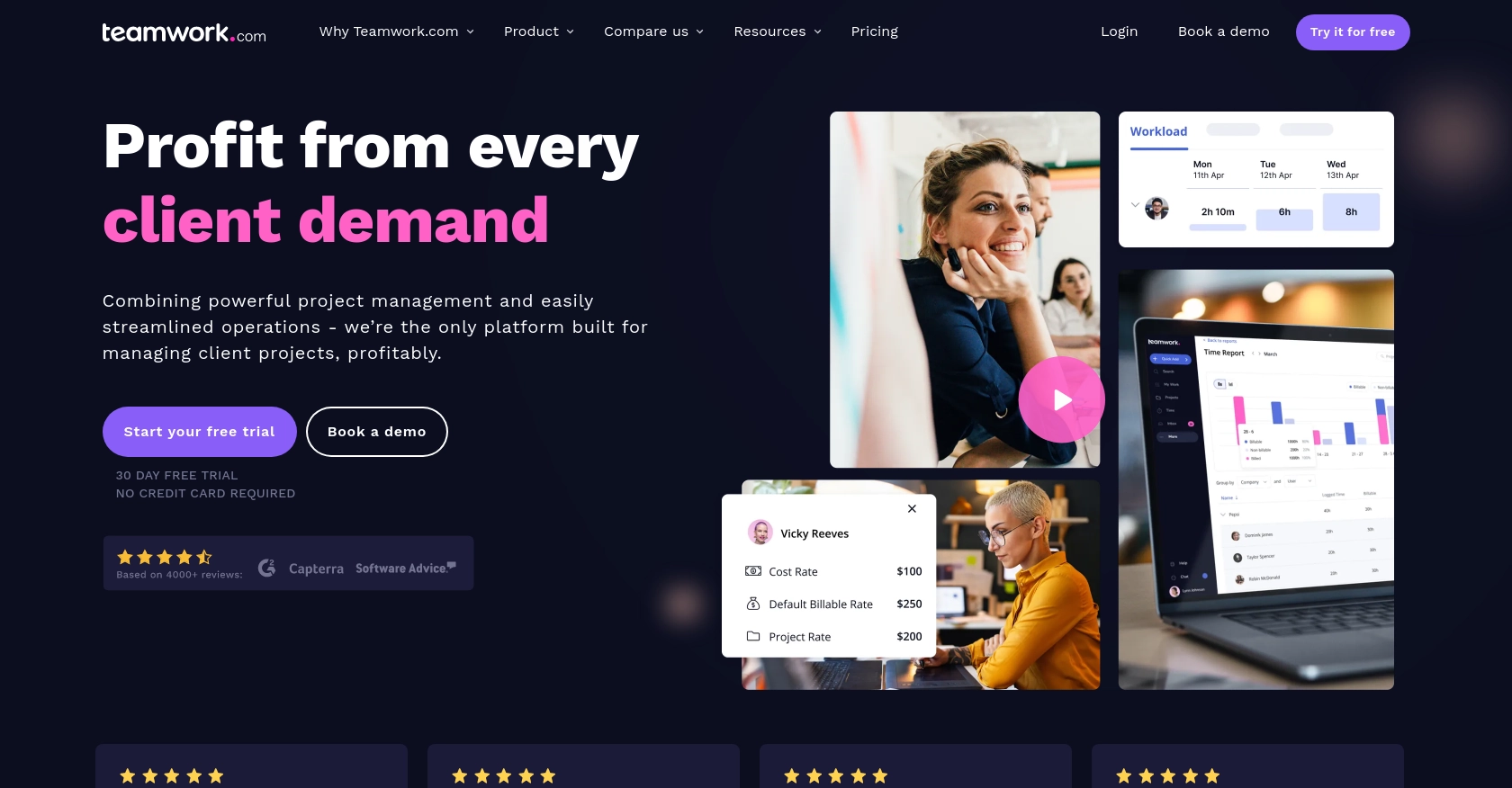
Introduction to Teamwork CRM
Teamwork CRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. It offers a comprehensive suite of tools that enable teams to track leads, manage pipelines, and close deals effectively. With its user-friendly interface and powerful features, Teamwork CRM is a popular choice for businesses looking to enhance their sales operations.
Integrating with the Teamwork CRM API allows developers to access and manipulate CRM data programmatically, providing opportunities to automate and streamline various business processes. For example, a developer might use the Teamwork CRM API to retrieve user information, enabling seamless synchronization of user data across different platforms and applications.
Setting Up a Teamwork CRM Test Account
Before you can start using the Teamwork CRM API, you need to set up a test account. This will allow you to experiment with API calls without affecting live data. Follow these steps to create a sandbox environment for your development needs.
Creating a Teamwork CRM Free Trial Account
To get started, you need to create a free trial account with Teamwork CRM. This will provide you with access to all the necessary features to test the API integration:
- Visit the Teamwork CRM website and click on the "Try for Free" button.
- Fill out the registration form with your details, including your email address and company information.
- Once registered, you will receive a confirmation email. Follow the instructions in the email to activate your account.
Generating API Credentials for Teamwork CRM
After setting up your account, you need to generate API credentials to authenticate your requests. Teamwork CRM uses OAuth 2.0 for secure authentication:
- Log in to your Teamwork CRM account and navigate to the Developer Portal.
- Click on "Create an App" to register a new application.
- Fill in the required details, such as the app name and description.
- Once your app is created, you will receive a client ID and client secret. Keep these credentials secure as they are required for API authentication.
Authenticating with Teamwork CRM API Using OAuth 2.0
To authenticate your API requests, you need to implement the OAuth 2.0 login flow:
- Use the client ID and client secret obtained earlier to request an access token.
- Include the access token in the Authorization header of your API requests as follows:
Authorization: Bearer {access_token}
Refer to the Teamwork CRM authentication documentation for detailed instructions on implementing OAuth 2.0.
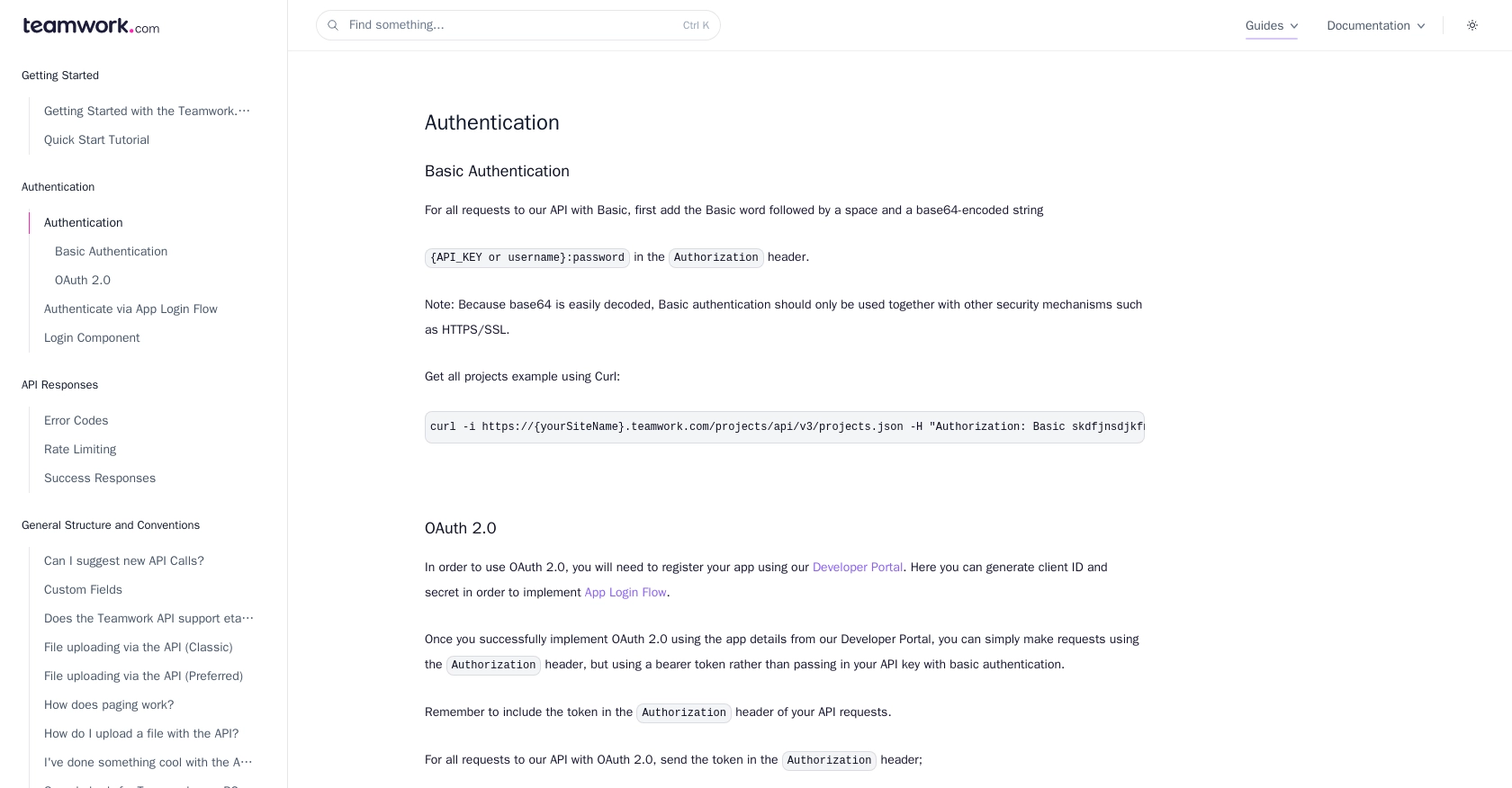
sbb-itb-96038d7
Making API Calls to Retrieve Users from Teamwork CRM Using PHP
To interact with the Teamwork CRM API and retrieve user information, you'll need to set up your PHP environment and make the necessary API calls. This section will guide you through the process, including setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment for Teamwork CRM API
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP 7.4 or later and the cURL extension enabled. You can verify your PHP version and extensions by running the following command in your terminal:
php -v
To enable the cURL extension, ensure your php.ini
file includes the following line:
extension=curl
Writing PHP Code to Retrieve Users from Teamwork CRM
With your environment set up, you can now write the PHP code to make an API call to Teamwork CRM and retrieve user data. Create a new PHP file named get_teamwork_users.php
and add the following code:
<?php
// Set the API endpoint URL
$apiUrl = "https://{yourSiteName}.teamwork.com/crm/v2/users";
// Set the access token
$accessToken = "Your_Access_Token";
// Initialize cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer $accessToken",
"Content-Type: application/json"
));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display user information
foreach ($data['users'] as $user) {
echo "User ID: " . $user['id'] . "\n";
echo "Name: " . $user['name'] . "\n";
echo "Email: " . $user['email'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
with the access token obtained during the authentication process. This script initializes a cURL session, sets the necessary headers, and executes a GET request to the Teamwork CRM API to fetch user data.
Handling API Responses and Errors in Teamwork CRM
After executing the API call, it's crucial to handle the response and any potential errors. The code above checks for cURL errors and decodes the JSON response to display user information. If the API call is successful, you should see a list of users with their IDs, names, and email addresses.
Refer to the Teamwork CRM error codes documentation for more information on handling specific error codes such as 401 Unauthorized or 429 Rate Limit Exceeded.
Verifying API Call Success in Teamwork CRM
To ensure the API call was successful, log in to your Teamwork CRM account and verify that the retrieved user data matches the information in your CRM. This verification step helps confirm that your integration is functioning correctly.
By following these steps, you can efficiently retrieve user data from Teamwork CRM using PHP, enabling seamless integration with your applications and systems.
Conclusion and Best Practices for Using Teamwork CRM API with PHP
Integrating with the Teamwork CRM API using PHP can significantly enhance your ability to manage and automate CRM data processes. By following the steps outlined in this guide, you can efficiently retrieve user data and ensure seamless synchronization across your applications.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your API credentials, such as access tokens, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the rate limits imposed by Teamwork CRM. According to the Teamwork CRM rate limit documentation, the maximum is 150 requests per minute for most plans. Implement logic to handle HTTP 429 errors and retry requests after the specified time.
- Error Handling: Implement robust error handling to manage various HTTP status codes. Refer to the Teamwork CRM error codes documentation for guidance on handling specific errors like 401 Unauthorized or 500 Internal Server Error.
- Data Standardization: Ensure that data retrieved from Teamwork CRM is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Teamwork CRM can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?