Using the Intercom API to Create Or Update Companies (with PHP examples)
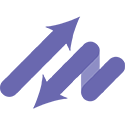
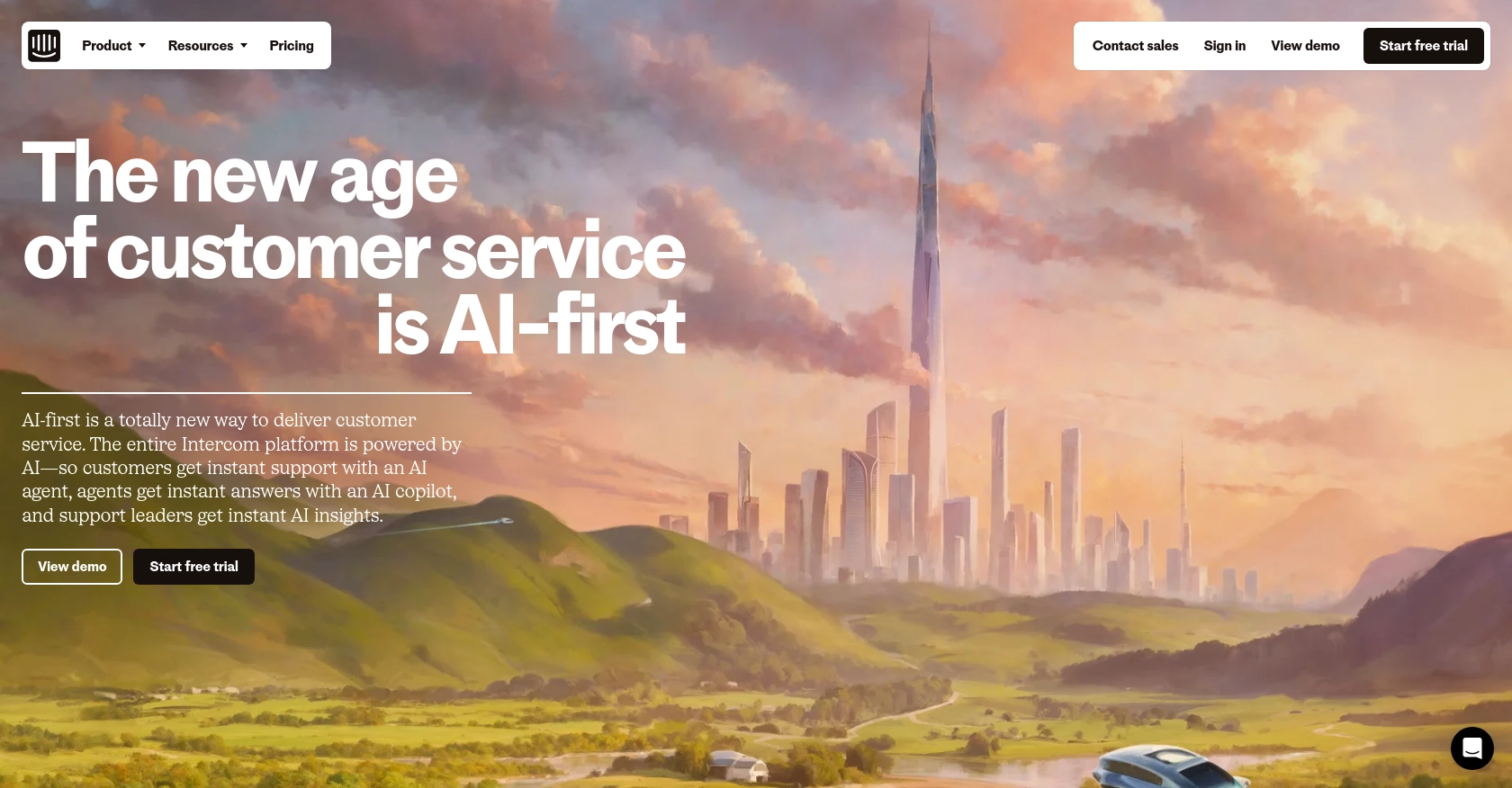
Introduction to Intercom API for Company Management
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for managing customer interactions, including live chat, email marketing, and customer data management.
Developers often need to integrate with Intercom to streamline customer data management and enhance communication workflows. By leveraging the Intercom API, developers can automate the creation and updating of company profiles, ensuring that customer data is always current and accessible. For example, a developer might use the Intercom API to automatically update company details when changes occur in an external CRM system, thereby maintaining data consistency across platforms.
Setting Up Your Intercom Developer Account for API Integration
Before you can start using the Intercom API to create or update companies, you need to set up a developer account. This involves creating a development workspace and configuring OAuth authentication, which is essential for secure API access.
Creating an Intercom Developer Workspace
To begin, you'll need to create a development workspace on Intercom. This workspace allows you to build and test your integration without affecting live data. Follow these steps:
- Visit the Intercom Developer Hub and sign up for a free developer account.
- Once registered, navigate to the Developer Hub and create a new app. Ensure it's associated with your main Intercom workspace.
- Use the development workspace to configure webhooks, define endpoints, and set up OAuth for your app.
Configuring OAuth for Intercom API Access
Intercom uses OAuth 2.0 for authentication, which allows your app to access user data securely. Here's how to set it up:
- In your Developer Hub, select your app and go to the Authentication page.
- Enable the "Use OAuth" option to start the configuration process.
- Provide the necessary information, including Redirect URLs, which must be HTTPS. These URLs are where Intercom will send the authorization code after user approval.
- Specify the permissions your app requires. Only select the scopes necessary for your use case, such as reading and writing company data.
- Save your changes and note down your
client_id
andclient_secret
, which you'll use to obtain an access token.
For more details on setting up OAuth, refer to the Intercom OAuth documentation.
Generating an Access Token for API Requests
Once OAuth is configured, you can generate an access token to authenticate your API requests:
- Redirect users to the following URL to obtain an authorization code:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
- After user authorization, Intercom will redirect to your specified URL with a
code
parameter. - Exchange this code for an access token by making a POST request to:
https://api.intercom.io/auth/eagle/token
- Include the following parameters in your request body:
code
: The authorization code received.client_id
: Your app's client ID.client_secret
: Your app's client secret.
Upon successful exchange, you'll receive an access token, which you can use to authenticate your API calls. For more information, visit the Intercom API documentation.
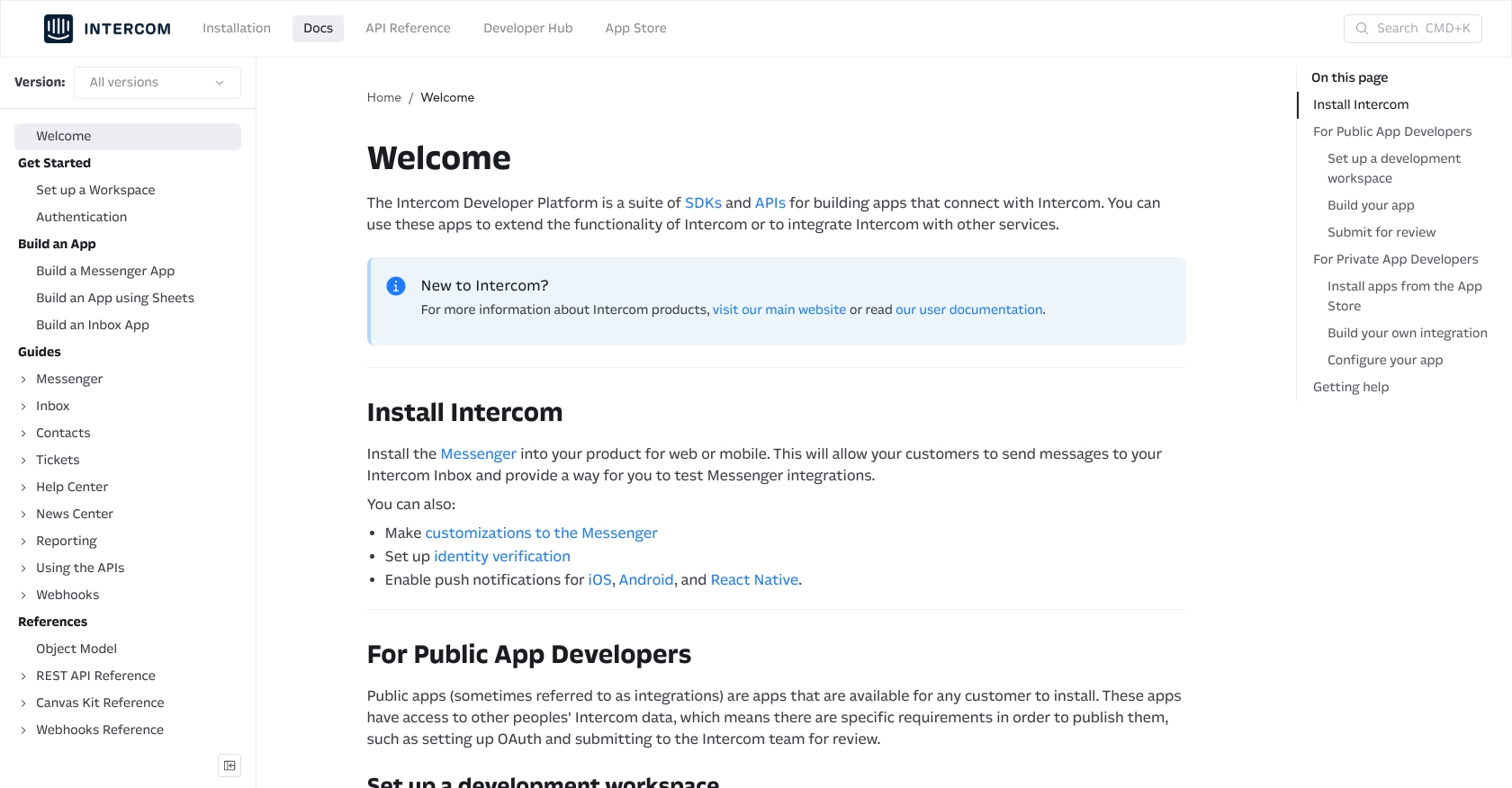
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Intercom Using PHP
To interact with the Intercom API for creating or updating company data, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the PHP code, and handling potential errors.
Setting Up Your PHP Environment for Intercom API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or higher and the Guzzle HTTP client, which simplifies making HTTP requests.
- Ensure PHP is installed on your machine. You can verify this by running
php -v
in your terminal. - Install Composer, the PHP package manager, if you haven't already. Download it from Composer's official website.
- Use Composer to install Guzzle by running the following command in your project directory:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Companies in Intercom
With your environment set up, you can now write PHP code to interact with the Intercom API. Below is an example of how to create or update a company using the API.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$companyData = [
'company_id' => 'unique_company_id',
'name' => 'Example Company',
'remote_created_at' => time(),
'custom_attributes' => [
'industry' => 'Software',
'monthly_spend' => 1000
]
];
$response = $client->post('https://api.intercom.io/companies', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
'Intercom-Version' => '2.11'
],
'json' => $companyData
]);
if ($response->getStatusCode() === 200) {
echo "Company created or updated successfully.";
} else {
echo "Failed to create or update company.";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. The $companyData
array contains the company details you wish to create or update.
Handling API Responses and Errors in Intercom Integration
After making the API call, it's crucial to handle responses and potential errors effectively. The Intercom API returns various status codes:
- 200: Success. The company was created or updated.
- 400: Bad Request. Check the request data for errors.
- 401: Unauthorized. Verify your access token.
- 404: Not Found. Ensure the endpoint is correct.
Always check the response status code and handle errors gracefully to ensure a robust integration.
Verifying API Call Success in Intercom Sandbox
To confirm the success of your API call, log into your Intercom sandbox account and verify the company data. If the company was created or updated successfully, it should appear in your Intercom dashboard.
By following these steps, you can efficiently manage company data in Intercom using PHP, ensuring your customer information is always up-to-date and accurate.
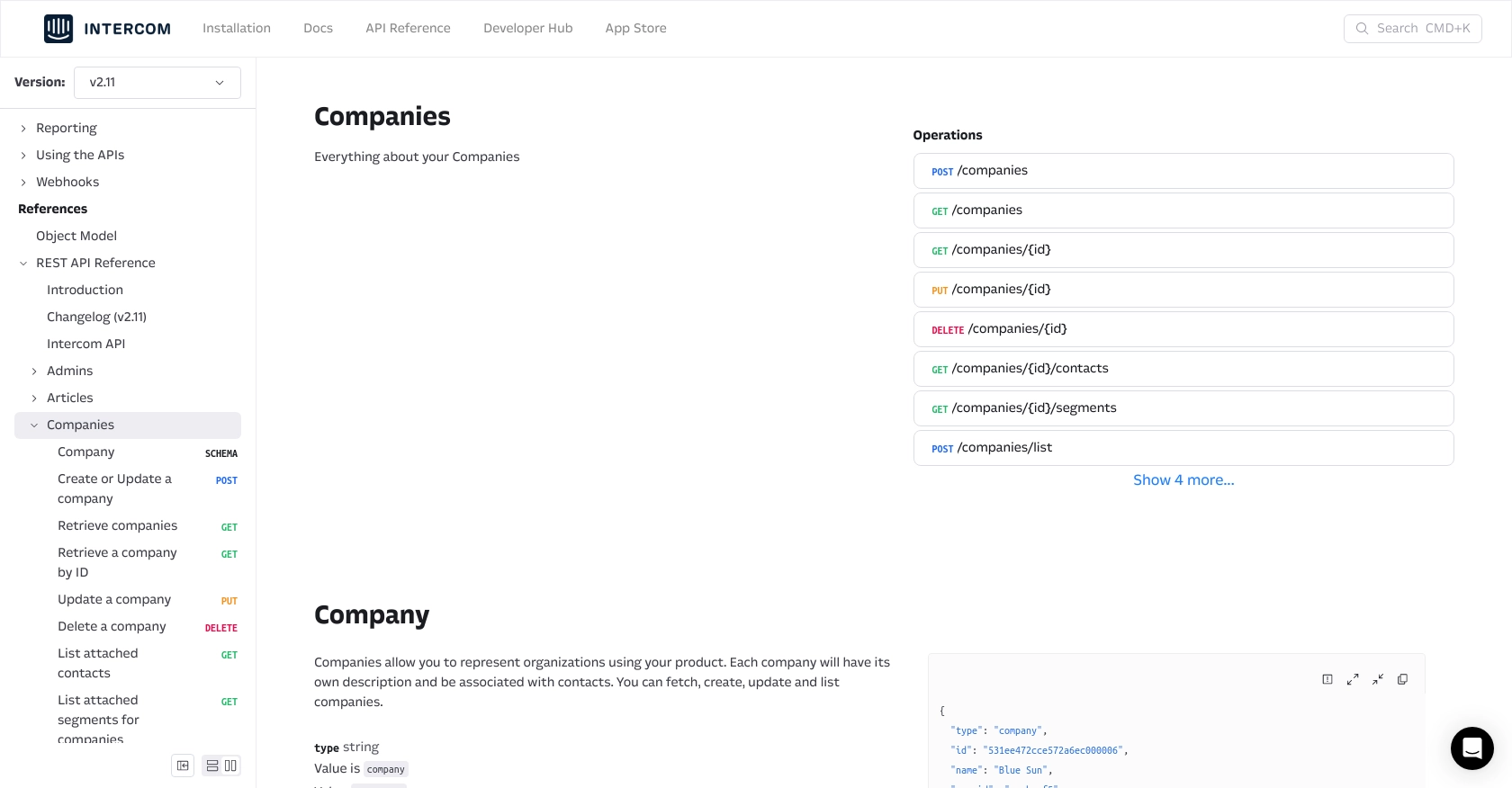
Best Practices for Intercom API Integration with PHP
When integrating with the Intercom API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Securely Store Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access. - Handle Rate Limiting: Intercom's API may impose rate limits. Implement retry logic with exponential backoff to handle
429 Too Many Requests
responses gracefully. For more details, refer to the Intercom API documentation. - Data Standardization: Ensure that data fields are standardized across platforms to maintain consistency. This includes mapping fields correctly between your CRM and Intercom.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes effectively. Log errors for debugging and alerting purposes.
Streamlining Integration Development with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Intercom. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundant efforts.
- Enhance Customer Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Conclusion on Using Intercom API for Company Management with PHP
Integrating with the Intercom API using PHP allows developers to automate and streamline company data management, ensuring that customer information is always up-to-date. By following best practices and leveraging tools like Endgrate, you can enhance your integration capabilities and focus on delivering value to your users.
Start building efficient and scalable integrations today with Intercom and Endgrate, and take your customer communication to the next level.
Read More
Ready to get started?