Using the Hubspot API to Get Products (Professional/Enterprise Only) (with Javascript examples)
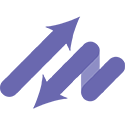
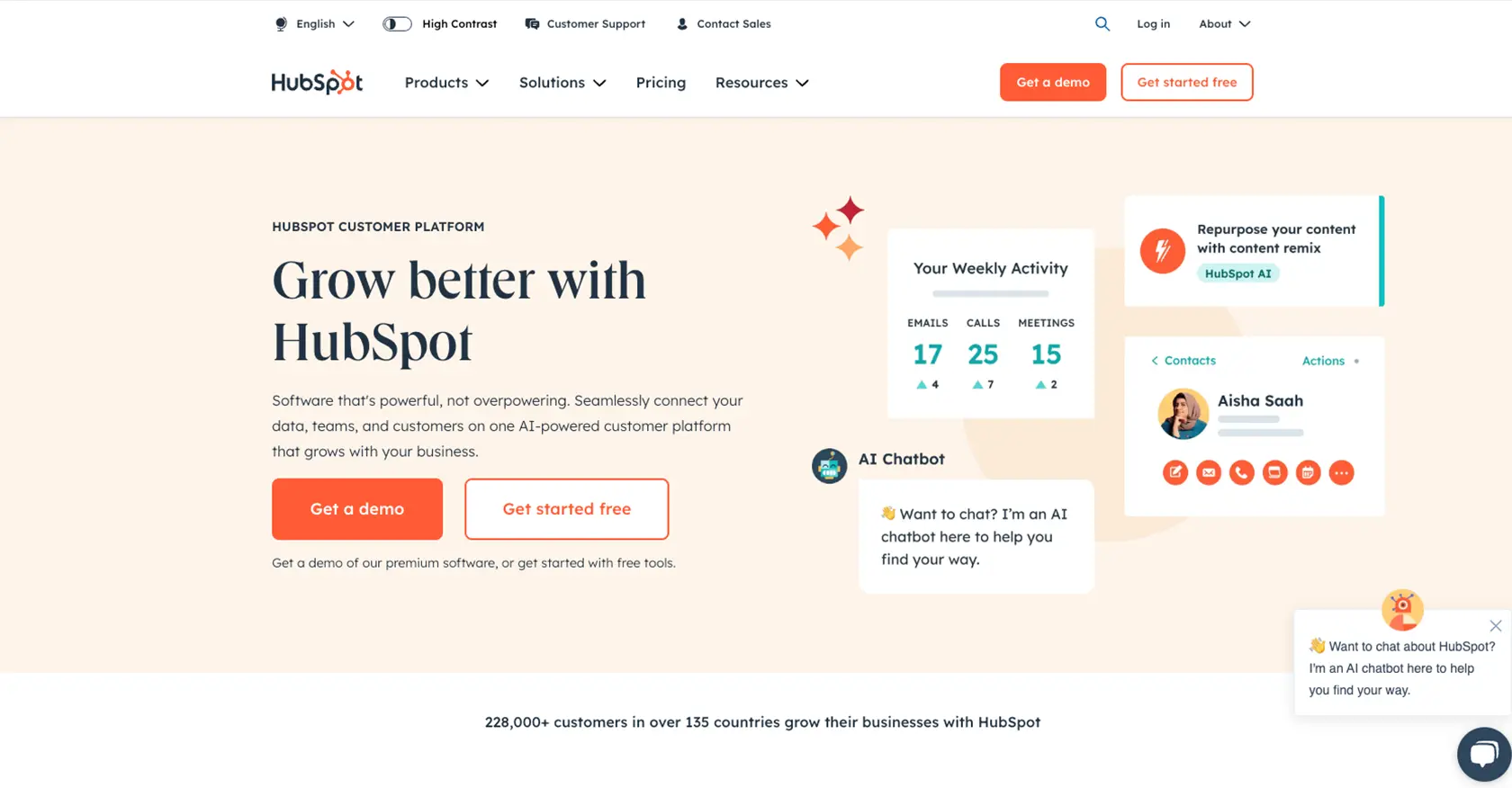
Introduction to HubSpot API for Product Management
HubSpot is a renowned CRM platform that offers a suite of tools to enhance marketing, sales, and customer service efforts. Its robust API capabilities allow developers to integrate seamlessly with various HubSpot features, enabling businesses to automate and optimize their workflows.
For developers working with B2B SaaS products, integrating with HubSpot's API can significantly streamline product management tasks. By accessing the HubSpot API, developers can efficiently manage product data, automate inventory updates, and synchronize product information across platforms.
In this article, we will explore how to use JavaScript to interact with the HubSpot API, specifically focusing on retrieving product information. This integration is particularly beneficial for businesses using HubSpot's Professional or Enterprise tiers, as it allows for advanced product management and data synchronization.
Setting Up Your HubSpot Sandbox Account for Product API Integration
Before diving into the HubSpot API for product management, it's essential to set up a sandbox account. This environment allows developers to test API interactions without affecting live data, ensuring a smooth integration process.
Creating a HubSpot Developer Account
To begin, you'll need a HubSpot developer account. This account provides access to create apps and test integrations:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and follow the registration process.
- Once registered, log in to your developer account.
Setting Up a HubSpot Sandbox Environment
HubSpot offers a sandbox environment for testing API integrations. Here's how to set it up:
- In your developer account, navigate to the "Test Accounts" section.
- Create a new test account by following the on-screen instructions.
- Once created, you'll have access to a sandbox environment where you can safely test API calls.
Creating a HubSpot App for OAuth Authentication
Since the HubSpot API uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials:
- Go to the "Apps" section in your developer account.
- Click on "Create an app" and fill in the required details, such as the app name and description.
- Under the "Auth" tab, configure the OAuth settings. Ensure you set the redirect URL to your application's URL.
- Save the app to generate a client ID and client secret, which you'll use for OAuth authentication.
Configuring OAuth Scopes for Product API Access
To interact with the HubSpot Product API, you'll need to configure the appropriate OAuth scopes:
- Navigate to the "Scopes" section of your app settings.
- Select the necessary scopes for product management, such as
e-commerce
for accessing product data. - Save your changes to update the app's permissions.
With your sandbox account and app set up, you're ready to start making API calls to manage products using JavaScript. This setup ensures a secure and efficient integration process, allowing you to test and refine your API interactions in a controlled environment.
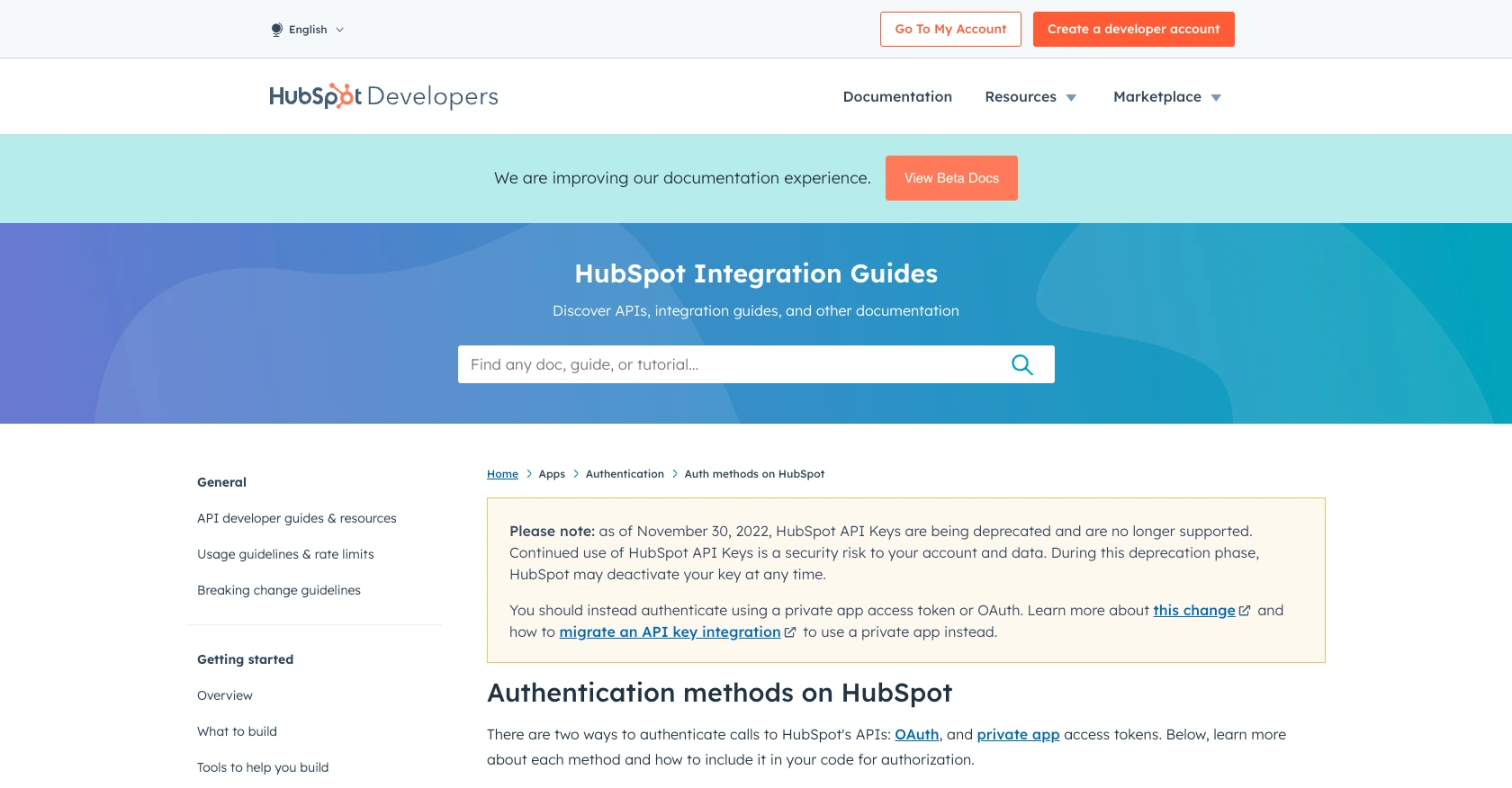
sbb-itb-96038d7
Making API Calls to Retrieve Products from HubSpot Using JavaScript
With your HubSpot sandbox account and OAuth app configured, you can now proceed to make API calls to retrieve product information. This section will guide you through the process of setting up your JavaScript environment and executing the necessary API calls to interact with HubSpot's Product API.
Setting Up Your JavaScript Environment for HubSpot API Integration
Before making API calls, ensure your JavaScript environment is properly set up. You'll need Node.js and npm (Node Package Manager) installed on your machine. Follow these steps to prepare your environment:
- Install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal. - Create a new project directory and navigate into it using your terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library for making HTTP requests with
npm install axios
.
Writing JavaScript Code to Retrieve Products from HubSpot
With your environment ready, you can now write the JavaScript code to interact with the HubSpot API. The following example demonstrates how to retrieve product data using Axios:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.hubapi.com/crm/v3/objects/products';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get products from HubSpot
async function getProducts() {
try {
const response = await axios.get(endpoint, { headers });
const products = response.data.results;
console.log('Retrieved Products:', products);
} catch (error) {
console.error('Error fetching products:', error.response.data);
}
}
// Execute the function
getProducts();
Replace YOUR_ACCESS_TOKEN
with the OAuth access token obtained from your HubSpot app setup. This token is crucial for authenticating your API requests.
Verifying Successful API Calls and Handling Errors
After executing the code, you should see the retrieved product data logged in your console. To verify the success of your API call:
- Check the console output for the list of products retrieved from HubSpot.
- Log in to your HubSpot sandbox account and navigate to the Products section to confirm the data matches.
In case of errors, the code will log the error details. Common issues include invalid tokens or incorrect endpoint URLs. Ensure your access token is valid and the endpoint is correctly specified.
For more information on error codes and handling, refer to the HubSpot API documentation.
Optimizing API Usage and Managing Rate Limits
HubSpot imposes rate limits on API calls to ensure fair usage. For Professional and Enterprise accounts, the limit is 150 requests per 10 seconds per app. To manage these limits effectively:
- Implement request throttling to avoid exceeding rate limits.
- Cache frequently accessed data to minimize unnecessary API calls.
- Monitor your API usage through the HubSpot developer portal.
For detailed rate limit information, visit the HubSpot API usage guidelines.
.webp)
Conclusion and Best Practices for HubSpot API Integration Using JavaScript
Integrating with the HubSpot API for product management can significantly enhance your business operations by automating and synchronizing product data across platforms. By following the steps outlined in this article, you can efficiently set up your environment, authenticate using OAuth, and make API calls to retrieve product information using JavaScript.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Implement request throttling and caching strategies to manage HubSpot's API rate limits effectively. This ensures your application remains within the allowed limits and avoids unnecessary API calls.
- Monitor API Usage: Regularly check your API usage through the HubSpot developer portal to ensure compliance with rate limits and optimize your integration's performance.
- Standardize Data Fields: When synchronizing product data, ensure that data fields are standardized across platforms to maintain consistency and accuracy.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including HubSpot, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, providing an easy and intuitive integration experience for your customers. Visit Endgrate to learn more about how it can help streamline your integration efforts.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/products
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?