How to Create or Update Candidates with the Recruitee API in PHP
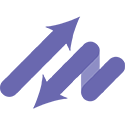
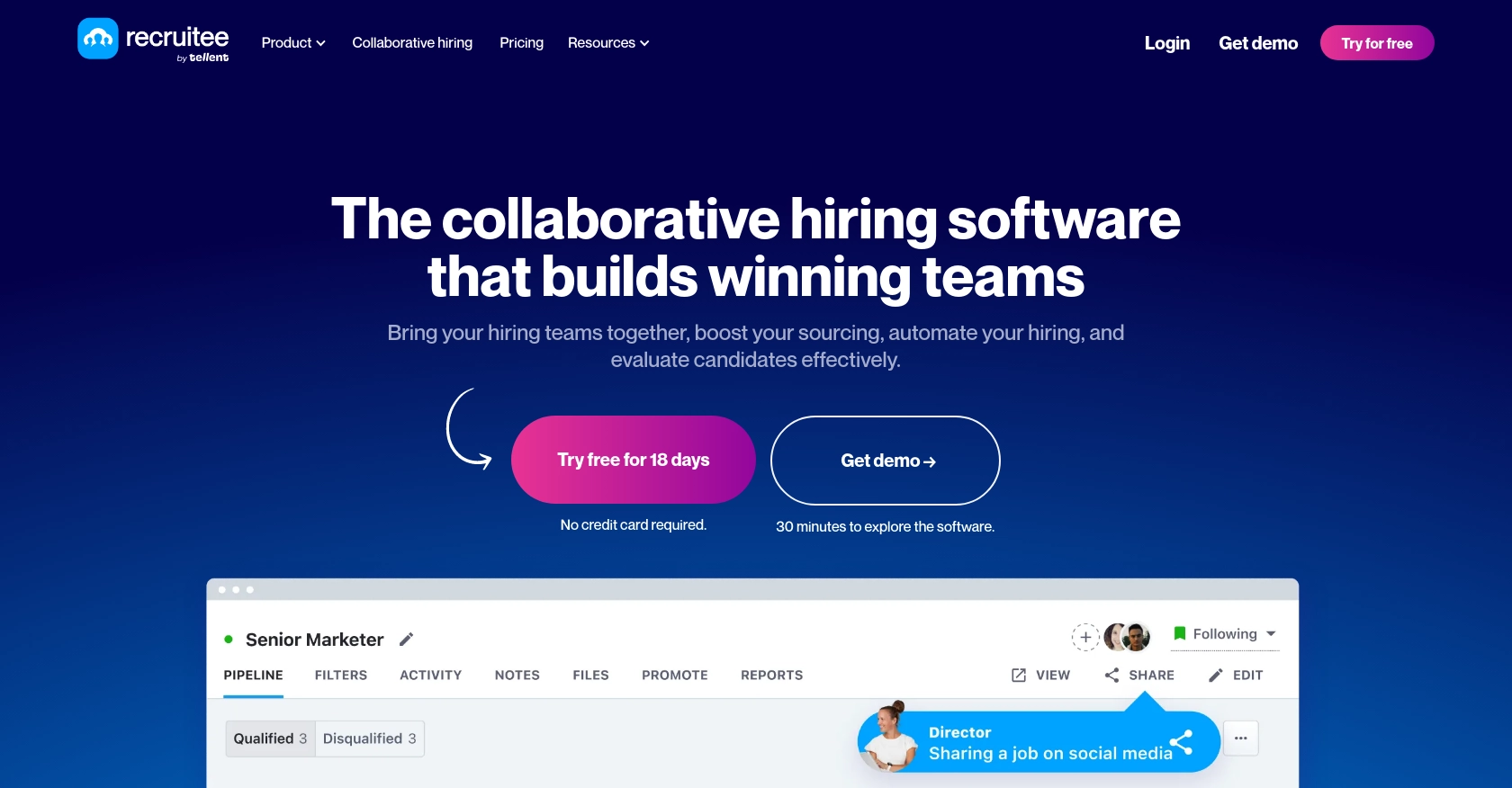
Introduction to Recruitee API for Candidate Management
Recruitee is a powerful applicant tracking system (ATS) designed to streamline the recruitment process for businesses of all sizes. It offers a comprehensive suite of tools that assist in managing job postings, tracking candidates, and collaborating with hiring teams.
Integrating with Recruitee's API allows developers to automate and enhance recruitment workflows. For example, you can programmatically create or update candidate profiles directly from an external application, ensuring that your candidate database is always up-to-date and reducing manual data entry.
Setting Up a Recruitee Test Account for API Integration
Before you can start integrating with the Recruitee API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Recruitee provides a straightforward way to generate API tokens, which are essential for authenticating your requests.
Creating a Recruitee Account
If you don't already have a Recruitee account, you can sign up for a free trial on their website. This will give you access to the necessary features to test API integrations.
- Visit the Recruitee website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating a Personal API Token
Recruitee uses API tokens for authentication. Follow these steps to generate your token:
- Navigate to Settings in the top navigation bar.
- Select Apps and plugins from the sidebar.
- Click on Personal API tokens.
- Click + New token in the top-right corner to generate a new token.
- Copy the generated token and store it securely, as you'll need it for API requests.
Note: The API token does not expire unless manually revoked, providing a stable authentication method for your integration.
For more detailed information, refer to the Recruitee API documentation.
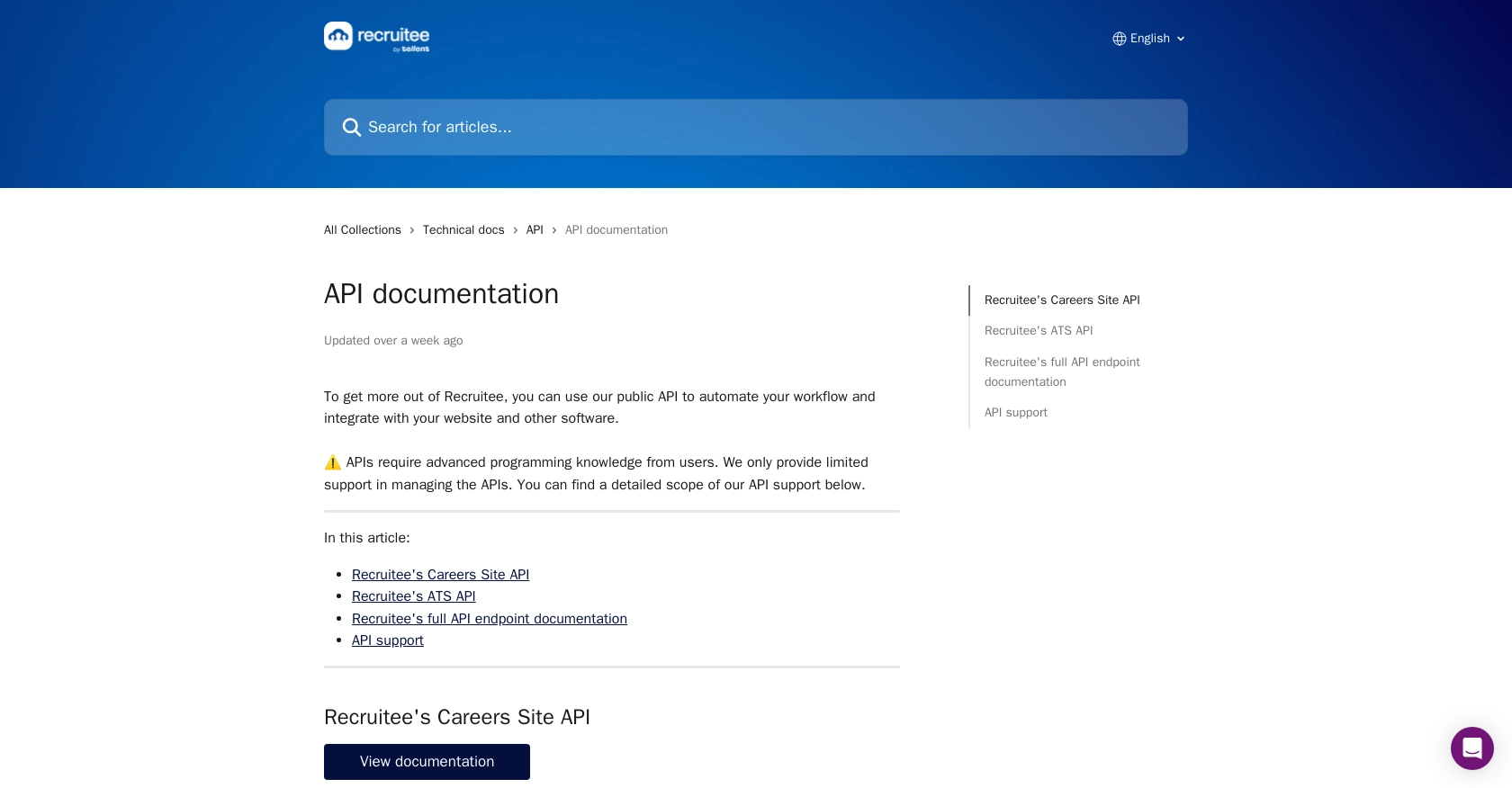
sbb-itb-96038d7
Making API Calls to Create or Update Candidates with Recruitee in PHP
To interact with the Recruitee API for creating or updating candidate profiles, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses and errors effectively.
Setting Up Your PHP Environment for Recruitee API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for managing dependencies
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Creating a Candidate with the Recruitee API Using PHP
To create a new candidate in Recruitee, you'll need to make a POST request to the appropriate endpoint. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$company_id = 'your_company_id';
$api_token = 'your_api_token';
$response = $client->post("https://api.recruitee.com/c/{$company_id}/candidates", [
'headers' => [
'Authorization' => "Bearer {$api_token}",
'Content-Type' => 'application/json',
],
'json' => [
'candidate' => [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'phone' => '1234567890',
],
],
]);
if ($response->getStatusCode() === 201) {
echo "Candidate created successfully!";
} else {
echo "Failed to create candidate.";
}
Replace your_company_id
and your_api_token
with your actual Recruitee company ID and API token. This code sends a POST request to create a candidate with basic information such as name, email, and phone number.
Updating an Existing Candidate with the Recruitee API Using PHP
To update an existing candidate, you'll need to make a PATCH request to the candidate's specific endpoint. Here's how you can do it:
$response = $client->patch("https://api.recruitee.com/c/{$company_id}/candidates/{candidate_id}", [
'headers' => [
'Authorization' => "Bearer {$api_token}",
'Content-Type' => 'application/json',
],
'json' => [
'candidate' => [
'name' => 'Jane Doe',
'email' => 'jane.doe@example.com',
],
],
]);
if ($response->getStatusCode() === 200) {
echo "Candidate updated successfully!";
} else {
echo "Failed to update candidate.";
}
Replace {candidate_id}
with the ID of the candidate you wish to update. This code updates the candidate's name and email address.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Check the status code of the response to determine if the request was successful. Common status codes include:
- 201: Created successfully
- 200: Updated successfully
- 400: Bad request, check your input data
- 401: Unauthorized, verify your API token
For more detailed error handling, refer to the Recruitee API documentation.
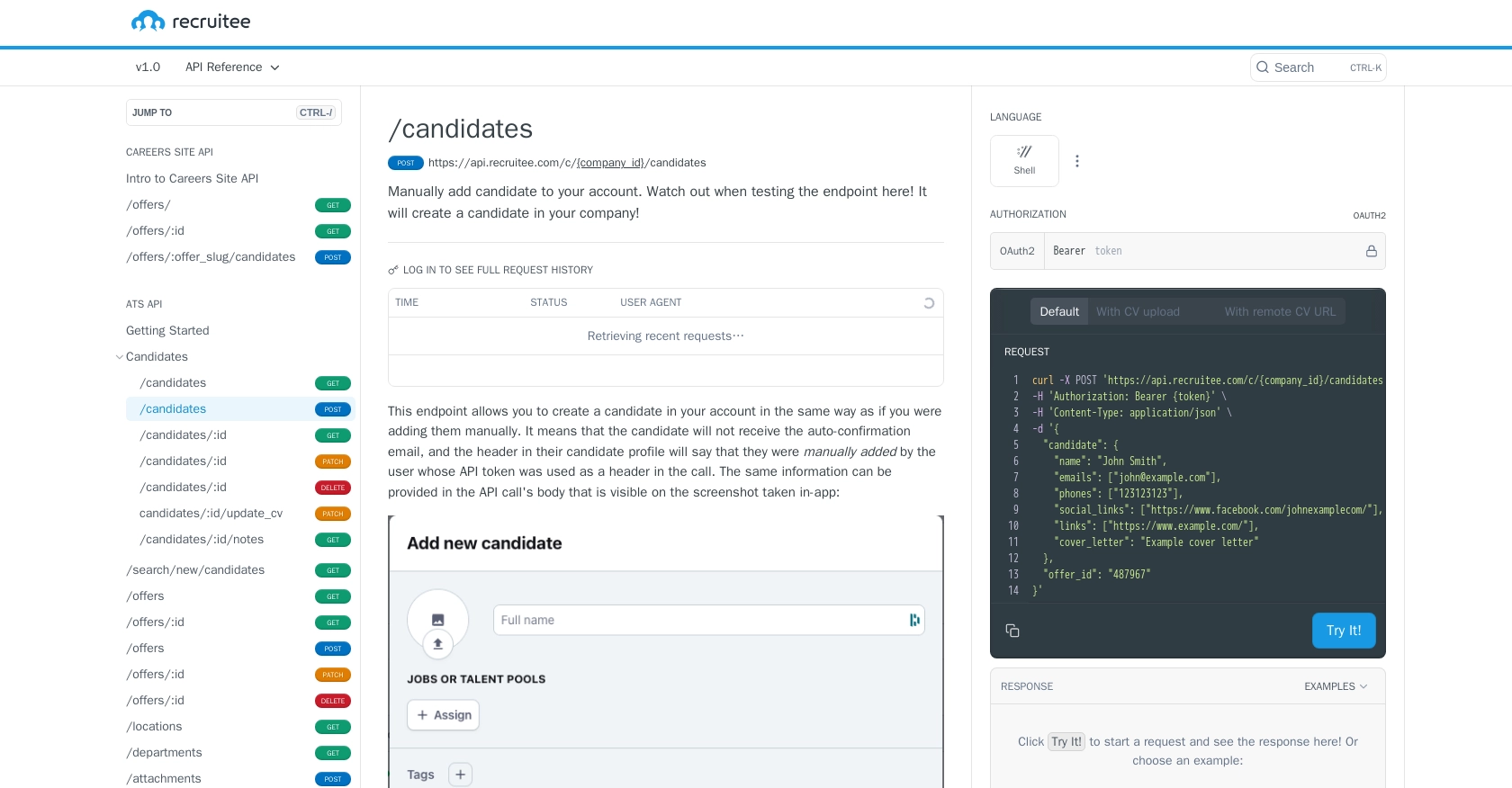
Conclusion and Best Practices for Recruitee API Integration in PHP
Integrating with the Recruitee API using PHP allows developers to efficiently manage candidate data, streamlining recruitment processes and reducing manual data entry. By following the steps outlined in this guide, you can create and update candidate profiles programmatically, ensuring your recruitment database remains current and accurate.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Tokens: Always store your API tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Validation: Validate and sanitize input data before making API calls to prevent errors and ensure data integrity.
- Error Handling: Implement comprehensive error handling to manage different response codes and provide meaningful feedback to users.
Enhancing Your Integration with Endgrate
While integrating with Recruitee's API directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Recruitee.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?