How to Get Companies with the Apollo API in Javascript
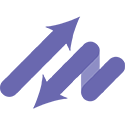
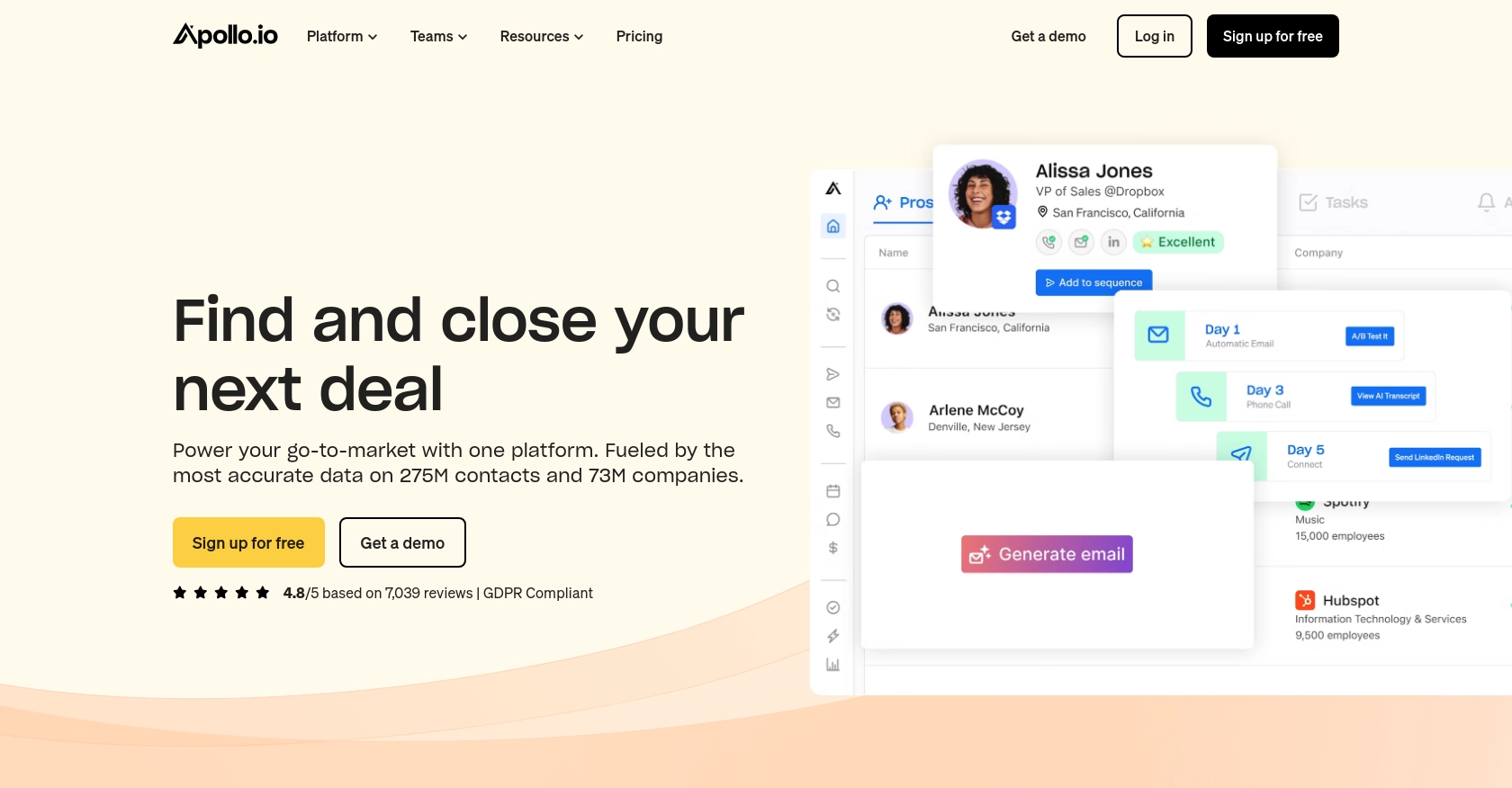
Introduction to Apollo API for Company Data Retrieval
Apollo is a comprehensive sales intelligence and engagement platform that empowers businesses to discover and connect with potential leads. With a vast database of over 200 million contacts and 70 million companies, Apollo provides tools for sales teams to enhance their outreach strategies and improve conversion rates.
Developers may want to integrate with the Apollo API to access detailed company information, which can be crucial for lead generation and market analysis. For example, a developer might use the Apollo API to retrieve company data and integrate it into a CRM system, enabling sales teams to have up-to-date information on potential clients.
This article will guide you through the process of using JavaScript to interact with the Apollo API, specifically focusing on retrieving company data. By following this tutorial, you'll learn how to efficiently access and manage company information using the Apollo platform.
Setting Up Your Apollo API Test Account
Before you can start retrieving company data using the Apollo API, you need to set up a test account. This will allow you to experiment with the API in a controlled environment without affecting live data.
Create an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the API and allow you to explore its features.
- Visit the Apollo website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and password.
- Follow the instructions to complete the registration process.
Generate an Apollo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests. Apollo uses API key-based authentication, which is straightforward and secure.
- Log in to your Apollo account and navigate to the API settings.
- Find the section for API keys and click on "Generate New API Key."
- Copy the generated API key and store it securely, as you'll need it for making API requests.
Configure API Key for Authentication
With your API key ready, you can now configure it in your JavaScript code to authenticate your requests to the Apollo API.
// Example of setting up headers with API key
const headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
};
Replace YOUR_API_KEY_HERE
with the API key you generated earlier.
With these steps completed, you're now ready to start making requests to the Apollo API and retrieve company data. In the next section, we'll cover how to make these API calls using JavaScript.
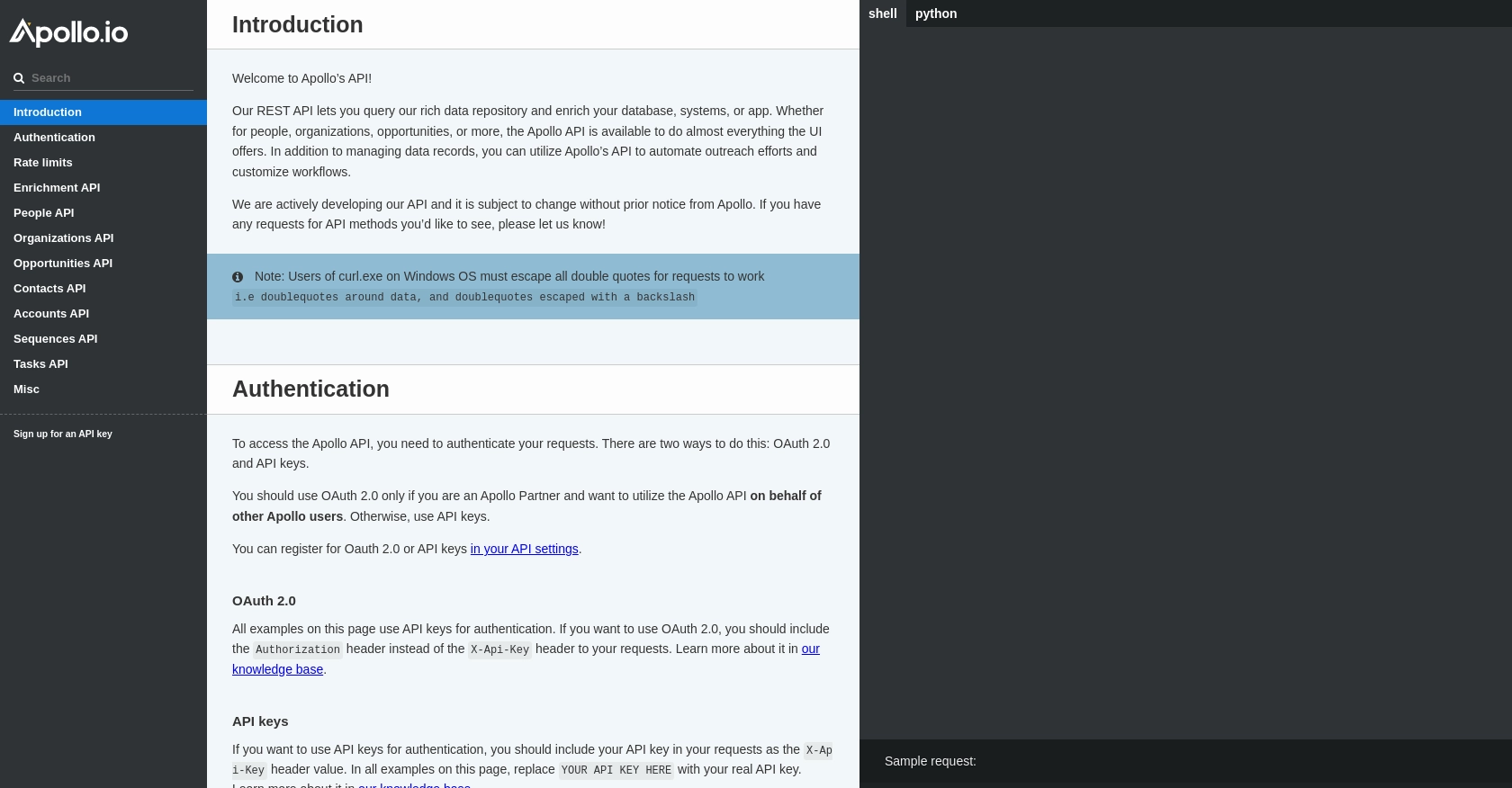
sbb-itb-96038d7
Making API Calls to Retrieve Company Data with Apollo API in JavaScript
To effectively interact with the Apollo API and retrieve company data, you'll need to set up your JavaScript environment and make HTTP requests using the API key you generated earlier. This section will guide you through the necessary steps to achieve this.
Setting Up Your JavaScript Environment for Apollo API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js, which requires you to have Node.js and npm installed on your machine.
- Ensure Node.js is installed by running
node -v
in your terminal. - Initialize a new Node.js project by running
npm init -y
. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Example Code for Making a GET Request to Apollo API
With your environment set up, you can now write JavaScript code to make a GET request to the Apollo API and retrieve company data. Below is an example of how to do this using the axios
library:
// Import the axios library
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.apollo.io/v1/organizations/enrich';
const headers = {
'Content-Type': 'application/json',
'Cache-Control': 'no-cache',
'X-Api-Key': 'YOUR_API_KEY_HERE'
};
// Define the query parameters
const params = {
domain: 'example.com' // Replace with the domain of the company you want to retrieve
};
// Make the GET request
axios.get(endpoint, { headers, params })
.then(response => {
console.log('Company Data:', response.data);
})
.catch(error => {
console.error('Error fetching company data:', error);
});
Replace YOUR_API_KEY_HERE
with your actual API key and example.com
with the domain of the company you wish to retrieve data for.
Understanding the Response and Handling Errors
Upon a successful request, the Apollo API will return detailed company information. You can verify the success of your request by checking the response data in the console. If the request fails, the error will be logged, and you can handle it accordingly.
Common error codes include:
- 401 Unauthorized: Check if your API key is correct.
- 404 Not Found: Ensure the domain parameter is valid.
- 429 Too Many Requests: You have exceeded the rate limit.
For more details on error codes, refer to the Apollo API documentation.
Verifying API Call Success in Apollo Test Account
To confirm that your API call was successful, you can cross-check the retrieved company data with the information available in your Apollo test account. This ensures that the data is accurate and up-to-date.
By following these steps, you can efficiently retrieve and manage company data using the Apollo API in JavaScript, enhancing your sales intelligence and engagement strategies.
Conclusion and Best Practices for Using Apollo API in JavaScript
Integrating with the Apollo API using JavaScript provides a powerful way to access and manage company data, enhancing your sales and marketing strategies. By following the steps outlined in this guide, you can efficiently retrieve company information and integrate it into your systems.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API key securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the rate limits imposed by Apollo. The API allows up to 50 requests per minute. Implement logic to handle
429 Too Many Requests
errors by retrying after a delay. - Data Transformation: Standardize and transform the retrieved data to fit your application's needs, ensuring consistency across your systems.
Enhancing Integration Capabilities with Endgrate
While integrating with Apollo API is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Apollo.
By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case, reducing redundancy across different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
Ready to get started?