Using the ButterflyMX API to Get Devices in Python
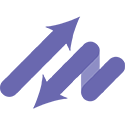
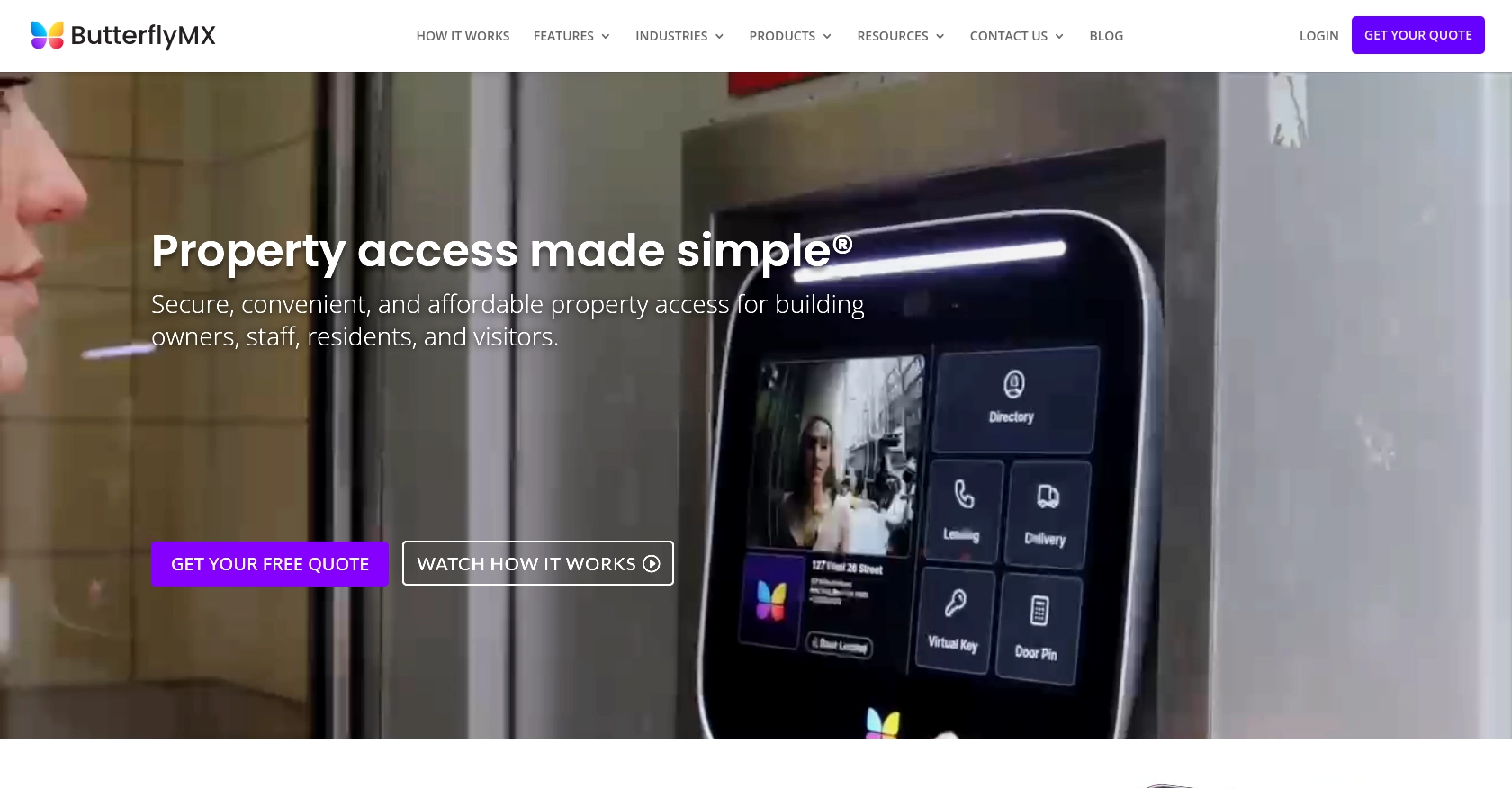
Introduction to ButterflyMX API
ButterflyMX is a cutting-edge property access solution that simplifies building entry for residents, visitors, and property staff. With its innovative intercom system, ButterflyMX enhances security and convenience in residential and commercial properties.
Developers may want to integrate with the ButterflyMX API to access and manage device data, such as door intercoms and access panels. For example, a developer might use the ButterflyMX API to retrieve a list of devices in a building to monitor and manage access points efficiently.
Setting Up a ButterflyMX Sandbox Account for API Integration
To begin integrating with the ButterflyMX API, you'll need access to a sandbox account. This environment allows you to test API interactions without affecting live data. Unfortunately, creating a sandbox account isn't as straightforward as signing up online. You'll need to contact ButterflyMX directly to request access to a sandbox account.
Contacting ButterflyMX for Sandbox Access
Follow these steps to request a sandbox account:
- Visit the ButterflyMX API documentation page for contact information.
- Reach out to their support or sales team, specifying your need for a sandbox environment for API testing.
- Provide any necessary details about your project to expedite the process.
Creating a ButterflyMX App for OAuth2 Authentication
Once you have access to a sandbox account, the next step is to set up OAuth2 authentication. This involves creating an app within the ButterflyMX platform to obtain the necessary credentials.
- Log in to your ButterflyMX sandbox account.
- Navigate to the app creation section under the developer settings.
- Create a new app and note down the Client ID and Client Secret.
- Set the redirect URI to:
https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx
.
Obtaining an Access Token
With your app created, you can now obtain an access token to authenticate API requests:
import requests
# Define the token endpoint and payload
token_url = "https://accountssandbox.butterflymx.com/oauth/token"
payload = {
'grant_type': 'authorization_code',
'code': 'YOUR_AUTHORIZATION_CODE',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET',
'redirect_uri': 'https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx'
}
# Request the access token
response = requests.post(token_url, data=payload)
tokens = response.json()
print(tokens)
Replace YOUR_AUTHORIZATION_CODE
, YOUR_CLIENT_ID
, and YOUR_CLIENT_SECRET
with the actual values obtained during the app setup.
Handling Access Token Renewal
Access tokens are temporary and will expire. To renew an access token, use the refresh token provided in the initial response:
refresh_payload = {
'grant_type': 'refresh_token',
'refresh_token': 'YOUR_REFRESH_TOKEN',
'client_id': 'YOUR_CLIENT_ID',
'client_secret': 'YOUR_CLIENT_SECRET'
}
# Request a new access token
refresh_response = requests.post(token_url, data=refresh_payload)
new_tokens = refresh_response.json()
print(new_tokens)
Ensure you store the new access and refresh tokens securely after renewal.
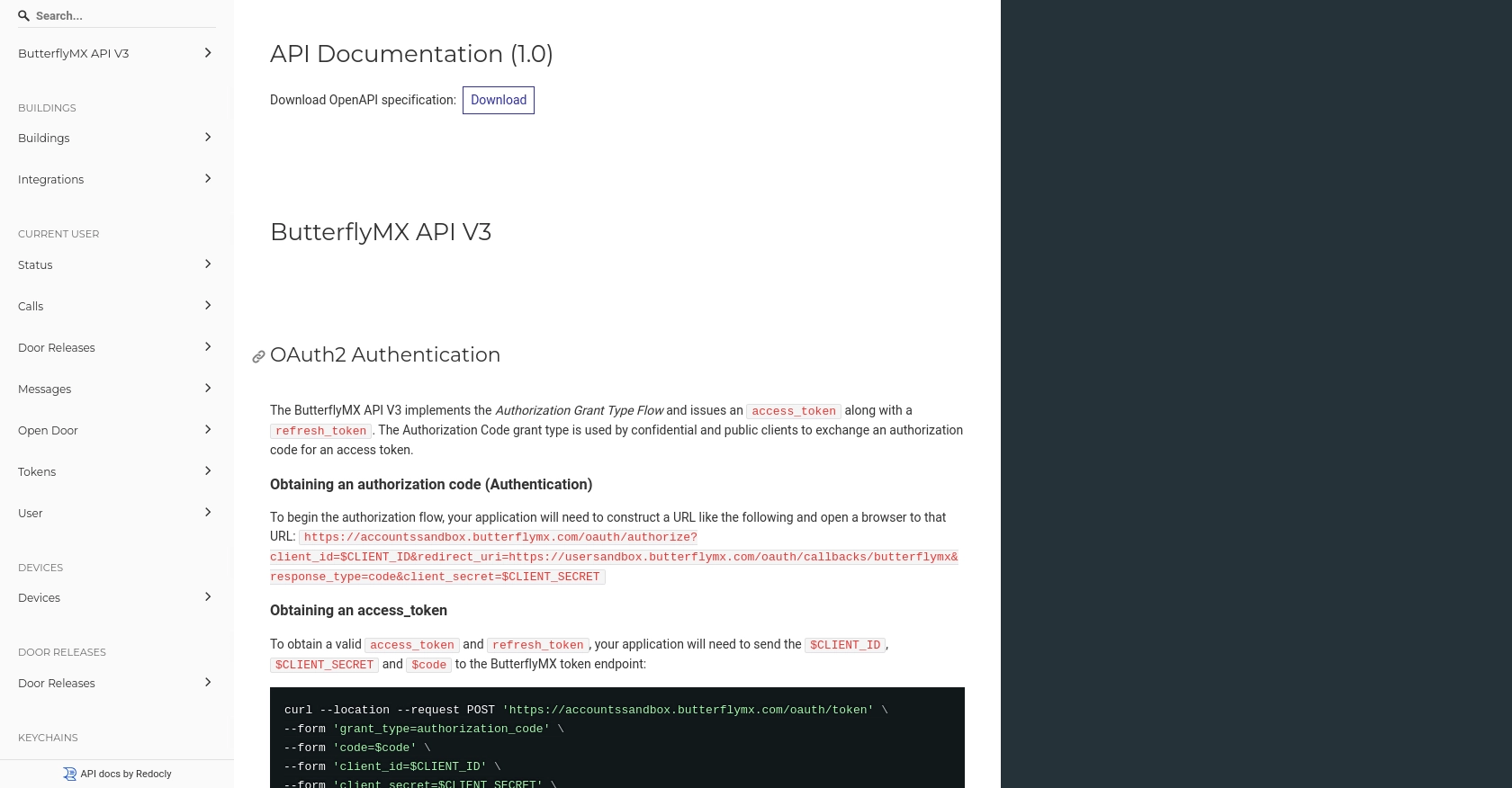
sbb-itb-96038d7
Making API Calls to Retrieve Devices Using ButterflyMX API in Python
To interact with the ButterflyMX API and retrieve device information, you'll need to use Python. This section will guide you through the steps to make API calls, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for ButterflyMX API Integration
Before you begin, ensure you have Python 3.11.1 installed on your machine. You'll also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Retrieve Devices from ButterflyMX API
Once your environment is ready, you can write the Python script to fetch devices. Create a file named get_devices.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://apisandbox.butterflymx.com/v3/devices"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/vnd.api+json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
devices = response.json()
print(devices)
else:
print(f"Failed to retrieve devices: {response.status_code}")
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the authentication process.
Verifying Successful API Requests and Handling Errors
After running your script, you should see a list of devices printed in the console if the request is successful. You can verify the data by checking the sandbox account for consistency.
If the request fails, the script will print an error message with the status code. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and correctly included in the headers.
- 404 Not Found: Ensure the endpoint URL is correct.
- 500 Internal Server Error: This may indicate an issue with the ButterflyMX server. Try again later.
For more detailed error handling, refer to the ButterflyMX API documentation.
Conclusion and Best Practices for Using ButterflyMX API in Python
Integrating with the ButterflyMX API can significantly enhance the management of property access systems, providing developers with the tools to efficiently monitor and control devices like intercoms and access panels. By following the steps outlined in this guide, you can successfully authenticate and retrieve device data using Python.
Best Practices for Secure and Efficient API Integration with ButterflyMX
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and potential issues with API requests.
Streamlining Integrations with Endgrate
For developers looking to simplify the integration process across multiple platforms, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations and focusing on your core product. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers.
Visit Endgrate to learn more about how it can help streamline your integration efforts with platforms like ButterflyMX and beyond.
Read More
Ready to get started?