How to Get Contacts with the Teamleader API in Python
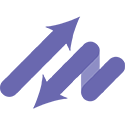
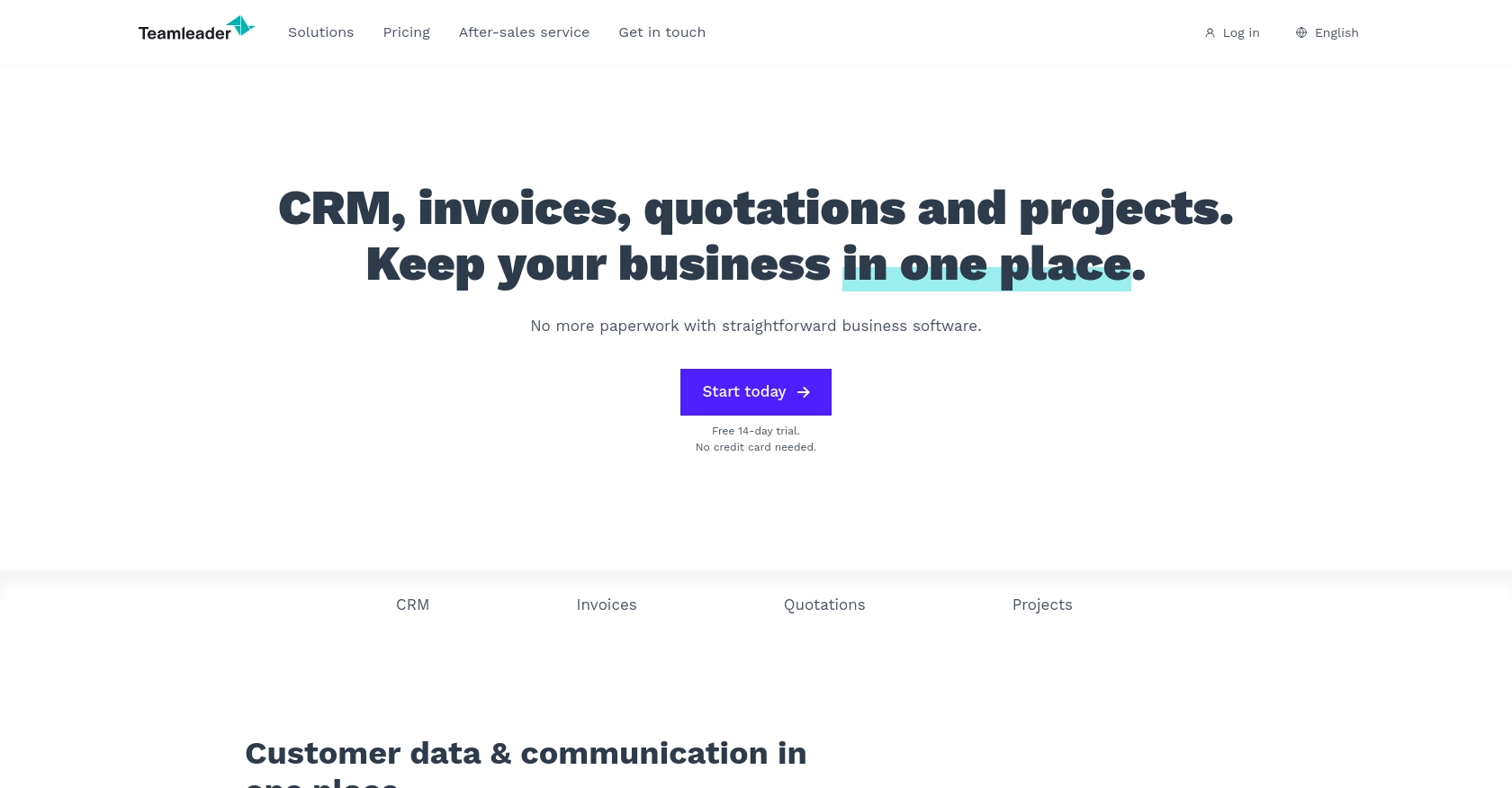
Introduction to Teamleader API
Teamleader is a versatile business management software that combines CRM, project management, and invoicing into one seamless platform. It is designed to help businesses streamline their operations, improve customer relationships, and enhance productivity.
Integrating with the Teamleader API allows developers to access and manage customer data efficiently. For example, a developer might want to retrieve contact information from Teamleader to synchronize it with another CRM system, ensuring that all customer data is up-to-date across platforms.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start interacting with the Teamleader API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. Follow the instructions to create your account. If you already have an account, simply log in.
Configuring OAuth Authentication for Teamleader API
The Teamleader API uses OAuth for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to set up OAuth:
- Navigate to the Developer section in your Teamleader account.
- Create a new app by providing the required details such as the app name and description.
- Once the app is created, you'll receive a client ID and client secret. Make sure to store these securely as they will be used to authenticate your API requests.
- Set the redirect URI to a valid endpoint where you can handle the OAuth callback.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate API requests:
- Direct users to the Teamleader authorization URL, including your client ID and redirect URI.
- Upon user approval, you'll receive an authorization code at your redirect URI.
- Exchange this authorization code for an access token by making a POST request to the Teamleader token endpoint, including your client ID, client secret, and authorization code.
import requests
# Define the token endpoint and payload
token_url = "https://app.teamleader.eu/oauth2/access_token"
payload = {
"client_id": "Your_Client_ID",
"client_secret": "Your_Client_Secret",
"code": "Authorization_Code",
"grant_type": "authorization_code",
"redirect_uri": "Your_Redirect_URI"
}
# Request the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
Replace Your_Client_ID
, Your_Client_Secret
, Authorization_Code
, and Your_Redirect_URI
with your actual values.
With the access token, you can now authenticate your API calls to Teamleader and start retrieving contact information.
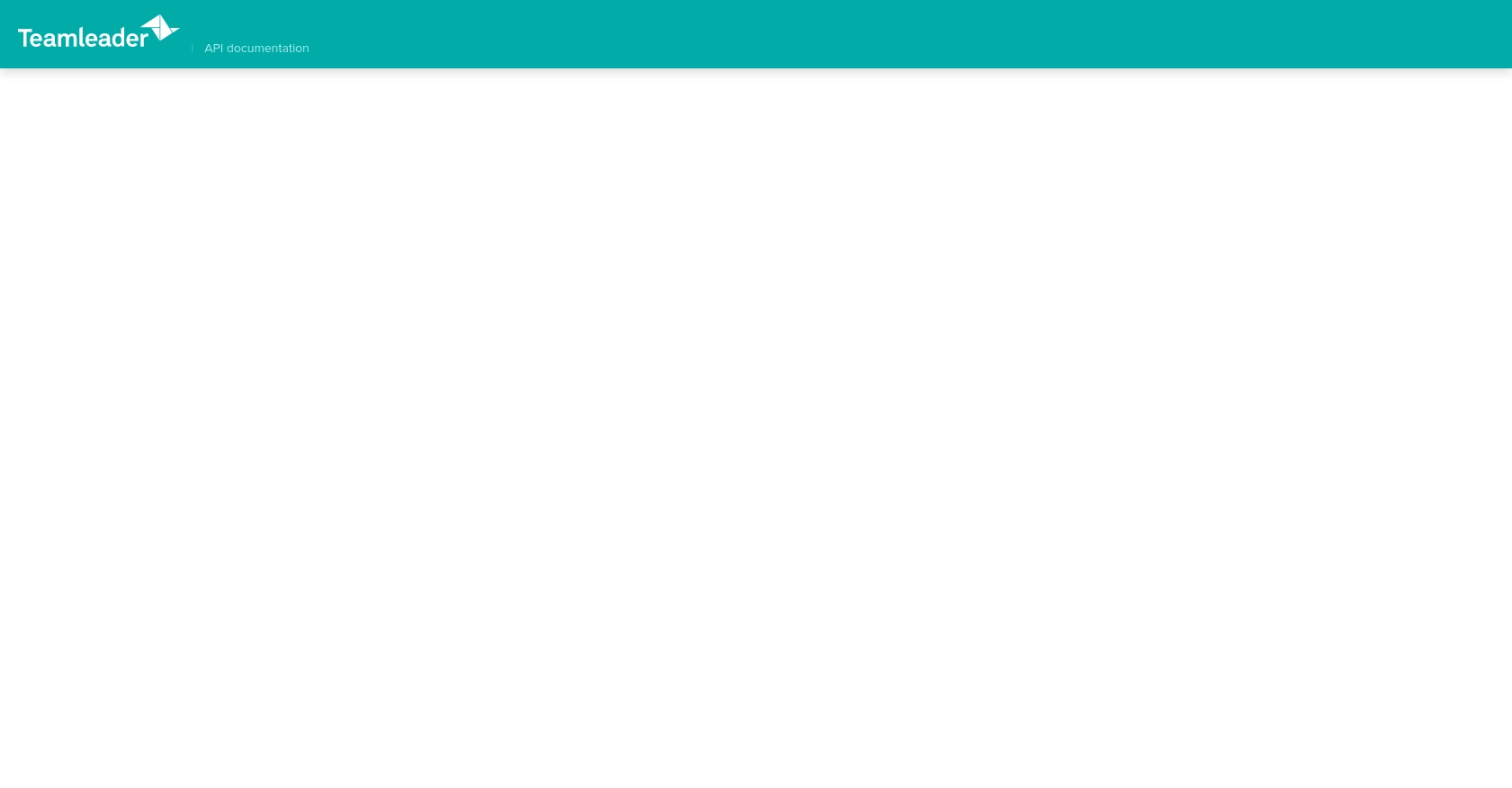
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Teamleader Using Python
To interact with the Teamleader API and retrieve contact information, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Teamleader API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing the Python Code to Fetch Contacts from Teamleader
With your environment set up, you can now write the code to fetch contacts from Teamleader. Create a new Python file named get_teamleader_contacts.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.teamleader.eu/contacts.list"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json()
for contact in contacts:
print(contact)
else:
print("Failed to retrieve contacts:", response.status_code, response.text)
Replace Your_Access_Token
with the access token you obtained earlier.
Running the Python Script and Verifying the Output
Run the script from your terminal or command line using the following command:
python get_teamleader_contacts.py
If successful, the script will print out the list of contacts retrieved from your Teamleader sandbox account. Verify the output by checking the contacts in your Teamleader account to ensure they match.
Handling Errors and Understanding Teamleader API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Teamleader API may return various HTTP status codes to indicate the result of your request:
- 200 OK: The request was successful, and the contacts were retrieved.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct.
- 403 Forbidden: You do not have permission to access the requested resource.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more information on error codes, refer to the Teamleader API documentation.
Conclusion and Best Practices for Using Teamleader API with Python
Integrating with the Teamleader API using Python allows developers to efficiently manage and synchronize contact data across platforms. By following the steps outlined in this guide, you can set up OAuth authentication, make API calls, and handle potential errors effectively.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Teamleader API to avoid throttling. Implement retry logic with exponential backoff to manage rate limits gracefully.
- Data Standardization: Ensure that the data retrieved from Teamleader is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process by providing a single endpoint to connect with various platforms, including Teamleader.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Whether you need to build once for multiple use cases or provide an intuitive integration experience for your customers, Endgrate can help streamline your integration efforts.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a unified API approach.
Read More
Ready to get started?