How to Create Pin with the ButterflyMX API in Javascript
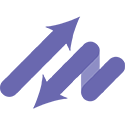
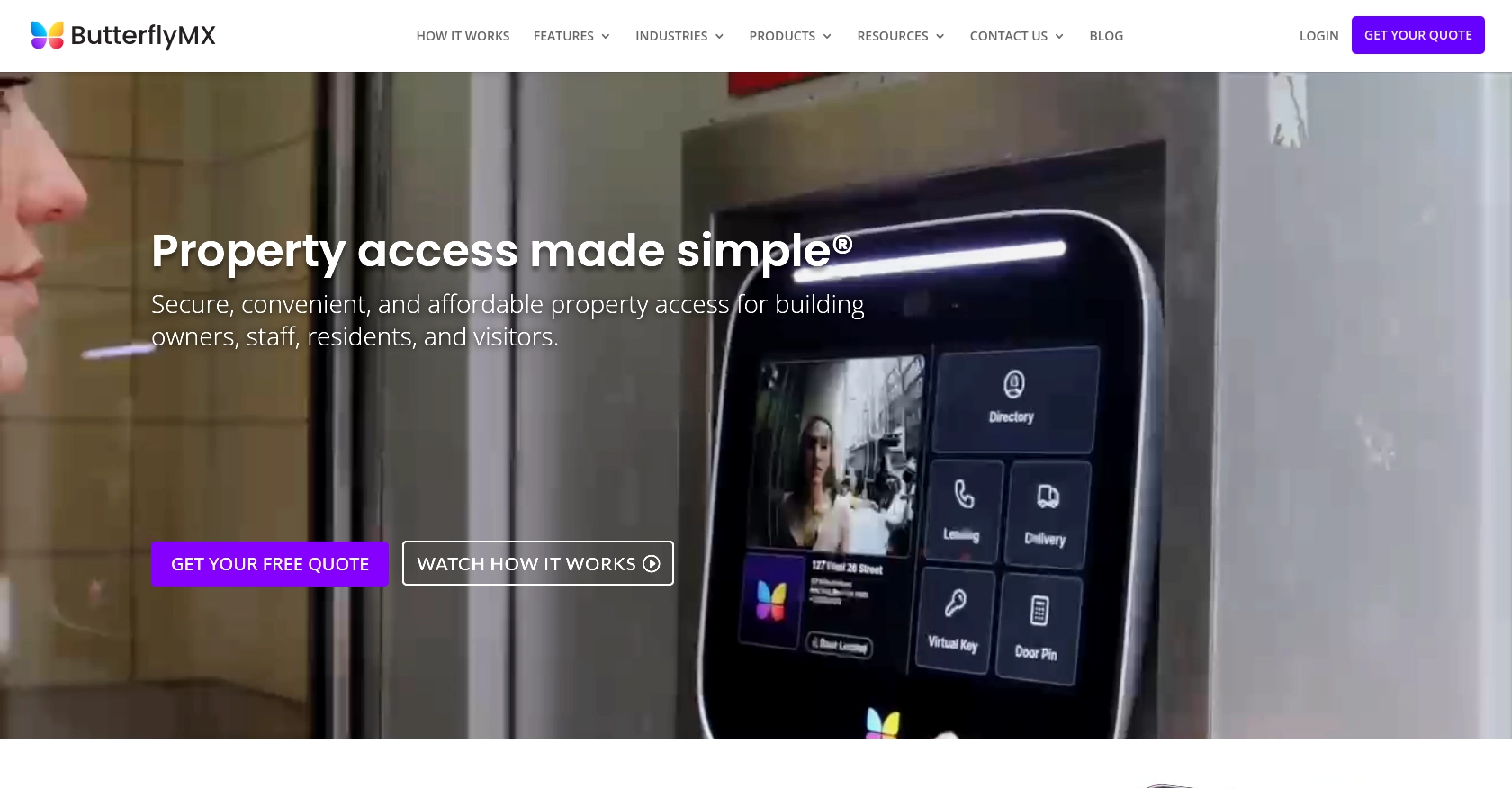
Introduction to ButterflyMX API
ButterflyMX is a cutting-edge property access solution that simplifies building entry for residents, visitors, and property staff. It offers a seamless experience through its smart intercom system, which integrates with various building management systems to enhance security and convenience.
Developers may want to integrate with the ButterflyMX API to automate and manage access control features, such as creating and managing PINs for tenants. For example, a developer could use the ButterflyMX API to generate unique PINs for new tenants, streamlining the onboarding process and enhancing security measures.
Setting Up Your ButterflyMX Sandbox Account
Before you can start integrating with the ButterflyMX API, you'll need to set up a sandbox account. This environment allows developers to test and experiment with API calls without affecting live data.
Contacting ButterflyMX for Sandbox Access
To obtain a sandbox account, you must contact ButterflyMX directly. Unfortunately, you cannot create a sandbox account directly through their website. Reach out to their support team or sales representatives to request access to a sandbox environment.
Creating a ButterflyMX App for OAuth2 Authentication
Once you have access to the sandbox account, you'll need to create an app to use OAuth2 authentication. Follow these steps to set up your app:
- Log in to your ButterflyMX sandbox account.
- Navigate to the app creation section in the developer portal.
- Provide the necessary details for your app, such as the app name and description.
- Set the redirect URI to:
https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx
. - Save your app to generate the
client_id
andclient_secret
.
Obtaining an Authorization Code
To begin the OAuth2 flow, construct a URL to obtain an authorization code:
const authUrl = `https://accountssandbox.butterflymx.com/oauth/authorize?client_id=${CLIENT_ID}&redirect_uri=https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx&response_type=code&client_secret=${CLIENT_SECRET}`;
Open this URL in a browser to authorize your app and receive an authorization code.
Exchanging the Authorization Code for Access Tokens
Use the authorization code to request an access token and refresh token:
const tokenRequest = {
method: 'POST',
body: new URLSearchParams({
grant_type: 'authorization_code',
code: AUTH_CODE,
client_id: CLIENT_ID,
client_secret: CLIENT_SECRET,
redirect_uri: 'https://usersandbox.butterflymx.com/oauth/callbacks/butterflymx'
})
};
fetch('https://accountssandbox.butterflymx.com/oauth/token', tokenRequest)
.then(response => response.json())
.then(data => {
console.log('Access Token:', data.access_token);
console.log('Refresh Token:', data.refresh_token);
})
.catch(error => console.error('Error:', error));
Store the access_token
and refresh_token
securely for future API calls.
With your sandbox account and app set up, you're ready to start interacting with the ButterflyMX API using JavaScript.
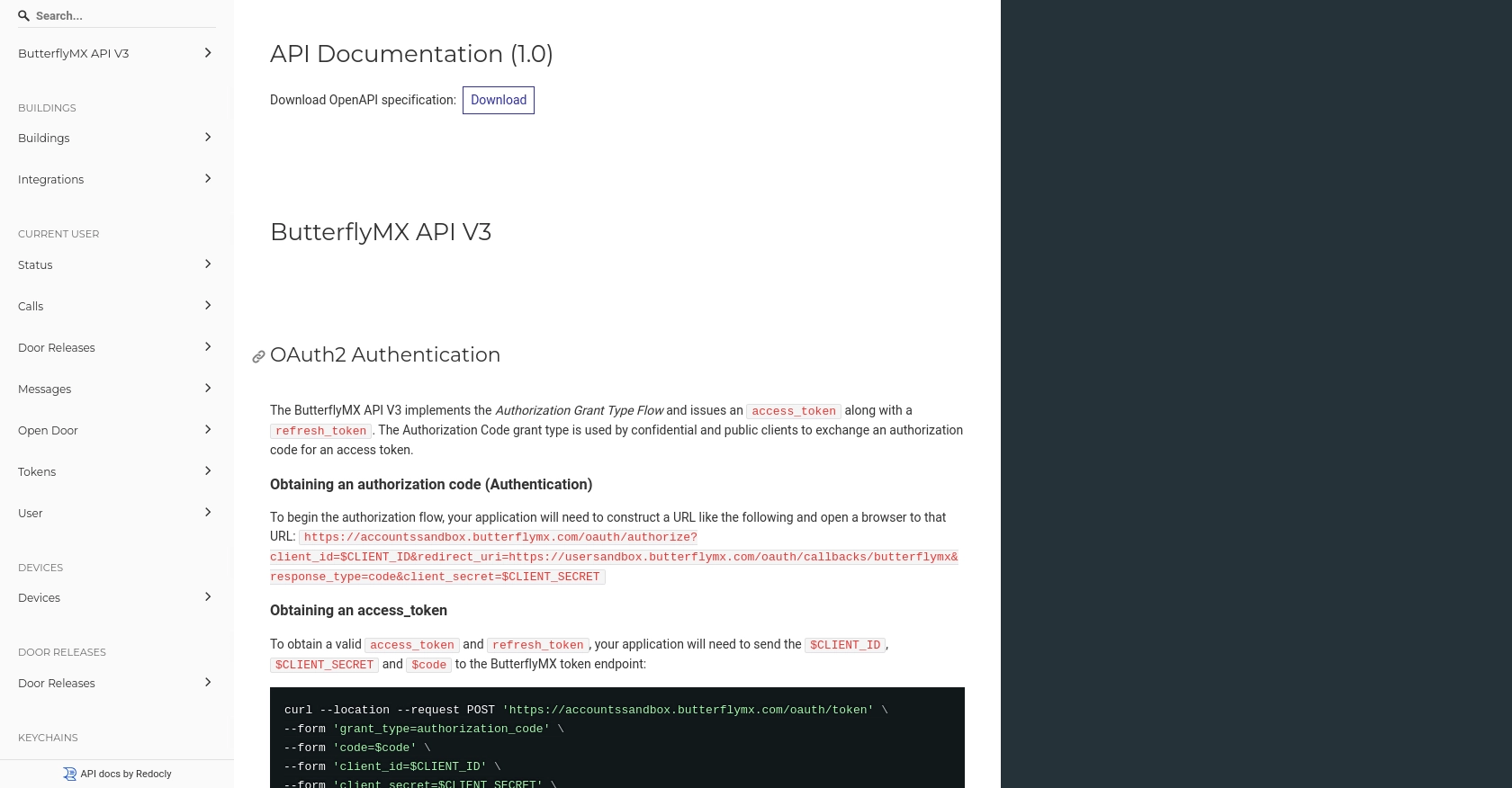
sbb-itb-96038d7
Making API Calls to Create a Pin with ButterflyMX API Using JavaScript
Now that you have set up your ButterflyMX sandbox account and obtained the necessary access tokens, you can proceed to make API calls to create a PIN for a tenant. This section will guide you through the process using JavaScript.
Prerequisites for Using JavaScript with ButterflyMX API
Before making API calls, ensure you have the following installed on your machine:
- Node.js (latest version recommended)
- npm (Node Package Manager)
Additionally, you will need to install the node-fetch
package to make HTTP requests:
npm install node-fetch
Creating a PIN for a Tenant Using ButterflyMX API
To create a PIN for a tenant, you will need to make a POST request to the ButterflyMX API. Below is a sample code snippet demonstrating how to achieve this:
const fetch = require('node-fetch');
const createPin = async (tenantId, pinCode) => {
const url = 'https://apisandbox.butterflymx.com/v3/pins';
const accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your actual access token
const requestBody = {
data: {
type: 'pins',
id: tenantId,
attributes: {
code: pinCode
}
}
};
const response = await fetch(url, {
method: 'PUT',
headers: {
'Content-Type': 'application/vnd.api+json',
'Authorization': `Bearer ${accessToken}`
},
body: JSON.stringify(requestBody)
});
if (response.ok) {
console.log('PIN created successfully');
} else {
console.error('Failed to create PIN', await response.json());
}
};
// Example usage
createPin('990619300', '1234');
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier, and provide the appropriate tenantId
and pinCode
.
Verifying the API Call Success in ButterflyMX Sandbox
After running the code, you can verify the successful creation of the PIN by checking the tenant's details in your ButterflyMX sandbox account. The newly created PIN should be listed under the tenant's information.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. The ButterflyMX API may return various error codes, such as:
- 400 Bad Request: Indicates that the request was malformed or missing required parameters.
- 401 Unauthorized: Occurs if the access token is invalid or expired.
- 404 Not Found: The specified tenant ID does not exist.
Ensure your application gracefully handles these errors by checking the response status and providing meaningful feedback to the user.
Best Practices for Using ButterflyMX API in JavaScript
When working with the ButterflyMX API, it's essential to follow best practices to ensure security, efficiency, and maintainability of your integration. Here are some recommendations:
- Securely Store Access Tokens: Always store your access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
Enhance Your Integration Experience with Endgrate
Integrating multiple platforms can be time-consuming and complex. With Endgrate, you can streamline your integration process by leveraging a single API endpoint that connects to various platforms, including ButterflyMX. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can simplify your integration process and enhance your product's capabilities.
Read More
Ready to get started?