Using the Copper API to Create or Update Companies in Javascript
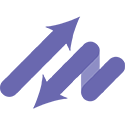
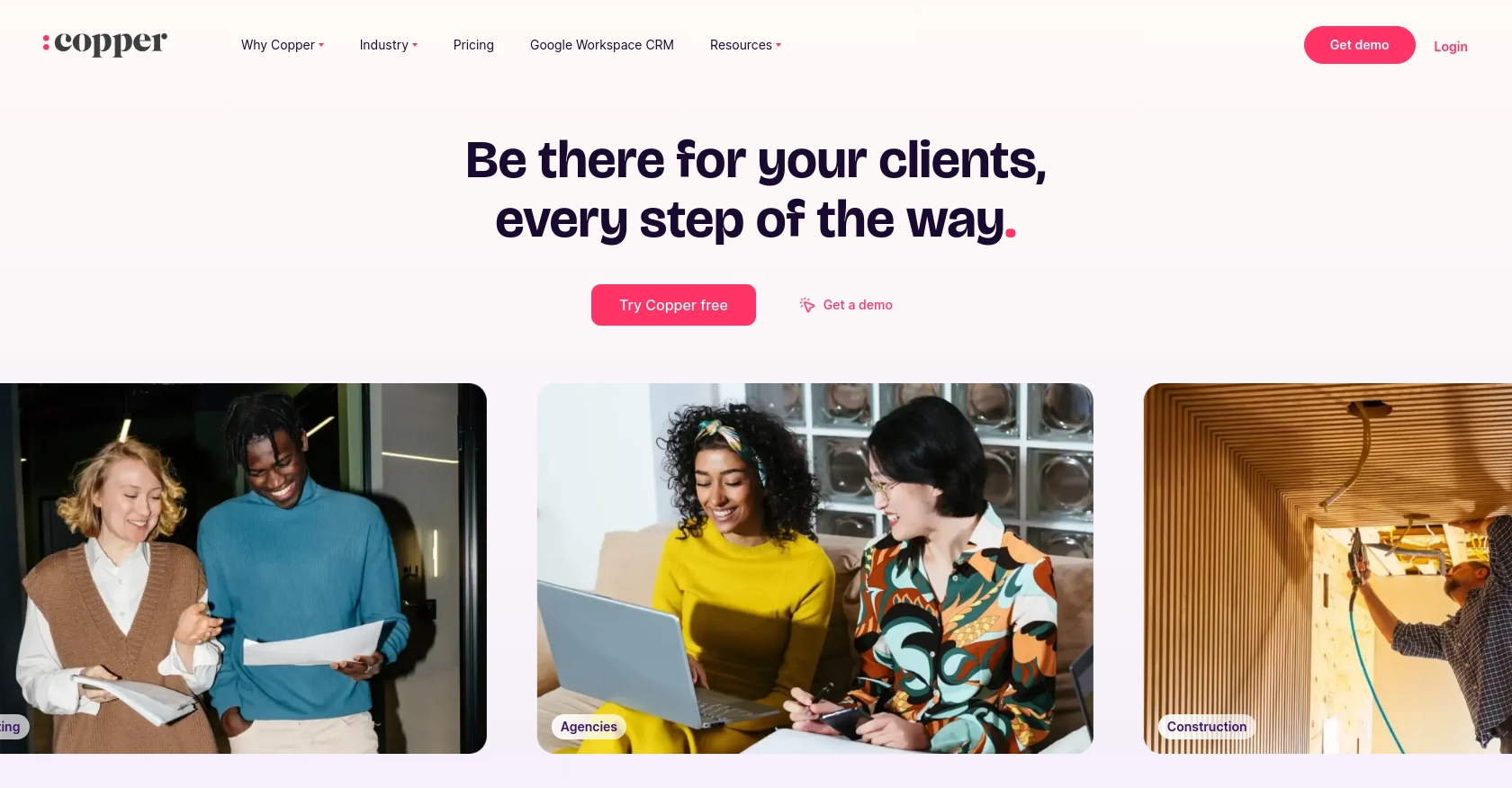
Introduction to Copper API for Company Management
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with an efficient way to manage customer relationships. Its intuitive interface and robust features make it a popular choice for companies looking to enhance their CRM capabilities.
Developers may want to integrate with the Copper API to automate and streamline various business processes. For example, using the Copper API, developers can create or update company records programmatically, ensuring that the CRM data remains accurate and up-to-date. This can be particularly useful for businesses that need to synchronize data between Copper and other systems, reducing manual data entry and improving operational efficiency.
Setting Up Your Copper Test or Sandbox Account
Before you can start integrating with the Copper API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Copper Account
If you don't already have a Copper account, you can sign up for a free trial on the Copper website. This will give you access to the platform and its features, allowing you to explore its capabilities.
Access the Copper Developer Portal
Once you have your Copper account, navigate to the Copper Developer Portal. This portal provides the necessary tools and documentation to help you integrate with Copper's API.
Generate API Key and Secret
To authenticate your API requests, you'll need an API key and secret. Follow these steps to generate them:
- Log in to your Copper account and go to the settings page.
- Navigate to the "API Keys" section.
- Click on "Create API Key" and fill in the required details.
- Once created, you'll receive an API key and secret. Make sure to store these securely, as you'll need them for authentication.
Configure API Authentication
Copper uses a custom authentication method that requires you to sign your requests. Here's how you can set it up in JavaScript:
const crypto = require('crypto');
const axios = require('axios');
const apiKey = 'your_api_key';
const apiSecret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'GET'; // or POST, PATCH, etc.
const path = '/v1/companies'; // example endpoint
const body = ''; // request body if applicable
const signature = crypto.createHmac('sha256', apiSecret)
.update(timestamp + method + path + body)
.digest('hex');
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp
};
// Example API call
axios.get('https://api.copper.co' + path, { headers })
.then(response => console.log(response.data))
.catch(error => console.error(error));
Test Your API Calls
With your API key and secret configured, you can now test your API calls in the Copper sandbox environment. This allows you to verify that your integration works as expected before deploying it to production.
By following these steps, you'll be well-prepared to start integrating with the Copper API and automating your company management processes.
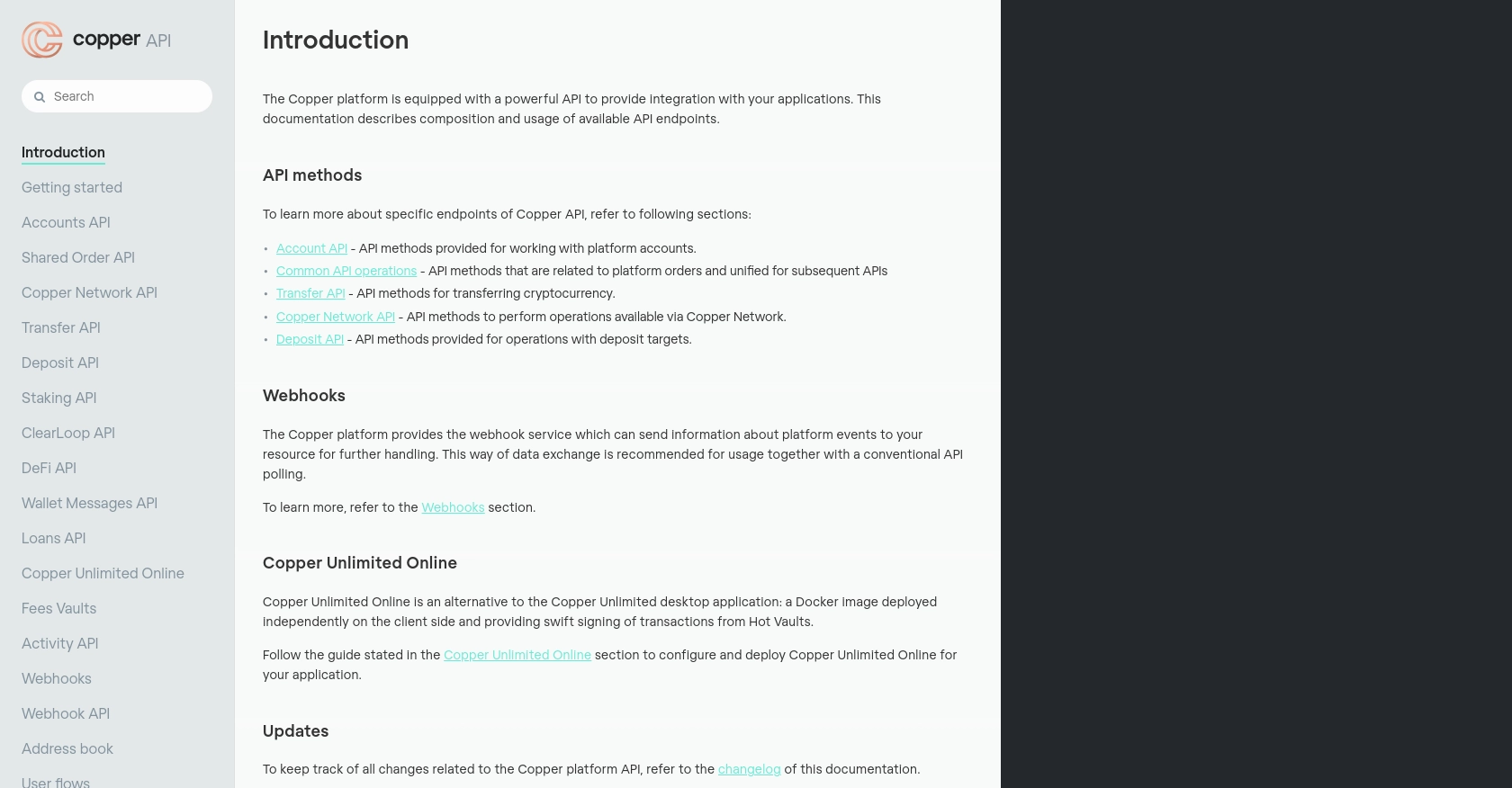
sbb-itb-96038d7
Making API Calls to Copper for Company Management in JavaScript
To interact with the Copper API for creating or updating company records, you'll need to set up your JavaScript environment and make authenticated requests. This section will guide you through the necessary steps to perform these API calls effectively.
Setting Up Your JavaScript Environment for Copper API Integration
Before making API calls, ensure you have Node.js installed on your machine. You'll also need the axios
and crypto
libraries for making HTTP requests and generating signatures, respectively. Install them using the following command:
npm install axios crypto
Creating and Updating Companies Using Copper API
With your environment ready, you can now create or update company records in Copper. Below is an example of how to make a POST request to create a new company:
const crypto = require('crypto');
const axios = require('axios');
const apiKey = 'your_api_key';
const apiSecret = 'your_api_secret';
const timestamp = Date.now().toString();
const method = 'POST';
const path = '/v1/companies';
const body = JSON.stringify({
name: 'New Company',
domain: 'newcompany.com'
});
const signature = crypto.createHmac('sha256', apiSecret)
.update(timestamp + method + path + body)
.digest('hex');
const headers = {
'Authorization': `ApiKey ${apiKey}`,
'X-Signature': signature,
'X-Timestamp': timestamp,
'Content-Type': 'application/json'
};
axios.post('https://api.copper.co' + path, body, { headers })
.then(response => console.log('Company Created:', response.data))
.catch(error => console.error('Error Creating Company:', error));
Handling API Responses and Errors
After making an API call, it's crucial to handle the response and any potential errors. Successful responses will typically return a 2xx status code, while errors may return 4xx or 5xx codes. Here's how you can manage these responses:
- Check the status code to determine if the request was successful.
- Log the response data for successful requests to verify the company details.
- Handle errors by logging them and implementing retry logic if necessary.
Verifying API Call Success in Copper Sandbox
To ensure your API calls are successful, verify the changes in your Copper sandbox account. Check if the new or updated company records appear as expected. This step is vital for confirming that your integration works correctly before deploying it to a live environment.
By following these steps, you can efficiently manage company records in Copper using JavaScript, streamlining your CRM processes and enhancing data accuracy.
Conclusion and Best Practices for Copper API Integration in JavaScript
Integrating with the Copper API using JavaScript offers a streamlined approach to managing company records, enhancing your CRM processes, and ensuring data accuracy. By following the steps outlined in this guide, you can effectively create and update company records, automate data synchronization, and reduce manual entry.
Best Practices for Secure and Efficient Copper API Integration
- Secure API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Copper's API rate limits. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Validation: Validate data before sending it to the Copper API to prevent errors and ensure data integrity.
- Error Handling: Implement comprehensive error handling to manage API response errors and network issues effectively.
- Testing in Sandbox: Always test your API calls in the Copper sandbox environment before deploying to production to ensure everything works as expected.
Enhance Your Integration Experience with Endgrate
Consider leveraging Endgrate to further simplify your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration efforts and enhance your business operations.
Read More
Ready to get started?