How to Get Tax Rates with the Sage Accounting API in Javascript
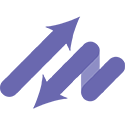
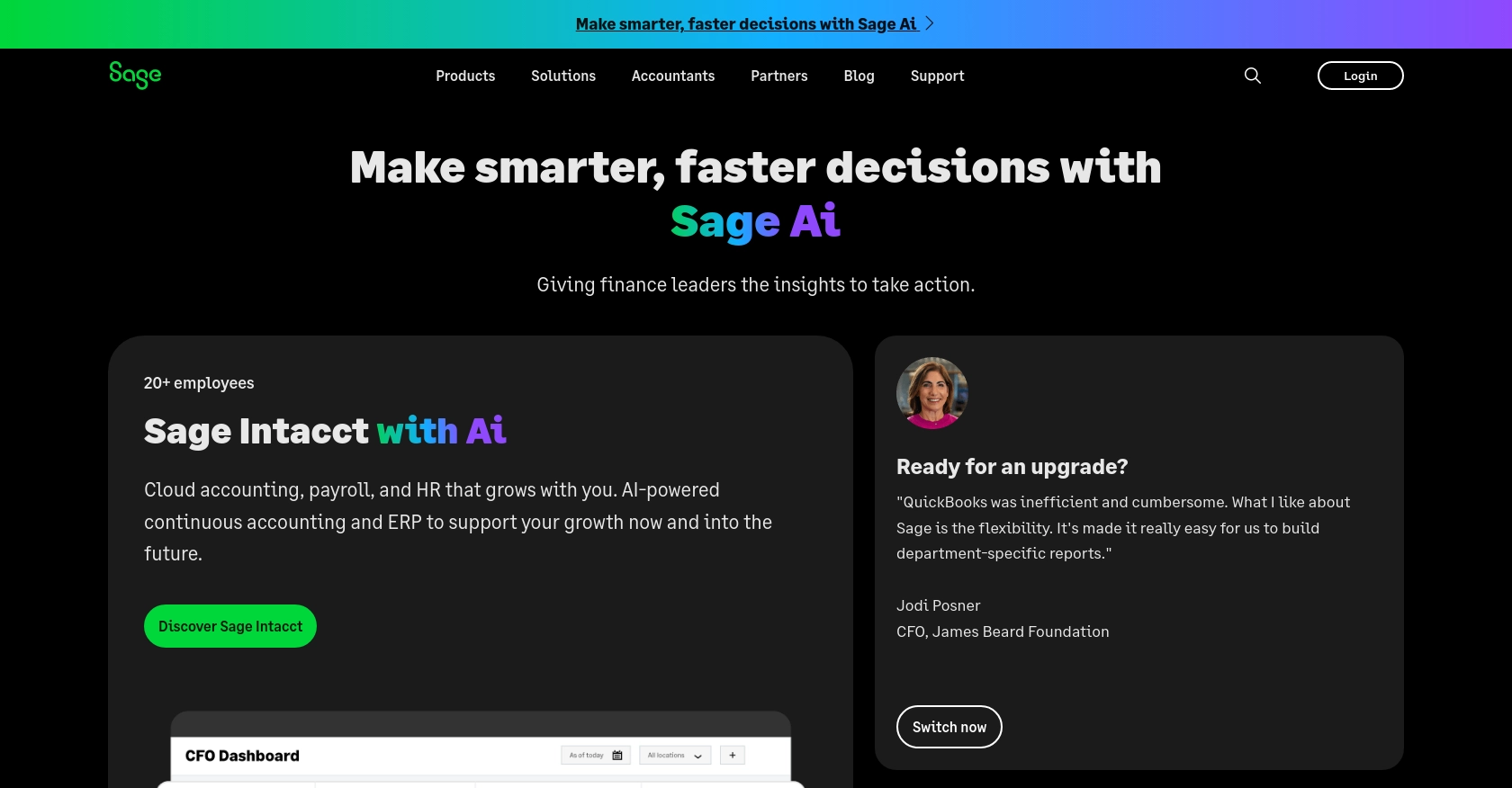
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small and medium-sized businesses. It offers a comprehensive suite of tools to manage finances, streamline operations, and ensure compliance with tax regulations. With its user-friendly interface and powerful features, Sage Accounting is a preferred choice for businesses aiming to enhance their financial management processes.
Integrating with the Sage Accounting API allows developers to automate and optimize various accounting tasks, such as retrieving tax rates. For example, a developer might use the Sage Accounting API to fetch current tax rates and apply them to invoices automatically, ensuring accurate and up-to-date tax calculations.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start interacting with the Sage Accounting API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting any real data. Follow these steps to get started:
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the guide on creating an app to complete your registration.
Set Up a Trial Business for Development
Next, you'll need to create a trial business account. Sage offers trial accounts for various regions and subscription tiers, allowing you to test your integration thoroughly.
- Go to the Sage Accounting Quick Start Guide and choose the appropriate region and subscription tier for your needs.
- Sign up for a trial account by following the instructions provided.
Create a Sage App for OAuth Authentication
Since the Sage Accounting API uses OAuth for authentication, you'll need to create an app to obtain your client ID and client secret.
- Log in to your Sage Developer account and navigate to the Create an App section.
- Click on "Create App" and enter a name and callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Save your app to generate the client ID and client secret, which you will use for authentication.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account. This provides 12 months of free access for testing your integration.
- Submit a request to upgrade your account by providing your name, email address, app name, client ID, and region as detailed in the Upgrade Your Account guide.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
With your Sage Accounting test account set up, you are now ready to start making API calls and exploring the capabilities of the Sage Accounting API.
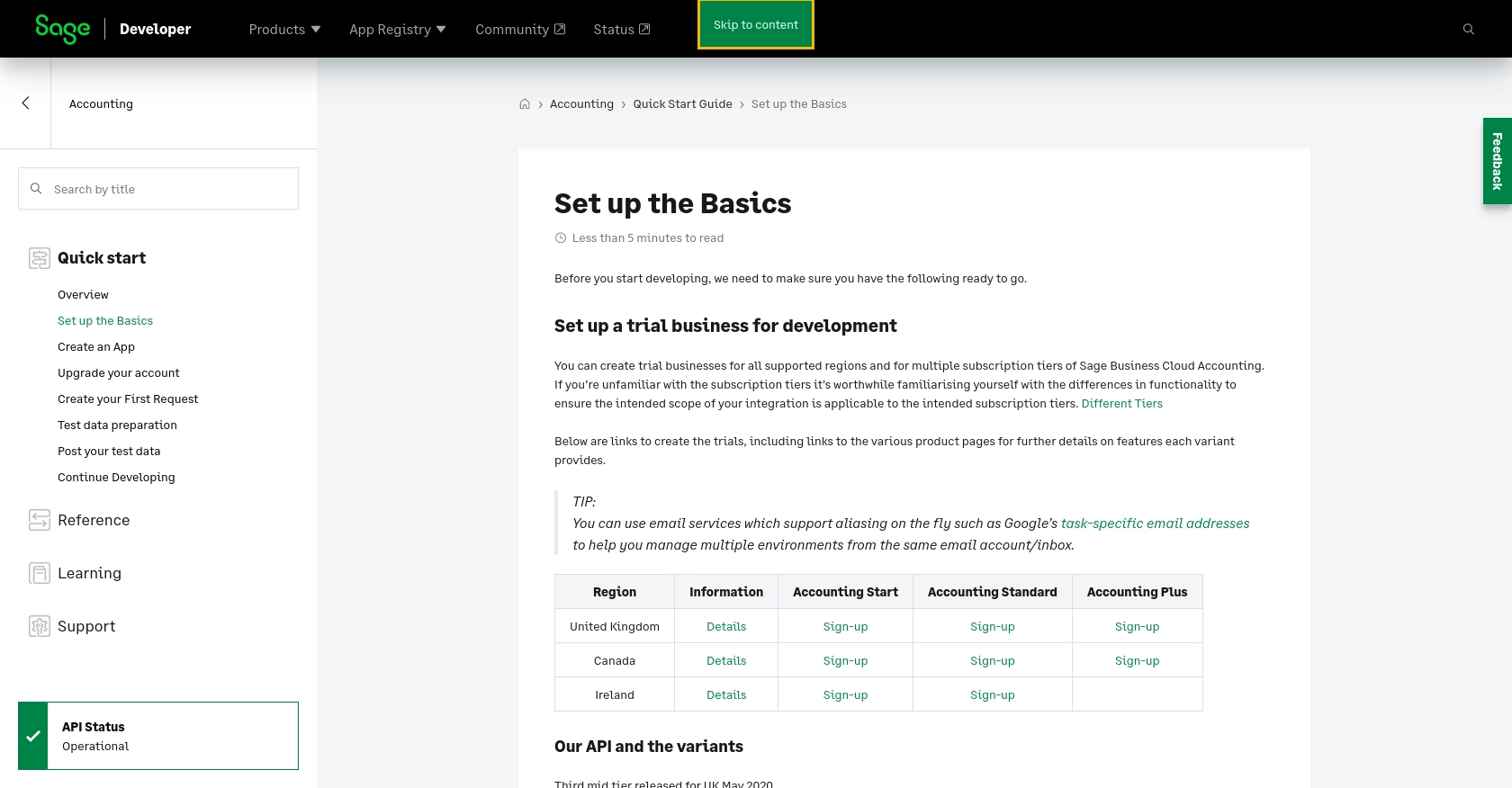
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates with Sage Accounting API in JavaScript
To interact with the Sage Accounting API and retrieve tax rates, you'll need to use JavaScript to make HTTP requests. This section will guide you through setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor such as Visual Studio Code.
- Install the Axios library for making HTTP requests by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Tax Rates
Now that your environment is set up, you can write the JavaScript code to retrieve tax rates from the Sage Accounting API. Create a file named getTaxRates.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.accounting.sage.com/v3.1/tax_rates';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to fetch tax rates
async function fetchTaxRates() {
try {
const response = await axios.get(endpoint, { headers });
const taxRates = response.data;
console.log('Tax Rates:', taxRates);
} catch (error) {
console.error('Error fetching tax rates:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchTaxRates();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process.
Understanding the Code and Expected Output
In the code above, we use the Axios library to make a GET request to the Sage Accounting API's tax rates endpoint. The response is expected to contain a list of tax rates, which we log to the console.
Run the script using the following command:
node getTaxRates.js
You should see the tax rates printed in the console, similar to the following sample output:
[
{
"id": "string",
"displayed_as": "string",
"name": "string",
"percentage": 0.1
},
...
]
Handling Errors and Verifying API Requests
It's crucial to handle errors gracefully when making API calls. The code above includes a try-catch
block to catch and log any errors that occur during the request. Common errors include network issues or invalid authentication tokens.
To verify that your request succeeded, you can cross-check the returned tax rates with the data in your Sage Accounting sandbox account. Ensure that the tax rates match the expected values.
For more information on error codes, refer to the Sage Accounting API documentation.
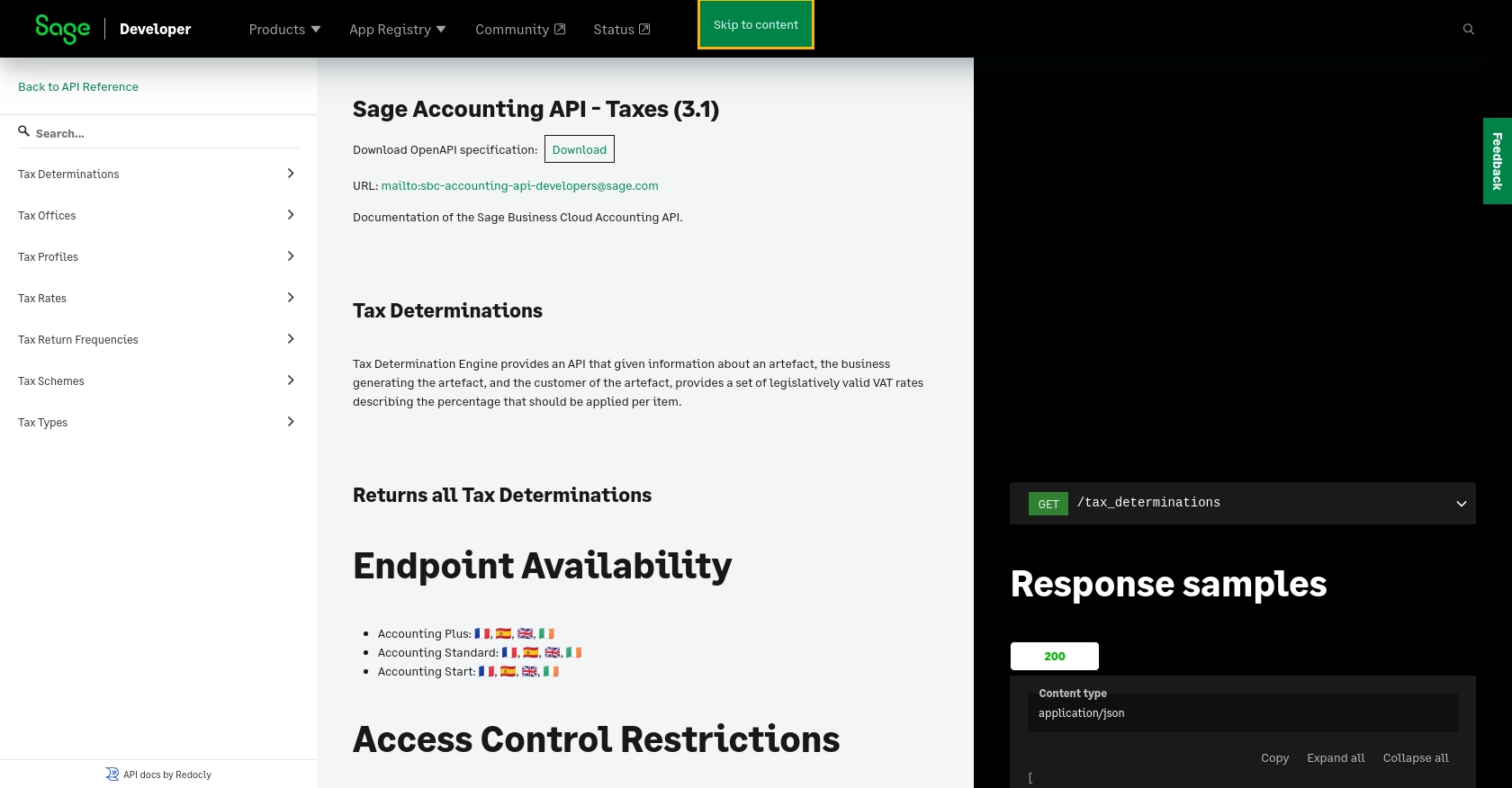
Conclusion and Best Practices for Using Sage Accounting API in JavaScript
Integrating with the Sage Accounting API to retrieve tax rates using JavaScript can significantly enhance your application's financial management capabilities. By automating tax calculations, you ensure accuracy and compliance, saving time and reducing errors.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the tax rates and other data retrieved from the API are standardized and formatted correctly for your application's needs.
- Error Handling: Implement comprehensive error handling to manage network issues, authentication errors, and other potential problems. Log errors for troubleshooting and monitoring.
Enhancing Integration with Endgrate
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can manage various integrations through a unified API, reducing complexity and focusing on your core product development. This approach allows you to build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration process by visiting Endgrate's website and discover how you can save time and resources while delivering a seamless experience to your users.
Read More
Ready to get started?