How to Get Calendar Events with the Google Calendar API in Javascript
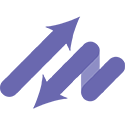
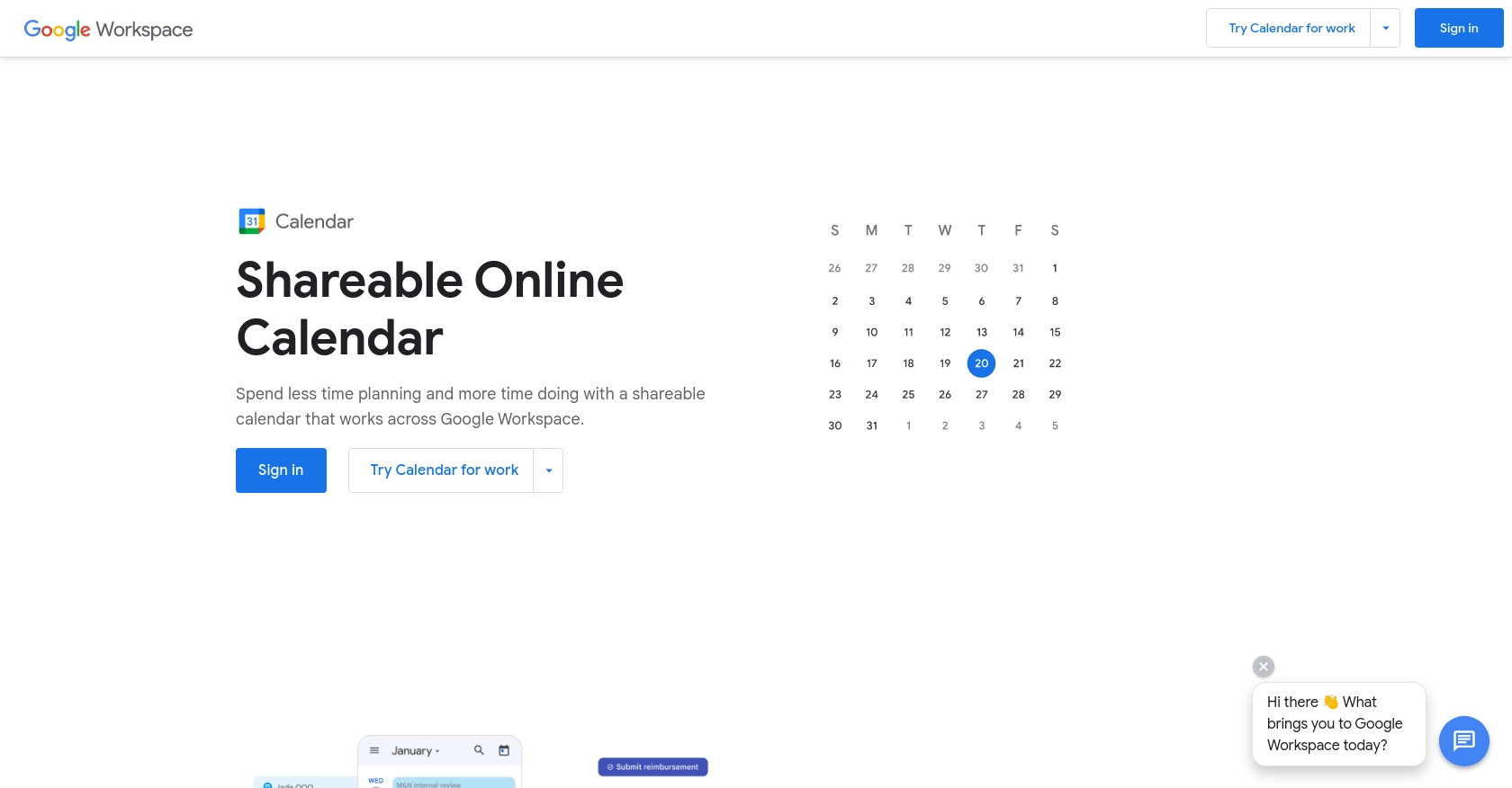
Introduction to Google Calendar API Integration
Google Calendar is a widely used time-management and scheduling service that allows users to create and edit events. It is a part of the Google Workspace suite and is known for its seamless integration with other Google services, making it a popular choice for both personal and professional use.
Developers often integrate with the Google Calendar API to automate and enhance calendar functionalities within their applications. For example, a developer might want to retrieve calendar events to display them in a custom dashboard or synchronize them with another scheduling tool. This integration can streamline workflows and improve productivity by providing real-time access to calendar data.
Setting Up Your Google Calendar API Test Account
Before you can start integrating with the Google Calendar API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This will allow you to securely access calendar data and perform API requests.
Create a Google Cloud Project
To begin, you'll need a Google Cloud project. Follow these steps to create one:
- Go to the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and select your organization if applicable.
- Click Create to set up your project.
Enable Google Calendar API
Next, you need to enable the Google Calendar API for your project:
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for Google Calendar API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
Set up the OAuth consent screen to define how your application will request access:
- Navigate to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as application name and support email, then click Save and Continue.
- Add any necessary scopes and test users if your app is external, then click Save and Continue.
Create OAuth Credentials
Finally, create OAuth 2.0 credentials to authenticate your app:
- Go to Menu > APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for your credentials and add your authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the official documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
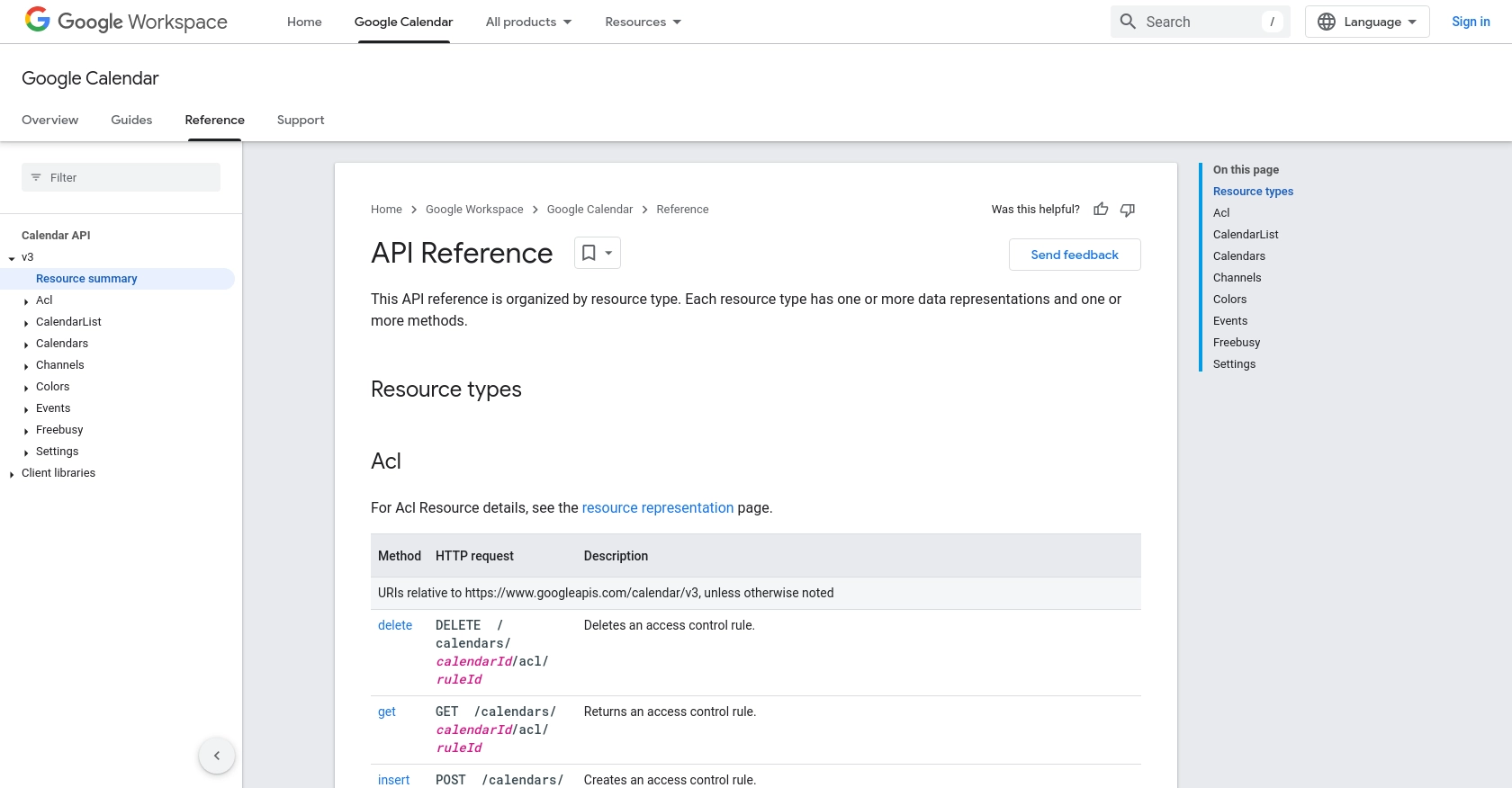
sbb-itb-96038d7
Making API Calls to Retrieve Google Calendar Events Using JavaScript
To interact with the Google Calendar API and retrieve calendar events using JavaScript, you'll need to set up your development environment and write code to make authenticated API requests. This section will guide you through the necessary steps to achieve this.
Prerequisites for Google Calendar API Integration with JavaScript
Before proceeding, ensure you have the following installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
With Node.js and NPM installed, you can manage dependencies and run JavaScript code efficiently.
Installing Required Dependencies for Google Calendar API
To interact with the Google Calendar API, you'll need to install the googleapis
package. Open your terminal and run the following command:
npm install googleapis
Writing JavaScript Code to Fetch Google Calendar Events
Now, let's write the JavaScript code to authenticate and fetch events from Google Calendar. Create a file named getCalendarEvents.js
and add the following code:
const { google } = require('googleapis');
const { OAuth2 } = google.auth;
// Initialize OAuth2 client
const oAuth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set the access token
oAuth2Client.setCredentials({
refresh_token: 'YOUR_REFRESH_TOKEN',
});
// Initialize Google Calendar API
const calendar = google.calendar({ version: 'v3', auth: oAuth2Client });
// Function to list calendar events
async function listEvents() {
try {
const response = await calendar.events.list({
calendarId: 'primary',
timeMin: (new Date()).toISOString(),
maxResults: 10,
singleEvents: true,
orderBy: 'startTime',
});
const events = response.data.items;
if (events.length) {
console.log('Upcoming 10 events:');
events.map((event, i) => {
const start = event.start.dateTime || event.start.date;
console.log(`${start} - ${event.summary}`);
});
} else {
console.log('No upcoming events found.');
}
} catch (error) {
console.error('Error fetching events:', error);
}
}
listEvents();
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, and YOUR_REFRESH_TOKEN
with your actual OAuth 2.0 credentials.
Running the JavaScript Code to Retrieve Events
Once your code is ready, run it using the following command in your terminal:
node getCalendarEvents.js
You should see a list of upcoming events from your Google Calendar printed in the console.
Handling Errors and Verifying API Requests
While making API requests, it's crucial to handle potential errors. The code above includes a try-catch
block to catch and log any errors that occur during the API call. Ensure that your OAuth credentials are correct and that the Google Calendar API is enabled for your project.
To verify that the request succeeded, you can cross-check the output with your Google Calendar to ensure the events match.
For more detailed information on the API call, refer to the official documentation: Google Calendar API Events: get.
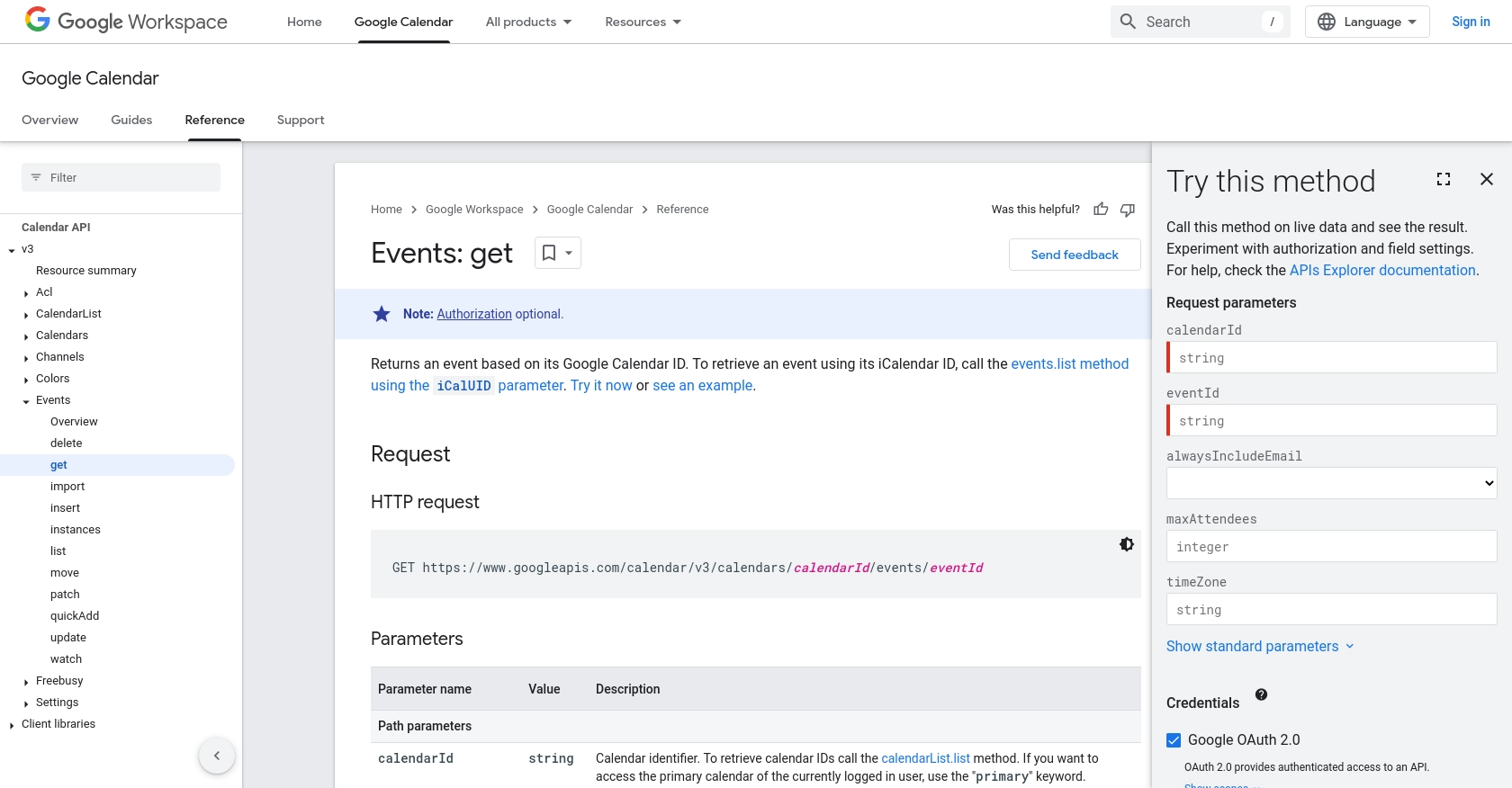
Conclusion and Best Practices for Google Calendar API Integration with JavaScript
Integrating with the Google Calendar API using JavaScript can significantly enhance your application's scheduling capabilities by providing seamless access to calendar events. This integration allows developers to automate workflows, synchronize data, and create custom solutions tailored to user needs.
Best Practices for Secure and Efficient Google Calendar API Usage
- Securely Store Credentials: Ensure that your OAuth 2.0 credentials, such as client ID and client secret, are stored securely and not exposed in your codebase. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the Google Calendar API's rate limits to avoid exceeding the allowed number of requests. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Optimize Data Handling: Transform and standardize data fields to ensure consistency across your application. This will help in maintaining data integrity and improve the user experience.
- Implement Error Handling: Use robust error handling mechanisms to manage API call failures. Log errors for debugging and provide meaningful feedback to users when issues arise.
Leverage Endgrate for Streamlined Integration Solutions
For developers looking to simplify and accelerate their integration processes, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including Google Calendar, allowing you to focus on your core product while outsourcing complex integrations. This approach not only saves time and resources but also enhances the integration experience for your customers.
By following these best practices and leveraging tools like Endgrate, you can create efficient, secure, and scalable integrations with the Google Calendar API, ultimately delivering more value to your users.
Read More
- https://endgrate.com/provider/googlecalendar
- https://developers.google.com/calendar/api/v3/reference
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/calendar/api/v3/reference/events/get
Ready to get started?