Using the Hubspot API to Create or Update Companies (with PHP examples)
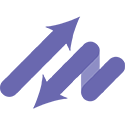
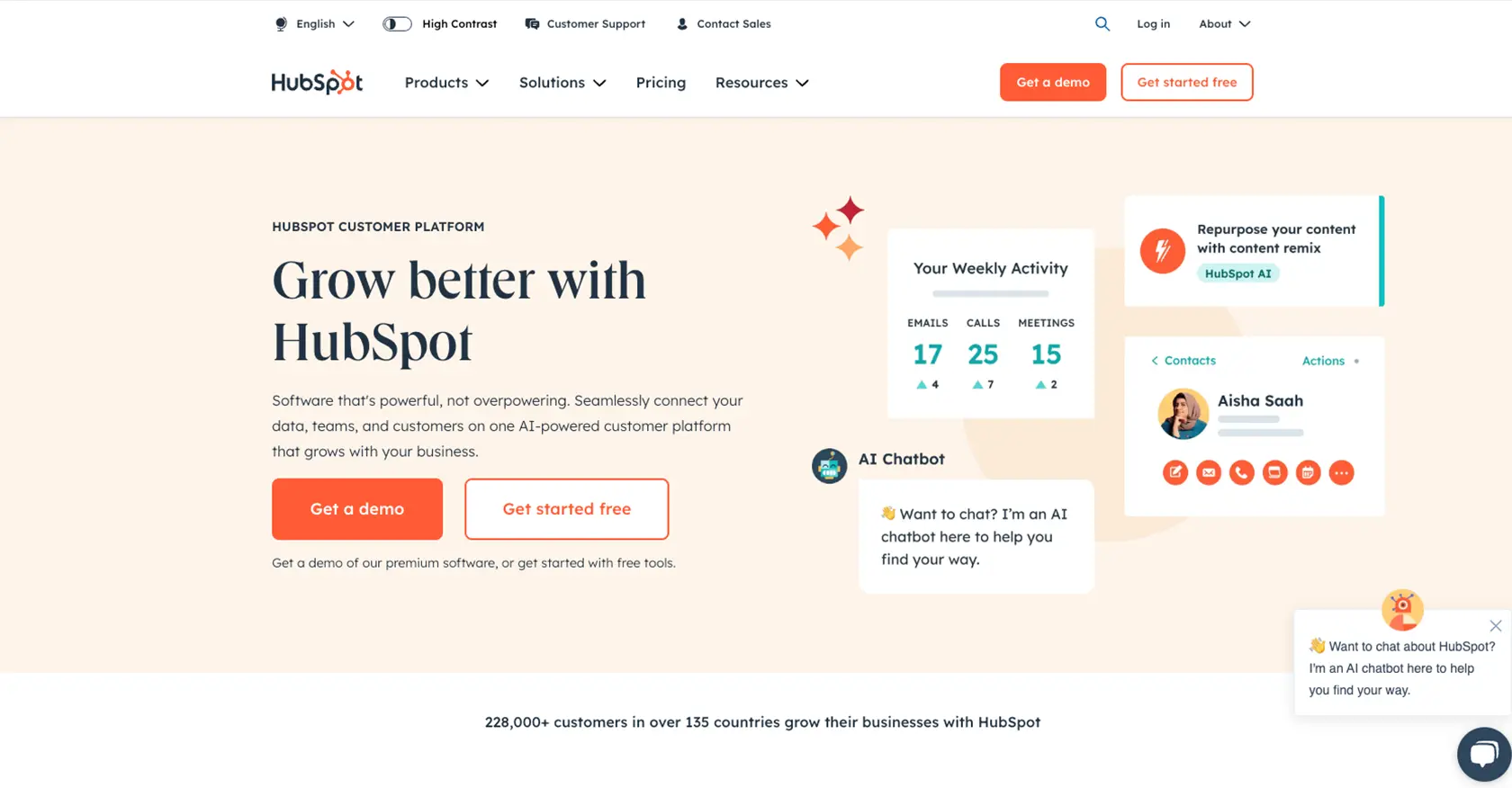
Introduction to HubSpot API for Company Management
HubSpot is a powerful CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. With its robust API, developers can integrate HubSpot's functionalities into their own applications, enhancing the ability to manage customer relationships effectively.
Connecting with the HubSpot API allows developers to automate and streamline various CRM processes. For example, using the API to create or update company records can help businesses maintain accurate and up-to-date information about their clients and partners. This is particularly useful for B2B SaaS products that need to manage large volumes of company data efficiently.
In this article, we will explore how to use PHP to interact with the HubSpot API for creating or updating company records. This integration can be a game-changer for developers looking to enhance their applications with seamless CRM capabilities.
Setting Up Your HubSpot Developer Account for API Integration
Before you can start creating or updating companies using the HubSpot API, you'll need to set up a developer account and configure the necessary authentication. HubSpot provides a sandbox environment that allows developers to test their integrations without affecting live data.
Step-by-Step Guide to Creating a HubSpot Developer Account
-
Sign Up for a Developer Account:
Visit the HubSpot Developer Portal and create a developer account. This account will allow you to create and manage your apps, monitor their performance, and access HubSpot's API documentation.
-
Create a Test Account:
Once your developer account is set up, create a test account. This sandbox environment will enable you to test API calls without impacting your actual HubSpot data.
Configuring OAuth Authentication for HubSpot API
HubSpot uses OAuth for authentication, which provides a secure way to authorize API requests. Follow these steps to set up OAuth for your app:
-
Create a Private App:
In your HubSpot account, navigate to the Settings section, then go to Integrations and select Private Apps. Click on Create a private app and fill in the necessary details.
-
Set Scopes and Permissions:
Under the Scopes tab, search for and select the necessary scopes for managing companies, such as
crm.objects.companies.write
andcrm.objects.companies.read
. This will grant your app the required permissions to create and update company records. -
Generate an Access Token:
After setting the scopes, click Create app. You will receive an access token, which you should securely store as it will be used to authenticate your API requests.
For more detailed information on authentication, refer to the HubSpot Authentication Documentation.
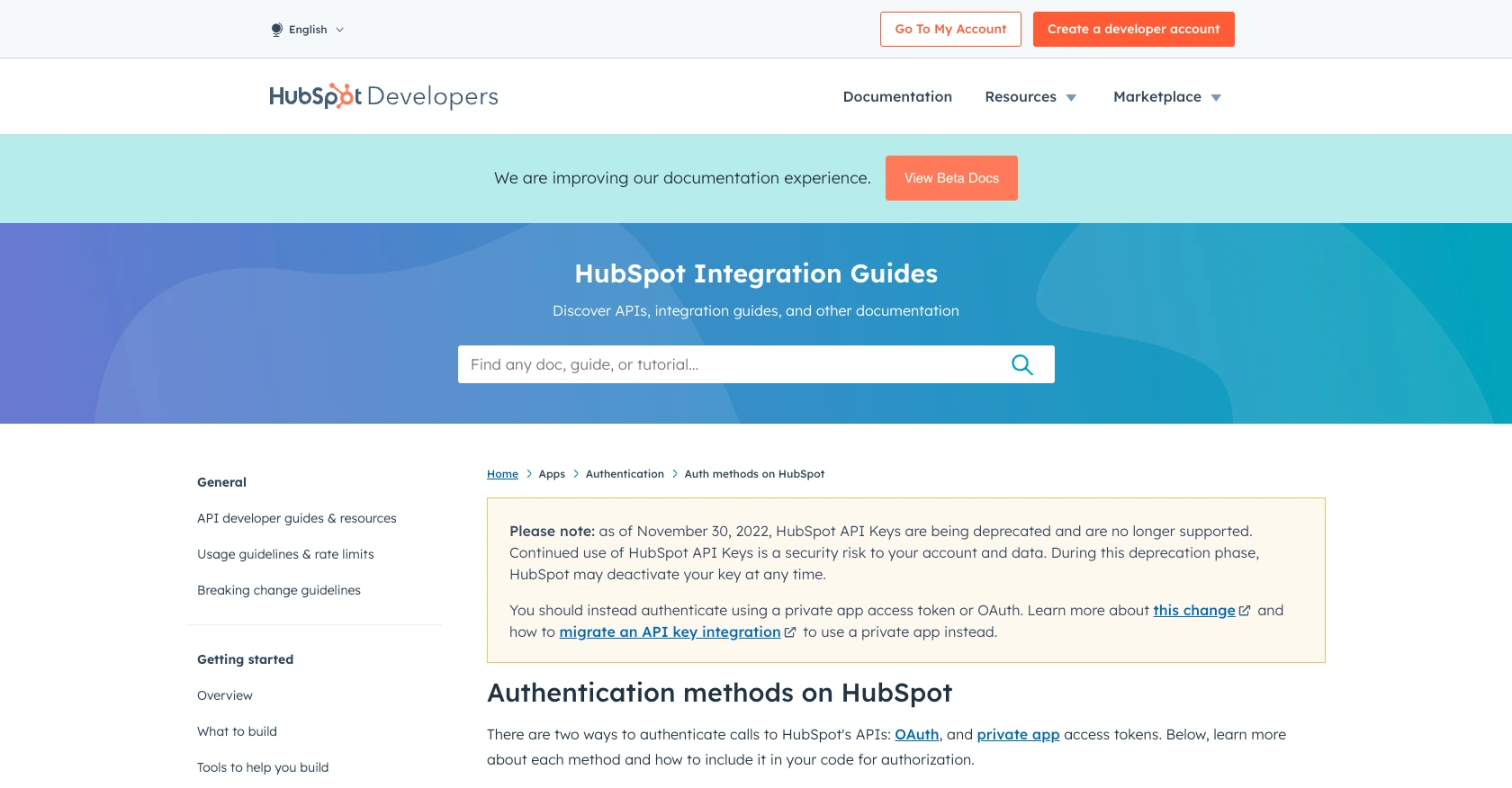
sbb-itb-96038d7
Making API Calls to HubSpot for Creating or Updating Companies Using PHP
To interact with the HubSpot API for creating or updating company records, you need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
- The
hubspot/api-client
package
Install the HubSpot API client using Composer:
composer require hubspot/api-client
Creating a New Company in HubSpot Using PHP
To create a new company in HubSpot, you'll need to make a POST request to the HubSpot API endpoint. Here's a step-by-step guide:
require 'vendor/autoload.php';
use HubSpot\Factory;
$accessToken = 'your_access_token';
$client = Factory::createWithAccessToken($accessToken);
$properties = [
'name' => 'Example Company',
'domain' => 'example.com',
'city' => 'Boston',
'industry' => 'Technology'
];
$response = $client->crm()->companies()->basicApi()->create(['properties' => $properties]);
if ($response->getStatusCode() === 201) {
echo "Company created successfully!";
} else {
echo "Failed to create company.";
}
Replace your_access_token
with the access token generated from your HubSpot app. This code initializes the HubSpot client, sets the company properties, and sends a POST request to create the company.
Updating an Existing Company in HubSpot Using PHP
To update an existing company, use a PATCH request with the company's ID. Here's how:
$companyId = '123456';
$updateProperties = [
'city' => 'Cambridge',
'phone' => '555-555-5555'
];
$response = $client->crm()->companies()->basicApi()->update($companyId, ['properties' => $updateProperties]);
if ($response->getStatusCode() === 200) {
echo "Company updated successfully!";
} else {
echo "Failed to update company.";
}
Replace 123456
with the actual company ID you wish to update. This code updates the specified properties of the company.
Handling API Responses and Errors
It's crucial to handle API responses and errors effectively. Check the status code of the response to determine if the request was successful. Common status codes include:
- 200: Success
- 201: Created
- 400: Bad Request
- 401: Unauthorized
- 429: Rate Limit Exceeded
For detailed error handling, refer to the HubSpot API documentation.
Verifying API Requests in HubSpot
After making API calls, verify the changes in your HubSpot test account. Check the Companies section to ensure the new or updated company records appear as expected.
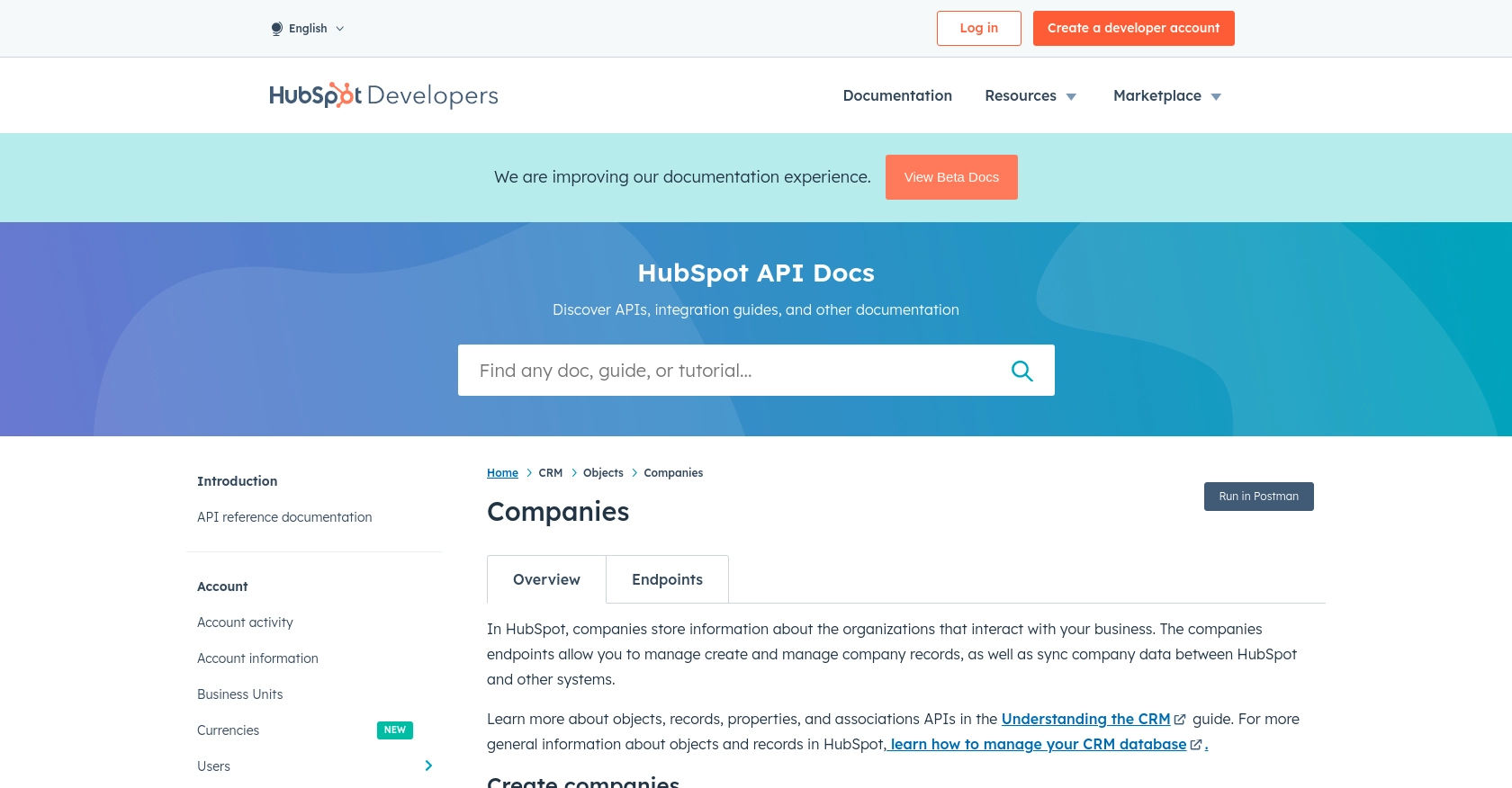
Conclusion and Best Practices for Using HubSpot API with PHP
Integrating with the HubSpot API using PHP offers developers a powerful way to manage company data efficiently. By following the steps outlined in this article, you can create and update company records seamlessly, enhancing your application's CRM capabilities.
Best Practices for Secure and Efficient HubSpot API Integration
- Secure Storage of Access Tokens: Ensure that your OAuth access tokens are stored securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which are 100 requests every 10 seconds for OAuth apps. Implement request throttling to avoid hitting these limits. For more details, refer to the HubSpot API usage guidelines.
- Data Standardization: Standardize and validate data fields before sending them to the API to ensure consistency and prevent errors.
- Error Handling: Implement robust error handling to manage API response codes effectively, ensuring that your application can gracefully handle failures and retries.
Enhance Your Integration Strategy with Endgrate
While integrating with HubSpot's API can significantly enhance your application's functionality, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including HubSpot. By leveraging Endgrate, you can streamline your integration efforts, focus on your core product, and deliver a seamless experience to your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/companies
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?