Using the Copper API to Get People (with Python examples)
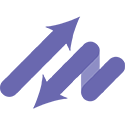
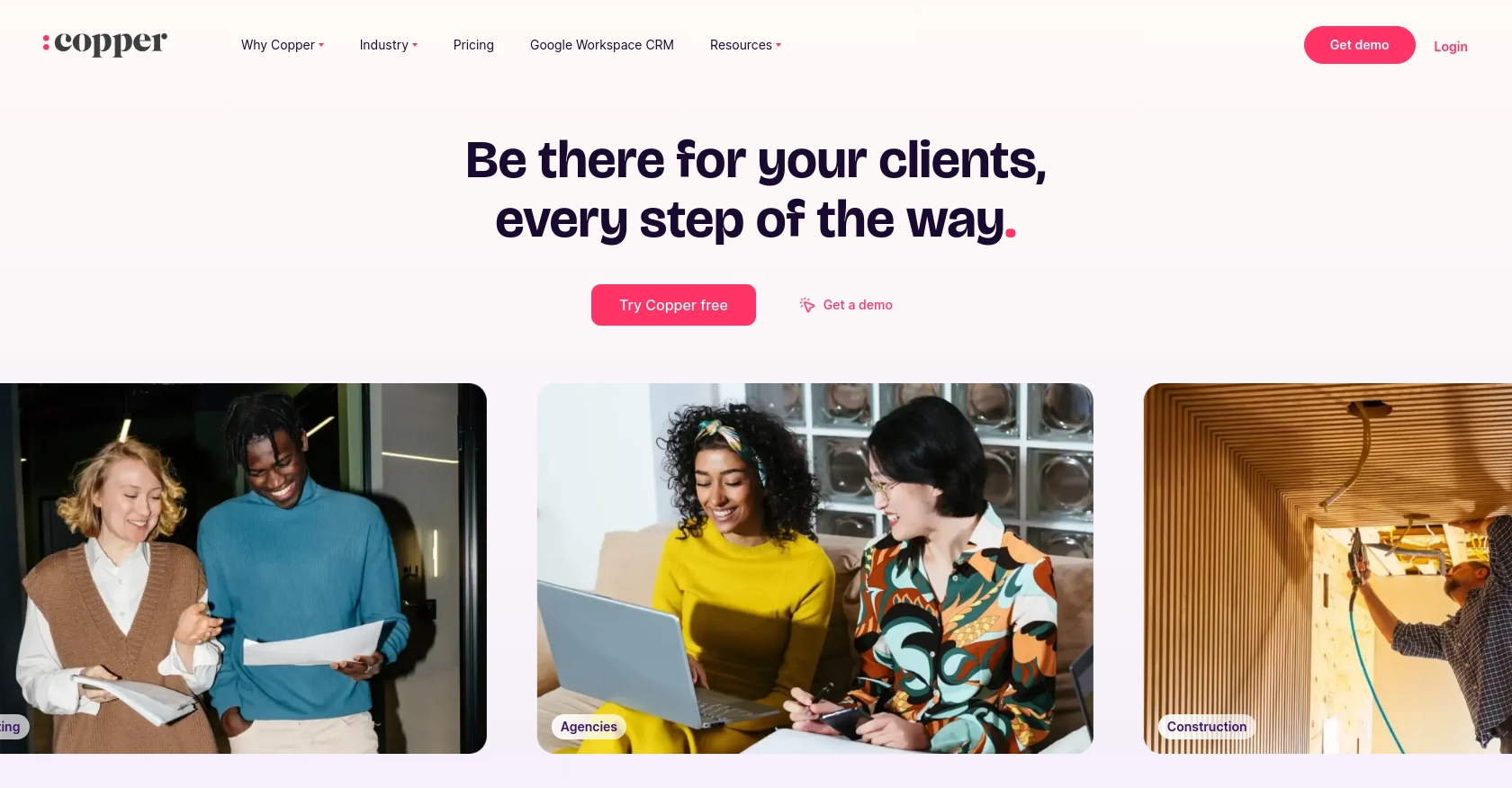
Introduction to Copper API Integration
Copper is a robust platform designed to streamline the management of digital assets and transactions. It offers a comprehensive suite of tools for secure and efficient handling of cryptocurrency operations, making it a preferred choice for businesses looking to integrate digital asset management into their workflows.
Integrating with Copper's API allows developers to automate and enhance various processes, such as retrieving and managing user data. For example, you can use the Copper API to access detailed information about people associated with your organization, enabling more personalized and efficient interactions.
This article will guide you through using Python to interact with the Copper API, specifically focusing on retrieving people data. By following this guide, developers can seamlessly integrate Copper's capabilities into their applications, enhancing functionality and user experience.
Setting Up Your Copper API Test Account
Before you can start integrating with the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data. Follow these steps to get started:
Create a Copper Account
- Visit the Copper website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete your registration.
- Once your account is created, log in to access the Copper dashboard.
Generate Copper API Keys
To authenticate your API requests, you'll need to generate an API key and secret:
- Navigate to the API section in your Copper dashboard.
- Click on "Generate API Key" to create a new key.
- Copy the API key and secret provided. Store them securely as you'll need them for authentication.
Configure API Authentication
Copper uses a custom authentication method that requires signing requests. Here's how to set it up:
import hashlib
import hmac
import requests
import time
ApiKey = 'Your_API_Key'
Secret = 'Your_API_Secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/accounts"
body = ""
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
url = 'https://api.copper.co' + path
headers = {
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
}
response = requests.get(url, headers=headers)
print(response.json())
Verify API Access
Test your setup by making a simple API call to verify that your authentication is working correctly. Use the example code above to retrieve account information and ensure you receive a successful response.
By following these steps, you'll be ready to start integrating Copper's API into your applications, allowing you to leverage its powerful features for managing digital assets and transactions.
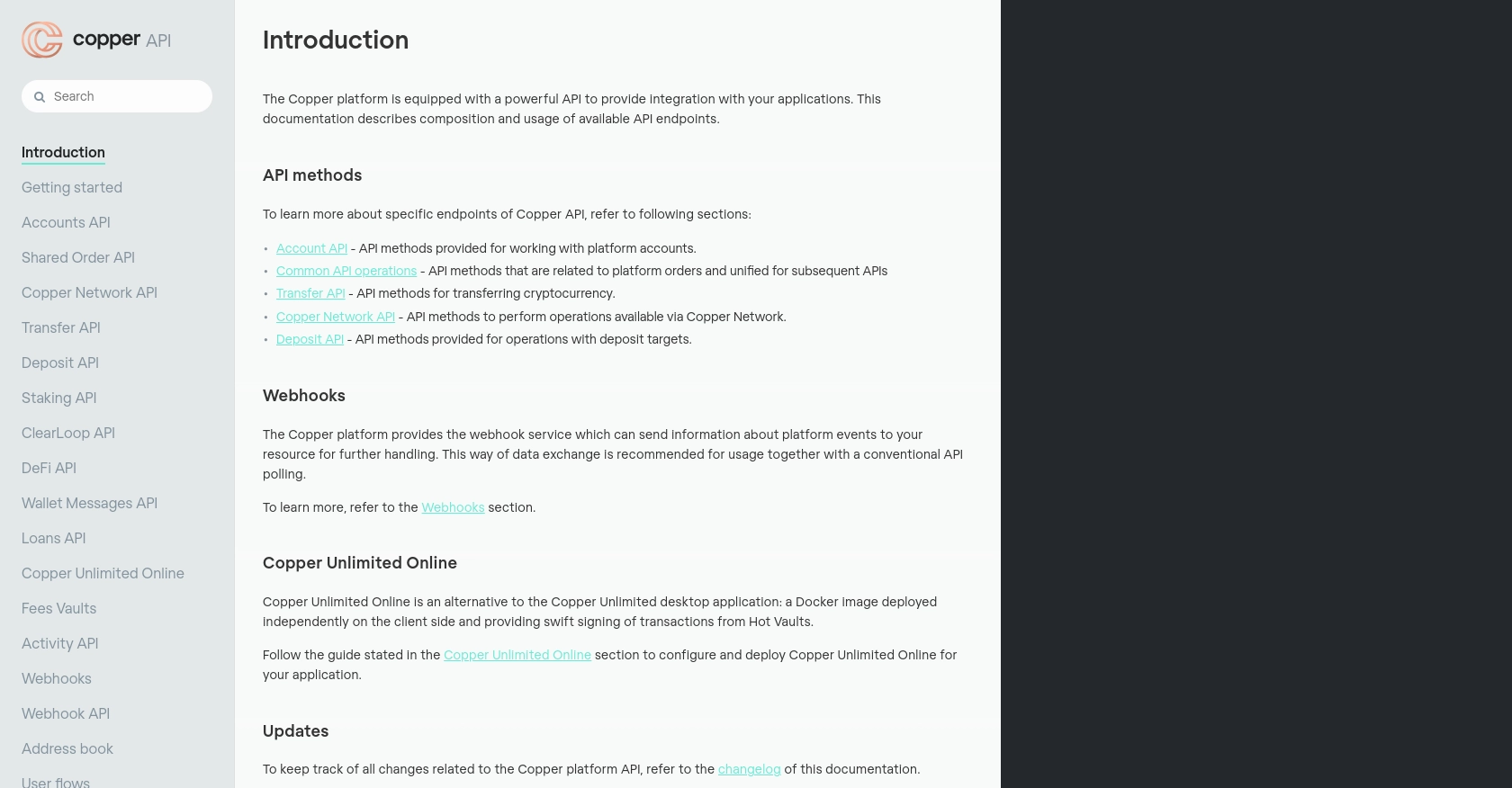
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Copper Using Python
To interact with the Copper API and retrieve people data, you'll need to set up your Python environment and understand the necessary API calls. This section will guide you through the process, ensuring you can efficiently access and manage people data within your application.
Python Environment Setup for Copper API Integration
Before making API calls, ensure your Python environment is correctly configured:
- Install Python 3.11.1 or later.
- Ensure you have
pip
installed for managing packages. - Install the
requests
library using the following command:
pip install requests
Example Code for Retrieving People Data from Copper API
With your environment ready, you can now write the Python code to make API calls to Copper:
import hashlib
import hmac
import requests
import time
# Replace with your Copper API credentials
ApiKey = 'Your_API_Key'
Secret = 'Your_API_Secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/platform/people"
body = ""
# Generate the signature for authentication
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
# Define the API endpoint and headers
url = 'https://api.copper.co' + path
headers = {
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
}
# Make the GET request to retrieve people data
response = requests.get(url, headers=headers)
# Output the response
if response.status_code == 200:
print("People data retrieved successfully:")
print(response.json())
else:
print("Failed to retrieve people data:", response.status_code, response.text)
Understanding the API Response and Handling Errors
Upon executing the code, you should receive a JSON response containing the people data. Here's how to verify and handle potential errors:
- Check the response status code. A status code of
200
indicates success. - If the request fails, the code will print the status code and error message for debugging.
- Refer to Copper's documentation for detailed information on error codes and troubleshooting steps.
Verifying Data in Copper's Test Environment
After retrieving the data, verify it by checking the Copper test environment:
- Log in to your Copper test account.
- Navigate to the people section to ensure the data matches the API response.
By following these steps, you can effectively integrate Copper's API into your Python applications, enabling seamless access to people data and enhancing your application's capabilities.
Conclusion and Best Practices for Copper API Integration
Integrating the Copper API into your Python applications can significantly enhance your ability to manage digital assets and user data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve and utilize people data from Copper, streamlining your operations and improving user interactions.
Best Practices for Secure and Efficient Copper API Usage
- Secure API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code and consider using environment variables.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic with exponential backoff.
- Data Standardization: When integrating data from Copper, ensure that your application standardizes and transforms data fields as needed to maintain consistency across your systems.
- Error Handling: Implement robust error handling to manage API response errors effectively. Use Copper's documentation to understand error codes and troubleshoot issues.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline their integration processes further, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore the possibilities with Endgrate and see how it can simplify your integration strategy. Visit Endgrate to learn more.
Read More
Ready to get started?