How to Import Data from CSV with the CSV API in PHP
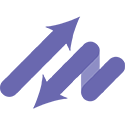
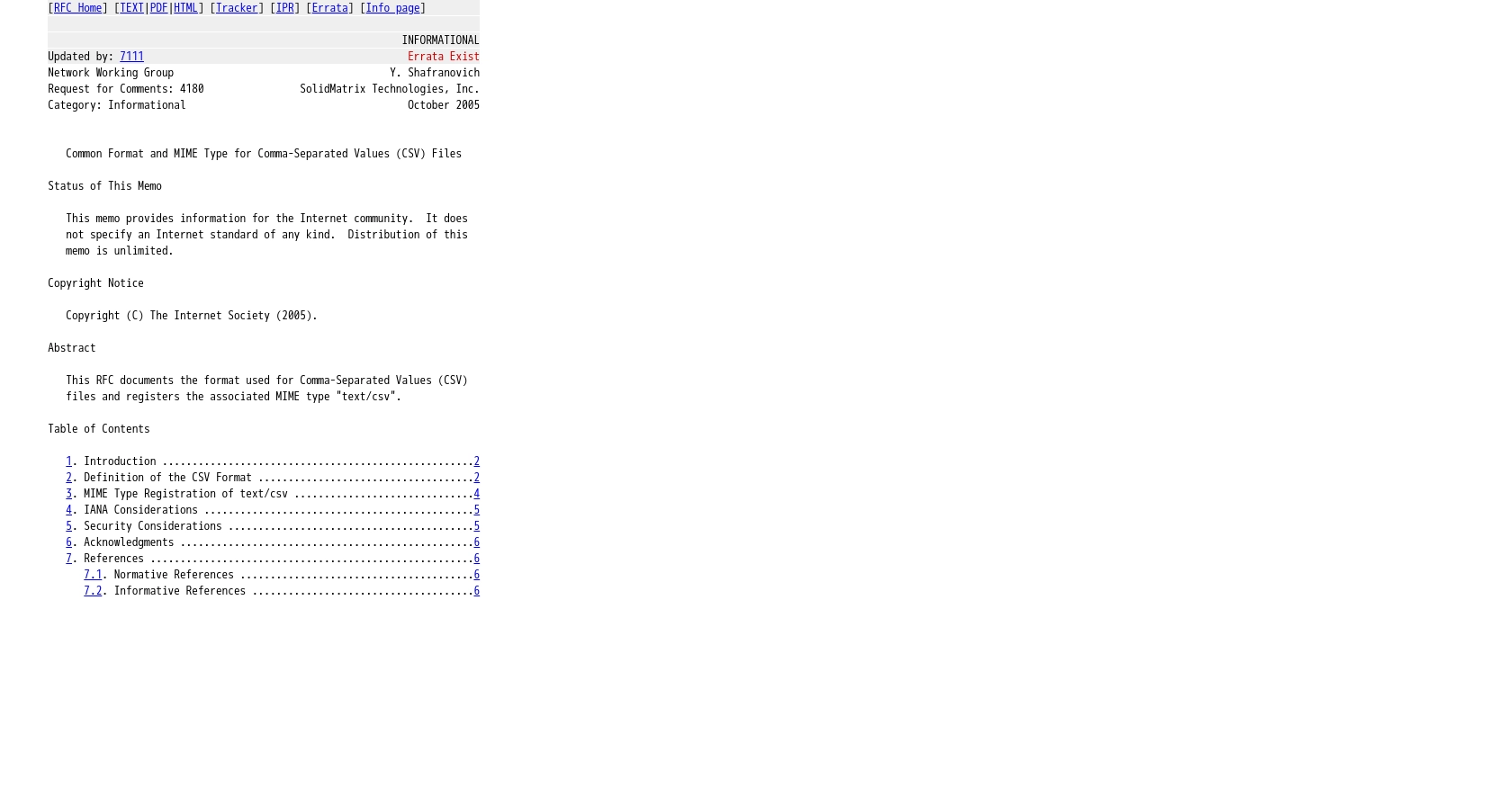
Introduction to CSV Integration
CSV (Comma-Separated Values) is a widely used format for data exchange, allowing developers to import and export data across various applications. Its simplicity and compatibility make it an essential tool for software developers working with data-driven applications.
Integrating with a CSV API can significantly enhance a developer's ability to manage data efficiently. For example, a developer might want to import user data from a CSV file into a web application, enabling seamless data migration and integration with existing systems.
This article will guide you through the process of importing data from CSV files using the CSV API in PHP, providing detailed steps to ensure a smooth integration experience.
Setting Up Your CSV Test Environment
Before you begin importing data using the CSV API in PHP, it's essential to set up a test environment. This will allow you to safely experiment with the integration without affecting live data.
Create a CSV Test Account
To start, you need to create a CSV test account. This account will serve as your sandbox environment, enabling you to upload and manipulate CSV files without impacting your production data.
- Visit the CSV integration provider's website and sign up for a free trial or demo account.
- Follow the registration process, providing the necessary information to create your test account.
- Once your account is set up, log in to access the sandbox environment.
Generate CSV API Credentials
With your test account ready, the next step is to generate the necessary API credentials. These credentials will allow your PHP application to authenticate and interact with the CSV API.
- Navigate to the API section of your test account dashboard.
- Locate the option to create new API credentials.
- Generate a client ID and client secret, which you will use in your PHP code to authenticate API requests.
- Store these credentials securely, as they are essential for accessing the CSV API.
Upload Sample CSV Files
To fully utilize the test environment, upload sample CSV files that you can use for testing the import functionality.
- Prepare a few CSV files with sample data that you wish to import.
- Use the CSV upload feature in your test account to upload these files.
- Ensure that the CSV files are formatted correctly and contain the necessary headers and data.
Understanding CSV Authentication
The CSV integration uses a custom authentication method, leveraging CSV libraries to load data into the database. This approach requires a unique frontend for handling CSV uploads and downloads.
Ensure that your PHP application is equipped to handle this custom authentication process, allowing seamless interaction with the CSV API.
Making API Calls to Import CSV Data Using PHP
To effectively import data from CSV files using the CSV API in PHP, you'll need to ensure your development environment is properly configured. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to interact with the CSV API.
Setting Up Your PHP Environment for CSV API Integration
Before making API calls, ensure that your PHP environment is ready. You'll need PHP installed on your machine, along with any required libraries for handling CSV data.
- Ensure PHP is installed on your system. You can download it from the official PHP website.
- Install necessary PHP extensions for handling CSV files, such as
php-curl
for making HTTP requests. - Verify your PHP installation by running
php -v
in your terminal.
Writing PHP Code to Import CSV Data
With your environment set up, you can now write the PHP code to import CSV data using the CSV API. This involves reading the CSV file, preparing the data, and making an API call to upload the data.
<?php
// Define the CSV file path
$csvFilePath = 'path/to/your/sample.csv';
// Open the CSV file for reading
if (($handle = fopen($csvFilePath, 'r')) !== FALSE) {
// Read the CSV file headers
$headers = fgetcsv($handle, 1000, ',');
// Prepare the data for API call
$data = [];
while (($row = fgetcsv($handle, 1000, ',')) !== FALSE) {
$data[] = array_combine($headers, $row);
}
fclose($handle);
// Convert data to JSON format
$jsonData = json_encode($data);
// Set the API endpoint
$apiUrl = 'https://api.yourcsvprovider.com/import';
// Initialize cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Bearer YOUR_API_TOKEN'
]);
// Execute the API call
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response: ' . $response;
}
// Close cURL session
curl_close($ch);
} else {
echo 'Failed to open CSV file.';
}
?>
Verifying Successful CSV Data Import
After executing the API call, it's crucial to verify that the data has been successfully imported into your test environment. You can do this by checking the response from the API call and reviewing the data in your sandbox account.
- Check the API response for success messages or error codes.
- Log in to your CSV test account and verify that the imported data appears as expected.
Handling Errors and Troubleshooting CSV API Calls
While making API calls, you may encounter errors. It's important to handle these gracefully to ensure a smooth integration process.
- Implement error handling in your PHP code to catch and log errors.
- Refer to the CSV API documentation for specific error codes and troubleshooting tips.
- Ensure your CSV files are correctly formatted and contain valid data.
Conclusion and Best Practices for CSV API Integration in PHP
Successfully integrating with the CSV API in PHP can greatly enhance your application's data management capabilities. By following the steps outlined in this guide, you can efficiently import data from CSV files, ensuring a seamless integration experience.
Best Practices for Managing CSV API Credentials
- Store your API credentials securely, using environment variables or secure vaults to prevent unauthorized access.
- Regularly rotate your API keys and tokens to maintain security.
Handling CSV API Rate Limiting
Be mindful of any rate limits imposed by the CSV API to avoid disruptions in service. Implement strategies such as:
- Batch processing of CSV data to minimize the number of API calls.
- Implementing exponential backoff strategies to handle rate limit errors gracefully.
Data Transformation and Standardization
Ensure that the data imported from CSV files is properly transformed and standardized to fit your application's requirements. This includes:
- Validating data formats and types before import.
- Mapping CSV fields to your application's data model.
Streamline Your Integrations with Endgrate
Consider using Endgrate to simplify your integration processes. With Endgrate, you can:
- Save time and resources by outsourcing complex integrations.
- Build once for each use case, reducing the need for multiple integrations.
- Provide an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration efforts and focus on your core product development.
Read More
Ready to get started?