How to Create or Update Invoices with the Sage 100 API in Python
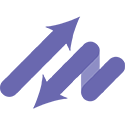
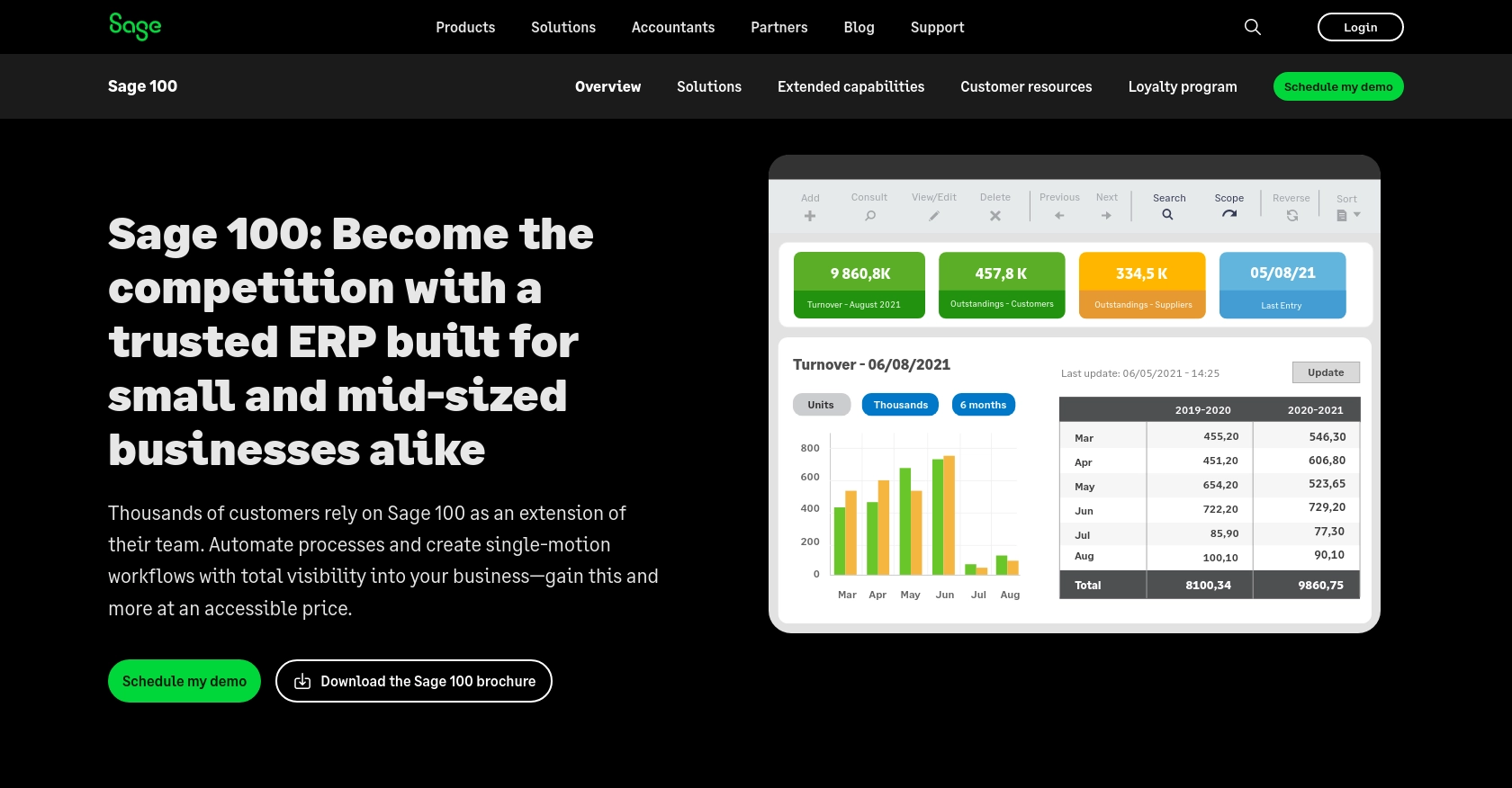
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive enterprise resource planning (ERP) solution designed to streamline business operations for small to medium-sized enterprises. It offers robust features for accounting, inventory management, and customer relationship management, making it a popular choice for businesses looking to enhance their operational efficiency.
Integrating with the Sage 100 API allows developers to automate and manage various business processes, such as invoicing. By leveraging the Sage 100 API, developers can create or update invoices programmatically, ensuring that financial data is accurate and up-to-date. For example, a developer might use the Sage 100 API to automatically generate invoices from sales data, reducing manual entry and minimizing errors.
Setting Up Your Sage 100 Test/Sandbox Account
Before diving into the Sage 100 API integration, it's essential to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data. Sage 100 provides a robust setup process to ensure developers can effectively test their integrations.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to install and configure the Sage 100 ODBC driver. This driver facilitates the connection between your application and the Sage 100 database.
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system. Ensure you use the correct server, database, and authentication settings.
- Verify the installation by testing the connection within the ODBC Data Source Administrator.
For detailed instructions, refer to the official Sage 100 ODBC configuration guide.
Creating a Sage 100 Sandbox Environment
Once the ODBC driver is configured, set up a sandbox environment in Sage 100. This environment allows you to test API interactions without impacting your production data.
- Log in to your Sage 100 account.
- Navigate to the system configuration settings.
- Enable the sandbox mode to create a separate testing environment.
Generating API Credentials for Sage 100
To authenticate API requests, you need to generate API credentials. Sage 100 uses a custom authentication method to secure API interactions.
- In your Sage 100 account, go to the API management section.
- Create a new API application and note down the client ID and client secret.
- Ensure that the application has the necessary permissions to create and update invoices.
Keep these credentials secure, as they are required for authenticating API requests.
Testing the Sage 100 API Connection
With your sandbox environment and API credentials ready, it's time to test the connection to the Sage 100 API.
- Use a tool like Postman or a simple Python script to make a test API call.
- Verify that the connection is successful and that you can retrieve or manipulate data as expected.
This step ensures that your setup is correct and ready for development.
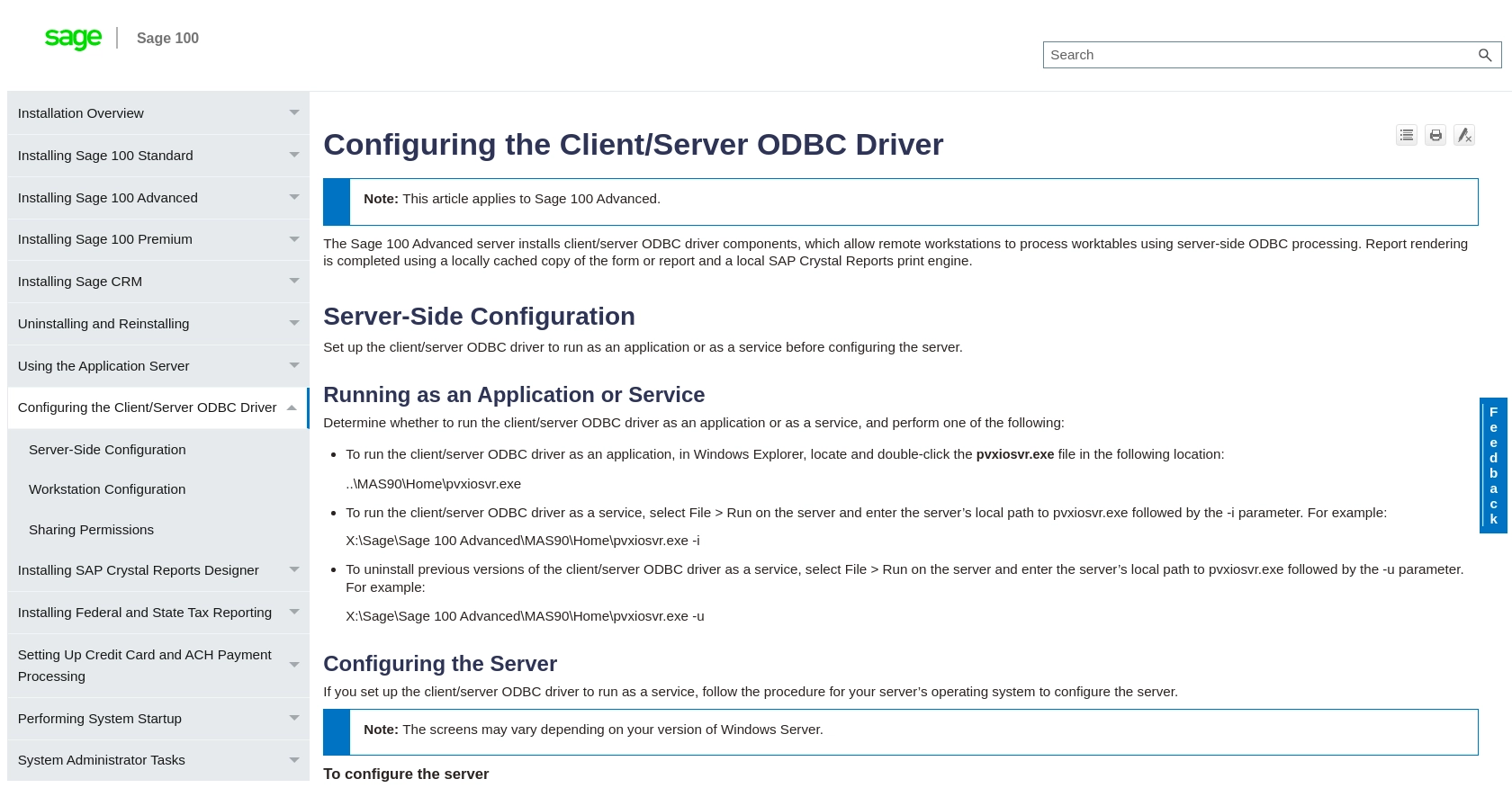
sbb-itb-96038d7
Making API Calls to Create or Update Invoices with Sage 100 in Python
To interact with the Sage 100 API for creating or updating invoices, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your Python Environment for Sage 100 API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x and the pyodbc
library to connect to the Sage 100 database via ODBC.
- Ensure Python 3.x is installed on your system. You can download it from the official Python website.
- Install the
pyodbc
library using pip:
pip install pyodbc
Writing Python Code to Create or Update Invoices in Sage 100
With your environment set up, you can now write Python code to interact with the Sage 100 API. Below is a sample script to create or update an invoice.
import pyodbc
# Define the DSN and credentials
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish a connection to the Sage 100 database
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
# Create a cursor object
cursor = connection.cursor()
# SQL query to create or update an invoice
sql_query = """
INSERT INTO AR_InvoiceHeader (InvoiceNo, CustomerNo, InvoiceDate, Amount)
VALUES (?, ?, ?, ?)
ON DUPLICATE KEY UPDATE
CustomerNo = VALUES(CustomerNo),
InvoiceDate = VALUES(InvoiceDate),
Amount = VALUES(Amount);
"""
# Define the invoice data
invoice_data = ('INV12345', 'CUST001', '2023-10-01', 1500.00)
# Execute the query
cursor.execute(sql_query, invoice_data)
# Commit the transaction
connection.commit()
# Close the connection
cursor.close()
connection.close()
print("Invoice created or updated successfully.")
Replace Your_DSN_Name
, Your_Username
, and Your_Password
with your actual Sage 100 credentials. The script uses an SQL query to insert or update an invoice in the AR_InvoiceHeader
table.
Verifying API Call Success and Handling Errors
After executing the API call, verify the success of the operation by checking the Sage 100 sandbox environment. Ensure the invoice data reflects the changes made by your script.
To handle errors, wrap your code in a try-except block to catch exceptions and log error messages:
try:
# Your code here
except pyodbc.Error as e:
print("Error occurred:", e)
This approach helps manage connection errors, timeouts, and query issues, ensuring robust error handling in your integration.
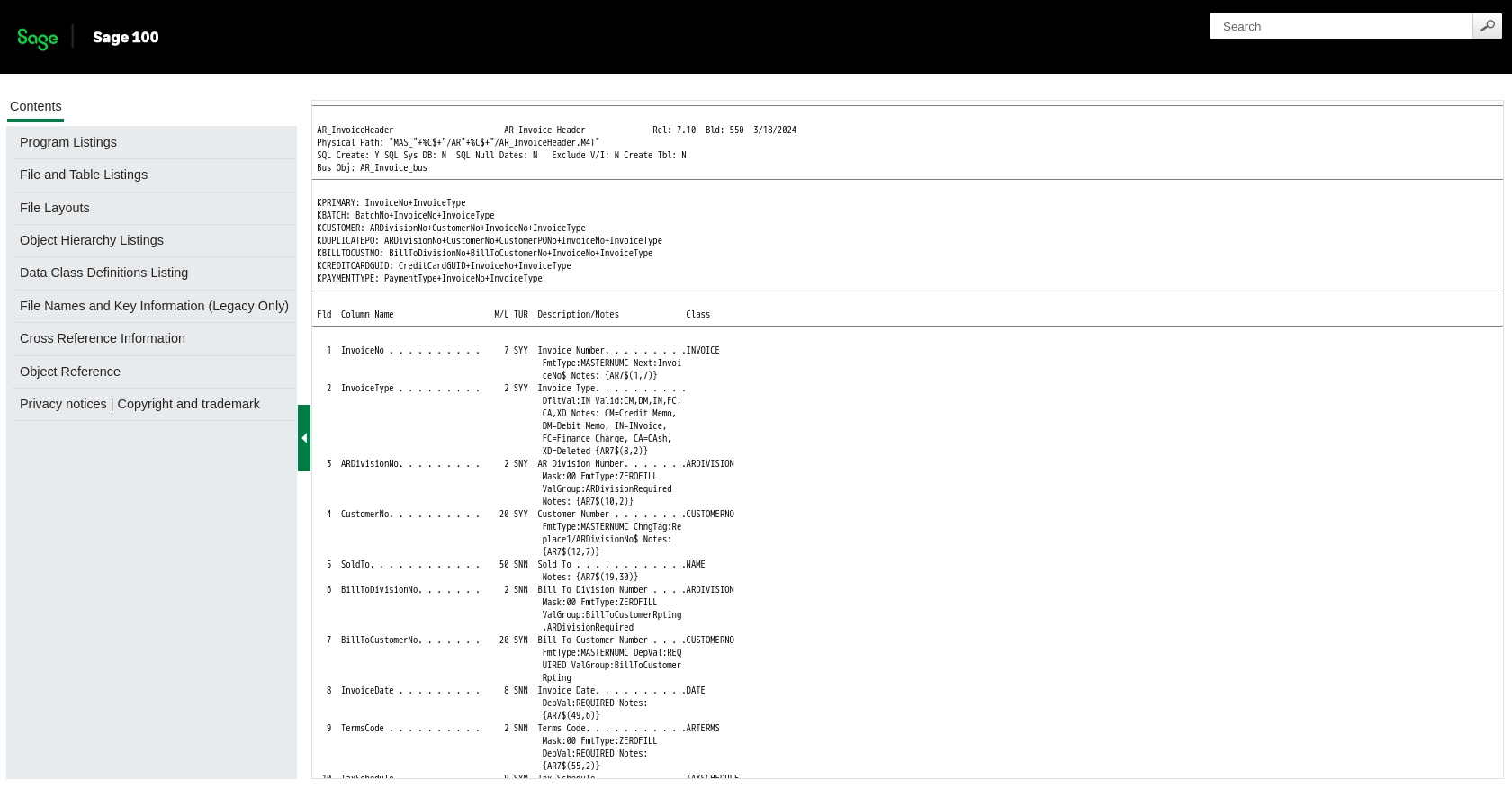
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API to create or update invoices can significantly enhance your business operations by automating financial processes and ensuring data accuracy. By following the steps outlined in this guide, you can efficiently set up your environment, write the necessary Python code, and handle any errors that may arise.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credentials: Always store your Sage 100 API credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send to and receive from the Sage 100 API is standardized. This helps maintain consistency across your systems.
- Error Logging: Implement comprehensive logging for your API interactions to track and debug any issues that may occur during integration.
Streamlining Integrations with Endgrate
While integrating with Sage 100 can be a powerful way to automate your business processes, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint for all your integration needs.
With Endgrate, you can save time and resources by building each use case once and applying it across various platforms. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Accounts_Receivable/AR_InvoiceHeader.htm?Highlight=AR_InvoiceHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Accounts_Receivable/AR_InvoiceDetail.htm?Highlight=AR_InvoiceDetail
Ready to get started?