Using the Hubspot API to Create or Update Custom Objects in Javascript
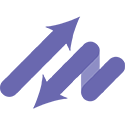
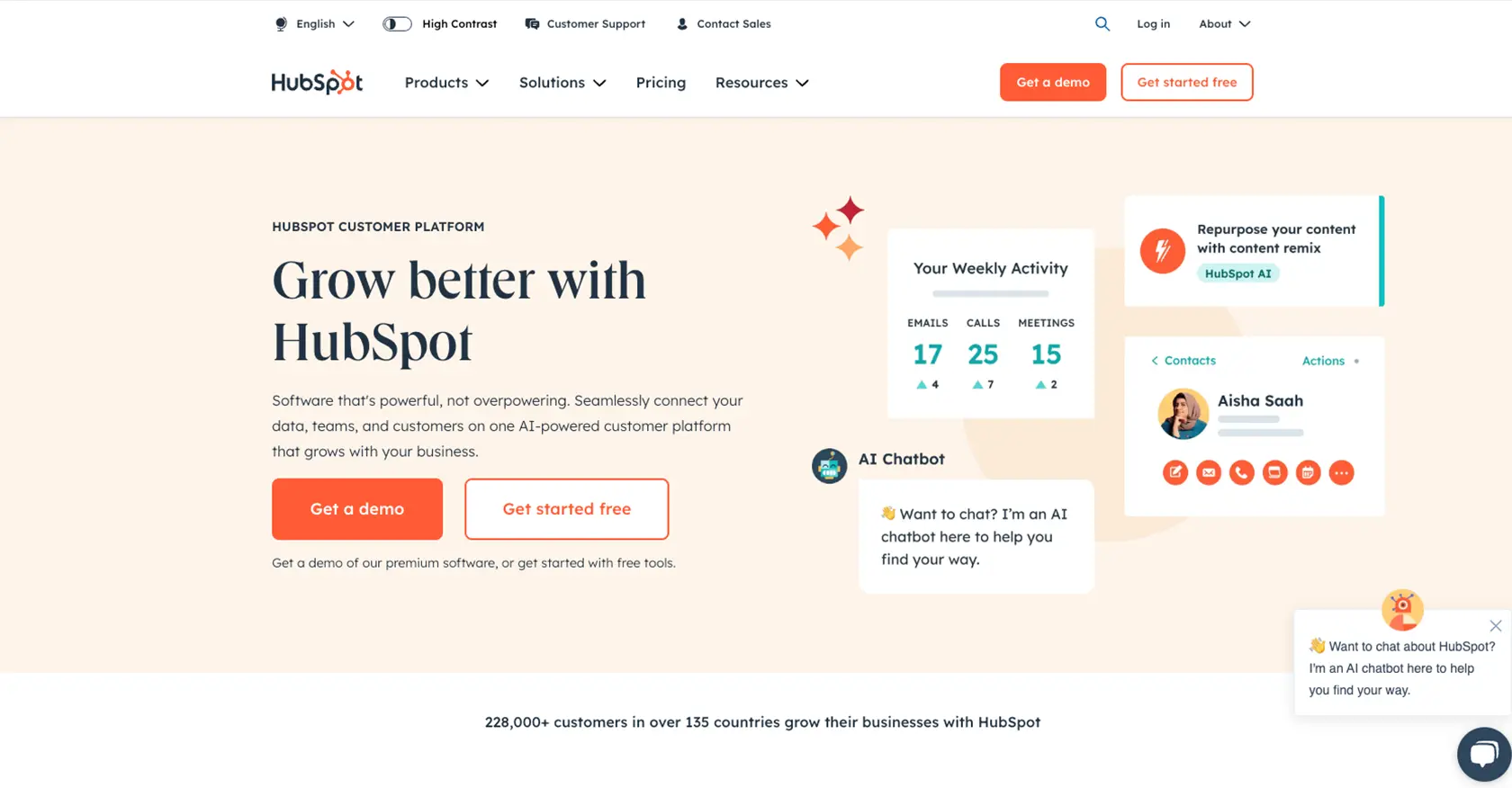
Introduction to HubSpot Custom Objects API
HubSpot is a powerful CRM platform that offers a wide range of tools to help businesses manage their customer relationships effectively. With its robust API, developers can create custom integrations to enhance the functionality of their applications and streamline business processes.
Integrating with HubSpot's Custom Objects API allows developers to create or update custom objects, providing flexibility in how data is organized and accessed. This is particularly useful for businesses that need to manage unique data sets that don't fit into standard CRM objects like contacts or deals.
For example, a developer might use the HubSpot API to create a custom object representing a product inventory, allowing sales teams to track product availability and details directly within HubSpot. This integration can significantly improve data accessibility and operational efficiency.
Setting Up Your HubSpot Developer Account for Custom Objects API
Before diving into the integration with HubSpot's Custom Objects API using JavaScript, you'll need to set up a HubSpot developer account. This account will allow you to create and manage the necessary applications and access tokens for API interactions.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is set up, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
To test your API integrations without affecting live data, it's advisable to use a sandbox account. Here's how to set it up:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- Use this sandbox account to safely test API calls and interactions.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for authentication, ensuring secure access to its APIs. Follow these steps to configure OAuth:
- In your developer dashboard, go to "Apps" and click on "Create an app."
- Fill in the app details, including name and description.
- Under "Auth," select OAuth 2.0 as the authentication method.
- Define the necessary scopes for your app, such as
crm.objects.custom.write
for creating or updating custom objects. - Save the app settings and note down the client ID and client secret provided.
For more details on OAuth setup, refer to the HubSpot OAuth Documentation.
Generating an OAuth Access Token
To interact with the HubSpot API, you'll need an OAuth access token. Here's how to obtain it:
- Direct users to the authorization URL provided by HubSpot, including your client ID and requested scopes.
- Once users authorize the app, they'll be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to HubSpot's token endpoint.
Ensure you securely store the access token and refresh it as needed. For more information, check the HubSpot API Usage Guidelines.
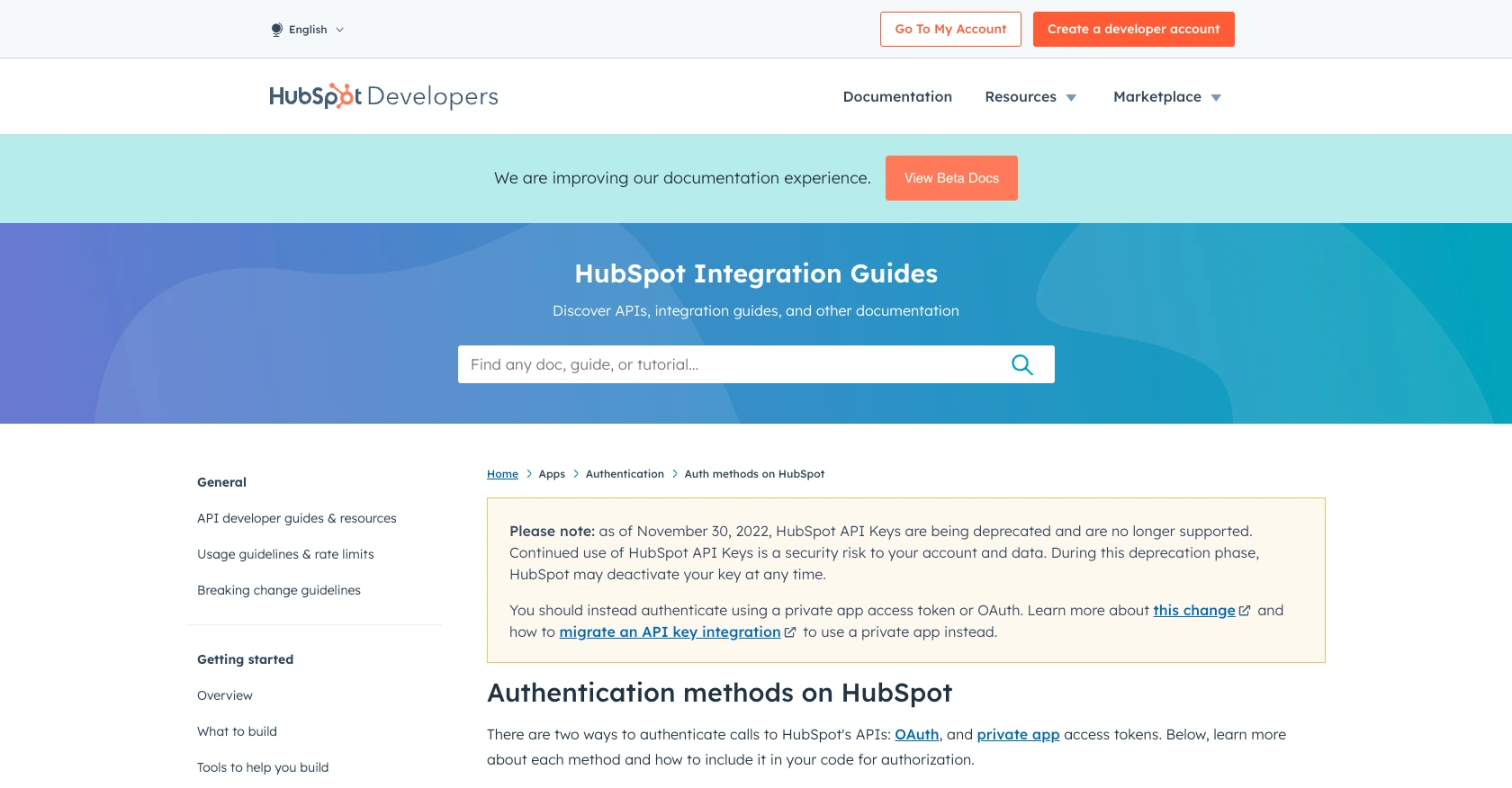
sbb-itb-96038d7
Making API Calls to HubSpot Custom Objects Using JavaScript
To interact with HubSpot's Custom Objects API using JavaScript, you'll need to set up your environment and write code to handle API requests. This section will guide you through the process, ensuring you can create or update custom objects efficiently.
Setting Up Your JavaScript Environment for HubSpot API Integration
Before making API calls, ensure your JavaScript environment is ready. You'll need Node.js installed on your machine, along with the necessary packages.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library for making HTTP requests by running
npm install axios
.
Example Code for Creating or Updating HubSpot Custom Objects
Below is an example of how to create or update custom objects in HubSpot using JavaScript and Axios. Replace Your_Access_Token
with the OAuth access token you obtained earlier.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.hubapi.com/crm/v3/objects/custom_object';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Define the custom object data
const customObjectData = {
properties: {
name: 'Example Object',
description: 'This is a sample custom object'
}
};
// Function to create or update a custom object
async function createOrUpdateCustomObject() {
try {
const response = await axios.post(endpoint, customObjectData, { headers });
console.log('Custom Object Created/Updated:', response.data);
} catch (error) {
console.error('Error creating/updating custom object:', error.response.data);
}
}
// Execute the function
createOrUpdateCustomObject();
Verifying API Call Success in HubSpot Sandbox
After running the code, verify the success of your API call by checking the custom objects in your HubSpot sandbox account. Navigate to the custom objects section to see if the new object appears as expected.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors. The example code above includes a try-catch
block to catch and log errors. HubSpot API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 429 Too Many Requests: Rate limit exceeded. Refer to HubSpot API Usage Guidelines for rate limits.
For a complete list of error codes, refer to the HubSpot Custom Objects API Documentation.
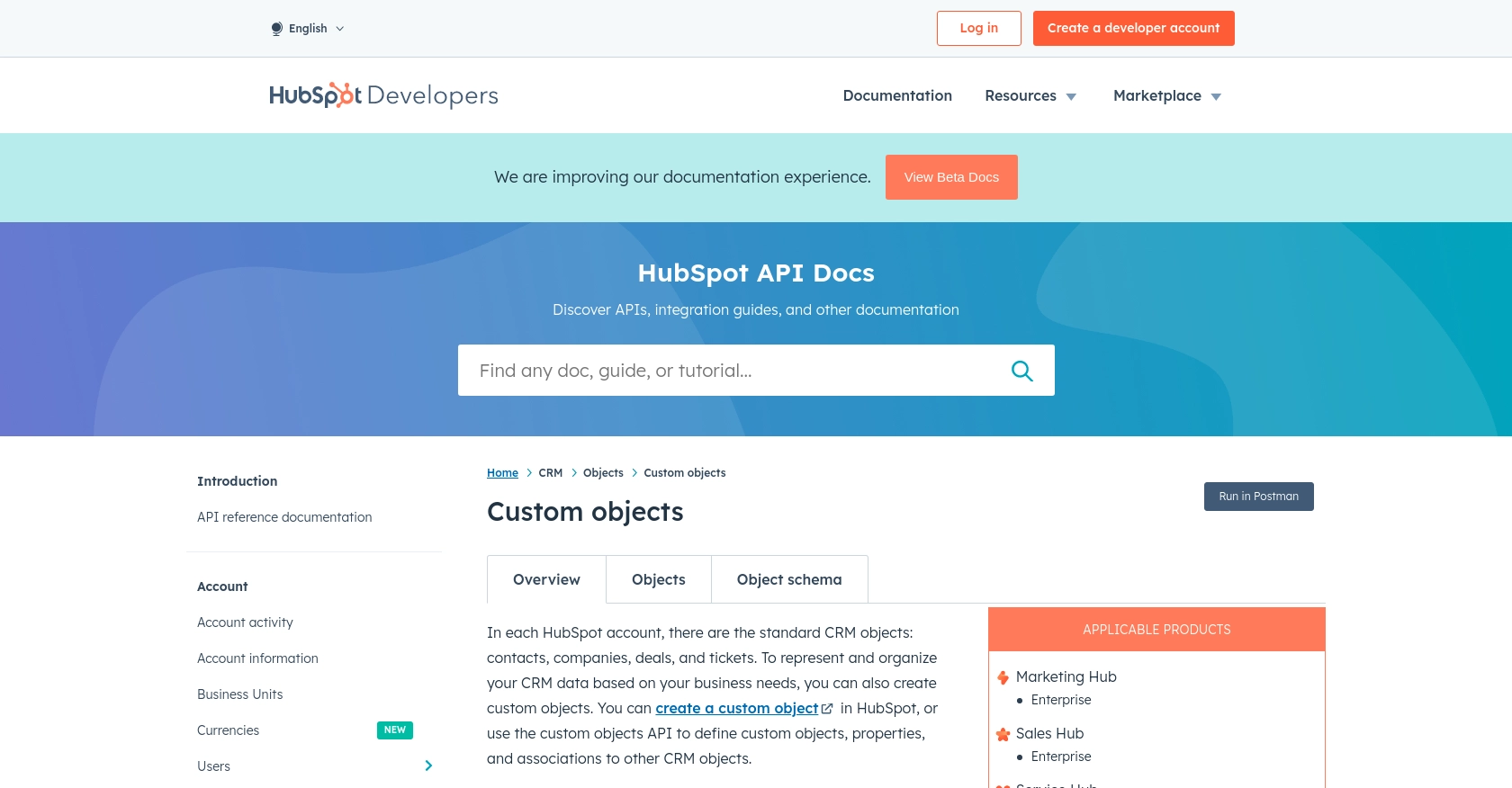
Conclusion and Best Practices for HubSpot Custom Objects API Integration
Integrating with HubSpot's Custom Objects API using JavaScript provides a powerful way to extend the capabilities of your CRM. By creating or updating custom objects, you can tailor HubSpot to fit unique business needs, enhancing data management and operational efficiency.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store Credentials: Always store your OAuth access tokens securely and refresh them as needed to maintain access.
- Handle Rate Limits: HubSpot enforces rate limits, such as 100 requests every 10 seconds for OAuth apps. Implement request throttling to avoid hitting these limits. For more details, refer to the HubSpot API Usage Guidelines.
- Data Transformation: Ensure that data fields are standardized and transformed as necessary to fit your custom object schema.
- Error Handling: Implement robust error handling to manage API response errors effectively, ensuring a smooth integration experience.
Leverage Endgrate for Seamless HubSpot Integrations
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing integrations across multiple platforms, including HubSpot. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Focus on your core product development while ensuring seamless integration experiences for your customers.
- Build once for each use case and apply it across various integrations, reducing redundancy and effort.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/crm-custom-objects
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?