Using the Salesloft API to Create or Update People (with Python examples)
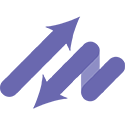
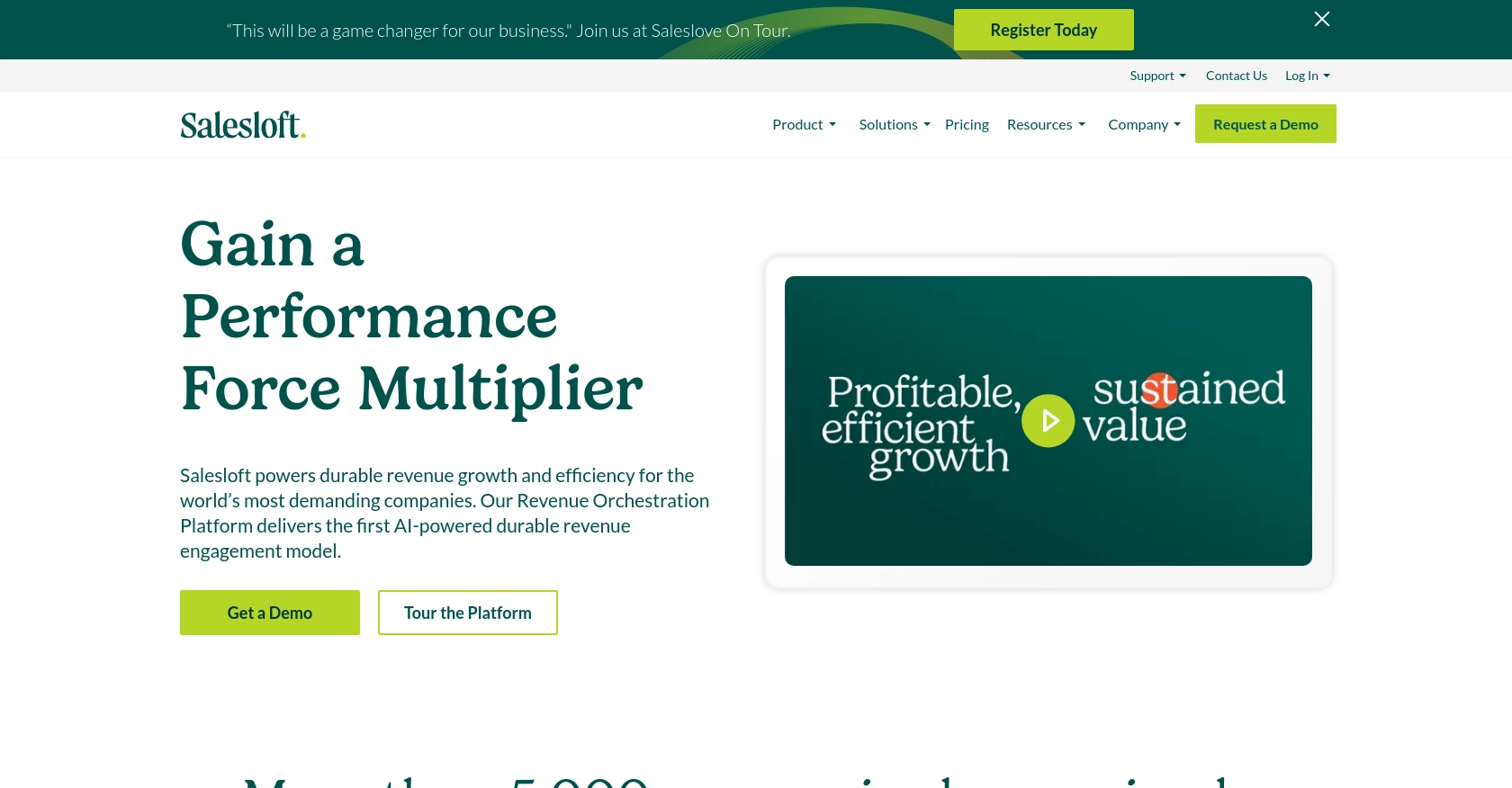
Introduction to Salesloft API Integration
Salesloft is a premier sales engagement platform that empowers sales teams to connect with prospects more effectively. It offers a suite of tools designed to streamline sales processes, enhance productivity, and improve communication with potential clients.
Integrating with the Salesloft API allows developers to automate and manage sales data efficiently. For example, you can use the API to create or update contact information for people within your Salesloft account, ensuring that your sales team always has access to the most current data.
This article will guide you through using Python to interact with the Salesloft API, specifically focusing on creating or updating people records. By following this tutorial, you'll learn how to leverage the API to enhance your sales operations and maintain accurate contact information.
Setting Up Your Salesloft Test/Sandbox Account
Before diving into the integration process, you'll need to set up a Salesloft test or sandbox account. This environment allows you to safely experiment with the API without affecting your live data.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. This trial will give you access to the necessary features to test the API integration.
- Visit the Salesloft website and click on the "Free Trial" button.
- Follow the instructions to create your account, providing the required information.
- Once your account is set up, log in to access the Salesloft dashboard.
Creating a Salesloft OAuth App for API Access
To interact with the Salesloft API, you'll need to create an OAuth app. This app will provide the credentials required for API authentication.
- Navigate to Your Applications in your Salesloft account settings.
- Select OAuth Applications and click on Create New.
- Fill out the necessary fields, such as the app name and description, and click Save.
- After saving, you'll receive your Client ID and Client Secret. Keep these credentials secure as you'll need them for authentication.
Obtaining Authorization Code and Access Tokens
With your OAuth app created, you can now obtain the authorization code and access tokens needed to authenticate API requests.
- Generate a request to the Salesloft authorization endpoint using your Client ID and Redirect URI:
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
- Authorize the app when prompted. Upon approval, you'll receive a code in the redirect URI.
- Exchange this code for an access token by making a POST request:
POST https://accounts.salesloft.com/oauth/token { "client_id": "YOUR_CLIENT_ID", "client_secret": "YOUR_CLIENT_SECRET", "code": "YOUR_AUTHORIZATION_CODE", "grant_type": "authorization_code", "redirect_uri": "YOUR_REDIRECT_URI" }
- Store the access token securely as it will be used to authenticate your API requests.
With your Salesloft test account and OAuth app set up, you're ready to start making API calls to create or update people records.
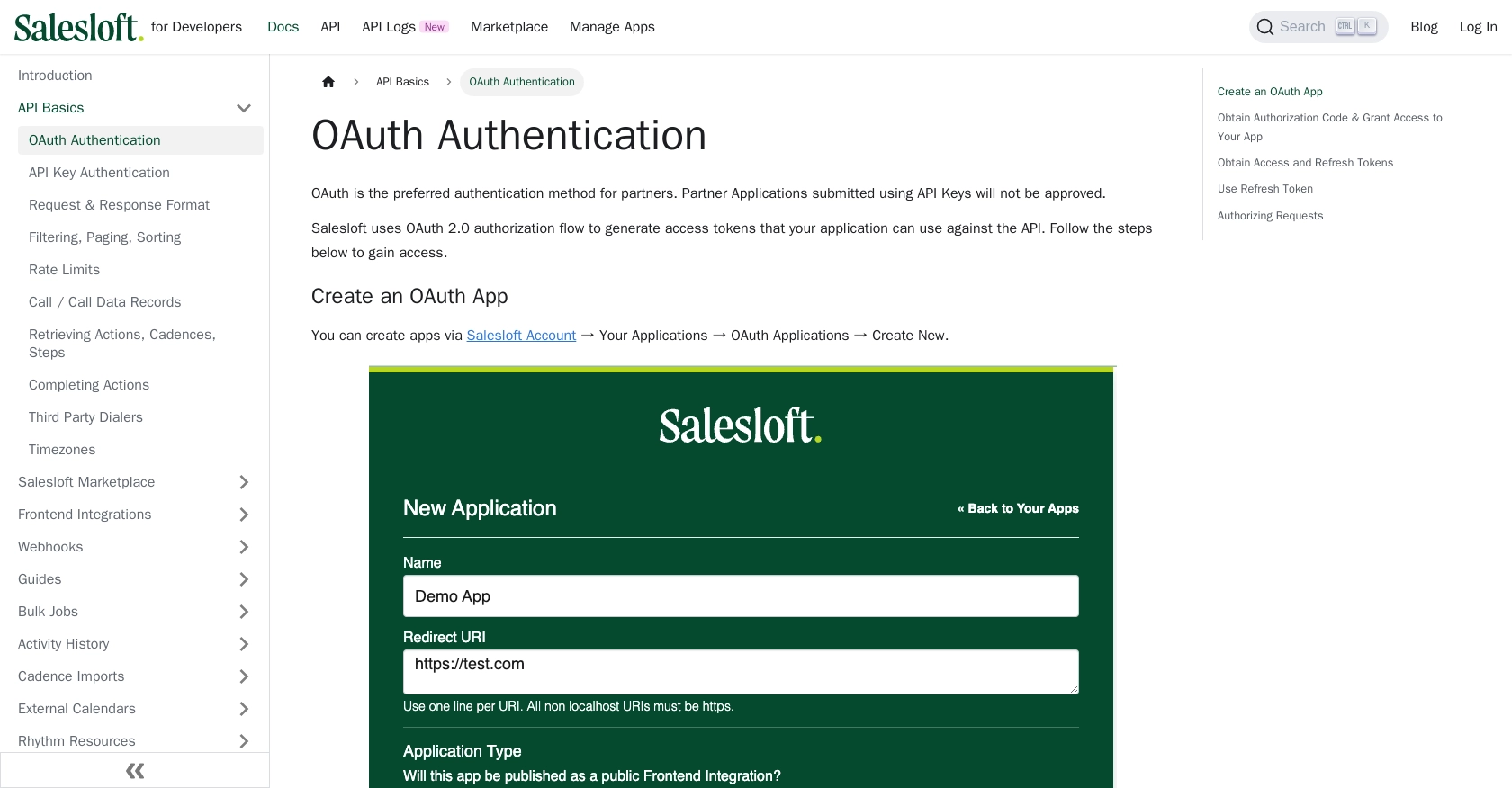
sbb-itb-96038d7
Making API Calls to Salesloft Using Python
Now that you have set up your Salesloft OAuth app and obtained the necessary access tokens, you can start making API calls to create or update people records. This section will guide you through the process of using Python to interact with the Salesloft API.
Prerequisites for Python Integration with Salesloft API
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Additionally, install the requests
library to handle HTTP requests:
pip install requests
Creating a Person in Salesloft
To create a new person in Salesloft, you will use the POST
method. Below is a Python script to achieve this:
import requests
# Set the API endpoint
url = "https://api.salesloft.com/v2/people"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the person data
person_data = {
"email_address": "example@salesloft.com",
"first_name": "John",
"last_name": "Doe"
}
# Send the POST request
response = requests.post(url, json=person_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Person created successfully:", response.json())
else:
print("Failed to create person:", response.status_code, response.json())
Replace YOUR_ACCESS_TOKEN
with your actual access token. This script sends a request to create a person with the specified email, first name, and last name.
Updating a Person in Salesloft
To update an existing person, use the PUT
method. Here’s how you can update a person's information:
import requests
# Set the API endpoint with the person's ID
person_id = "PERSON_ID"
url = f"https://api.salesloft.com/v2/people/{person_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Define the updated person data
updated_data = {
"first_name": "Jane",
"last_name": "Smith"
}
# Send the PUT request
response = requests.put(url, json=updated_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Person updated successfully:", response.json())
else:
print("Failed to update person:", response.status_code, response.json())
Replace PERSON_ID
with the ID of the person you wish to update and YOUR_ACCESS_TOKEN
with your access token.
Handling Errors and Verifying API Requests
To ensure your API requests are successful, always check the response status code. A status code of 200
indicates success, while other codes such as 403
, 404
, or 422
indicate errors. Refer to the Salesloft API documentation for more details on error handling.
After creating or updating a person, verify the changes in your Salesloft account to ensure the data reflects accurately.
By following these steps, you can efficiently manage people records in Salesloft using Python, enhancing your sales operations and data accuracy.
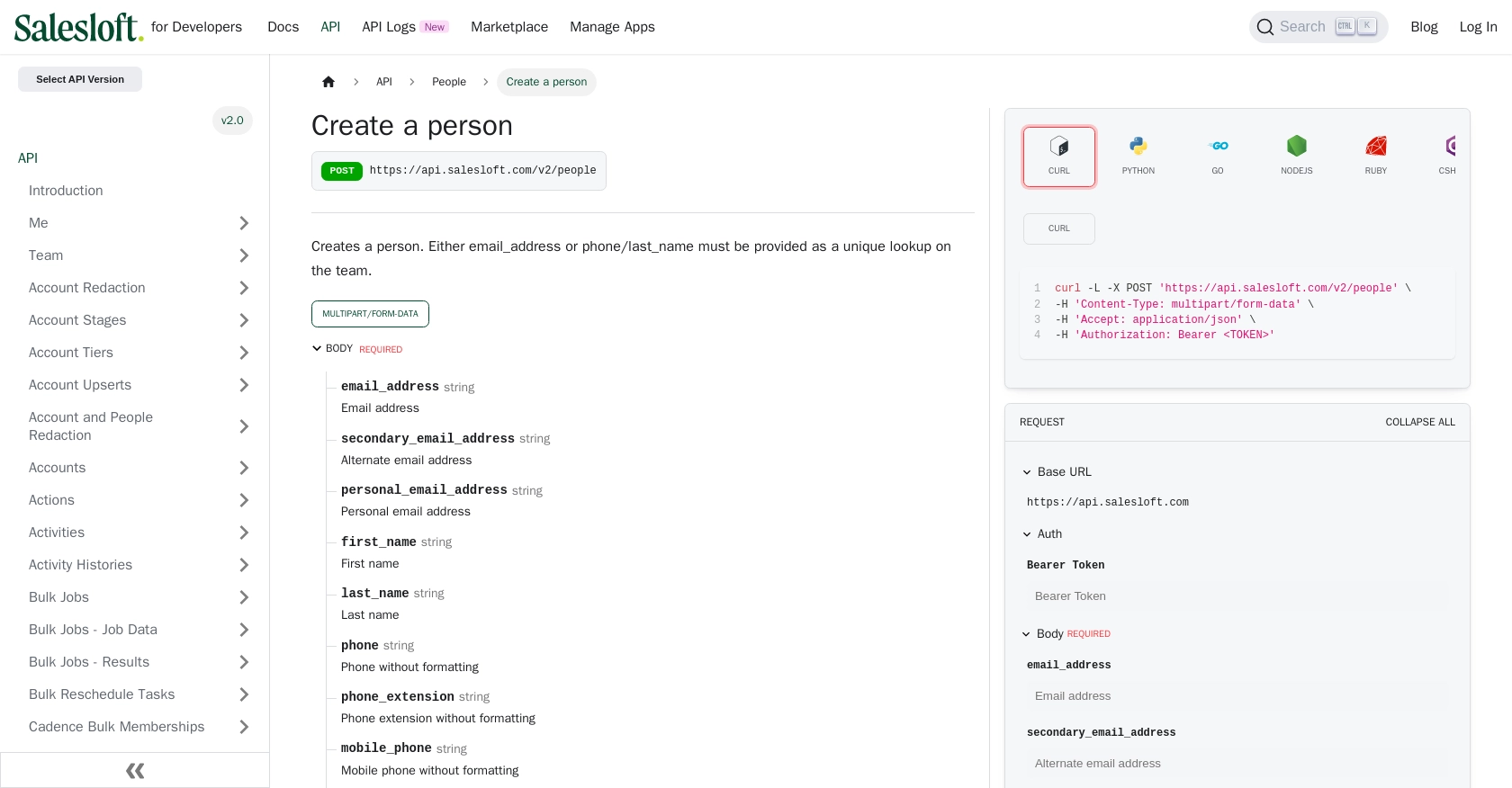
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using Python can significantly enhance your sales operations by automating the management of contact data. By following the steps outlined in this guide, you can efficiently create and update people records, ensuring your sales team has access to the most current information.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Storage of Credentials: Always store your access tokens and client secrets securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of the rate limits imposed by Salesloft, which are currently set at 600 cost per minute. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Salesloft API rate limits documentation.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Use the error messages provided by the API to debug and resolve issues efficiently.
- Data Standardization: Ensure that the data you send and receive is standardized and consistent with your internal systems to maintain data integrity.
Enhancing Integration Efficiency with Endgrate
While integrating with Salesloft directly can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Salesloft. This allows you to build once for each use case and avoid the hassle of maintaining multiple integrations.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/people-create/
Ready to get started?