How to Create Tasks with the Sugar Market API in Javascript
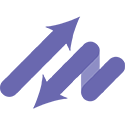
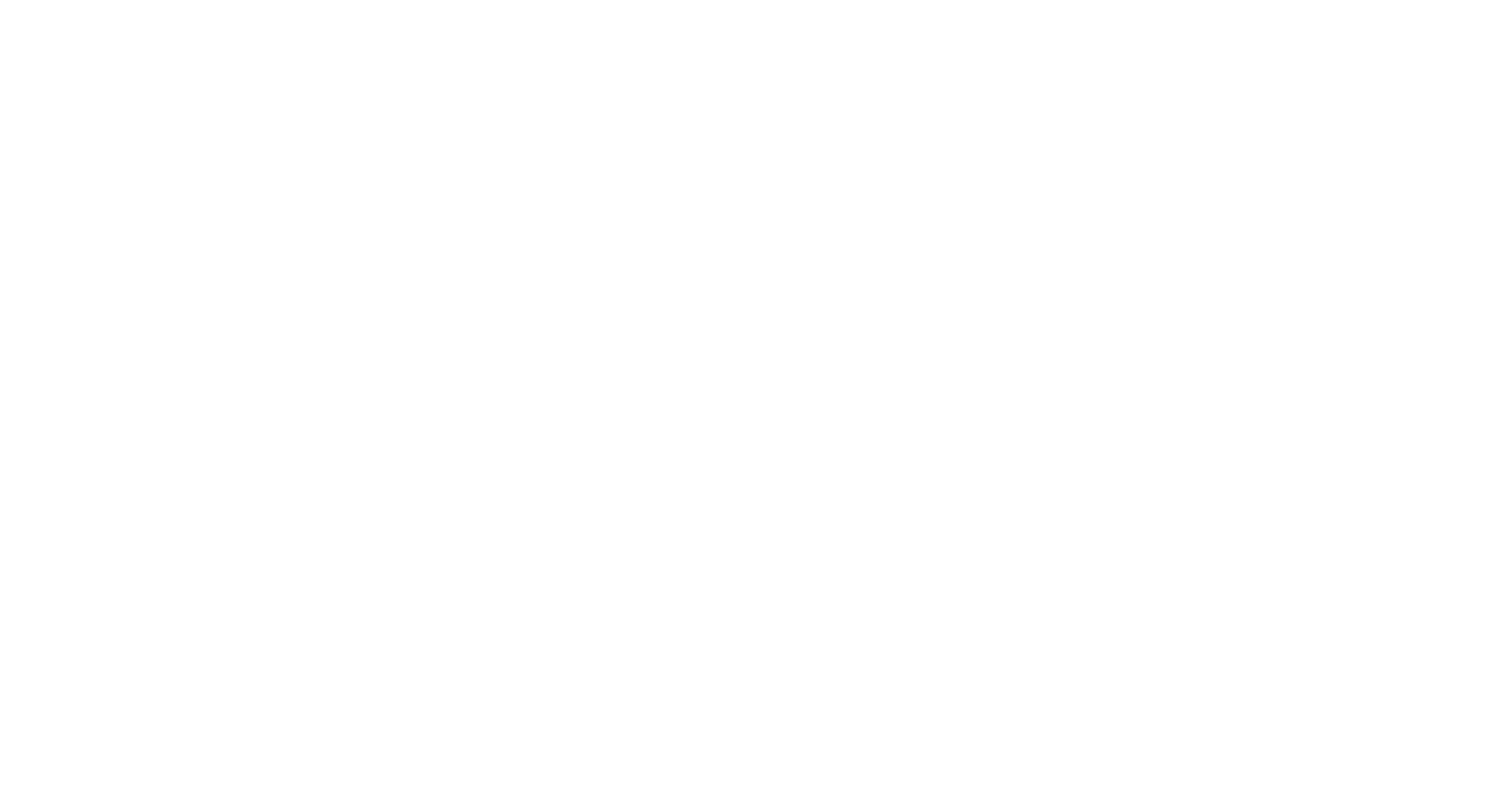
Introduction to Sugar Market API Integration
Sugar Market, part of the SugarCRM suite, is a powerful marketing automation platform designed to enhance marketing efforts through robust features like email marketing, lead nurturing, and campaign management. It offers businesses the tools they need to effectively manage and optimize their marketing strategies.
Integrating with the Sugar Market API allows developers to automate and streamline various marketing tasks. For example, developers can create tasks programmatically to manage marketing campaigns more efficiently. This can be particularly useful for automating task creation based on specific triggers or events, ensuring that marketing teams stay organized and responsive.
Setting Up Your Sugar Market Test Account
Before you can start creating tasks with the Sugar Market API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting live data.
Creating a Sugar Market Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment through the Sugar Market website. Follow the instructions provided to complete the registration process.
Generating API Credentials for Sugar Market
Once your account is set up, you'll need to generate the necessary API credentials to authenticate your requests. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the developer or integrations tab.
- Create a new API application, providing the required details such as application name and description.
- Once the application is created, you'll receive a client ID and client secret. Make sure to store these securely as you'll need them to authenticate your API requests.
Configuring OAuth for Sugar Market API
To interact with the Sugar Market API, you'll need to configure OAuth authentication. Here's how to set it up:
- Use the client ID and client secret obtained earlier to request an access token from the Sugar Market authorization server.
- Send a POST request to the token endpoint with the necessary parameters, including client ID, client secret, and grant type.
- Upon successful authentication, you'll receive an access token. This token must be included in the header of your API requests to authenticate them.
With your test account and API credentials ready, you're all set to start creating tasks using the Sugar Market API in JavaScript.
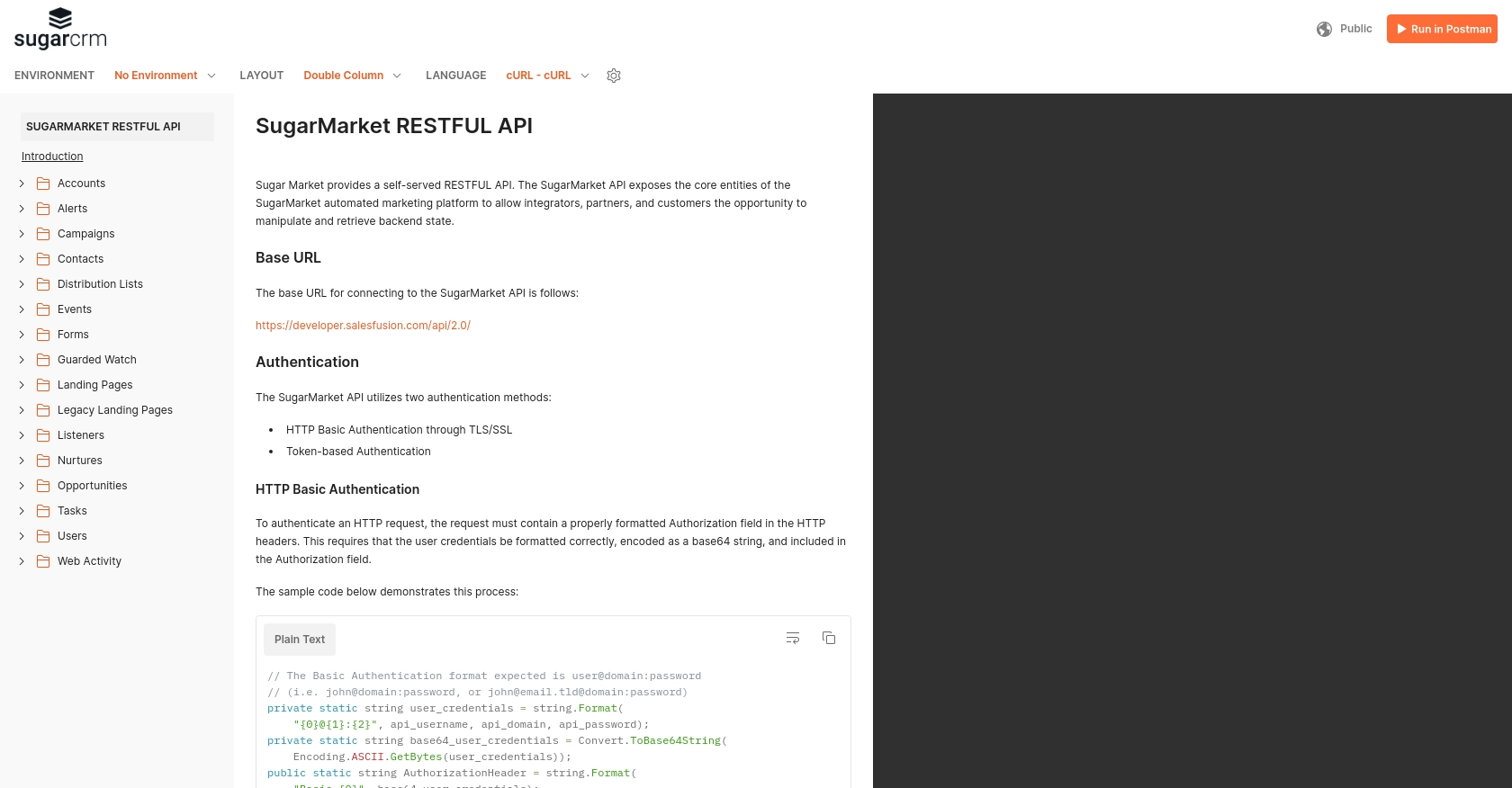
sbb-itb-96038d7
Making API Calls to Create Tasks with Sugar Market API in JavaScript
To create tasks using the Sugar Market API in JavaScript, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls, handling responses, and managing errors effectively.
Setting Up Your JavaScript Environment
Before you begin, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Node.js includes npm, which you'll use to manage dependencies.
Next, create a new project directory and initialize it with npm:
mkdir sugar-market-api
cd sugar-market-api
npm init -y
Installing Required Dependencies
You'll need the axios
library to make HTTP requests. Install it using npm:
npm install axios
Writing the JavaScript Code to Create Tasks
Create a new file named createTask.js
and add the following code:
const axios = require('axios');
// Replace with your actual client ID and client secret
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
// Function to get access token
async function getAccessToken() {
try {
const response = await axios.post('https://api.sugarmarket.com/oauth/token', {
client_id: clientId,
client_secret: clientSecret,
grant_type: 'client_credentials'
});
return response.data.access_token;
} catch (error) {
console.error('Error obtaining access token:', error.response.data);
}
}
// Function to create a task
async function createTask(accessToken) {
try {
const taskData = {
title: 'New Marketing Task',
description: 'Task description goes here',
due_date: '2023-12-31'
};
const response = await axios.post('https://api.sugarmarket.com/v1/tasks', taskData, {
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
console.log('Task created successfully:', response.data);
} catch (error) {
console.error('Error creating task:', error.response.data);
}
}
// Main function to execute the task creation
(async () => {
const accessToken = await getAccessToken();
if (accessToken) {
await createTask(accessToken);
}
})();
Running the JavaScript Code
Execute the script using Node.js:
node createTask.js
If successful, you should see a confirmation message in the console indicating that the task was created. If there are errors, the error message will provide details for troubleshooting.
Verifying Task Creation in Sugar Market
After running the script, log in to your Sugar Market sandbox account to verify that the task has been created. Navigate to the tasks section and check for the new task with the specified details.
Handling Errors and Debugging
When making API calls, it's essential to handle potential errors gracefully. The code above includes basic error handling, logging any issues to the console. For more detailed error handling, consider implementing additional checks based on the specific error codes returned by the API.
For more information on error codes and handling, refer to the Sugar Market API documentation.
Conclusion and Best Practices for Sugar Market API Integration
Integrating with the Sugar Market API using JavaScript can significantly enhance your marketing automation capabilities, allowing you to streamline task management and improve campaign efficiency. By following the steps outlined in this guide, you can create tasks programmatically, ensuring your marketing team remains organized and responsive to changing needs.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sugar Market API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized and consistent with your application's requirements. This will help maintain data integrity across systems.
Leverage Endgrate for Simplified Integration Management
While integrating with the Sugar Market API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and seamlessly connect with various platforms, including Sugar Market. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?