Using the Keap API to Create or Update Contacts (with PHP examples)
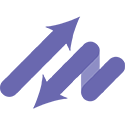
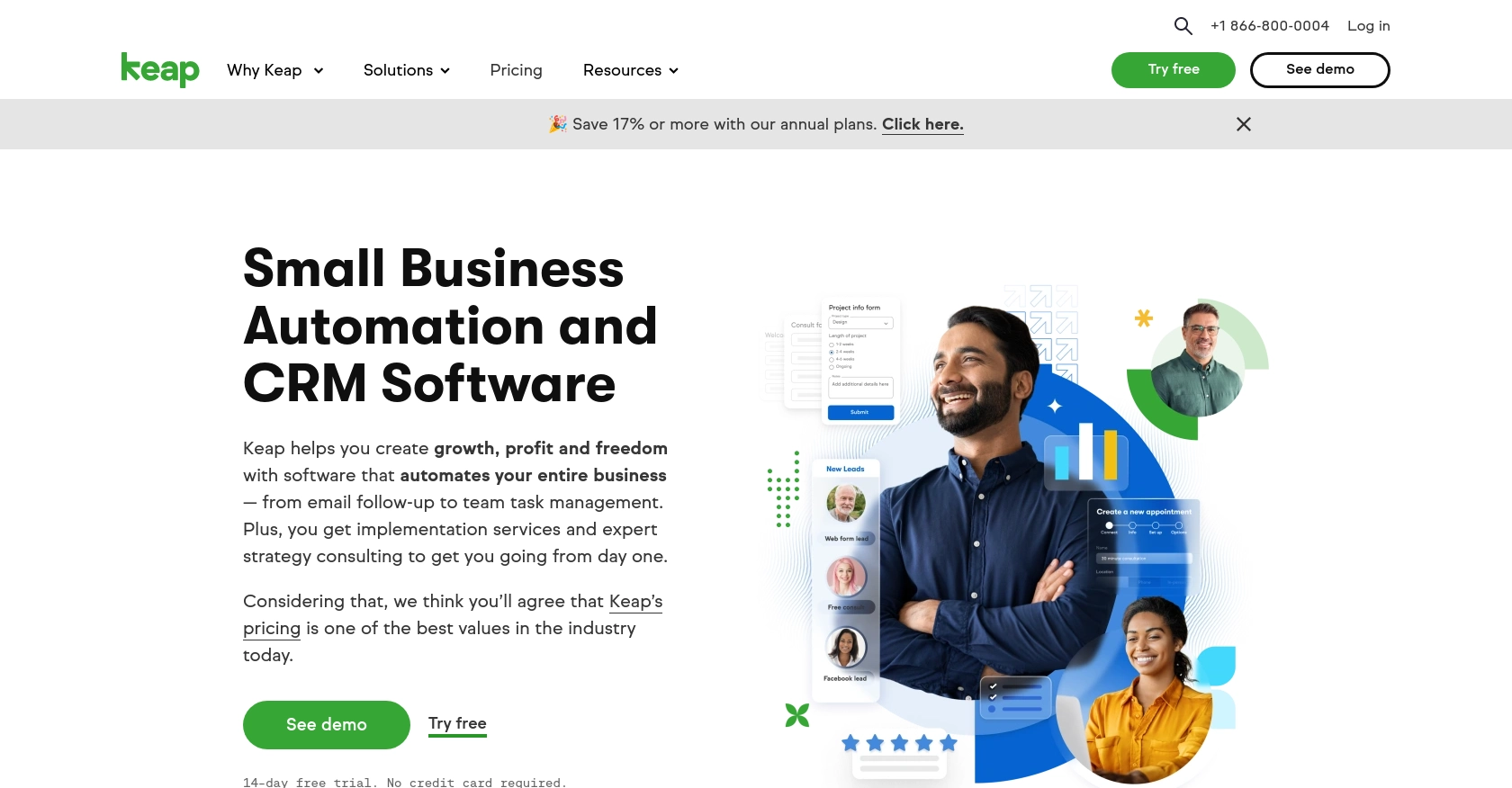
Introduction to Keap CRM Integration
Keap, formerly known as Infusionsoft, is a robust CRM platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and automation, Keap empowers businesses to nurture leads and enhance customer relationships.
Integrating with Keap's API allows developers to automate and manage customer interactions efficiently. For example, you can use the Keap API to create or update contact information directly from your application, ensuring that your CRM data is always up-to-date and accurate.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your API interactions without affecting live data. Follow these steps to get started:
Create a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources for building your integration.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your developer account.
Set Up a Keap Sandbox App
With your developer account ready, the next step is to create a sandbox app. This environment will allow you to safely test your API calls.
- Navigate to the "Sandbox" section in your developer account dashboard.
- Click on "Create Sandbox App" to initiate the setup process.
- Provide a name and description for your sandbox app.
- Submit the form to create your sandbox environment.
Configure OAuth Authentication for Keap API
The Keap API uses OAuth for authentication, which requires setting up an app to obtain client credentials.
- In your developer dashboard, go to the "Apps" section and click "Create App."
- Enter the required information, including the app name and redirect URI.
- After creating the app, you'll receive a client ID and client secret. Keep these credentials secure as you'll need them for API authentication.
For more detailed guidance, refer to the Keap REST API documentation.
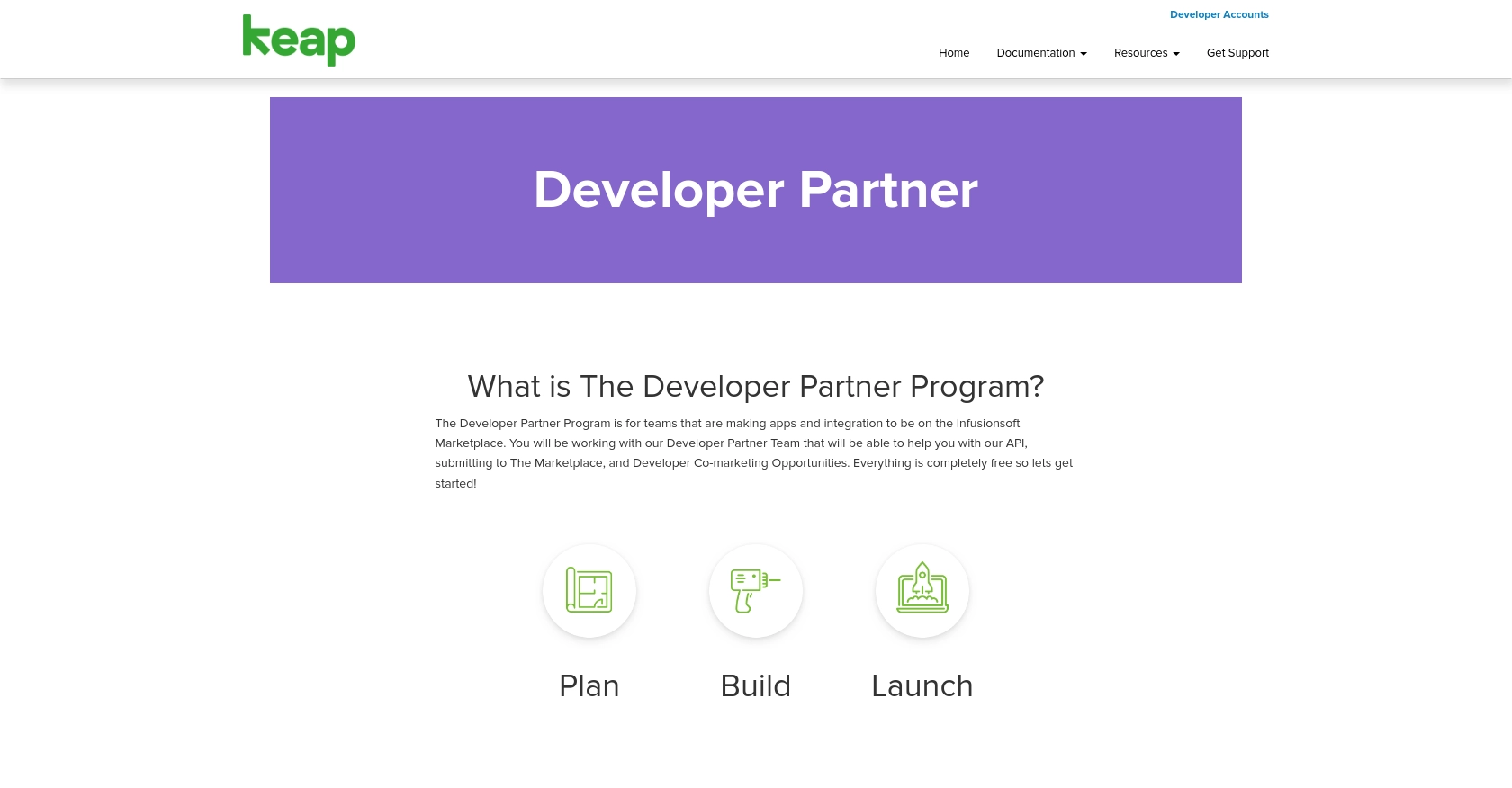
sbb-itb-96038d7
Making API Calls to Keap for Contact Management Using PHP
To interact with the Keap API for creating or updating contacts, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for Keap API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled in yourphp.ini
file.
Installing Required PHP Dependencies
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Contacts with Keap API Using PHP
Now, let's write a PHP script to create or update contacts in Keap. This example demonstrates how to authenticate and send a POST request to the Keap API.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your OAuth access token
try {
$response = $client->request('POST', 'https://api.infusionsoft.com/crm/rest/v1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
],
'json' => [
'given_name' => 'John',
'family_name' => 'Doe',
'email_addresses' => [
['email' => 'john.doe@example.com', 'field' => 'EMAIL1']
]
]
]);
if ($response->getStatusCode() === 200) {
echo "Contact created or updated successfully.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace YOUR_ACCESS_TOKEN
with the OAuth token obtained during authentication. This script sends a POST request to create or update a contact with the specified details.
Verifying API Call Success in Keap Sandbox
After running the script, verify the contact creation or update in your Keap sandbox environment. Log in to your sandbox account and check the contacts list to ensure the changes reflect correctly.
Handling Errors and Common Error Codes
When making API calls, handle potential errors gracefully. Common HTTP status codes you might encounter include:
- 400 Bad Request: The request was invalid. Check your request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on Keap's server. Try again later.
Use exception handling in your PHP code to catch and respond to these errors appropriately.
Best Practices for Keap API Integration
When working with the Keap API, it's essential to follow best practices to ensure a secure and efficient integration. Here are some key recommendations:
- Securely Store OAuth Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Keap's API rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully. For specific rate limit details, refer to the Keap REST API documentation.
- Data Standardization: Ensure that the data you send to Keap is standardized and validated. This helps maintain data integrity and prevents errors during API calls.
Leveraging Endgrate for Seamless Keap Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including Keap.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of integration.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundancy.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?