How to Get Policies with the Applied Epic API in Python
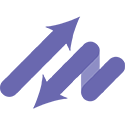
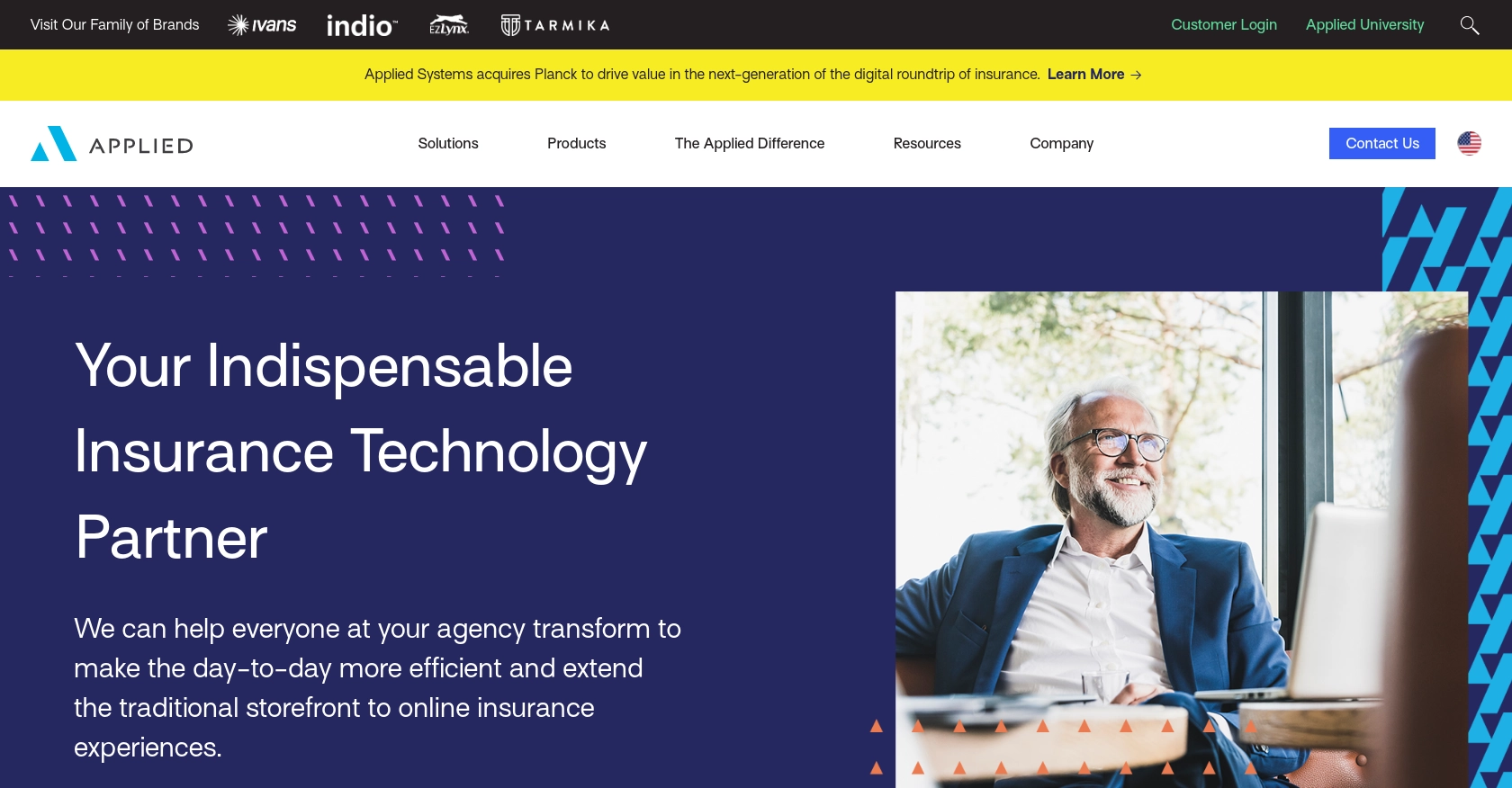
Introduction to Applied Epic API
Applied Epic is a comprehensive insurance management platform that offers a wide range of tools for managing policies, claims, and customer relationships. It is widely used by insurance agencies to streamline operations and enhance customer service.
Integrating with the Applied Epic API allows developers to access and manage policy data programmatically. For example, a developer might use the API to retrieve a list of policies for a specific client, enabling seamless integration with other systems for reporting or analytics purposes.
Setting Up Your Applied Epic Test Account
Before you can start interacting with the Applied Epic API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting real data.
Creating an Applied Epic Developer Account
To begin, you need to sign up for a developer account on the Applied Epic Developer Portal. Follow these steps:
- Visit the Applied Epic Developer Portal.
- Click on the "Sign Up" button and fill in the required information to create your account.
- Once registered, log in to your account to access the developer dashboard.
Generating API Credentials for Applied Epic
After setting up your developer account, you need to generate API credentials to authenticate your requests:
- Navigate to the "API Credentials" section in your developer dashboard.
- Create a new application by providing necessary details such as the application name and description.
- Once the application is created, you will receive a client ID and client secret. Keep these credentials secure as they are essential for API authentication.
Understanding Custom Authentication for Applied Epic API
The Applied Epic API uses a custom authentication method. Here’s how you can set it up:
- Use the client ID and client secret obtained from your developer account to authenticate API requests.
- Refer to the Applied Epic API Documentation for detailed instructions on implementing the custom authentication flow.
With your test account and API credentials ready, you can now proceed to make API calls to the Applied Epic platform. This setup ensures that you can develop and test your integration efficiently and securely.
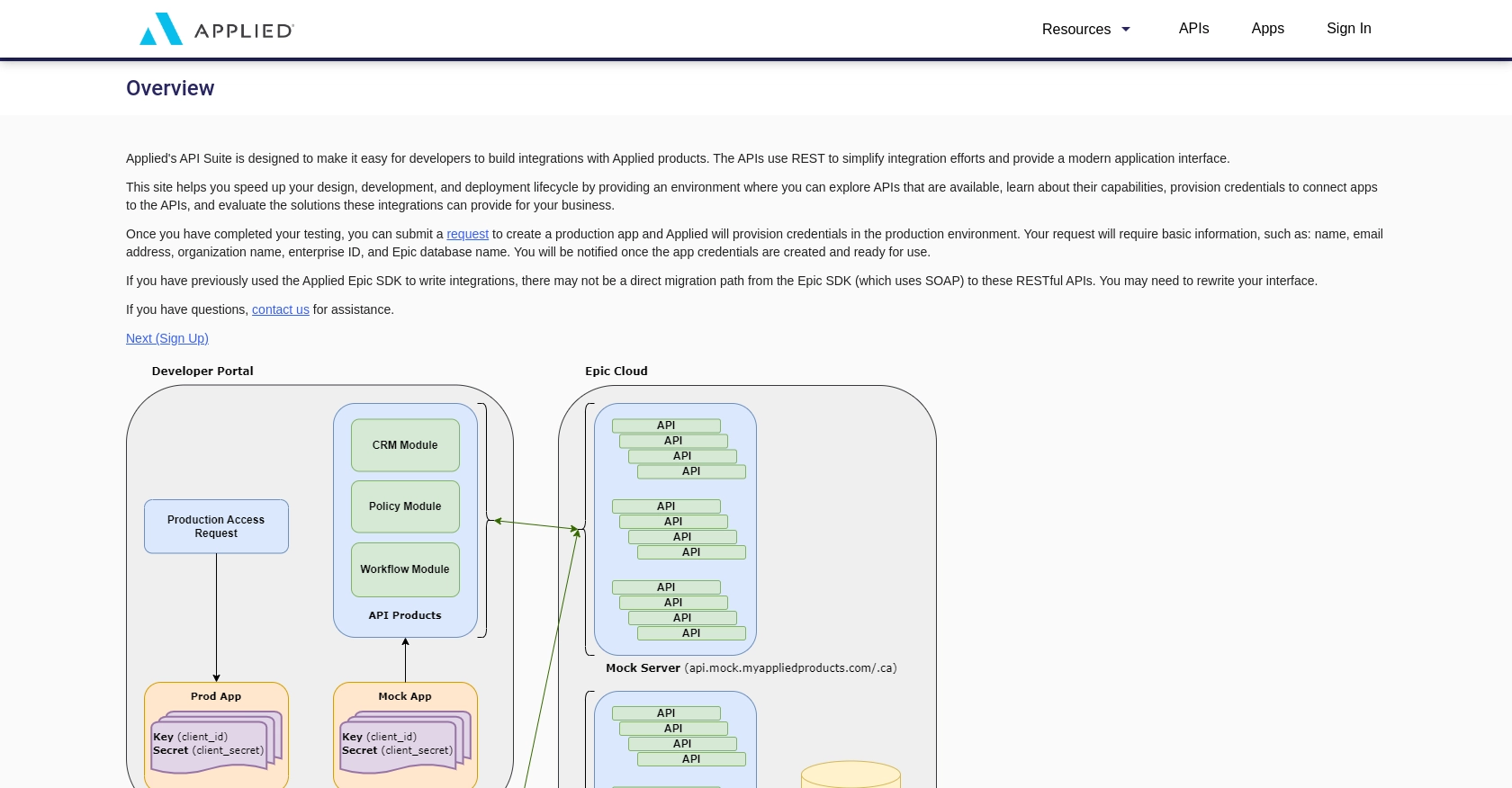
sbb-itb-96038d7
Making API Calls to Retrieve Policies from Applied Epic Using Python
To interact with the Applied Epic API and retrieve policy information, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the code, and executing API calls to fetch policies for a specific client.
Setting Up Your Python Environment for Applied Epic API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer,
pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Fetch Policies from Applied Epic API
Create a new Python file named get_applied_epic_policies.py
and add the following code:
import requests
# Set the API endpoint and headers
client_id = "your_client_id"
client_secret = "your_client_secret"
client_id_value = "your_client_id_value" # Replace with the actual client ID
endpoint = f"https://api.myappliedproducts.com/policy/v1/clients/{client_id_value}/policies"
headers = {
"Authorization": f"Bearer {client_id}:{client_secret}",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the policies and print their information
for policy in data["_embedded"]["policies"]:
print(f"Policy Number: {policy['policyNumber']}, Description: {policy['description']}")
else:
print(f"Failed to retrieve policies. Status Code: {response.status_code}")
Replace your_client_id
, your_client_secret
, and your_client_id_value
with your actual credentials and client ID.
Executing the Python Script to Retrieve Policies
Run the script from your terminal or command prompt using the following command:
python get_applied_epic_policies.py
If successful, you should see a list of policies printed in your terminal, each displaying the policy number and description.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The Applied Epic API may return various status codes indicating different outcomes:
- 200 OK: The request was successful, and policies are returned.
- 400 Bad Request: The request was malformed. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your credentials.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The specified client ID does not exist.
Always check the response status code and handle errors appropriately to ensure robust integration.
Conclusion and Best Practices for Applied Epic API Integration
Integrating with the Applied Epic API allows developers to efficiently manage and access policy data, enhancing the capabilities of insurance management systems. By following the steps outlined in this guide, you can successfully retrieve policy information using Python, ensuring seamless integration with other applications.
Best Practices for Secure and Efficient Applied Epic API Usage
- Securely Store Credentials: Always store your client ID and client secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Consider transforming and standardizing data fields to match your application's requirements, ensuring consistency across systems.
- Error Handling: Implement robust error handling to manage different API response codes, ensuring your application can gracefully handle failures.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Applied Epic. This allows you to focus on your core product while ensuring a seamless integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?