How to Get Accounts with the Salesflare API in PHP
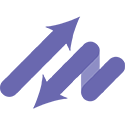
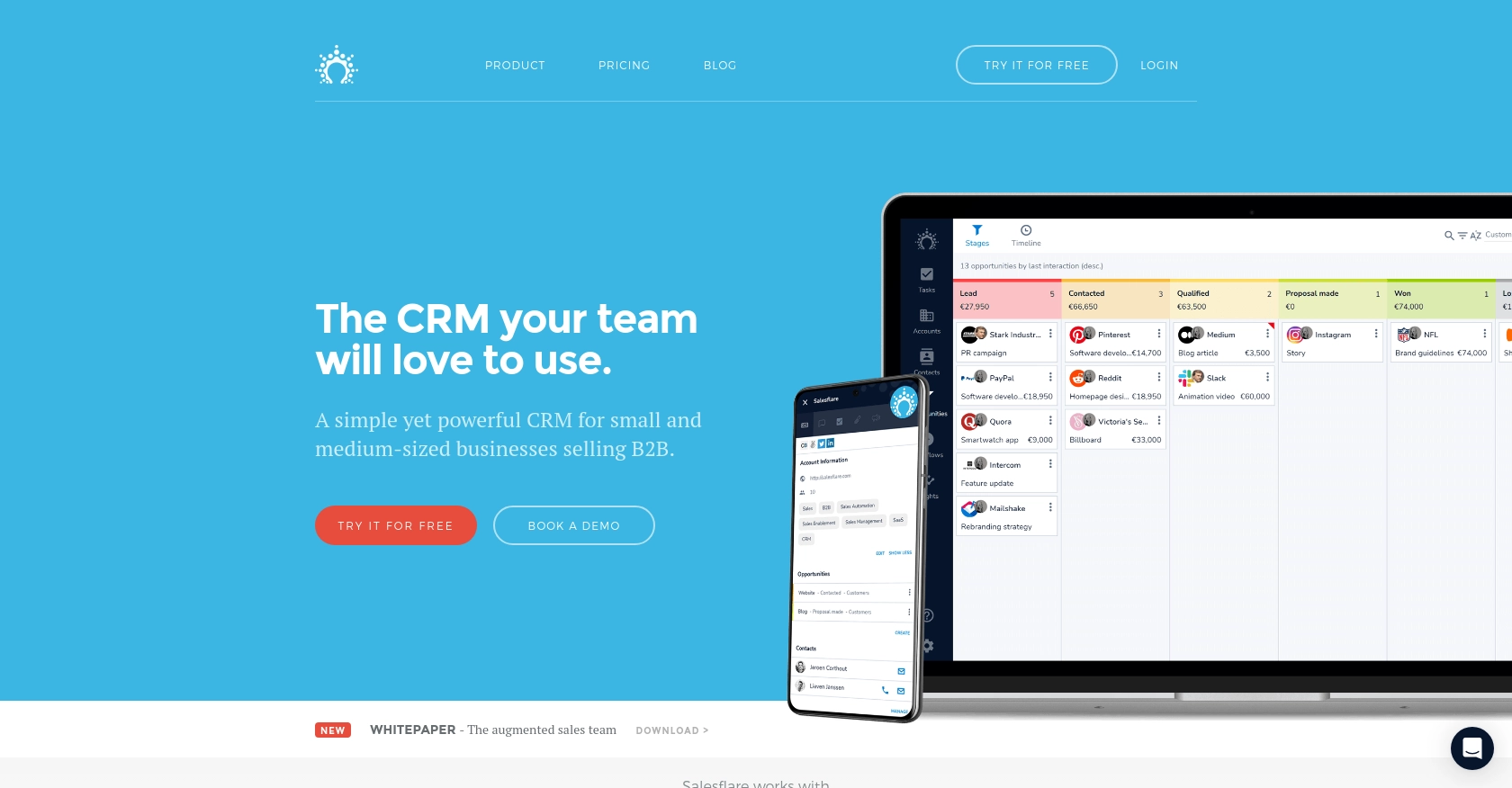
Introduction to Salesflare API Integration
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a range of features that help sales teams manage leads, track customer interactions, and automate repetitive tasks, all within a user-friendly interface.
Integrating with Salesflare's API allows developers to access and manage account data programmatically, enhancing the efficiency of sales operations. For example, a developer might use the Salesflare API to retrieve account information and sync it with other business tools, ensuring that sales teams have up-to-date data at their fingertips.
Setting Up Your Salesflare Test Account
Before you can start interacting with the Salesflare API, you'll need to set up a test account. Salesflare offers a straightforward process to get started, allowing developers to explore its features and functionalities.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on the Salesflare website. This trial will give you access to the platform's features, enabling you to test API interactions without any initial cost.
- Visit the Salesflare website.
- Click on the "Try for Free" button.
- Follow the on-screen instructions to create your account.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Once your account is set up, you can generate an API key to authenticate your requests.
- Log in to your Salesflare account.
- Navigate to the "Settings" section.
- Find the "API Keys" tab and click on it.
- Click on "Generate New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your API key ready, you're all set to start making API calls to Salesflare. Remember to keep your API key confidential to prevent unauthorized access.
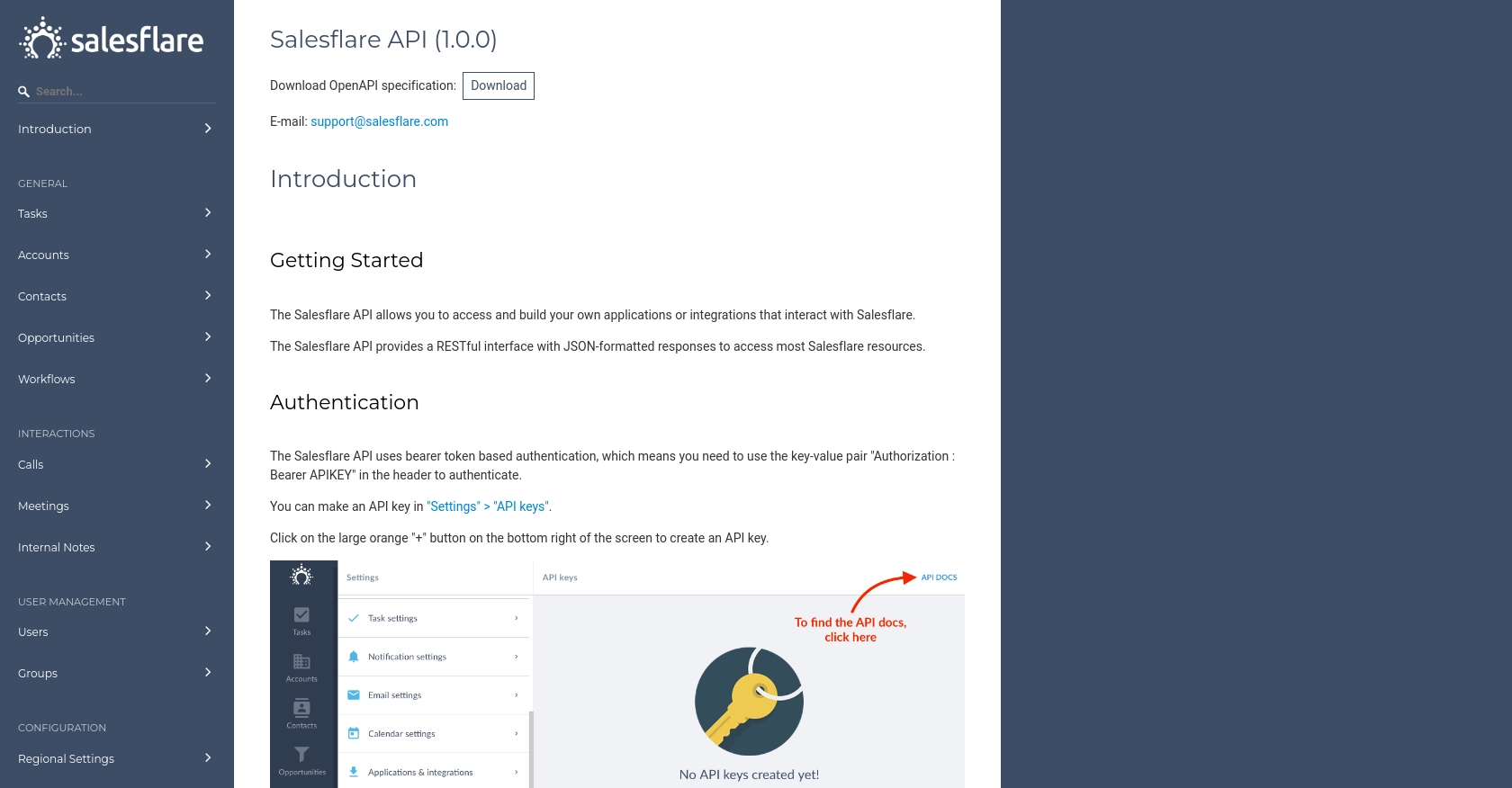
sbb-itb-96038d7
Making API Calls to Retrieve Accounts with Salesflare API in PHP
To interact with the Salesflare API and retrieve account information, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Salesflare API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Once you have PHP and Composer installed, you can proceed to install the necessary dependencies. For this tutorial, we'll use the GuzzleHTTP
library to handle HTTP requests. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Accounts from Salesflare
With your environment set up, you can now write the PHP code to make the API call. Create a new PHP file named get_salesflare_accounts.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the HTTP client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://api.salesflare.com/v1/accounts';
$headers = [
'Authorization' => 'Bearer YOUR_API_KEY',
'Accept' => 'application/json'
];
// Make the GET request to the Salesflare API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
$data = json_decode($response->getBody(), true);
foreach ($data['accounts'] as $account) {
echo 'Account Name: ' . $account['name'] . "\n";
}
} else {
echo 'Failed to retrieve accounts. Status code: ' . $response->getStatusCode();
}
Replace YOUR_API_KEY
with the API key you generated earlier. This script initializes a Guzzle HTTP client, sets the API endpoint and headers, and makes a GET request to retrieve account data from Salesflare. If successful, it will print the names of the accounts.
Running Your PHP Script and Verifying the Output
To execute your script, run the following command in your terminal:
php get_salesflare_accounts.php
If the request is successful, you should see a list of account names printed in your terminal. This confirms that your API call to Salesflare was successful.
Handling Errors and Troubleshooting Salesflare API Requests
When working with APIs, it's crucial to handle potential errors gracefully. The Salesflare API may return various HTTP status codes to indicate different outcomes:
- 200 OK: The request was successful.
- 401 Unauthorized: The API key is missing or invalid.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Ensure you check the status code of the response and handle each case appropriately in your application.
Conclusion and Best Practices for Salesflare API Integration in PHP
Integrating with the Salesflare API using PHP can significantly enhance your ability to manage account data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve account information and integrate it with other business tools, ensuring your sales team always has access to the latest data.
Best Practices for Secure and Efficient Salesflare API Usage
- Secure API Key Storage: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Salesflare API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with other systems you integrate with.
Streamlining Integrations with Endgrate
While integrating with Salesflare is a powerful way to enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Salesflare.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?