How to Get Deals with the Hubspot API in PHP
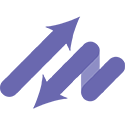
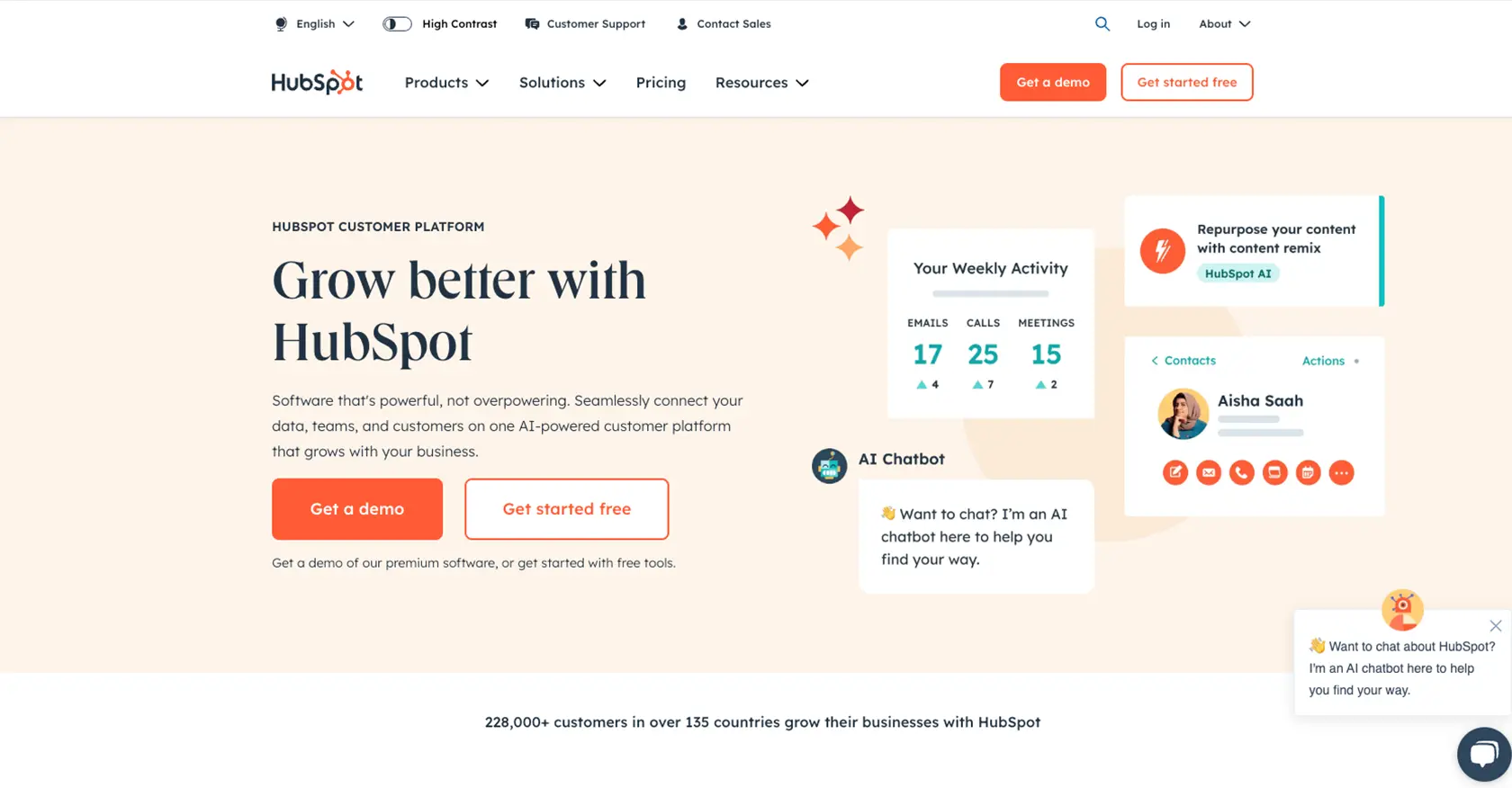
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a wide range of tools to enhance marketing, sales, and customer service efforts. Its robust API allows developers to seamlessly integrate HubSpot's functionalities into their own applications, enabling efficient data management and automation.
Integrating with the HubSpot API can be particularly beneficial for developers looking to manage sales processes. For example, retrieving deal information using the HubSpot API in PHP can help automate sales tracking and reporting, providing real-time insights into the sales pipeline.
This article will guide you through the process of using PHP to interact with the HubSpot API, specifically focusing on how to retrieve deals. By the end of this guide, you'll be equipped to efficiently access and manage deal data within the HubSpot platform.
Setting Up Your HubSpot Test Account for API Integration
Before diving into the HubSpot API to retrieve deals using PHP, you'll need to set up a HubSpot test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a HubSpot Developer Account
To get started, you'll need a HubSpot developer account. If you don't have one, follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and follow the registration process.
- Once registered, log in to your developer account.
Setting Up a HubSpot Sandbox Environment
HubSpot provides a sandbox environment for testing purposes. Here's how to set it up:
- In your developer account, navigate to the "Test Accounts" section.
- Create a new test account by following the on-screen instructions.
- This sandbox will mimic a real HubSpot account, allowing you to test API interactions.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for API authentication. Follow these steps to configure OAuth:
- In your developer account, go to "Apps" and click on "Create App."
- Fill in the necessary details for your app, such as name and description.
- Navigate to the "Auth" tab and select "OAuth" as your authentication method.
- Set up the necessary scopes for accessing deals, such as
crm.objects.deals.read
. - Save your app settings to generate a client ID and client secret.
Generating OAuth Tokens
With your app configured, you can now generate OAuth tokens:
- Use the client ID and client secret to request an access token from HubSpot's OAuth server.
- Follow the OAuth 2.0 flow to authenticate and obtain the access token.
- Store the access token securely, as it will be used in API requests.
For more details on OAuth setup, refer to the HubSpot Authentication Documentation.
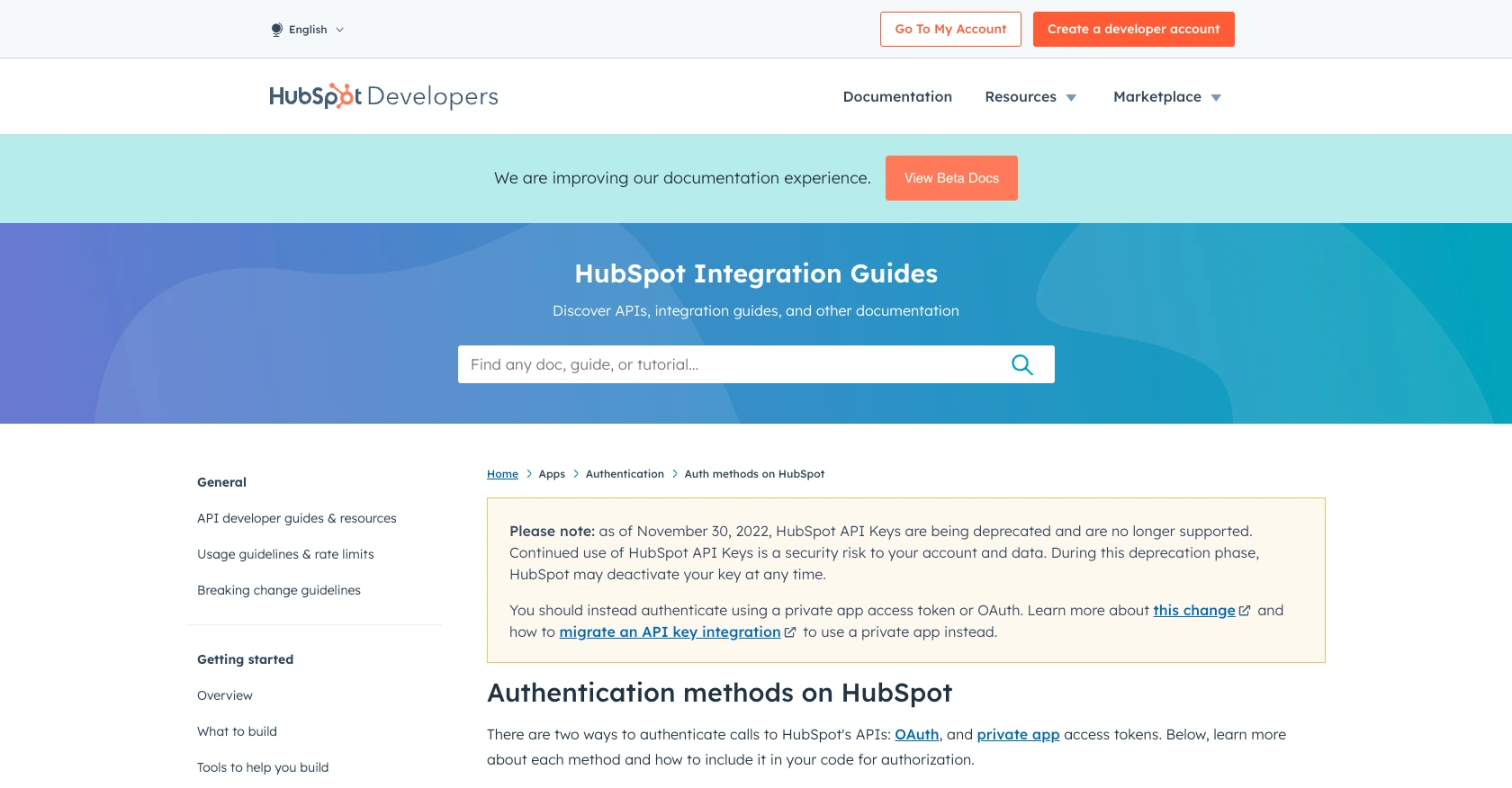
sbb-itb-96038d7
Making API Calls to Retrieve Deals from HubSpot Using PHP
To interact with the HubSpot API and retrieve deals using PHP, you need to ensure that your development environment is properly set up. This section will guide you through the necessary steps, including installing dependencies, writing the PHP code, and handling potential errors.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Install the HubSpot API client for PHP using Composer with the following command:
composer require hubspot/api-client
Writing PHP Code to Retrieve Deals from HubSpot
Once your environment is ready, you can write the PHP script to fetch deals from HubSpot. Create a file named get_hubspot_deals.php
and add the following code:
require 'vendor/autoload.php';
use HubSpot\Factory;
use HubSpot\Client\Crm\Deals\ApiException;
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
$hubSpot = Factory::createWithAccessToken($accessToken);
try {
$response = $hubSpot->crm()->deals()->basicApi()->getPage();
$deals = $response->getResults();
foreach ($deals as $deal) {
echo "Deal ID: " . $deal->getId() . "\n";
echo "Deal Name: " . $deal->getProperties()['dealname'] . "\n";
echo "Amount: " . $deal->getProperties()['amount'] . "\n\n";
}
} catch (ApiException $e) {
echo "Exception when calling HubSpot API: ", $e->getMessage(), PHP_EOL;
}
Replace Your_Access_Token
with the OAuth token you generated earlier. This script initializes the HubSpot client, retrieves a list of deals, and prints their details.
Verifying API Call Success and Handling Errors
After running the script, you should see the details of the deals printed in your terminal. To verify the success of the API call, check your HubSpot sandbox account to ensure the data matches the output.
If an error occurs, the script will catch the exception and display an error message. Common errors include:
- 401 Unauthorized: Check if the access token is valid and has the necessary scopes.
- 429 Too Many Requests: You have exceeded the rate limit. HubSpot allows 100 requests every 10 seconds for OAuth apps. Consider implementing a retry mechanism.
For more details on error codes, refer to the HubSpot Deals API Documentation.
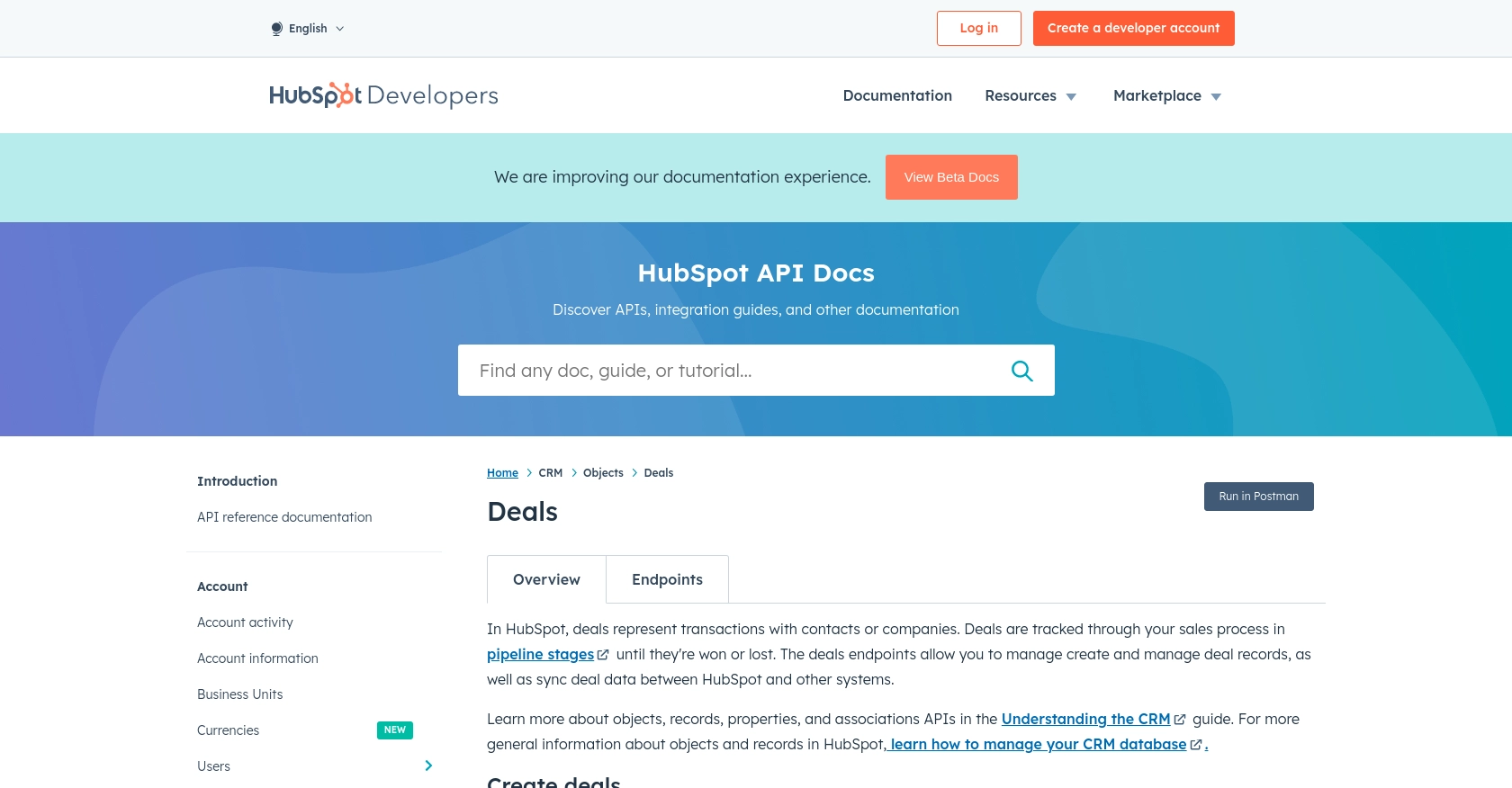
Conclusion and Best Practices for HubSpot API Integration in PHP
Integrating with the HubSpot API using PHP allows developers to efficiently manage and automate sales processes by accessing deal data. This guide has walked you through setting up a HubSpot developer account, configuring OAuth authentication, and retrieving deals using PHP.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store Credentials: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: HubSpot enforces a rate limit of 100 requests every 10 seconds for OAuth apps. Implement retry mechanisms to handle 429 errors gracefully.
- Optimize Data Handling: Consider caching frequently accessed data to reduce API calls and improve performance.
- Use Granular Scopes: When setting up OAuth, choose the most specific scopes needed for your application to enhance security.
Enhancing Your Integration Experience with Endgrate
While integrating with HubSpot directly can be powerful, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including HubSpot. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/deals
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?