How to Get Contacts with the Active Campaign API in Python
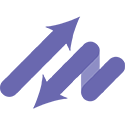
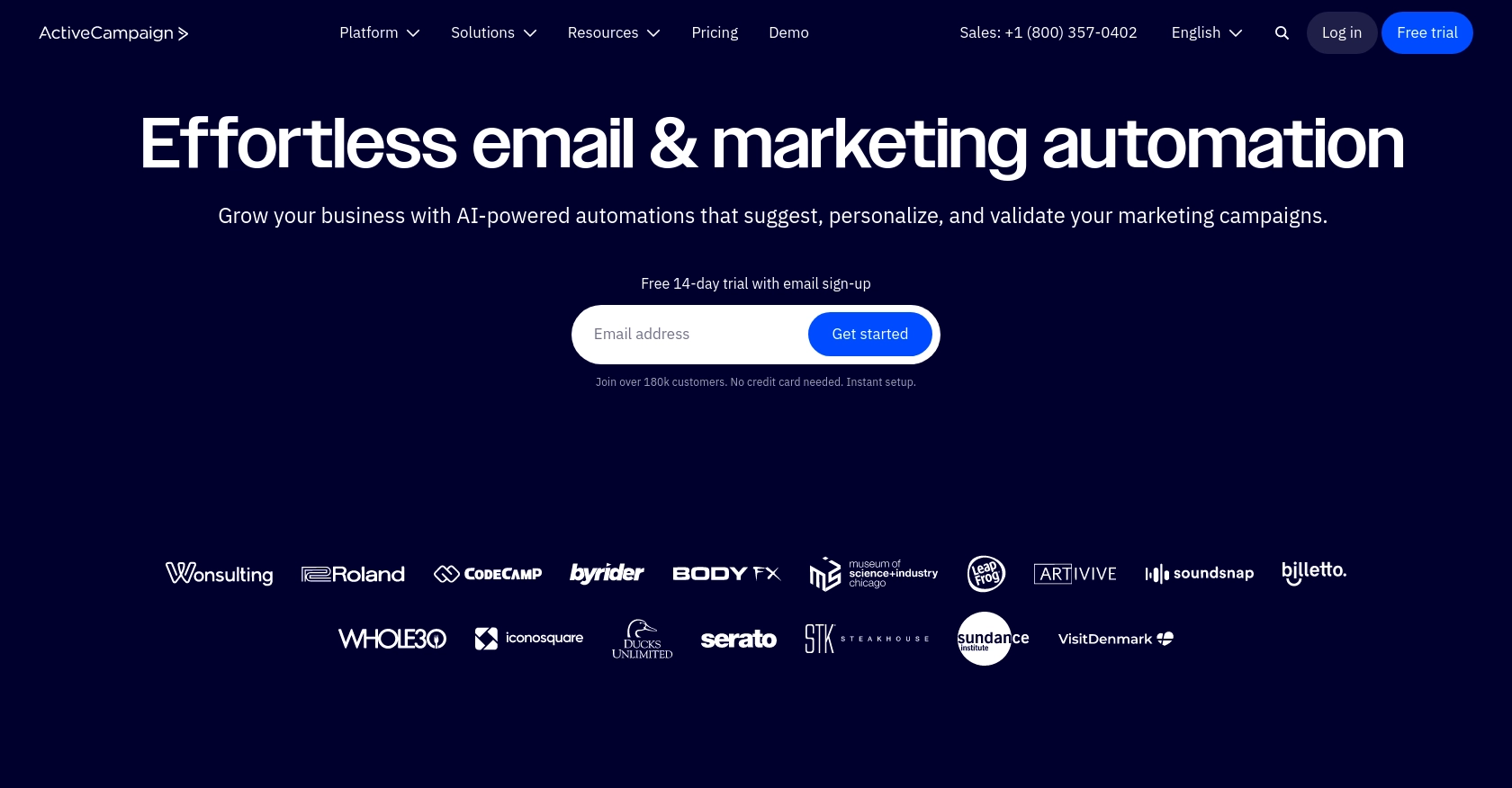
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that offers a suite of tools for email marketing, sales automation, and customer relationship management. It is widely used by businesses to enhance their marketing strategies and streamline customer interactions.
Developers often integrate with Active Campaign's API to access and manage contact data, enabling personalized marketing campaigns and automated workflows. For example, you can use the API to retrieve contact information and segment your audience based on their engagement levels, allowing for targeted email campaigns that improve conversion rates.
Setting Up Your Active Campaign Test Account
Before you can start using the Active Campaign API, you need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data.
Creating an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API.
- Visit the Active Campaign Free Trial page.
- Fill out the registration form with your details.
- Follow the instructions in the confirmation email to activate your account.
Generating Your API Key
Once your account is set up, you need to generate an API key to authenticate your requests. Follow these steps:
- Log in to your Active Campaign account.
- Navigate to the "Settings" page by clicking on your profile icon in the top right corner.
- Select the "Developer" tab from the sidebar.
- Here, you will find your unique API key. Make sure to keep this key secure and do not expose it in client-side code.
Understanding the Base URL
Active Campaign uses a base URL specific to your account for API requests. You can find this URL in the "Developer" tab under "Settings." The base URL typically follows this format:
https://.api-us1.com/api/3/
Ensure you replace <your-account-name>
with your actual account name when making API calls.
Setting Up OAuth for Authentication
Active Campaign supports API key-based authentication. Ensure you include your API key in the HTTP header as follows:
headers = {
"Api-Token": "your_api_key"
}
Replace your_api_key
with the API key you generated earlier.
With your test account and API key ready, you're all set to start interacting with the Active Campaign API using Python.
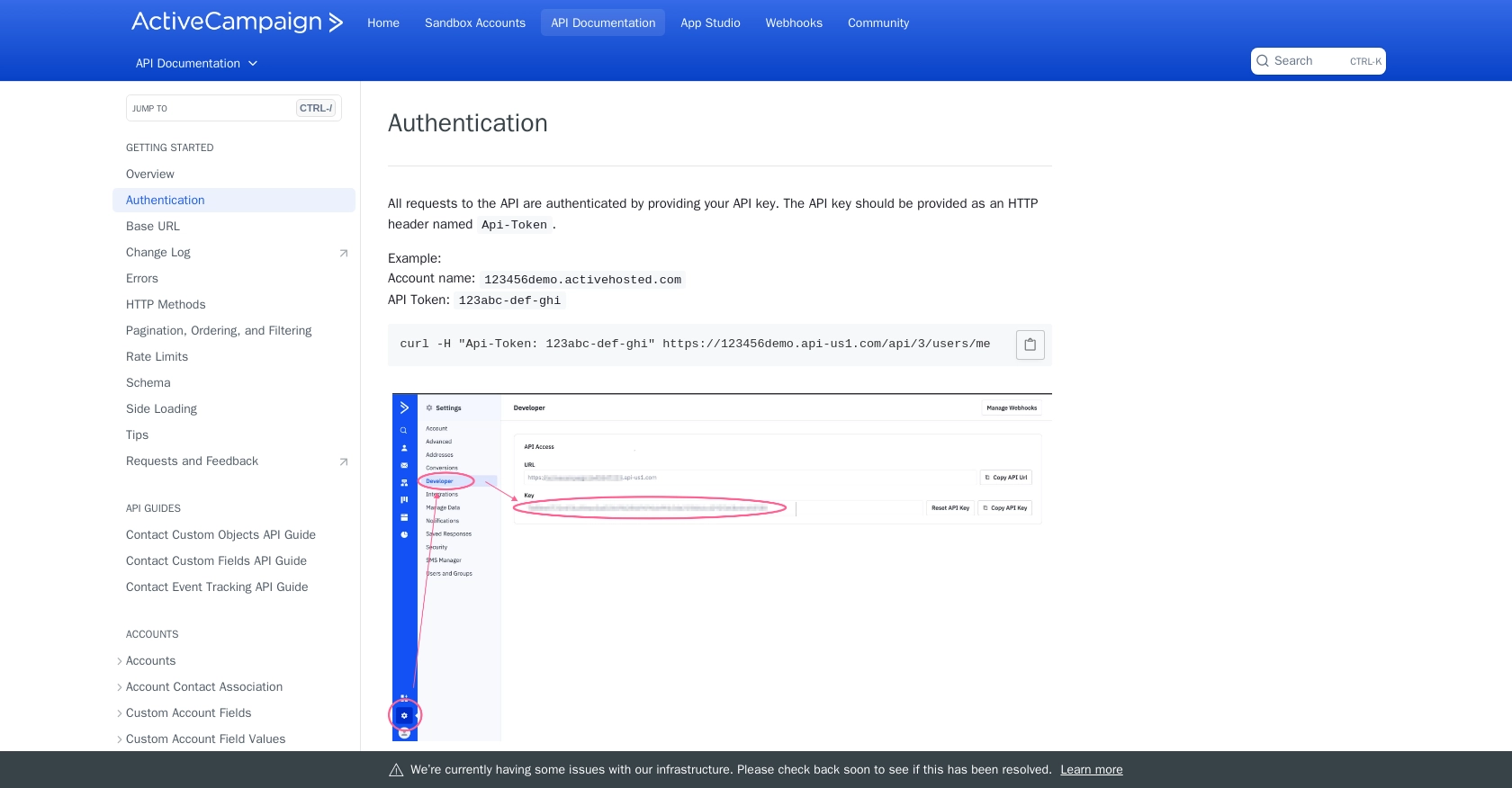
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Active Campaign Using Python
To interact with the Active Campaign API and retrieve contact data, you'll need to use Python. This section will guide you through the process of setting up your environment and making the necessary API calls.
Setting Up Your Python Environment for Active Campaign API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Fetch Contacts from Active Campaign
Now that your environment is ready, you can write a Python script to retrieve contacts from Active Campaign. Create a new file named get_active_campaign_contacts.py
and add the following code:
import requests
# Define the API endpoint and headers
base_url = "https://.api-us1.com/api/3/"
endpoint = "contacts"
url = f"{base_url}{endpoint}"
headers = {
"Api-Token": "your_api_key",
"accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json().get("contacts", [])
for contact in contacts:
print(f"Name: {contact.get('firstName')} {contact.get('lastName')}, Email: {contact.get('email')}")
else:
print(f"Failed to retrieve contacts: {response.status_code} - {response.text}")
Replace <your-account-name>
with your actual account name and your_api_key
with your API key.
Running the Python Script to Retrieve Contacts
Execute the script from your terminal using the following command:
python get_active_campaign_contacts.py
If successful, the script will print out the names and email addresses of the contacts retrieved from your Active Campaign account.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The script checks the response status code to ensure the request was successful. If the status code is not 200, it prints an error message with the status code and response text.
To verify the success of your API call, you can cross-check the retrieved contacts with the data in your Active Campaign account.
For more information on handling errors and understanding response codes, refer to the Active Campaign API documentation.
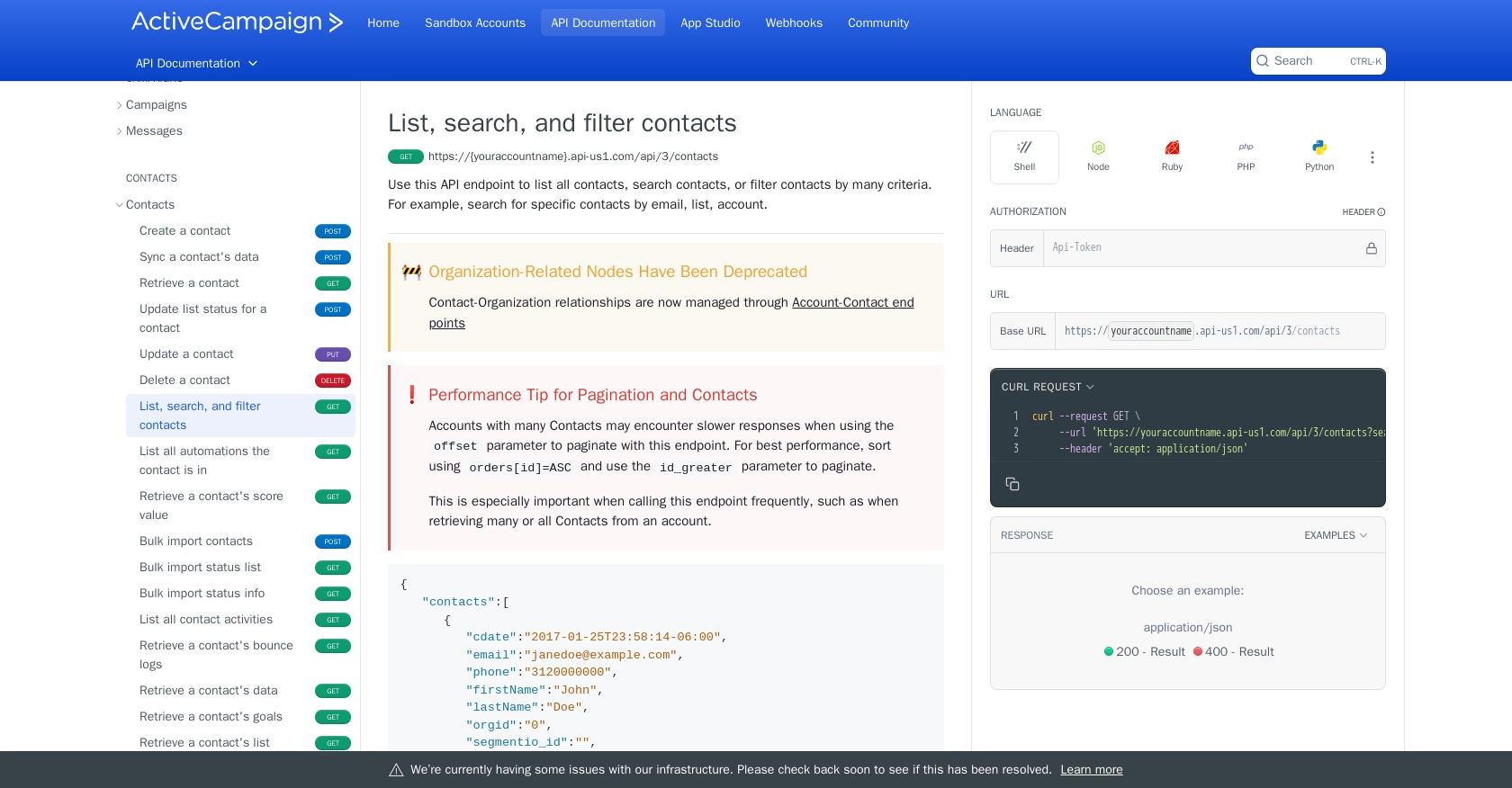
Best Practices for Using the Active Campaign API
When working with the Active Campaign API, it's essential to follow best practices to ensure efficient and secure integration. Here are some tips to help you get the most out of your API interactions:
- Secure Your API Key: Always keep your API key confidential. Avoid exposing it in client-side code or public repositories.
- Handle Rate Limits: Active Campaign imposes a rate limit of 5 requests per second per account. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the rate limits documentation.
- Optimize Data Retrieval: Use pagination and filtering to retrieve only the necessary data. This reduces the load on the API and speeds up your application.
- Error Handling: Implement robust error handling to manage different response codes and potential issues. This ensures your application can recover gracefully from API errors.
- Data Transformation: Standardize and transform data fields as needed to match your application's requirements, ensuring consistency across different integrations.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and resource-intensive. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Active Campaign.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-contacts
Ready to get started?