Using the Xero API to Create or Update Purchase Orders (with Javascript examples)
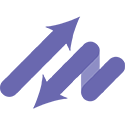
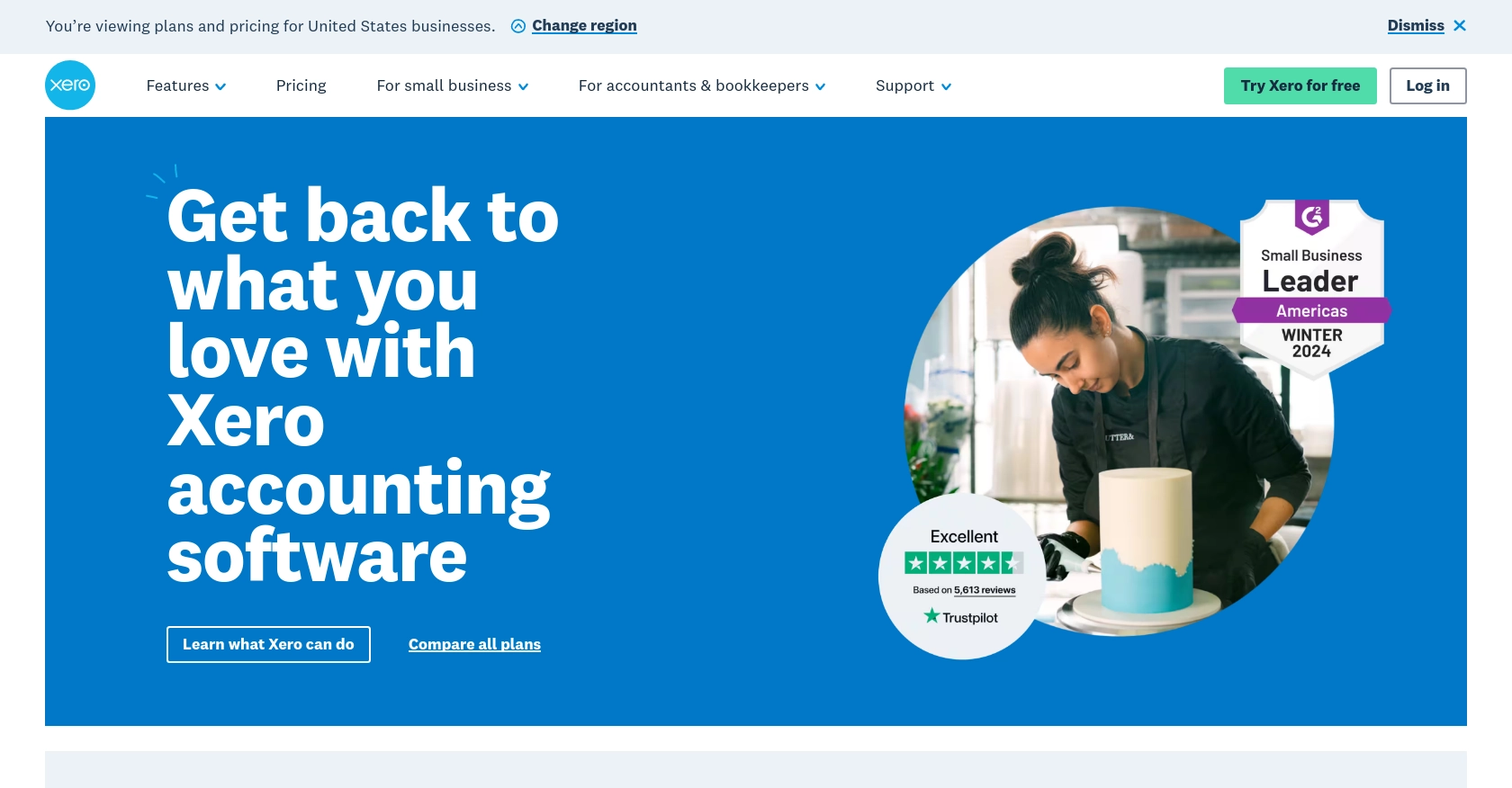
Introduction to Xero API for Purchase Orders
Xero is a powerful cloud-based accounting software platform that caters to small and medium-sized businesses. It offers a comprehensive suite of tools for managing finances, including invoicing, payroll, and purchase orders. Xero's robust API allows developers to integrate its functionalities into their applications, enabling seamless financial management and automation.
Connecting with the Xero API can significantly enhance a developer's ability to manage purchase orders efficiently. For example, by using the Xero API, developers can automate the creation and updating of purchase orders directly from their applications, reducing manual data entry and minimizing errors.
This article will guide you through using JavaScript to interact with the Xero API for creating or updating purchase orders, providing a step-by-step approach to streamline your financial operations.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API to create or update purchase orders, you'll need to set up a sandbox account. This environment allows you to test your integration without affecting live data, ensuring a smooth development process.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal.
- Click on "Sign Up" and fill in the required details to create your account.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
With your developer account ready, you can now create a sandbox organization:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Click on "New App" to create a new application.
- Fill in the necessary details such as app name, company URL, and OAuth 2.0 redirect URI.
- Choose the "Demo Company" option to set up a sandbox organization.
Configuring OAuth 2.0 Authentication
The Xero API uses OAuth 2.0 for authentication. Here's how to configure it:
- In your app settings, locate the "OAuth 2.0 Credentials" section.
- Note down your client ID and client secret, as you'll need these for authentication.
- Set up the necessary scopes for accessing purchase orders by visiting the Xero OAuth 2.0 Scopes page.
Generating Access Tokens
With OAuth 2.0 configured, you can now generate access tokens:
- Use the standard authorization code flow to obtain an access token. Refer to the Xero OAuth 2.0 Authorization Flow guide for detailed steps.
- Ensure you securely store the access token, as it will be used to authenticate API requests.
With your sandbox account and OAuth 2.0 authentication set up, you're ready to start making API calls to create or update purchase orders using JavaScript. This setup ensures you can test your integration thoroughly before deploying it in a live environment.
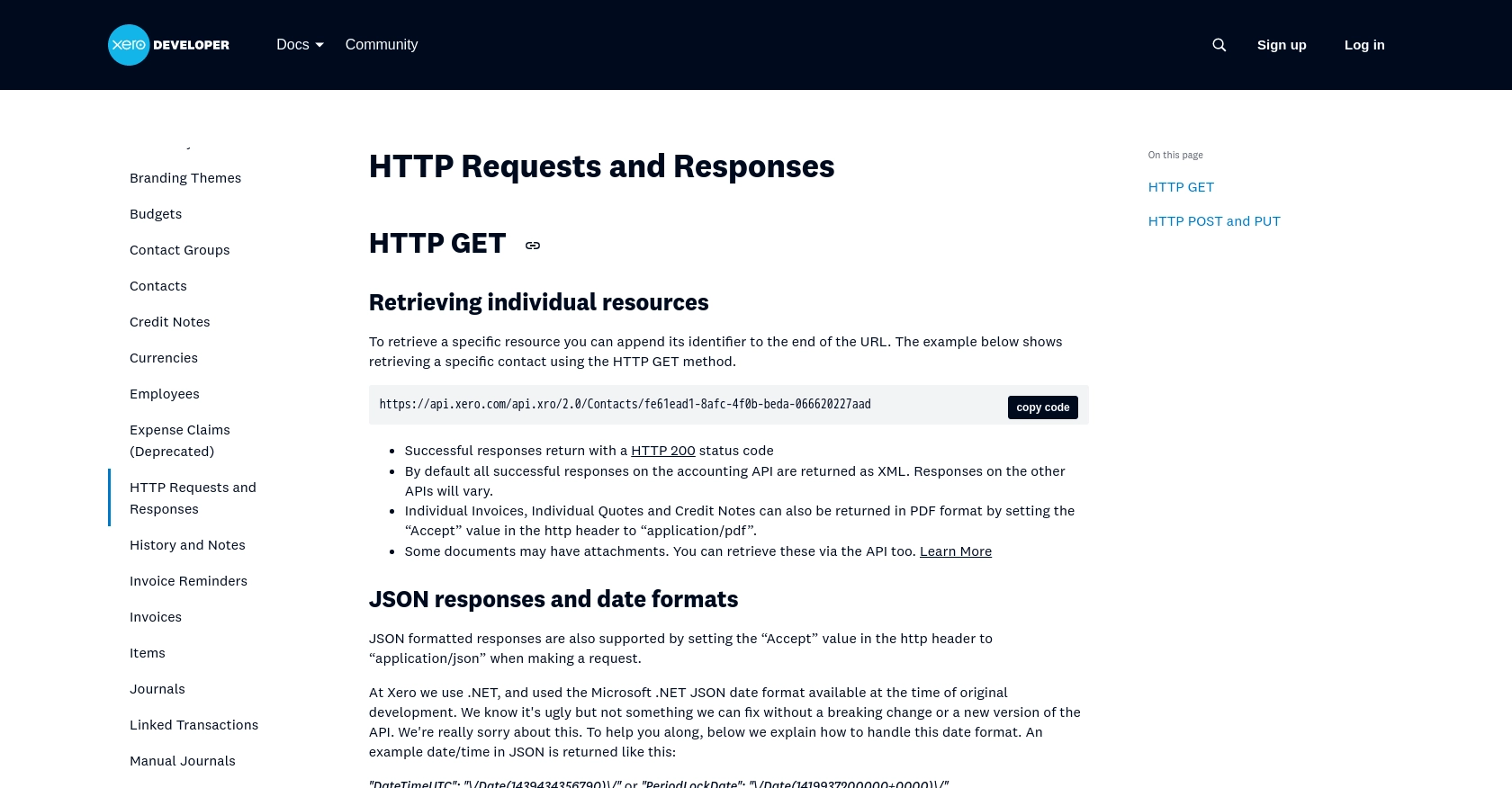
sbb-itb-96038d7
Making API Calls to Xero for Purchase Orders Using JavaScript
Now that you have your Xero sandbox account and OAuth 2.0 authentication set up, it's time to dive into making API calls to create or update purchase orders using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Xero API Integration
Before making API calls, ensure your development environment is ready:
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the necessary dependencies, such as Axios for making HTTP requests, by running
npm install axios
.
Creating or Updating Purchase Orders with Xero API
With your environment set up, you can now write the JavaScript code to interact with the Xero API:
const axios = require('axios');
// Set the API endpoint for purchase orders
const endpoint = 'https://api.xero.com/api.xro/2.0/PurchaseOrders';
// Set the access token and headers
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to create or update a purchase order
async function createOrUpdatePurchaseOrder(purchaseOrderData) {
try {
const response = await axios.post(endpoint, purchaseOrderData, { headers });
console.log('Purchase Order Response:', response.data);
} catch (error) {
console.error('Error creating or updating purchase order:', error.response ? error.response.data : error.message);
}
}
// Sample purchase order data
const purchaseOrderData = {
PurchaseOrders: [
{
Contact: {
Name: 'Sample Supplier'
},
Date: '2023-10-01',
LineItems: [
{
Description: 'Sample Item',
Quantity: 10,
UnitAmount: 100
}
]
}
]
};
// Call the function with sample data
createOrUpdatePurchaseOrder(purchaseOrderData);
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth 2.0 setup. The code above demonstrates how to create or update a purchase order by sending a POST request to the Xero API endpoint.
Verifying API Call Success and Handling Errors
After running the code, verify the success of your API call:
- Check the response data printed in the console to ensure the purchase order was created or updated successfully.
- Log in to your Xero sandbox account and navigate to the purchase orders section to confirm the changes.
Handle potential errors by checking the error response. The code snippet above includes basic error handling to log any issues encountered during the API call.
For more detailed error information, refer to the Xero API documentation.
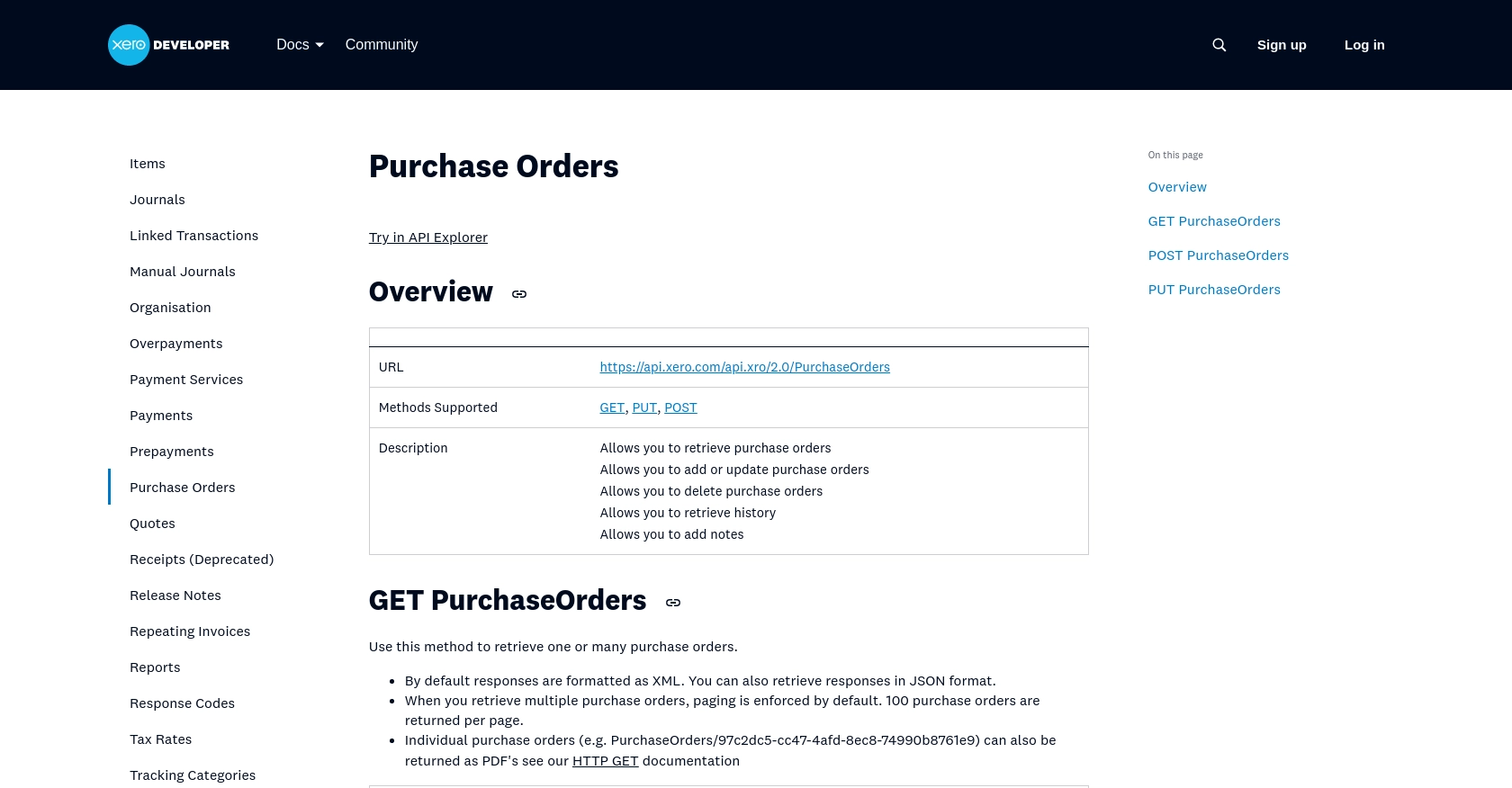
Best Practices for Xero API Integration and Error Handling
Successfully integrating with the Xero API requires adherence to best practices to ensure smooth and efficient operations. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. Be sure to implement logic to handle HTTP status code 429, which indicates too many requests. Refer to the Xero OAuth 2.0 API limits for more details.
- Standardize Data Fields: Ensure that the data fields used in your purchase orders are consistent with Xero's requirements. This helps prevent errors and ensures data integrity.
- Implement Robust Error Handling: Use try-catch blocks to manage errors effectively. Log error details for troubleshooting and refer to the Xero API documentation for guidance on handling specific error codes.
Streamlining Integration with Endgrate
While integrating with the Xero API can enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Xero.
With Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, eliminating the need to create multiple integrations for different platforms.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a streamlined integration strategy.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/purchaseorders
Ready to get started?