Using the Stripe API to Get Customers in Python
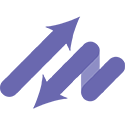
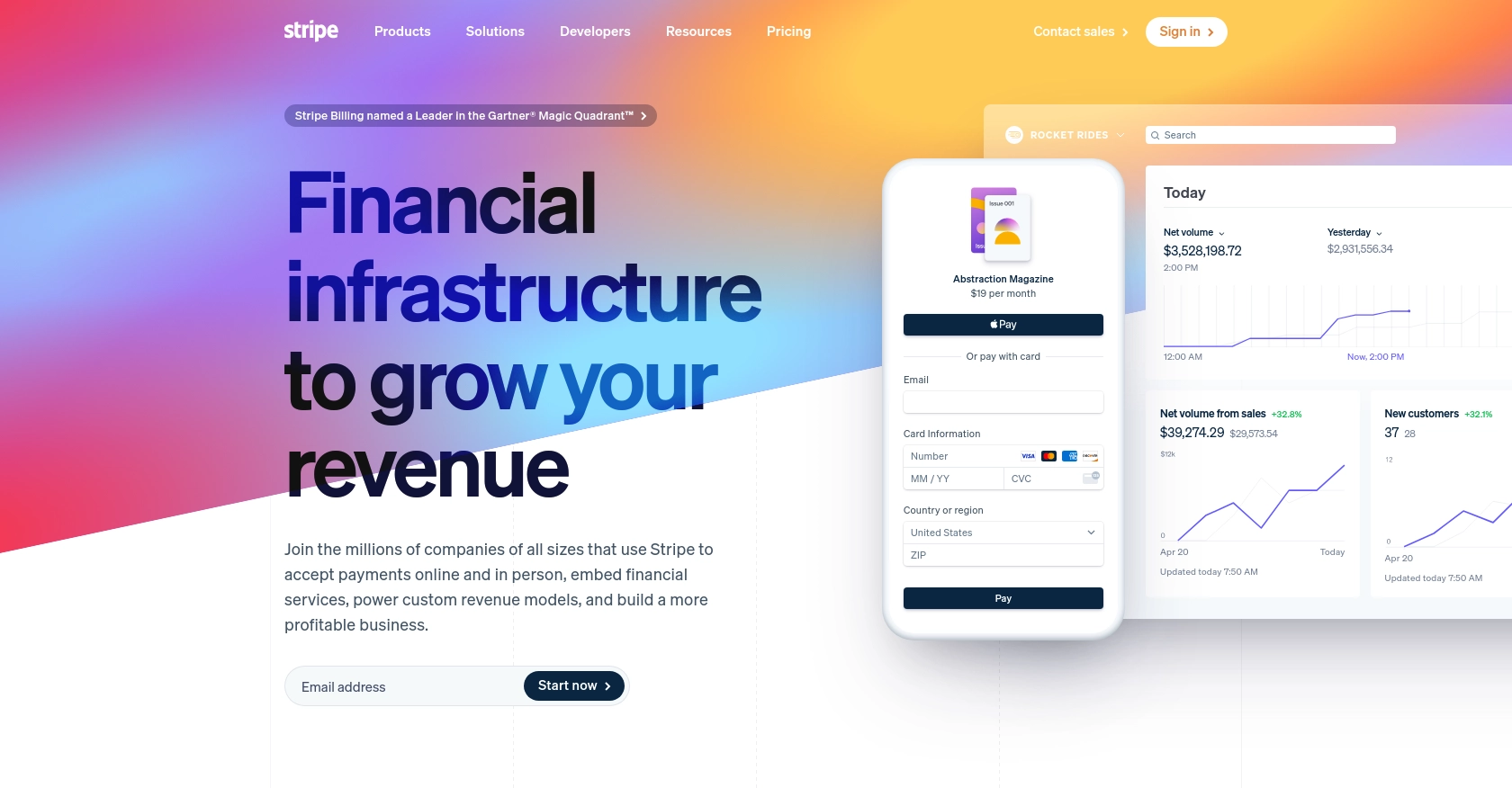
Introduction to Stripe API for Customer Management
Stripe is a powerful payment processing platform that enables businesses to manage online transactions with ease. Known for its flexibility and robust features, Stripe offers a comprehensive suite of tools for handling payments, subscriptions, and financial operations.
Developers often integrate with Stripe's API to streamline customer management processes. For example, using the Stripe API, you can efficiently retrieve customer data to analyze purchasing patterns or automate billing processes. This integration is particularly beneficial for SaaS businesses looking to enhance their financial operations and customer interactions.
Setting Up Your Stripe Test Account for API Integration
Before you can start using the Stripe API to manage customers, you'll need to set up a test account. Stripe provides a sandbox environment that allows developers to test their integrations without affecting live data. This is an essential step for ensuring that your API calls work as expected before deploying them in a production environment.
Creating a Stripe Account
If you don't already have a Stripe account, you can easily sign up for one. Visit the Stripe website and follow the instructions to create a free account. Once your account is set up, you'll have access to the Stripe Dashboard, where you can manage your API keys and other settings.
Accessing the Stripe Dashboard
Log in to your Stripe account and navigate to the Dashboard. Here, you'll find a variety of tools and resources to help you manage your account and integrations. The Dashboard is where you'll generate your API keys, which are necessary for authenticating your API requests.
Generating API Keys for Authentication
Stripe uses API keys to authenticate requests. You can find and manage your API keys in the Stripe Dashboard under the "Developers" section. Follow these steps to generate your API keys:
- Navigate to the "API keys" section in the Dashboard.
- Locate the "Secret Key" labeled with the prefix
sk_test_
for test mode. - Click "Reveal test key" to view your secret key.
- Copy the key and store it securely, as you'll need it to authenticate your API requests.
Remember, your API keys carry many privileges, so keep them secure and do not share them publicly.
Testing in Sandbox Mode
Stripe's test mode allows you to simulate transactions and other API interactions without affecting your live data. This is crucial for testing your integration thoroughly. Use the test API key you generated to make API calls in this mode.
Creating Test Customers in Stripe
To test retrieving customer data, you'll need to create some test customers in your Stripe account. Here's how you can do it:
- In the Dashboard, go to the "Customers" section.
- Click "Add customer" and fill in the necessary details.
- Create several test customers to use in your API calls.
These test customers will be used to verify that your API integration is working correctly.
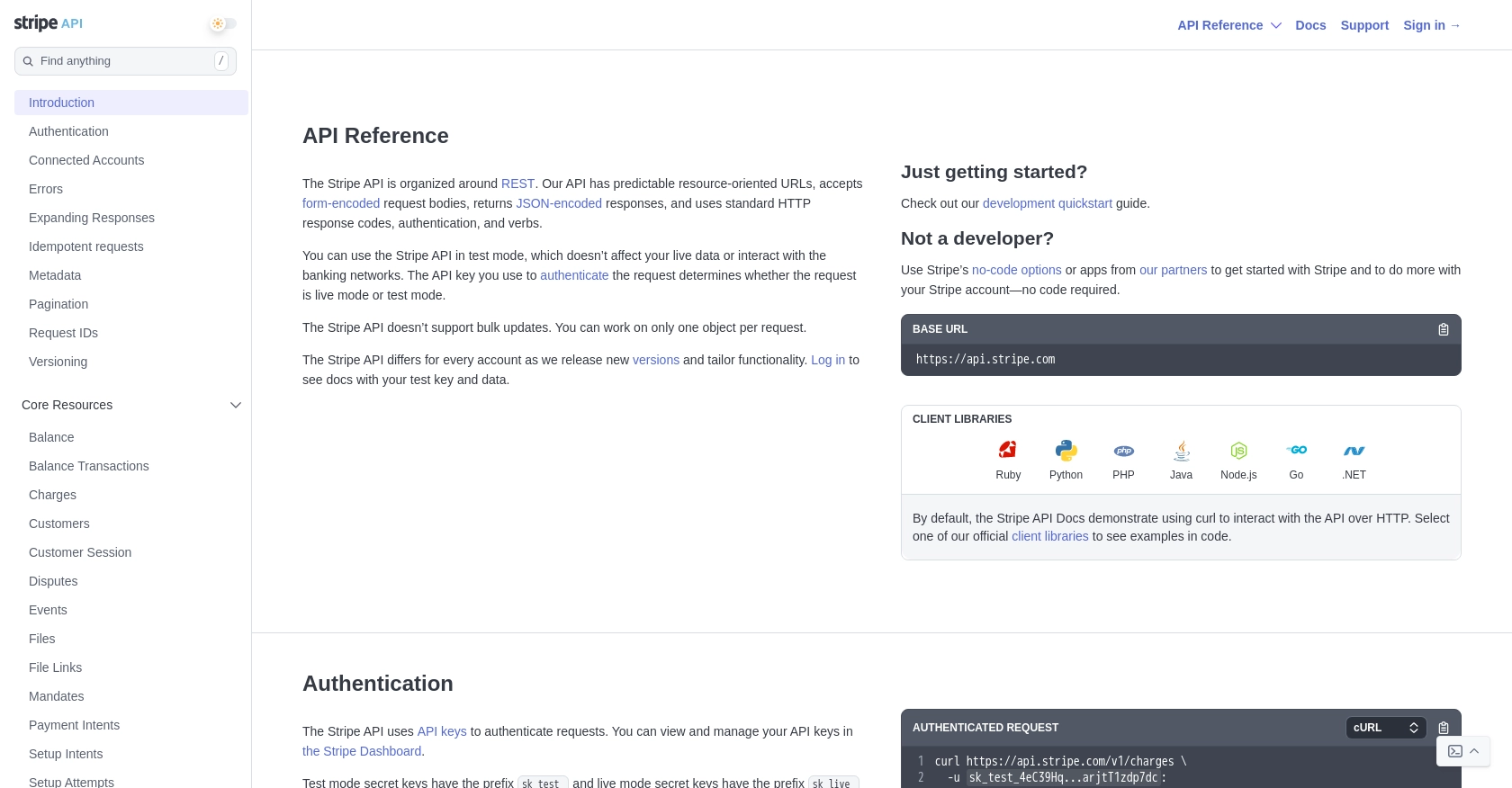
sbb-itb-96038d7
Making API Calls to Retrieve Customers Using Stripe API in Python
To interact with the Stripe API and retrieve customer data, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your environment, writing the necessary code, and executing API calls to fetch customer information from Stripe.
Setting Up Your Python Environment for Stripe API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Fetch Customers from Stripe
Create a new Python file named get_stripe_customers.py
and add the following code:
import requests
# Set the API endpoint and headers
url = "https://api.stripe.com/v1/customers"
headers = {
"Authorization": "Bearer sk_test_your_secret_key"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Parse the JSON data from the response
data = response.json()
# Loop through the customers and print their information
for customer in data["data"]:
print(customer)
Replace sk_test_your_secret_key
with your actual test secret key from the Stripe Dashboard.
Executing the Python Script to Retrieve Stripe Customers
Run the script from your terminal or command line:
python get_stripe_customers.py
You should see a list of customer objects printed to the console, representing the test customers you created in your Stripe account.
Verifying Successful API Requests in Stripe Dashboard
After running the script, verify that the API call was successful by checking the "Customers" section in your Stripe Dashboard. The customers retrieved by your script should match those listed in the dashboard.
Handling Errors and Understanding Stripe API Response Codes
Stripe uses standard HTTP response codes to indicate the success or failure of an API request. Here are some common codes:
- 200 OK: The request was successful.
- 400 Bad Request: The request was unacceptable, often due to missing parameters.
- 401 Unauthorized: No valid API key provided.
- 429 Too Many Requests: Too many requests hit the API too quickly. Implement exponential backoff for retries.
For more detailed error handling, refer to the Stripe API documentation.
Conclusion and Best Practices for Using Stripe API in Python
Integrating with the Stripe API to manage customer data in Python offers a streamlined approach to handling financial operations for your SaaS business. By following the steps outlined in this guide, you can efficiently retrieve customer information and enhance your billing processes.
Best Practices for Secure and Efficient Stripe API Integration
- Secure Your API Keys: Always keep your API keys confidential and avoid sharing them in public repositories or client-side code.
- Handle Rate Limiting: Stripe imposes rate limits on API requests. Implement exponential backoff strategies to handle
429 Too Many Requests
errors gracefully. - Store User Credentials Safely: Use secure methods to store user credentials and sensitive data, ensuring compliance with data protection regulations.
- Standardize Data Fields: Transform and standardize data fields to maintain consistency across your application and Stripe's API.
Enhancing Your Integration Experience with Endgrate
For developers looking to simplify their integration processes, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration needs by visiting Endgrate.
Read More
Ready to get started?