Using the Typeform API to Get Responses in Python
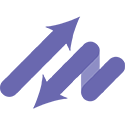
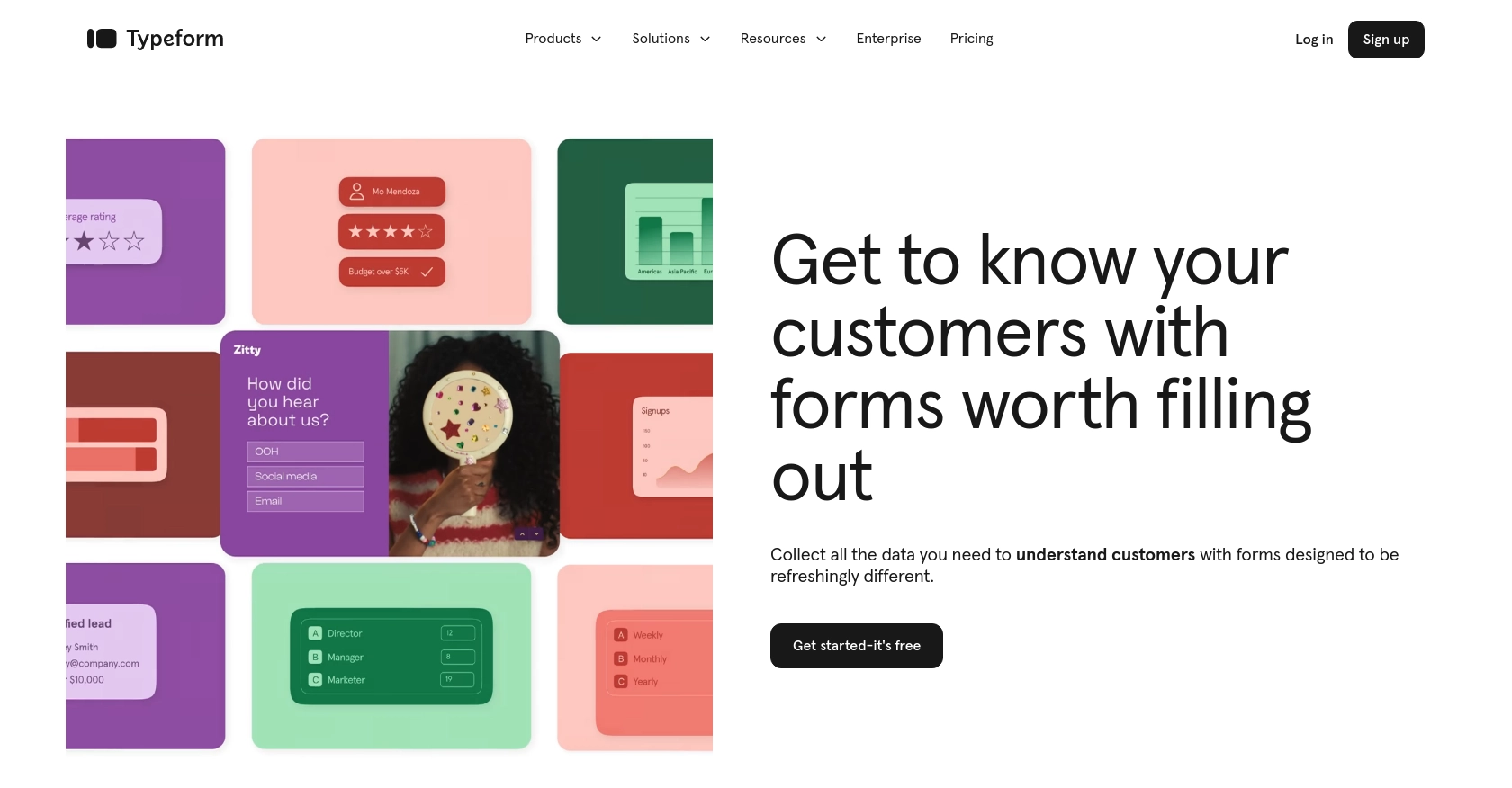
Introduction to Typeform API Integration
Typeform is a versatile online form builder that allows businesses to create engaging and interactive forms, surveys, and quizzes. Its user-friendly interface and customizable templates make it a popular choice for collecting data and feedback from users.
Integrating with Typeform's API enables developers to automate data retrieval and streamline workflows. For example, you can use the Typeform API to fetch survey responses and analyze them in real-time, enhancing decision-making processes and improving customer engagement strategies.
Setting Up Your Typeform Developer Account for API Integration
Before you can start using the Typeform API to retrieve responses, you'll need to set up a developer account and create an application. This process involves registering your application with Typeform and obtaining the necessary credentials for OAuth 2.0 authentication.
Step-by-Step Guide to Creating a Typeform Developer Account
- Sign Up or Log In: If you don't already have a Typeform account, visit the Typeform website and sign up for a free account. If you already have an account, simply log in.
- Access Developer Settings: In the upper-left corner, click the icon drop-down menu next to your organization name. Under the Organization section, click on Developer Apps.
- Register a New App: Click on Register a new app. Fill in the required fields, including the App Name, App Website, and Redirect URI(s). The Redirect URI is where users will be redirected after they log in and grant access.
- Save Your Credentials: After registering your app, you'll receive a
client_id
and aclient_secret
. Make sure to copy and store these credentials securely, as you'll need them to authenticate API requests.
Configuring OAuth 2.0 for Typeform API Access
Typeform uses OAuth 2.0 to secure its API endpoints. You'll need to follow these steps to authenticate your application:
- Authorization Request: Direct users to the following URL to obtain an authorization code:
Replacehttps://api.typeform.com/oauth/authorize?client_id={your_client_id}&redirect_uri={your_redirect_uri}&scope=responses:read
{your_client_id}
and{your_redirect_uri}
with your actual client ID and redirect URI. - Exchange Authorization Code for Access Token: Once you have the authorization code, exchange it for an access token by making a POST request to:
Replace placeholders with your actual values.import requests url = "https://api.typeform.com/oauth/token" data = { 'grant_type': 'authorization_code', 'code': '{authorization_code}', 'client_id': '{your_client_id}', 'client_secret': '{your_client_secret}', 'redirect_uri': '{your_redirect_uri}' } response = requests.post(url, data=data) access_token = response.json().get('access_token')
For more details on OAuth 2.0 authentication with Typeform, refer to the official Typeform documentation.
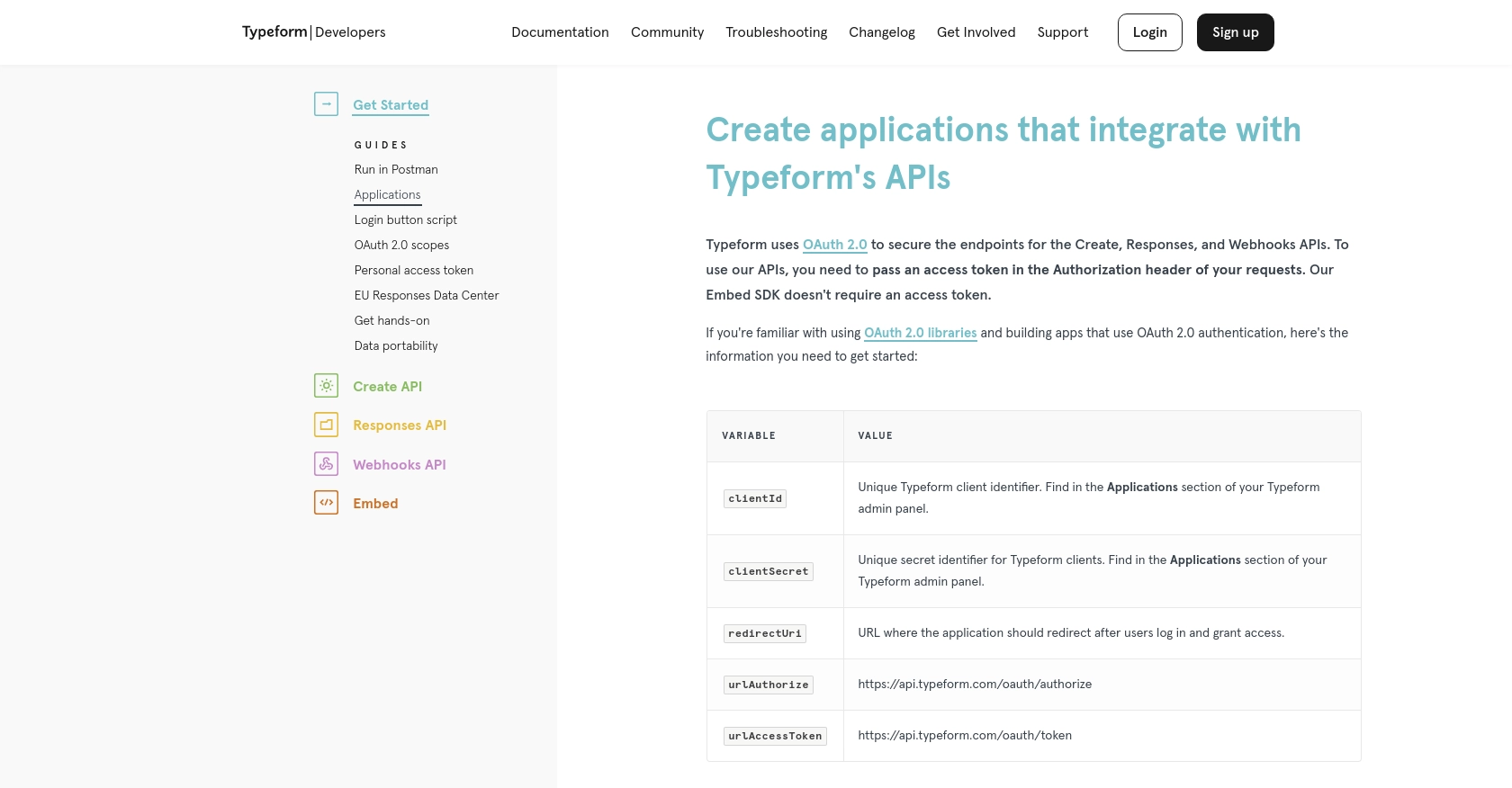
sbb-itb-96038d7
Making API Calls to Retrieve Typeform Responses Using Python
Once you have set up your Typeform developer account and configured OAuth 2.0 authentication, you can proceed to make API calls to retrieve responses from your Typeform forms. This section will guide you through the process of using Python to interact with the Typeform API and fetch form responses.
Prerequisites for Using Python with Typeform API
Before you begin, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Install the required requests
library by running the following command in your terminal:
pip install requests
Fetching Typeform Responses with Python
To retrieve responses from a Typeform form, you'll need to make a GET request to the Typeform API endpoint. Follow these steps to create a Python script that accomplishes this task:
- Create a Python Script: Open your preferred code editor and create a new file named
get_typeform_responses.py
. - Write the Code: Add the following code to your script:
import requests
# Replace with your actual form ID and access token
form_id = "your_form_id"
access_token = "your_access_token"
# Set the API endpoint and headers
url = f"https://api.typeform.com/forms/{form_id}/responses"
headers = {
"Authorization": f"Bearer {access_token}"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for item in data.get("items", []):
print(item)
else:
print(f"Failed to retrieve responses: {response.status_code} - {response.text}")
Replace your_form_id
and your_access_token
with your actual form ID and access token obtained during the OAuth 2.0 setup.
Running the Python Script and Handling Errors
To execute the script, run the following command in your terminal:
python get_typeform_responses.py
If successful, the script will print the responses from your Typeform form. In case of errors, the script will output the error code and message. Common error codes include:
- 400 Bad Request: Indicates a malformed request. Check your request parameters.
- 401 Unauthorized: Indicates invalid or missing authentication credentials. Verify your access token.
- 500 Internal Server Error: Indicates a server-side error. Try again later.
For more details on error handling, refer to the Typeform API documentation.
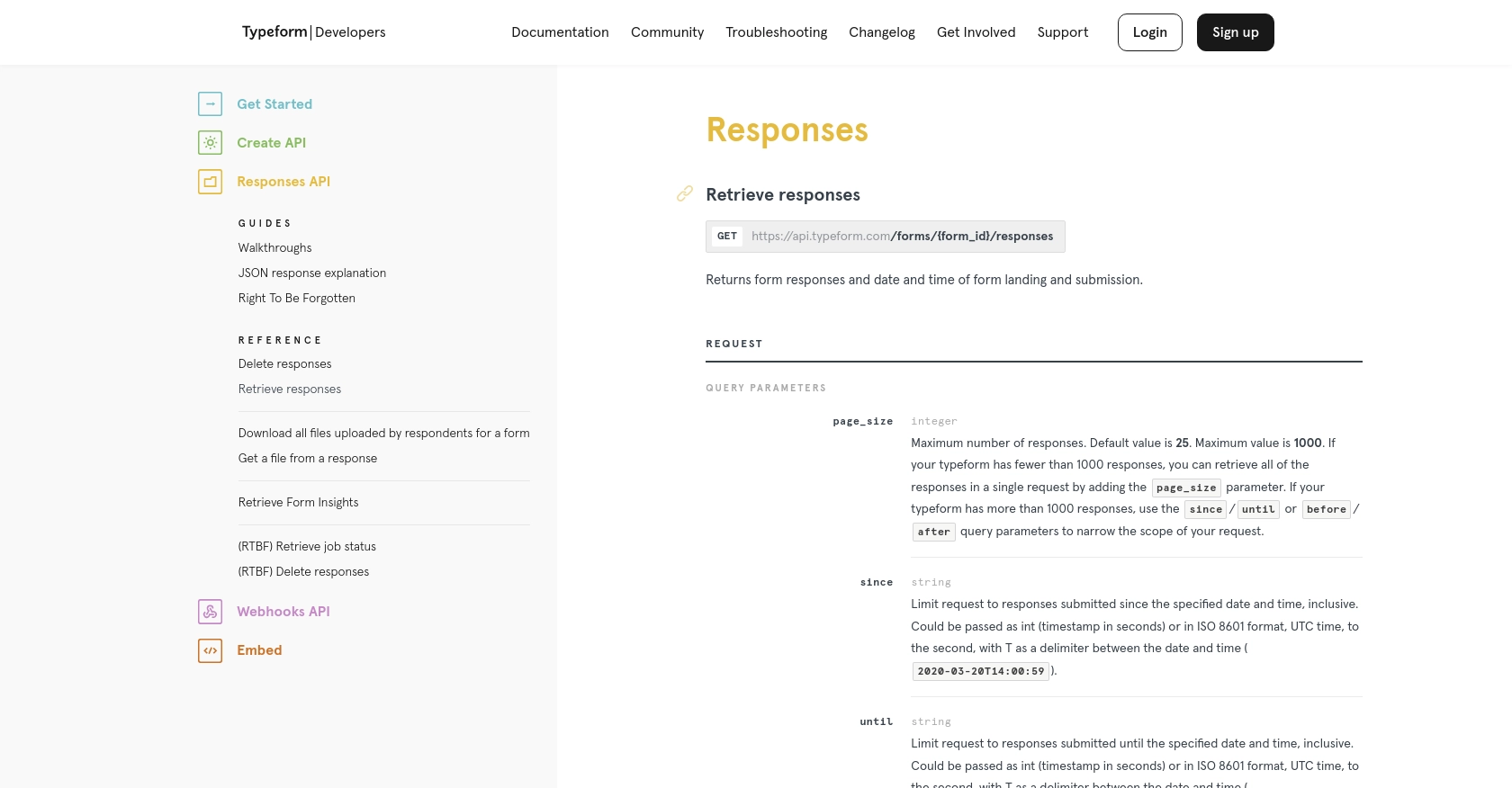
Conclusion and Best Practices for Using Typeform API with Python
Integrating Typeform's API into your applications can significantly enhance your data collection and analysis capabilities. By automating the retrieval of form responses, you can streamline workflows and improve decision-making processes.
Best Practices for Secure and Efficient Typeform API Integration
- Secure Storage of Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults. - Handle Rate Limiting: Be mindful of Typeform's API rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from Typeform is standardized and transformed as needed to fit your application's requirements.
Enhancing Integration Efficiency with Endgrate
While integrating with Typeform's API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Typeform. This allows you to build once for each use case and streamline your integration efforts.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
Ready to get started?