Using the Wrike API to Get Tasks in Javascript
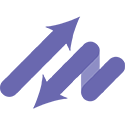
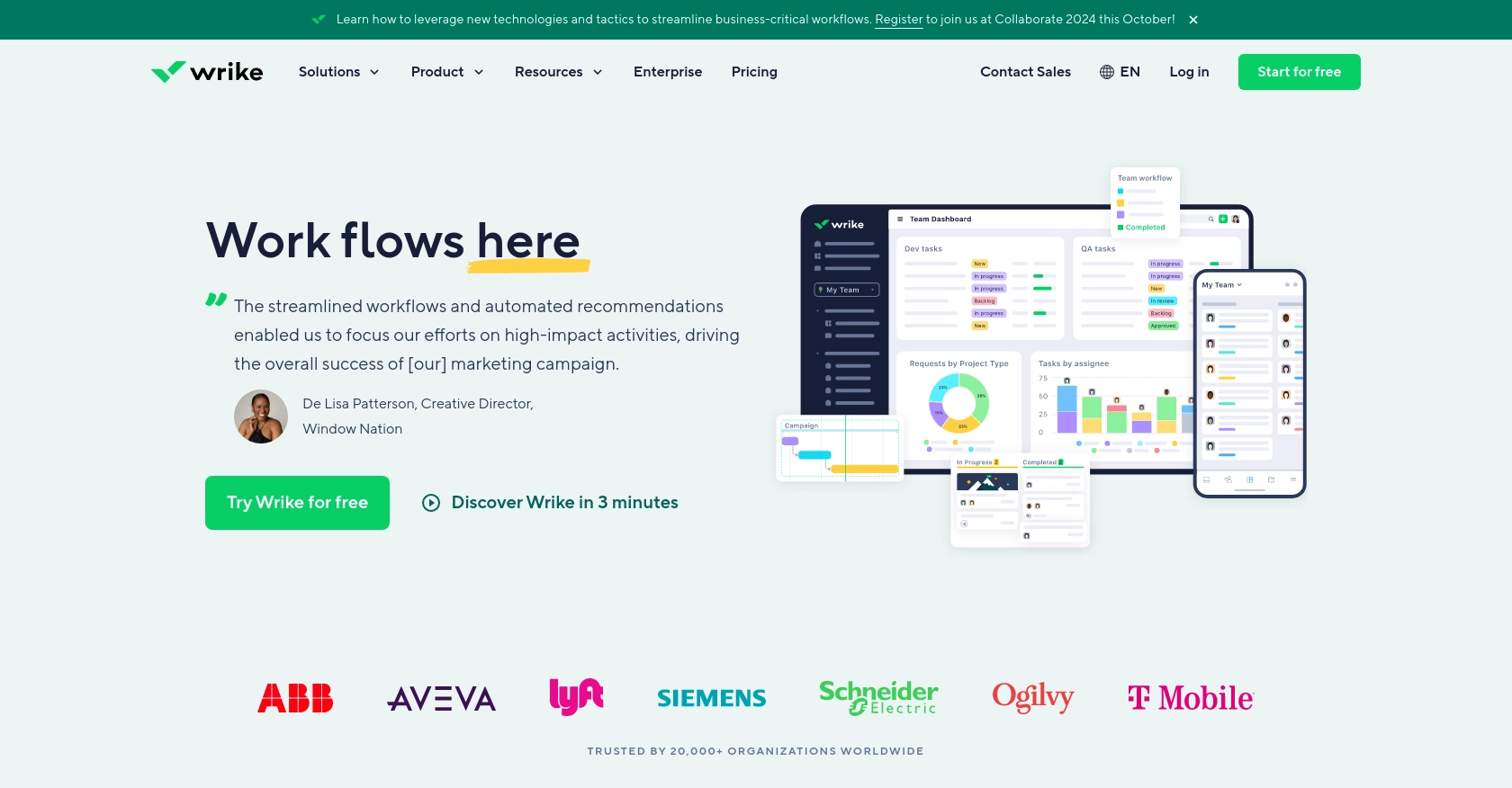
Introduction to Wrike API Integration
Wrike is a powerful project management and collaboration platform that helps teams streamline their workflows and enhance productivity. With features like task management, time tracking, and real-time collaboration, Wrike is a preferred choice for businesses looking to optimize their project management processes.
Integrating with the Wrike API allows developers to automate and customize their project management tasks. For example, you might want to retrieve tasks from Wrike to display them in a custom dashboard or to synchronize them with another application. This can enhance visibility and coordination across different tools and platforms.
Setting Up Your Wrike Test/Sandbox Account for API Integration
Before you can start interacting with the Wrike API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Wrike offers a free trial that you can use to explore its features and test your integrations.
Creating a Wrike Account
To begin, visit the Wrike website and sign up for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you will have access to the Wrike platform where you can manage tasks and projects.
Setting Up OAuth 2.0 for Wrike API Authentication
The Wrike API uses OAuth 2.0 for authentication, which provides a secure way to authorize API requests. Follow these steps to set up OAuth 2.0:
- Log in to your Wrike account and navigate to the Apps & Integrations section.
- Click on Create New App to start setting up your application.
- Fill in the required details such as the app name and description.
- Specify the redirect URI, which is the URL to which users will be redirected after they authorize your app.
- Once your app is created, you will receive a Client ID and Client Secret. Keep these credentials secure as they are required for API authentication.
Generating Access Tokens for Wrike API
With your app set up, you can now generate access tokens to authenticate API requests:
- Direct users to the Wrike authorization URL, including your Client ID and redirect URI as parameters.
- After users authorize the app, they will be redirected to your specified URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Wrike token endpoint, including your Client ID, Client Secret, and the authorization code.
- Store the access token securely, as it will be used to authenticate your API requests.
For more detailed information on OAuth 2.0 setup, refer to the Wrike OAuth 2.0 documentation.
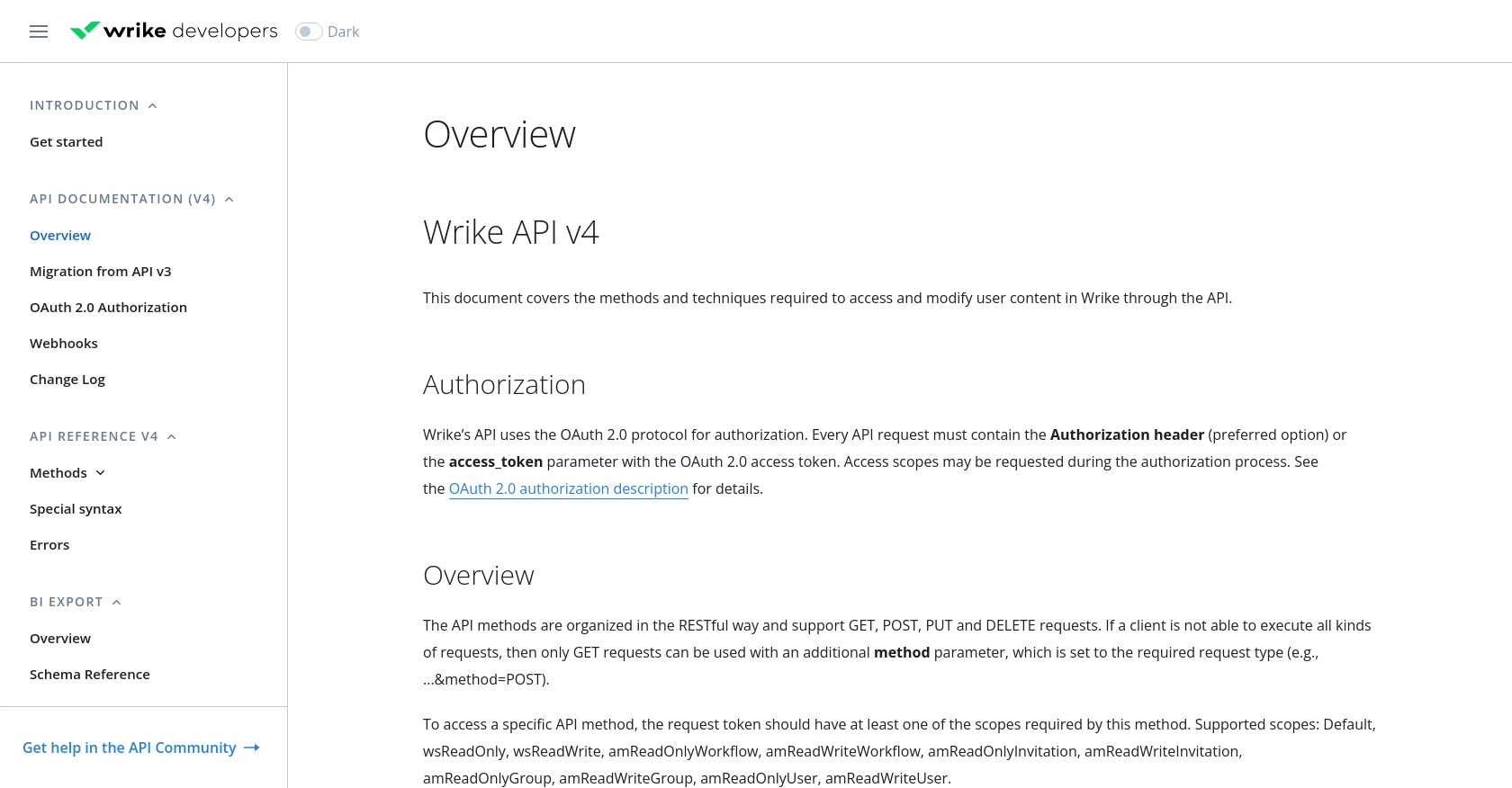
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Wrike Using JavaScript
To interact with the Wrike API and retrieve tasks using JavaScript, you'll need to ensure you have the right setup and dependencies. This section will guide you through the process of making API calls, handling responses, and managing errors effectively.
Setting Up Your JavaScript Environment for Wrike API
Before you begin, make sure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside of a browser. You can download it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the necessary dependencies. For this tutorial, we'll use the axios
library to make HTTP requests. Run the following command in your terminal to install it:
npm install axios
Writing JavaScript Code to Fetch Tasks from Wrike
Now that your environment is set up, you can write the JavaScript code to fetch tasks from Wrike. Create a new file named getWrikeTasks.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://www.wrike.com/api/v4/tasks';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to fetch tasks
async function fetchTasks() {
try {
const response = await axios.get(endpoint, { headers });
const tasks = response.data.data;
// Display the tasks
tasks.forEach(task => {
console.log(`Task ID: ${task.id}, Title: ${task.title}`);
});
} catch (error) {
console.error('Error fetching tasks:', error.response ? error.response.data : error.message);
}
}
// Call the function
fetchTasks();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth 2.0 setup. This code uses the axios
library to send a GET request to the Wrike API endpoint for tasks. It then processes the response to display task IDs and titles.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node getWrikeTasks.js
If the request is successful, you should see a list of task IDs and titles printed in the console. This confirms that the API call was made correctly and the tasks were retrieved from Wrike.
Handling Errors and Wrike API Error Codes
When making API calls, it's important to handle potential errors gracefully. The Wrike API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. You can find more details on error codes in the Wrike API documentation.
In the code above, errors are caught in the catch
block, and the error message or response data is logged to the console. This helps in diagnosing issues and ensuring robust error handling in your application.
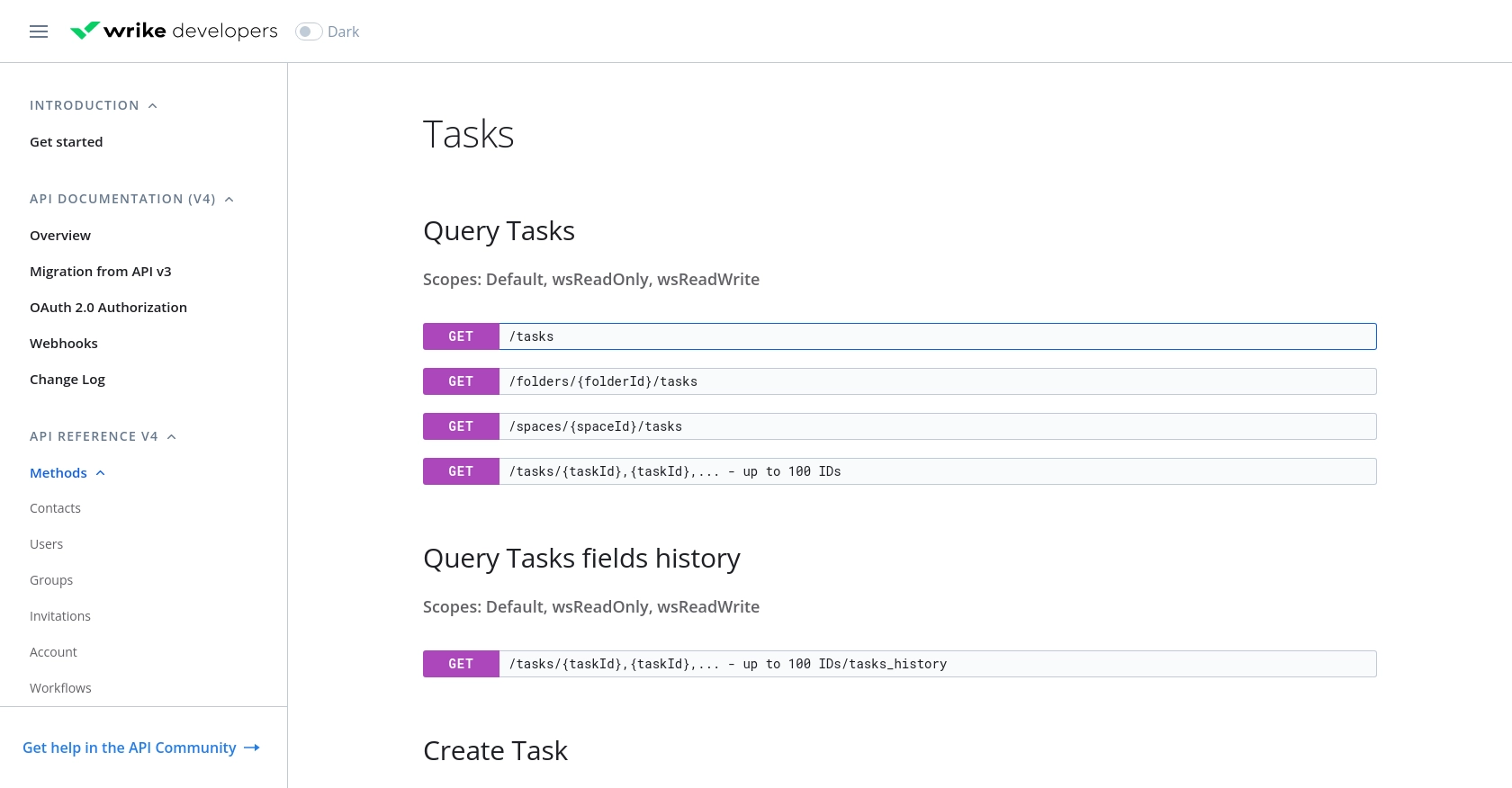
Conclusion and Best Practices for Wrike API Integration
Integrating with the Wrike API using JavaScript can significantly enhance your project management capabilities by automating task retrieval and synchronization. By following the steps outlined in this article, you can efficiently set up OAuth 2.0 authentication, make API calls, and handle potential errors.
Best Practices for Secure and Efficient Wrike API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handling Rate Limits: Be mindful of Wrike's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully. For more details, refer to the Wrike API documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with other systems and applications you integrate with.
Enhancing Your Integration Strategy with Endgrate
While building integrations with the Wrike API can be rewarding, it may also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a streamlined solution by providing a unified API endpoint for various integrations, including Wrike. This allows you to focus on your core product while outsourcing the complexities of integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
Ready to get started?