Using the Shopify API to Get Products (with Python examples)
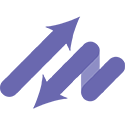
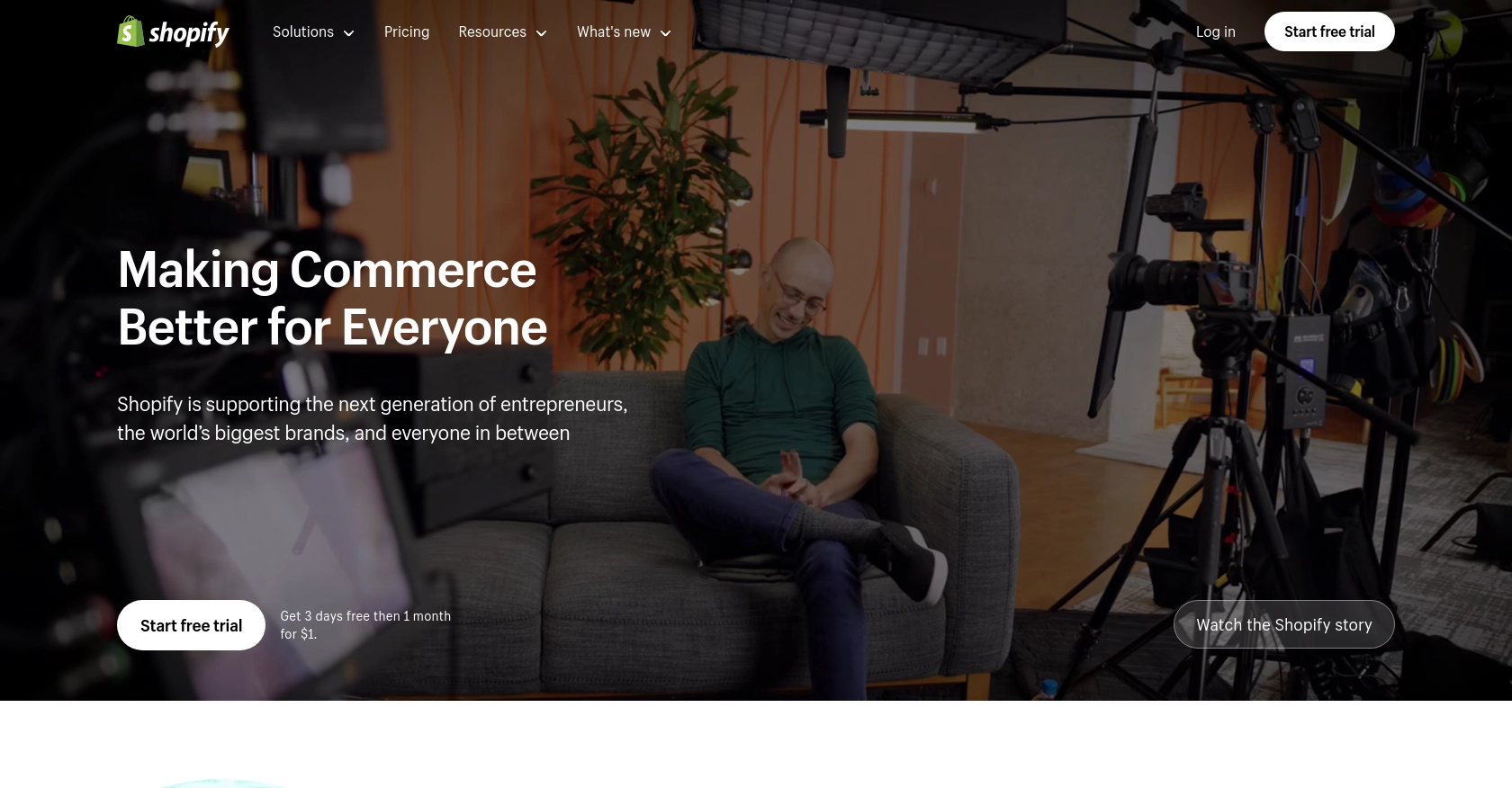
Introduction to Shopify API for Product Management
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust suite of tools for managing products, processing payments, and handling shipping logistics, making it a popular choice for businesses of all sizes.
Integrating with Shopify's API allows developers to automate and streamline various e-commerce operations. For example, using the Shopify API, developers can retrieve product information to display on external platforms or synchronize inventory across multiple sales channels. This capability is essential for businesses looking to maintain accurate product data and enhance their online presence.
In this article, we will explore how to use Python to interact with the Shopify API to retrieve product information. This integration can be particularly useful for developers aiming to build custom solutions that require access to Shopify's extensive product catalog.
Setting Up Your Shopify Test Account for API Integration
Before diving into the Shopify API, you'll need to set up a test account to safely experiment with API calls without affecting live data. Shopify provides a development store option that allows developers to test integrations and applications in a sandbox environment.
Creating a Shopify Development Store
To get started, you'll need to create a Shopify Partner account if you don't already have one. Follow these steps to set up your development store:
- Visit the Shopify Partners page and sign up for a free account.
- Once logged in, navigate to the Stores section in your Partner Dashboard.
- Click on Add store and select Development store as the store type.
- Fill in the necessary details, such as store name and password, and click Create store.
Your development store is now ready, and you can use it to test API interactions without any risk to actual business operations.
Setting Up OAuth Authentication for Shopify API
Shopify uses OAuth 2.0 for authentication, which requires creating a custom app to obtain the necessary credentials. Follow these steps to set up OAuth authentication:
- In your Shopify Partner Dashboard, navigate to Apps and click on Create app.
- Select Custom app and provide the required information, such as app name and developer email.
- Under API credentials, you'll find your API key and API secret key. Keep these safe as you'll need them for authentication.
- Set the necessary API scopes to define what data your app can access. For product retrieval, ensure you have the
read_products
scope enabled. - Click Save to finalize your app setup.
With your app created, you can now use the API key and secret to authenticate API requests and interact with your Shopify development store.
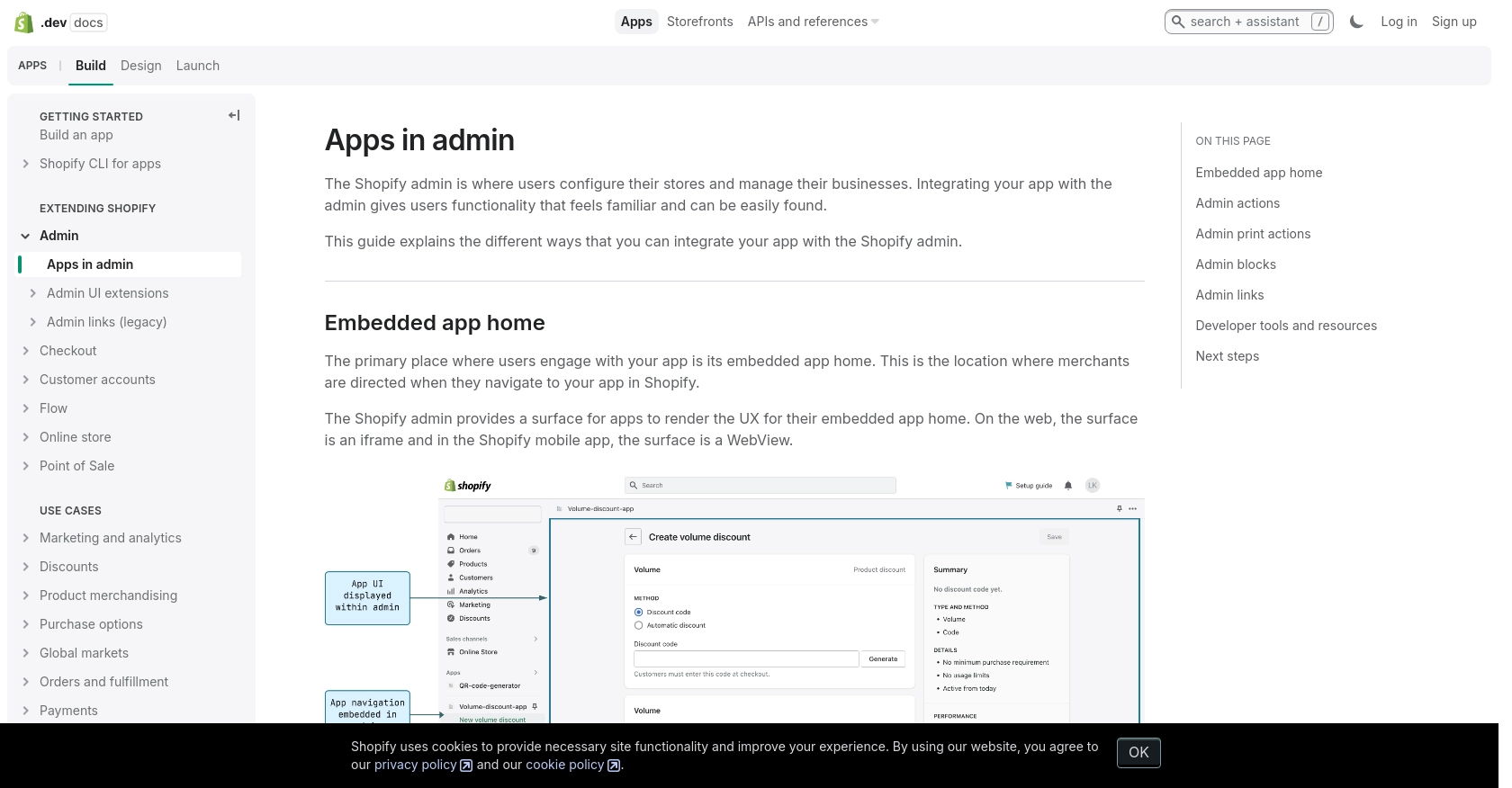
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Products Using Python
To interact with the Shopify API and retrieve product information, you'll need to use Python along with the requests
library. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Shopify API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python3 --version
in your terminal. - Install the
requests
library using pip:
pip3 install requests
Writing Python Code to Retrieve Products from Shopify
With your environment set up, you can now write the Python script to retrieve products from your Shopify store. Create a file named get_shopify_products.py
and add the following code:
import requests
# Set the API endpoint and headers
store_name = "your-development-store"
endpoint = f"https://{store_name}.myshopify.com/admin/api/2024-07/products.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
products = response.json()["products"]
for product in products:
print(f"Product ID: {product['id']}, Title: {product['title']}")
else:
print(f"Failed to retrieve products: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained from your Shopify app setup.
Running the Python Script to Fetch Shopify Products
Execute the script from your terminal using the following command:
python3 get_shopify_products.py
If successful, the script will print the IDs and titles of the products in your Shopify store. If there's an error, it will display the status code and error message.
Handling Errors and Verifying API Call Success
It's crucial to handle errors gracefully when making API calls. The Shopify API returns various status codes to indicate the result of your request:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid API key or access token.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more detailed error handling, refer to the Shopify API authentication documentation and the Shopify API product documentation.
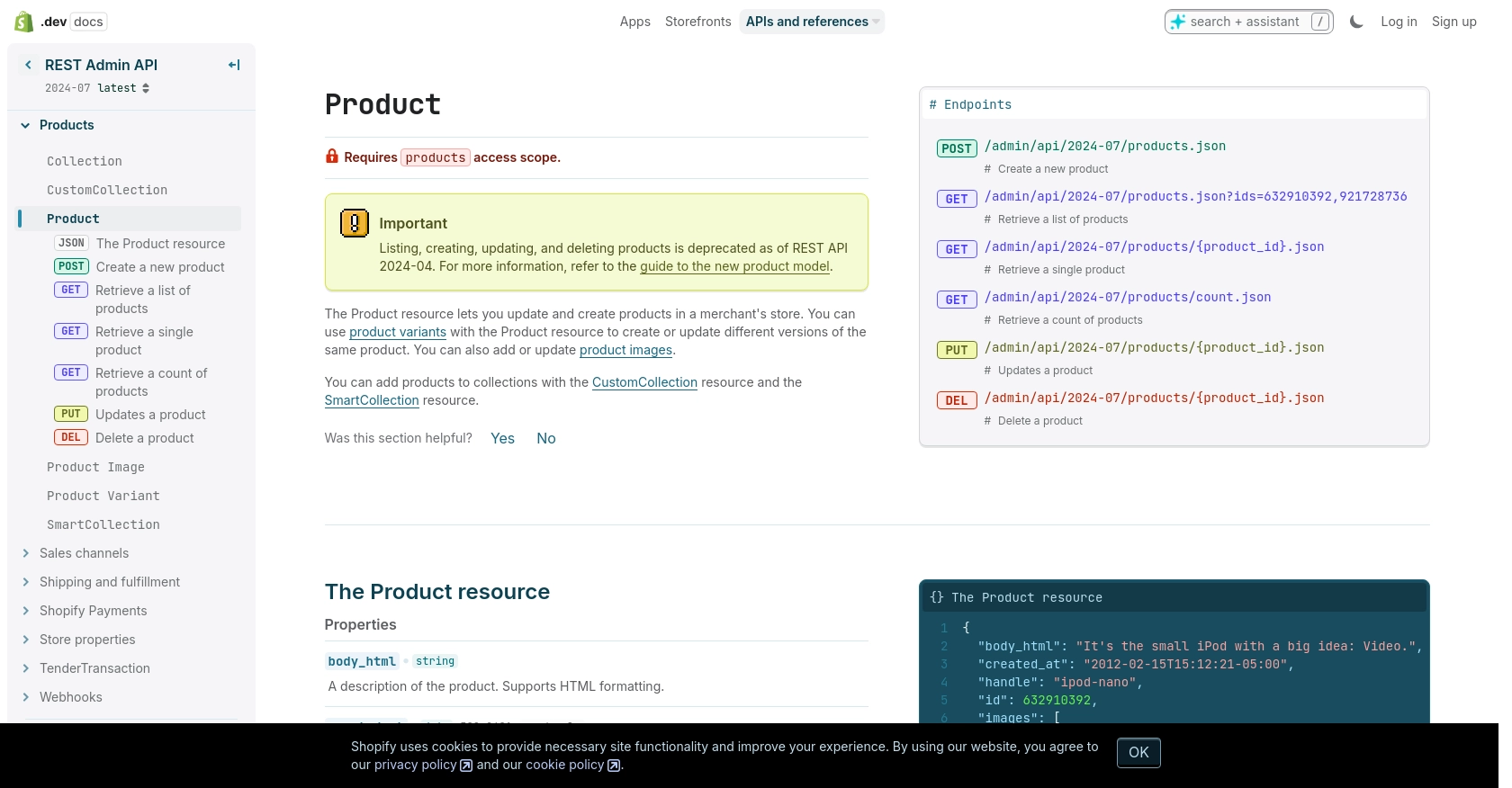
Conclusion and Best Practices for Using Shopify API with Python
Integrating with the Shopify API using Python provides a powerful way to manage and automate e-commerce operations. By retrieving product information programmatically, developers can enhance their applications and streamline workflows, ensuring that product data remains consistent across various platforms.
Best Practices for Secure and Efficient Shopify API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to keep your API key and secret safe.
- Handling Rate Limits: Shopify's API has a rate limit of 40 requests per app per store per minute. Monitor your API usage and implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Shopify API documentation. - Data Transformation: When retrieving product data, consider transforming and standardizing fields to match your application's requirements. This ensures consistency and simplifies data handling.
Streamline Your Integrations with Endgrate
While integrating with Shopify's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Shopify. By using Endgrate, you can focus on your core product development while outsourcing the intricacies of integration management.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration experience.
Read More
Ready to get started?