Using the Xero API to Create or Update Accounts in Python
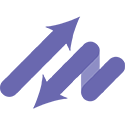
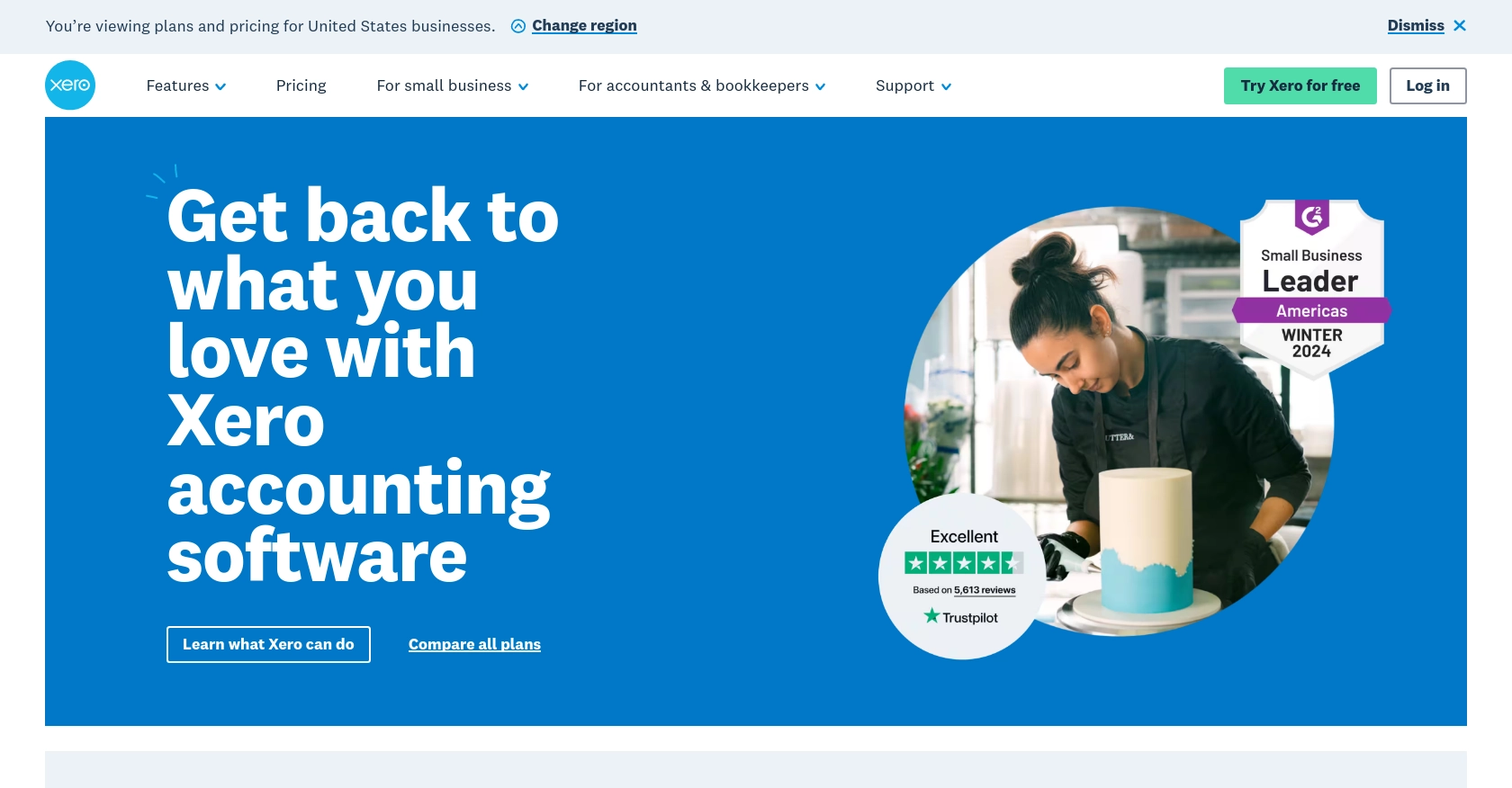
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features such as invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for businesses looking to streamline their financial operations.
Integrating with Xero's API allows developers to automate and enhance accounting processes, such as creating or updating accounts programmatically. For example, a developer might use the Xero API to automatically update account details based on transactions processed in another system, ensuring that financial data remains accurate and up-to-date.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before you can start integrating with the Xero API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your application without affecting live data. Xero provides a free trial or sandbox account specifically for developers to experiment with their API.
Creating a Xero Developer Account
To begin, you'll need to create a Xero developer account. Follow these steps:
- Visit the Xero Developer Portal.
- Sign up for a developer account if you don't already have one.
- Once registered, log in to your developer account.
Setting Up a Xero Sandbox Organization
After creating your developer account, you can set up a sandbox organization:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Create a new app by providing the necessary details, such as the app name and company URL.
- Once your app is created, you'll be able to connect it to a sandbox organization for testing.
Configuring OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for authentication. Here's how to set it up:
- In your app settings, note down the client ID and client secret. These are essential for OAuth 2.0 authentication.
- Set up the redirect URI, which is the URL to which users will be redirected after they authorize your app.
- Ensure you have the necessary scopes for accessing and managing accounts. Refer to the Xero OAuth 2.0 Scopes documentation for more details.
For a detailed guide on the OAuth 2.0 authentication flow, visit the Xero OAuth 2.0 Authentication Flow documentation.
Generating Access Tokens
With your app configured, you can now generate access tokens:
- Use the client ID and client secret to request an authorization code from Xero.
- Exchange the authorization code for an access token and a refresh token.
- Store these tokens securely, as they will be used to authenticate API requests.
Remember to handle token expiration and refresh tokens as per the guidelines provided in the Xero OAuth 2.0 Overview.
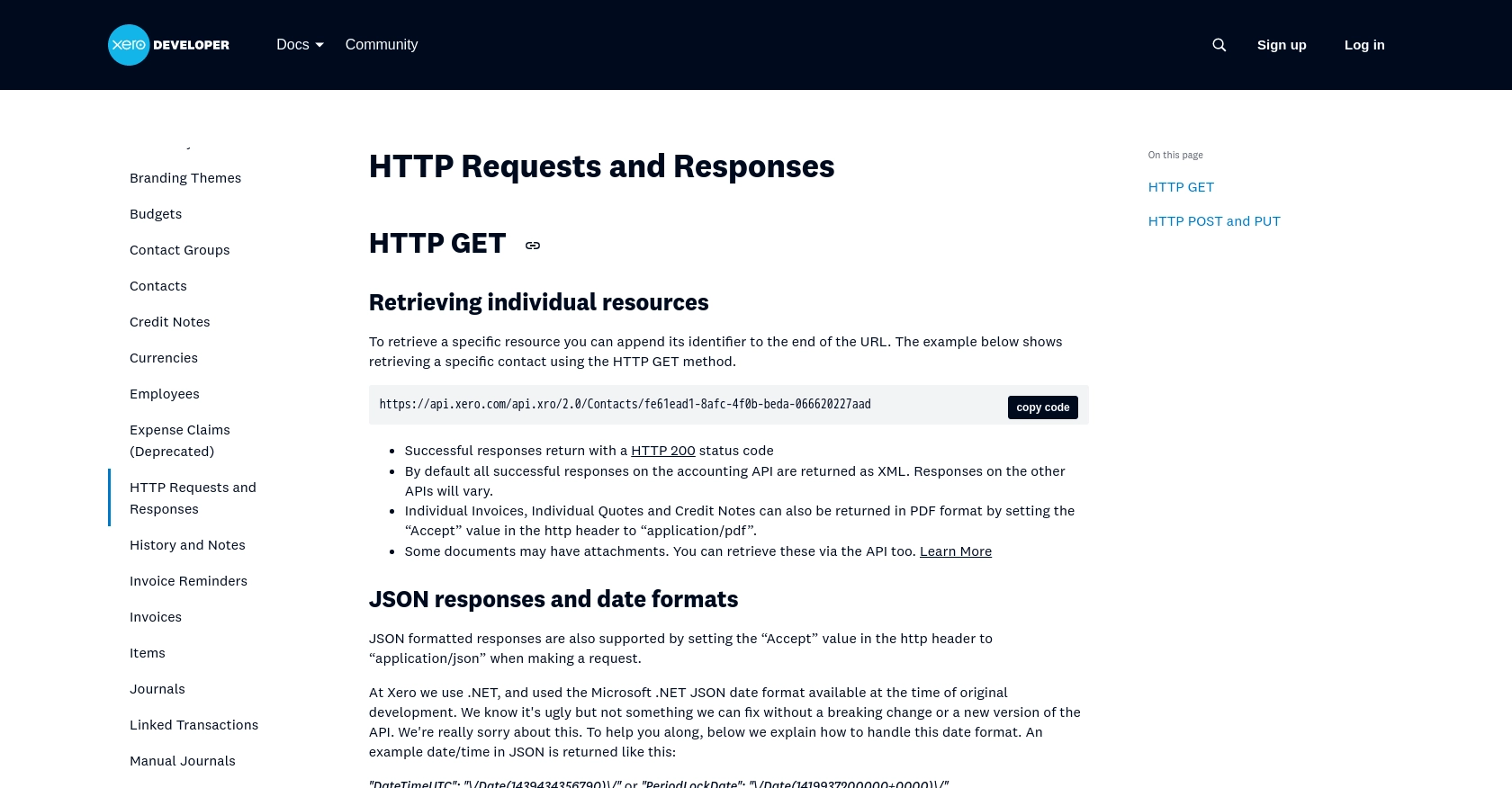
sbb-itb-96038d7
Making API Calls to Xero for Creating or Updating Accounts Using Python
To interact with the Xero API for creating or updating accounts, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment and making API calls to Xero.
Setting Up Your Python Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the necessary dependencies:
pip install requests
The requests
library will be used to handle HTTP requests to the Xero API.
Creating or Updating Accounts with Xero API in Python
Now, let's dive into the code to create or update accounts using the Xero API. Create a new Python file named xero_accounts.py
and add the following code:
import requests
# Set the API endpoint for creating or updating accounts
url = "https://api.xero.com/api.xro/2.0/Accounts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the account data
account_data = {
"Name": "Sample Account",
"Code": "123",
"Type": "EXPENSE"
}
# Send the POST request to create or update the account
response = requests.post(url, json=account_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Account created or updated successfully.")
else:
print(f"Failed to create or update account. Status code: {response.status_code}")
print(response.json())
Replace Your_Access_Token
with the access token you obtained during the OAuth 2.0 authentication setup.
Verifying API Call Success and Handling Errors
After running the script, you should verify the success of the API call by checking the response status code. A status code of 200 indicates success. If the request fails, the script will print the status code and error message returned by the API.
For more detailed error handling, refer to the Xero API Requests and Responses documentation.
Testing Your API Call in the Xero Sandbox
To ensure your API call works as expected, log in to your Xero sandbox account and verify that the account has been created or updated. This step is crucial for confirming that your integration is functioning correctly.
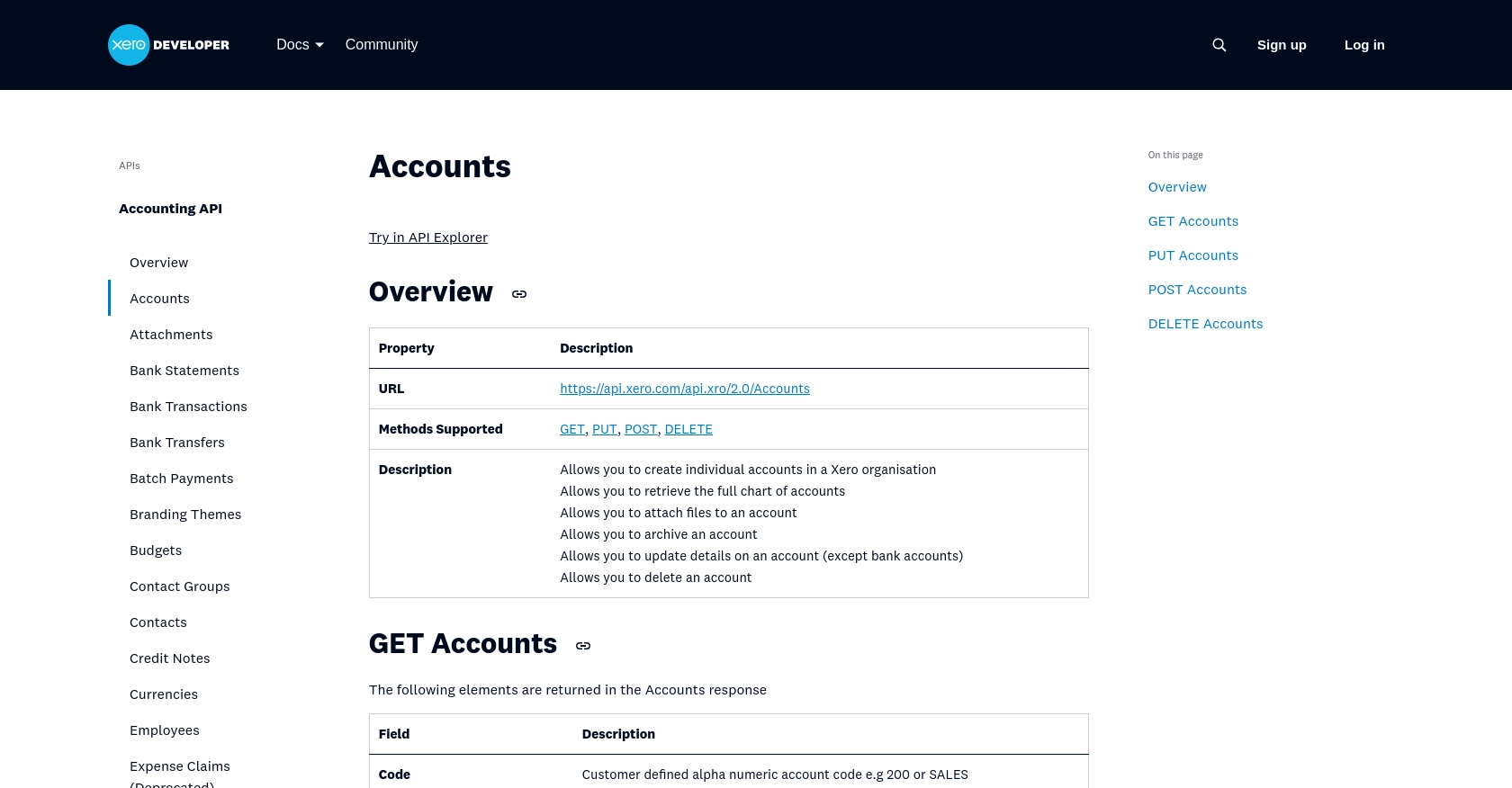
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API to create or update accounts in Python can significantly enhance your financial management processes by automating routine tasks and ensuring data accuracy. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth 2.0, and make API calls to manage accounts within Xero.
Best Practices for Secure and Efficient Xero API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handling Rate Limits: Be aware of Xero's API rate limits to avoid exceeding them. According to the Xero OAuth 2.0 API limits, ensure your application is designed to handle rate limiting gracefully by implementing retry logic with exponential backoff.
- Data Standardization: When dealing with financial data, ensure that your data fields are standardized and consistent with Xero's requirements to prevent errors during API calls.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the Xero API Requests and Responses documentation for detailed error codes and messages.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, reducing the complexity of maintaining multiple integrations. By outsourcing integrations, you can focus on your core product and provide an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by leveraging their unified API solution for seamless integration with platforms like Xero.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/accounts
Ready to get started?