How to Create or Update Leads with the Close API in Python
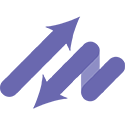
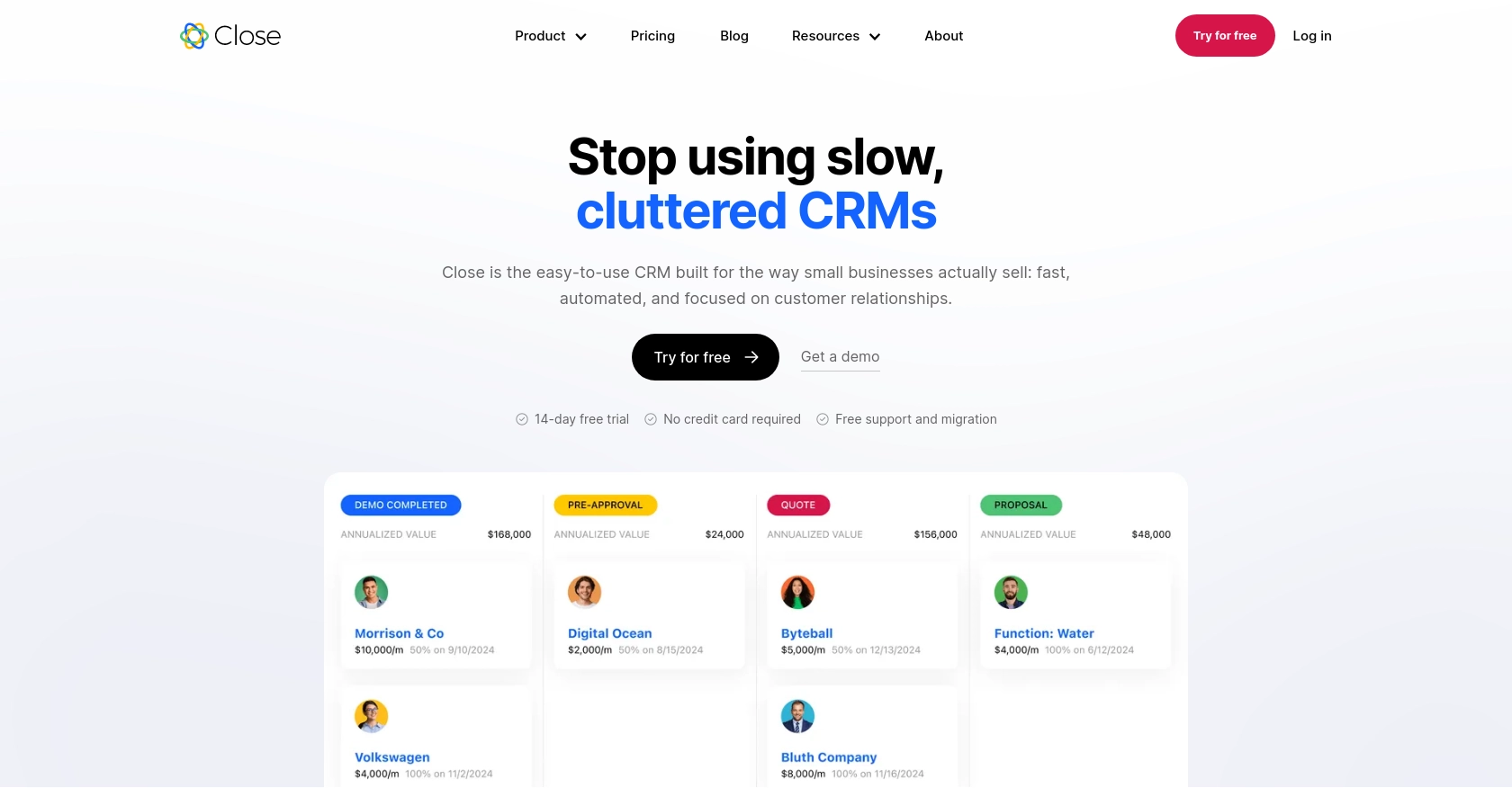
Introduction to Close CRM
Close is a powerful CRM platform designed to help businesses streamline their sales processes and improve customer relationship management. With its robust set of features, Close enables sales teams to manage leads, track communications, and automate workflows, making it a popular choice for businesses looking to enhance their sales efficiency.
Integrating with the Close API allows developers to automate and manage lead data effectively. For example, a developer might want to create or update leads in Close using Python to ensure that their sales team always has the most up-to-date information, enabling them to make informed decisions and close deals faster.
Setting Up Your Close CRM Test Account
Before you can start integrating with the Close API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial on the Close website. Follow the instructions to create your account and log in.
Generate an API Key for Authentication
Close uses API key-based authentication for secure access to its API. Here's how you can generate an API key:
- Log in to your Close account.
- Navigate to the Settings page.
- Under the API Keys section, click on Create API Key.
- Provide a name for your API key to help you identify it later.
- Click Create to generate the API key.
- Copy the API key and store it securely, as you'll need it for authentication in your Python scripts.
For more details on API key authentication, refer to the Close API Documentation.
Setting Up a Test Environment
To ensure that your API interactions are safe and isolated, it's recommended to use a test environment:
- Create a separate organization within Close for testing purposes.
- Use the API key generated for this test organization to make API calls.
- Regularly review and clean up test data to maintain a clutter-free environment.
By following these steps, you'll be ready to start integrating with the Close API using Python, ensuring a smooth and secure development process.
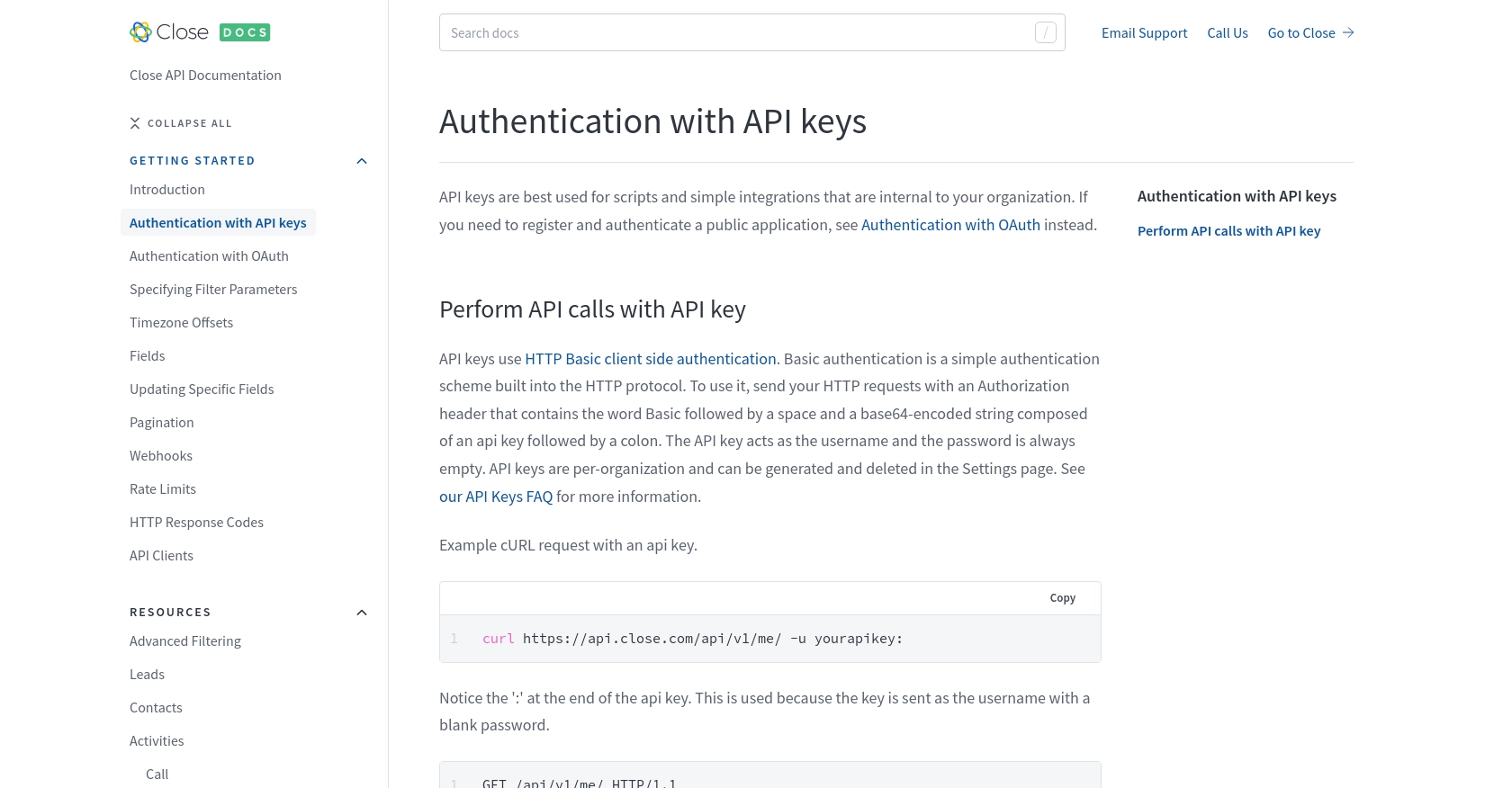
sbb-itb-96038d7
How to Make API Calls to Create or Update Leads in Close Using Python
In this section, we'll walk through the process of making API calls to create or update leads in Close using Python. This guide will cover the necessary Python version, dependencies, and provide example code to help you interact with the Close API effectively.
Prerequisites for Using Python with Close API
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or shell and install the requests
library using the following command:
pip install requests
Creating a Lead with Close API
To create a new lead in Close, you'll need to make a POST request to the Close API. Here's how you can do it using Python:
import requests
import json
# Set the API endpoint
url = "https://api.close.com/api/v1/lead/"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Basic your_api_key:"
}
# Define the lead data
lead_data = {
"name": "New Lead",
"contacts": [
{
"name": "John Doe",
"emails": [{"email": "john.doe@example.com"}]
}
]
}
# Make the POST request to create a lead
response = requests.post(url, headers=headers, data=json.dumps(lead_data))
# Check if the request was successful
if response.status_code == 200:
print("Lead created successfully:", response.json())
else:
print("Failed to create lead:", response.status_code, response.text)
Replace your_api_key
with the API key you generated earlier. This script sets up the API endpoint and headers, defines the lead data, and makes a POST request to create a new lead. If successful, it will print the details of the created lead.
Updating an Existing Lead with Close API
To update an existing lead, you'll need to make a PUT request. Here's an example:
import requests
import json
# Set the API endpoint with the lead ID
lead_id = "lead_id_here"
url = f"https://api.close.com/api/v1/lead/{lead_id}/"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Basic your_api_key:"
}
# Define the updated lead data
updated_data = {
"name": "Updated Lead Name"
}
# Make the PUT request to update the lead
response = requests.put(url, headers=headers, data=json.dumps(updated_data))
# Check if the request was successful
if response.status_code == 200:
print("Lead updated successfully:", response.json())
else:
print("Failed to update lead:", response.status_code, response.text)
Replace lead_id_here
with the ID of the lead you want to update and your_api_key
with your API key. This script updates the lead's name and checks if the request was successful.
Handling API Errors and Verifying Success
When making API calls, it's important to handle potential errors. The Close API uses standard HTTP response codes to indicate success or failure:
- 200: Request was successful.
- 400: There was an issue with the request.
- 401: Authentication is required.
- 404: The requested resource was not found.
For more details on HTTP response codes, refer to the Close API Documentation.
After making a successful API call, verify the changes by checking the lead data in your Close test environment.
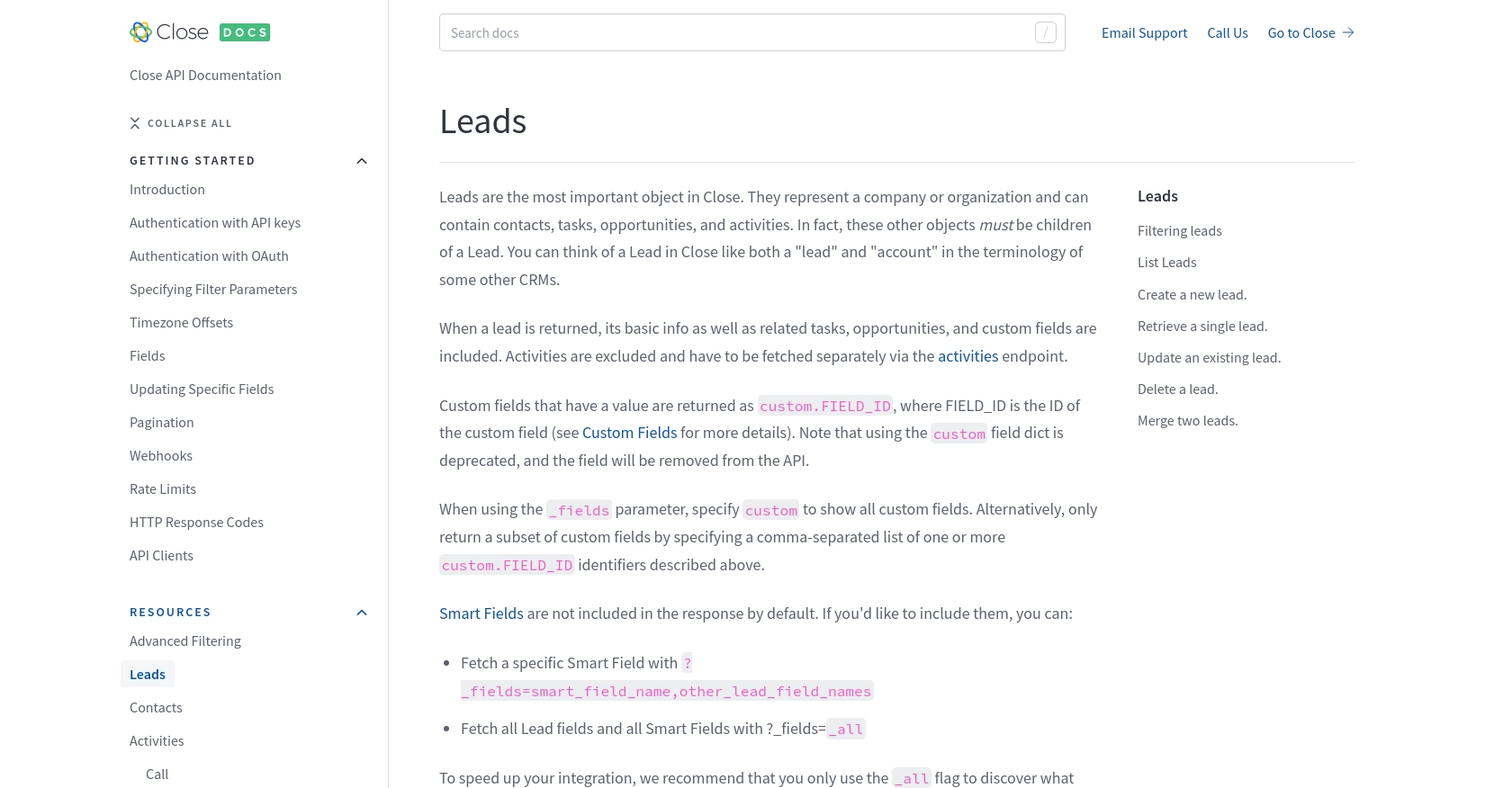
Conclusion: Best Practices for Using the Close API with Python
Integrating with the Close API using Python can significantly enhance your sales processes by automating lead management. However, to ensure a seamless experience, it's crucial to follow best practices.
Securely Store API Keys and Credentials
Always store your API keys securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
Handle Rate Limits and Optimize API Calls
The Close API enforces rate limits to maintain stability. If you encounter a 429 status code, pause your requests as specified by the rate_reset
value. For more details, refer to the Close API Rate Limits Documentation.
Transform and Standardize Data Fields
Ensure data consistency by transforming and standardizing fields before sending them to the API. This practice helps maintain data integrity across your systems.
Leverage Endgrate for Efficient Integration Management
Consider using Endgrate to streamline your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Close.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/leads/
Ready to get started?