How to Get Companies with the Apollo API in Python
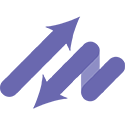
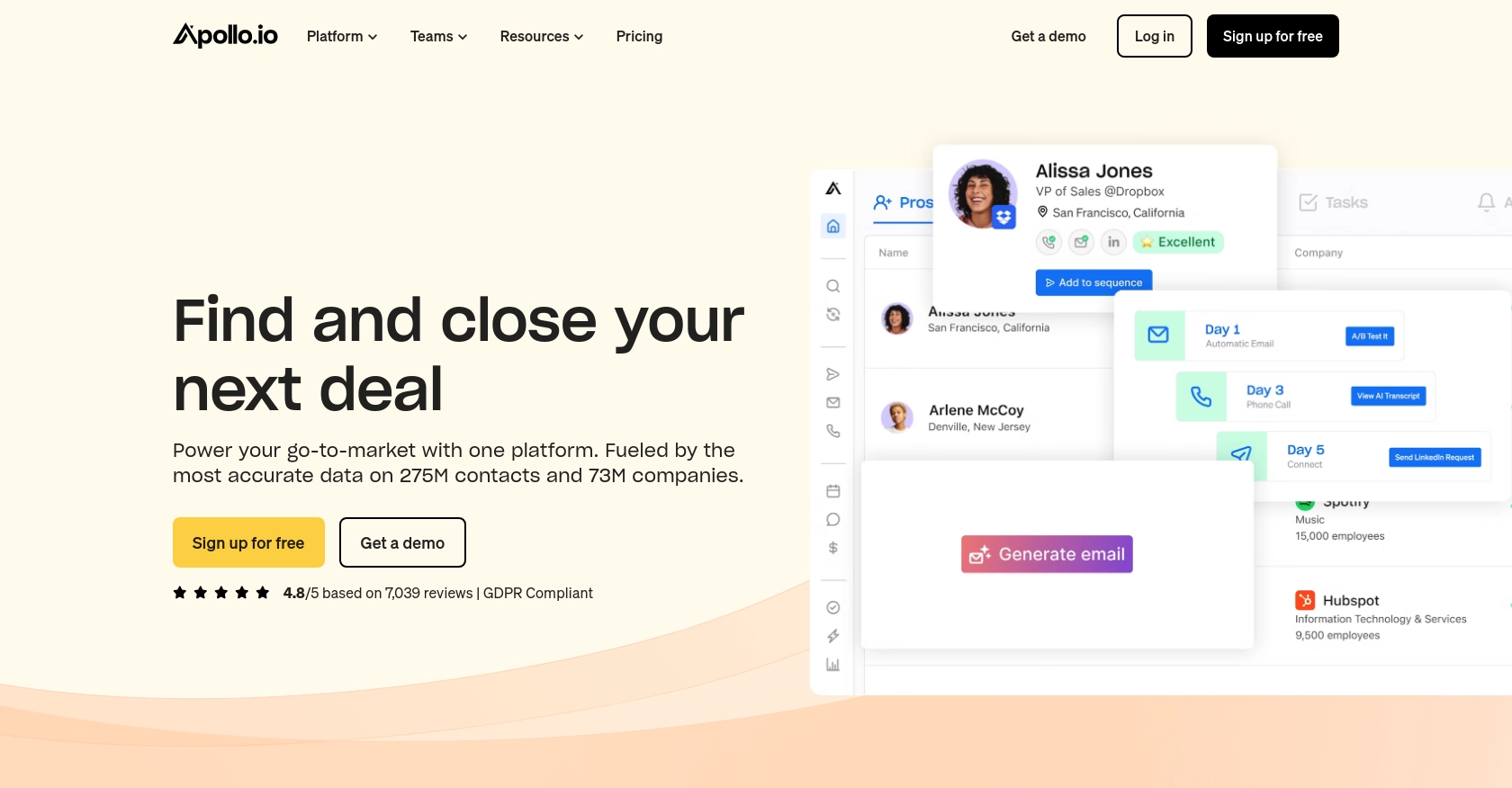
Introduction to Apollo API for Company Data Integration
Apollo is a powerful sales intelligence and engagement platform that provides businesses with access to a vast database of contacts and companies. It offers tools to enhance sales strategies, streamline outreach efforts, and improve lead generation.
Developers may want to integrate with the Apollo API to access detailed company information, which can be used to enrich CRM systems, automate data entry, and enhance marketing campaigns. For example, a developer could use the Apollo API to retrieve company data and integrate it into a custom dashboard for sales analysis.
Setting Up Your Apollo API Test Account
Before you can start using the Apollo API to retrieve company data, you'll need to set up a test account. This will allow you to experiment with the API without affecting any live data.
Create an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the necessary tools and features to begin testing the API.
- Visit the Apollo website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access the Apollo dashboard.
Generate an API Key for Authentication
The Apollo API uses API key-based authentication. Follow these steps to generate your API key:
- Log in to your Apollo account and navigate to the API settings section.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Configure API Key in Your Application
With your API key ready, you can now configure it in your application to authenticate requests to the Apollo API. Here's a basic example of how to set up the API key in Python:
import requests
# Define the API endpoint and headers
url = "https://api.apollo.io/v1/auth/health"
headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
# Make a request to verify the API key
response = requests.get(url, headers=headers)
print(response.text)
Replace YOUR_API_KEY_HERE
with the actual API key you generated. Running this code should return a response indicating whether the authentication was successful.
With your test account and API key set up, you're now ready to start making API calls to retrieve company data using the Apollo API.
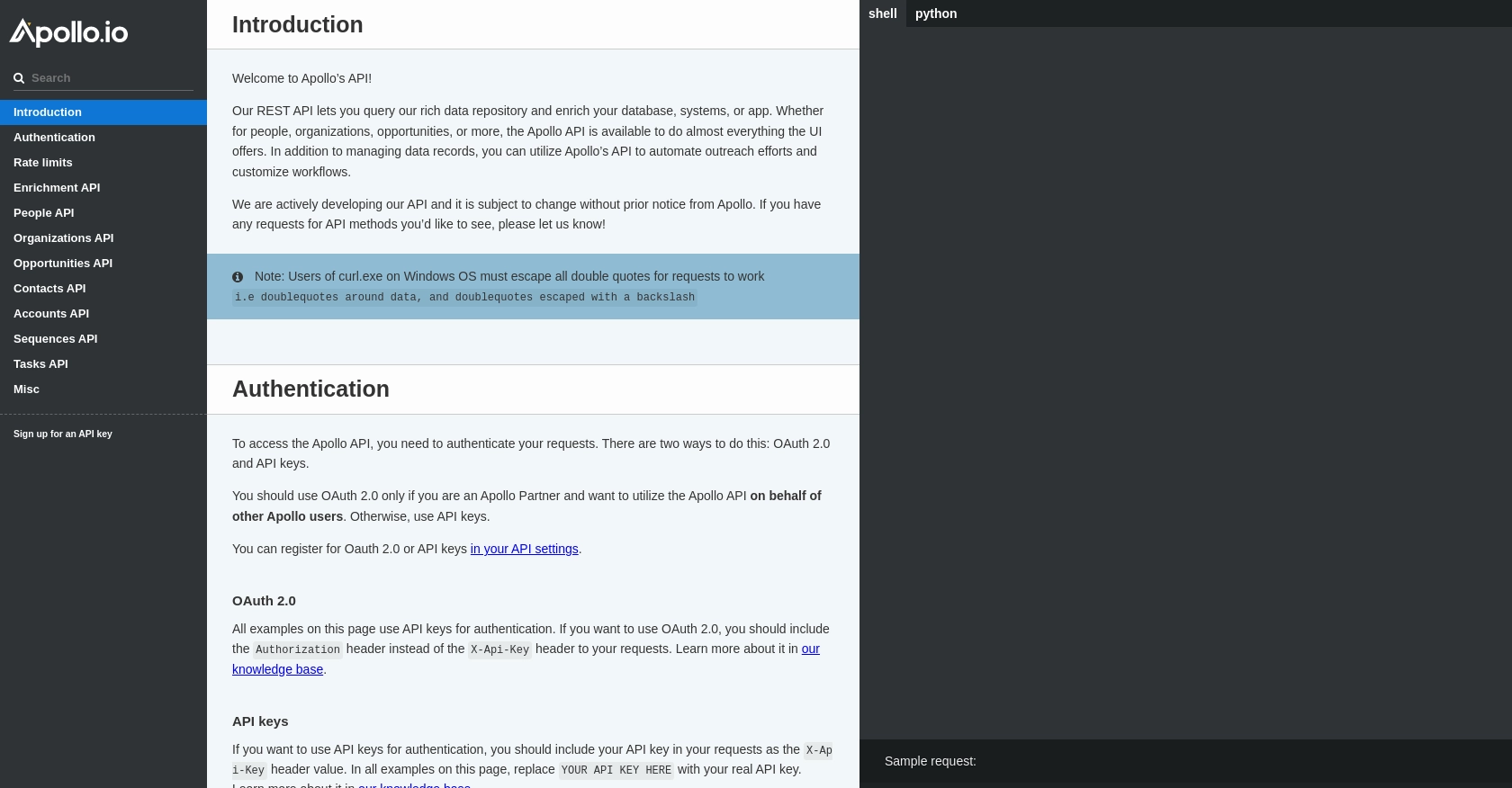
sbb-itb-96038d7
Making API Calls to Retrieve Company Data with Apollo API in Python
To effectively interact with the Apollo API and retrieve company data, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses.
Setting Up Your Python Environment for Apollo API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Company Data from Apollo API
Now that your environment is set up, you can write the Python code to interact with the Apollo API. Here's a step-by-step guide:
import requests
# Define the API endpoint for retrieving company data
url = "https://api.apollo.io/v1/organizations/enrich"
# Set the headers with your API key
headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
# Define the query parameters with the company domain
querystring = {"domain": "example.com"}
# Make the GET request to the Apollo API
response = requests.get(url, headers=headers, params=querystring)
# Check if the request was successful
if response.status_code == 200:
# Parse and print the JSON response
company_data = response.json()
print(company_data)
else:
print(f"Error: {response.status_code} - {response.text}")
Replace YOUR_API_KEY_HERE
with your actual API key and example.com
with the domain of the company you wish to retrieve data for.
Handling API Responses and Errors
After making the API call, it's crucial to handle the response correctly. The above code checks for a successful status code (200) and then parses the JSON response to extract company data. If the request fails, it prints an error message with the status code and response text.
To verify the request's success, you can cross-check the returned data with the Apollo dashboard. If the data matches, your integration is working correctly.
Understanding Apollo API Rate Limits
Be mindful of Apollo's rate limits to avoid exceeding the allowed number of requests. According to Apollo's documentation, the rate limits are:
- 50 requests per minute
- 100 requests per hour
- 300 requests per day
Monitor your API usage by checking the response headers, which provide details about your remaining requests.
For more information on error codes and rate limits, refer to the Apollo API documentation.
Best Practices for Using Apollo API in Python
When integrating with the Apollo API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Implement logic to handle rate limits by monitoring the response headers for remaining requests and implementing retries with exponential backoff if necessary.
- Data Standardization: Standardize and transform data fields to match your application's requirements, ensuring consistency across different data sources.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and error messages, ensuring your application can gracefully handle failures.
Conclusion and Call to Action for Using Endgrate
Integrating with the Apollo API can significantly enhance your sales and marketing efforts by providing access to rich company data. By following the steps outlined in this guide, you can efficiently retrieve and utilize this data within your applications.
However, managing multiple integrations can be time-consuming and complex. Consider using Endgrate to streamline your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can simplify your integration needs and enhance your product offerings.
Read More
Ready to get started?