How to Create Leads with the Zoho CRM API in PHP
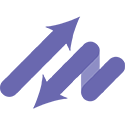
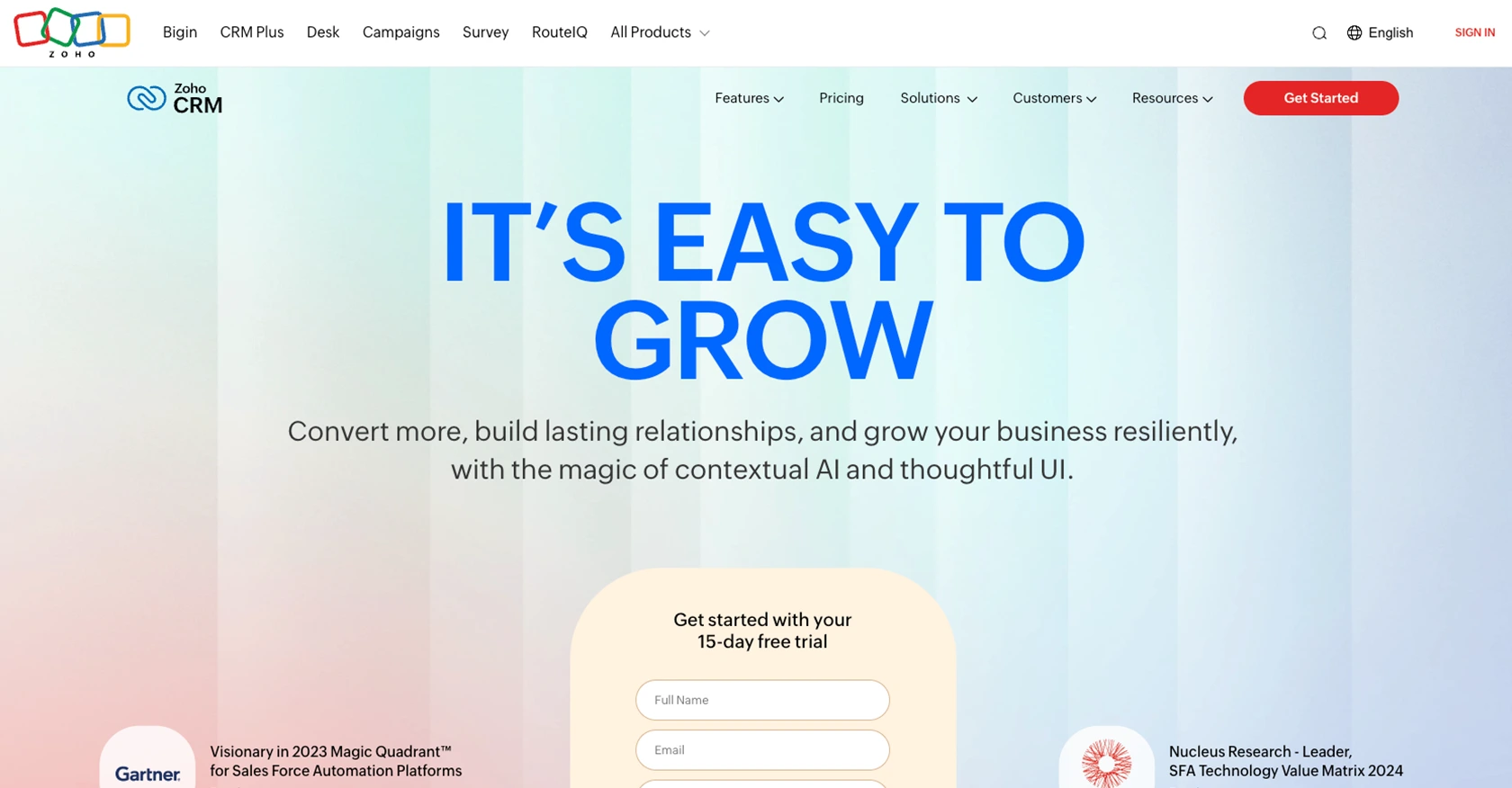
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and customer support in a unified system. Known for its flexibility and scalability, Zoho CRM is a popular choice for businesses looking to enhance their customer engagement and streamline their operations.
Integrating with Zoho CRM's API allows developers to automate and enhance various business processes. For example, creating leads programmatically can help businesses capture potential customer information from multiple sources, ensuring no opportunity is missed. This integration can be particularly useful for businesses that receive leads from diverse channels, such as websites, social media, and email campaigns.
In this article, we will explore how to create leads using the Zoho CRM API with PHP, providing developers with the tools they need to efficiently manage lead data and improve their sales processes.
Setting Up Your Zoho CRM Test/Sandbox Account
Creating a Zoho CRM Developer Account for API Integration
To begin integrating with the Zoho CRM API, you'll need to set up a developer account. This allows you to access Zoho's sandbox environment, where you can safely test API calls without affecting live data. Follow these steps to create your account:
- Visit the Zoho Developer Console.
- Sign up for a free developer account or log in if you already have one.
- Navigate to the "API Console" section to manage your applications and API keys.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for secure API authentication. To interact with the API, you must register your application and obtain the necessary credentials:
- In the Zoho Developer Console, click on "Add Client" to register a new application.
- Choose the client type that suits your application (e.g., Web-based, Mobile).
- Fill in the required details such as Client Name, Homepage URL, and Authorized Redirect URIs.
- Click "Create" to generate your Client ID and Client Secret.
- Save these credentials securely as they will be used for API authentication.
Generating Access Tokens for Zoho CRM API
Once your application is registered, you need to generate access tokens to authenticate API requests:
- Use the following URL format to request an authorization code:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoCRM.modules.ALL&client_id=YOUR_CLIENT_ID&response_type=code&access_type=offline&redirect_uri=YOUR_REDIRECT_URI
- After the user authorizes the application, Zoho will redirect to your specified URI with an authorization code.
- Exchange this code for an access token using a POST request:
curl -X POST https://accounts.zoho.com/oauth/v2/token \ -d "grant_type=authorization_code" \ -d "client_id=YOUR_CLIENT_ID" \ -d "client_secret=YOUR_CLIENT_SECRET" \ -d "redirect_uri=YOUR_REDIRECT_URI" \ -d "code=AUTHORIZATION_CODE"
- Store the access token securely for use in API requests. Remember that access tokens expire after an hour, so you'll need to refresh them periodically.
With your Zoho CRM test account and OAuth setup complete, you're ready to start making API calls to create leads and manage your CRM data programmatically.
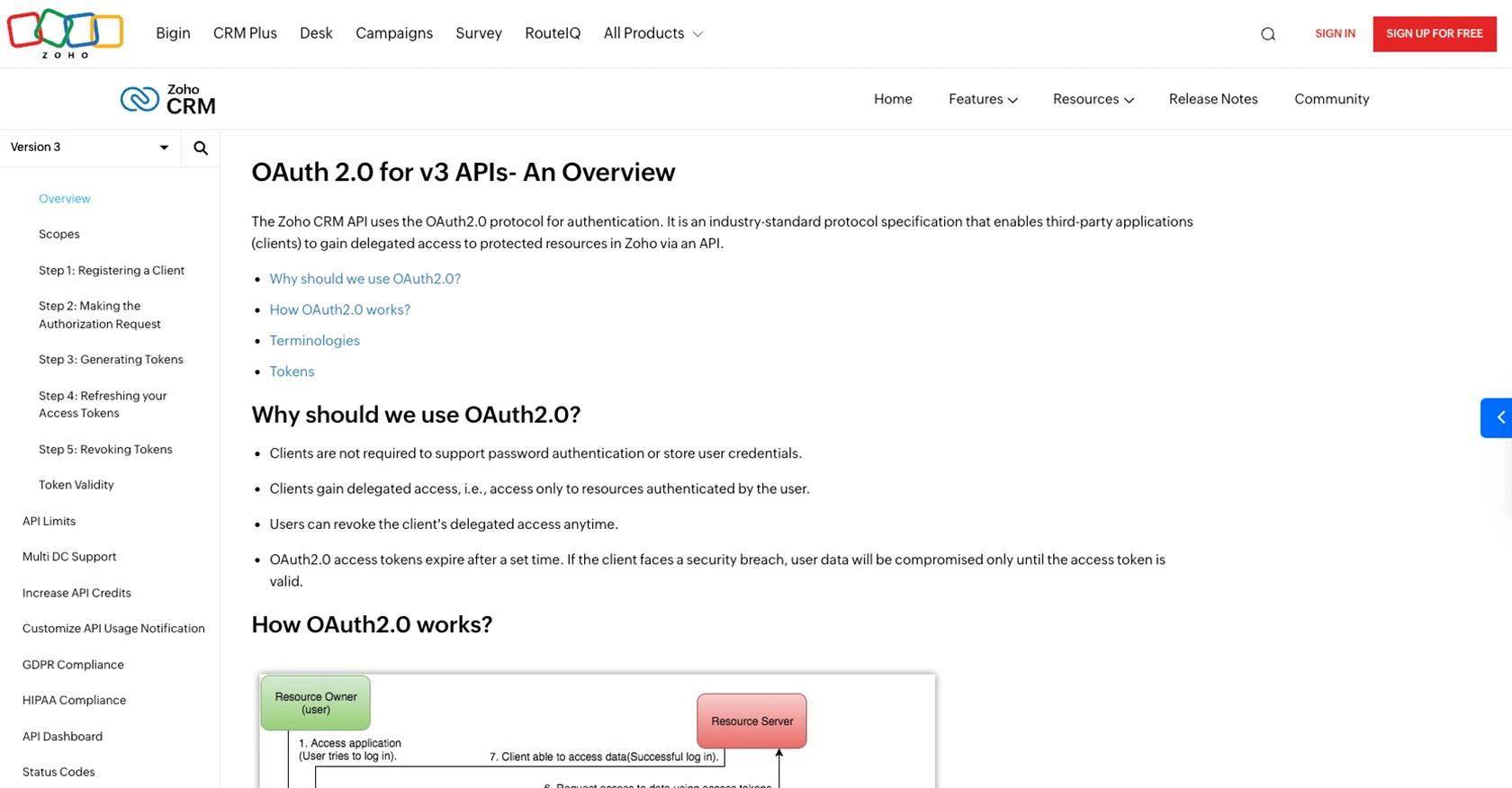
sbb-itb-96038d7
Making API Calls to Create Leads in Zoho CRM Using PHP
Setting Up Your PHP Environment for Zoho CRM API Integration
To interact with the Zoho CRM API using PHP, ensure your environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled to make HTTP requests. Follow these steps to set up your environment:
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
. - Install Composer, a dependency manager for PHP, if you haven't already.
Installing Required PHP Libraries for Zoho CRM API
To simplify API requests, use the Guzzle HTTP client, a popular PHP library. Install it via Composer:
composer require guzzlehttp/guzzle
Creating Leads in Zoho CRM with PHP
With your environment ready, you can now create leads in Zoho CRM using the API. Here's a step-by-step guide:
- Create a new PHP file,
create_lead.php
, and include the Guzzle library: - Initialize the Guzzle client and set up the API endpoint and headers:
- Prepare the lead data you want to create:
- Make the POST request to create the lead:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$endpoint = 'https://www.zohoapis.com/crm/v3/Leads';
$headers = [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
];
$leadData = [
'data' => [
[
'Company' => 'Example Corp',
'Last_Name' => 'Doe',
'First_Name' => 'John',
'Email' => 'john.doe@example.com',
'Phone' => '1234567890'
]
]
];
try {
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $leadData
]);
$responseBody = json_decode($response->getBody(), true);
echo 'Lead created successfully: ' . $responseBody['data'][0]['id'];
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Handling API Responses and Errors
After making the API call, check the response to ensure the lead was created successfully. The response will include the lead ID if successful. Handle errors by catching exceptions and displaying relevant error messages.
For more information on error codes and handling, refer to the Zoho CRM API documentation.
Verifying Lead Creation in Zoho CRM
To verify that the lead was created, log in to your Zoho CRM account and navigate to the Leads module. The new lead should appear in the list. If not, review the API response for any errors or issues.
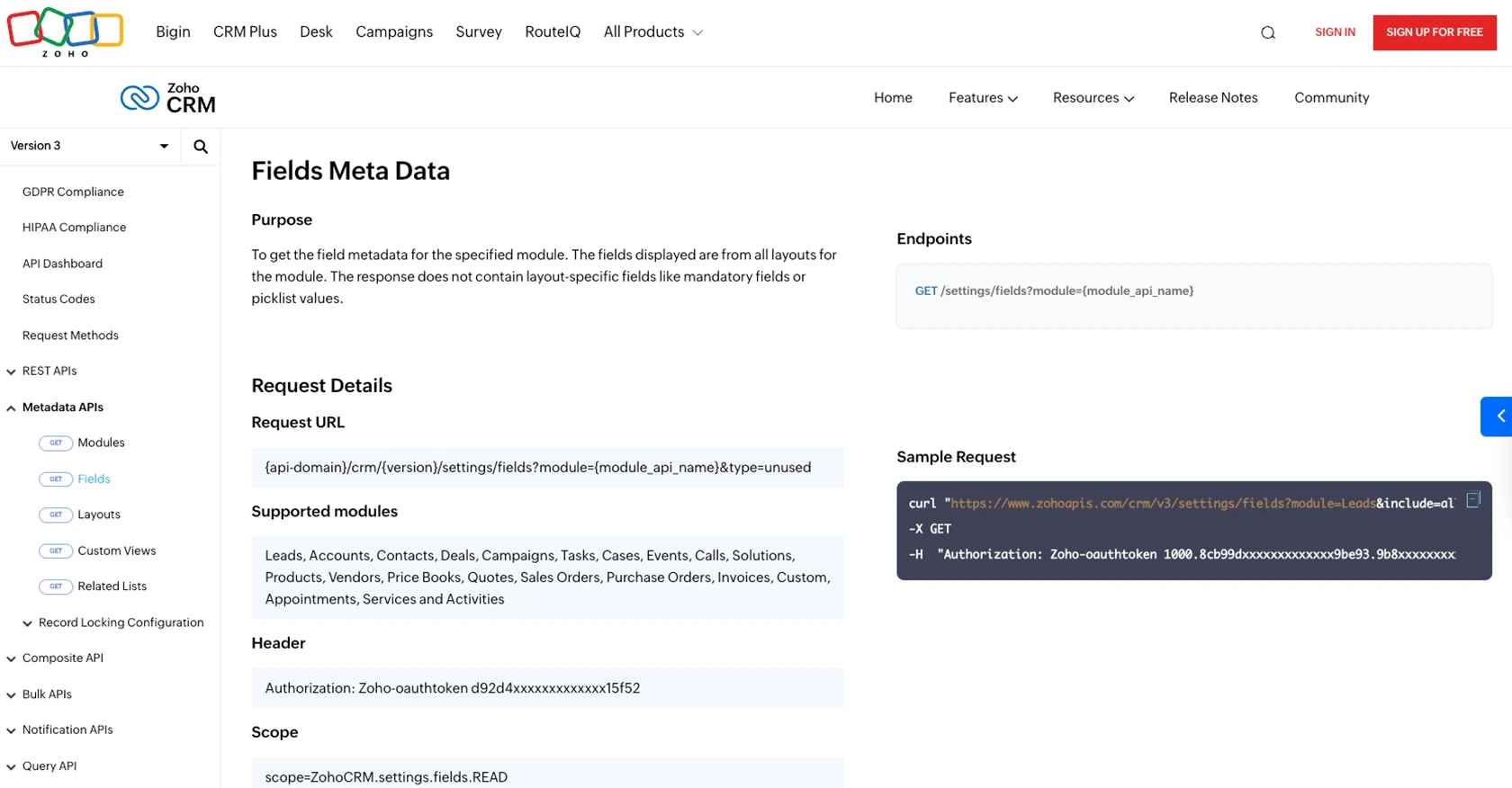
Best Practices for Zoho CRM API Integration
When integrating with the Zoho CRM API, it's essential to follow best practices to ensure security, efficiency, and scalability. Here are some recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid exposing them in public repositories or client-side code.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic for requests that exceed the limit. For more details, refer to the API limits documentation.
- Refresh Tokens Regularly: Access tokens expire after an hour. Implement a mechanism to refresh tokens using the refresh token to maintain uninterrupted API access.
- Validate API Responses: Always check API responses for success or error codes. Implement error handling to manage exceptions gracefully.
- Optimize Data Handling: Standardize and transform data fields to match your CRM schema, ensuring consistency across different data sources.
Enhance Your Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?