Using the Zoho Books API to Get Purchase Orders (with PHP examples)
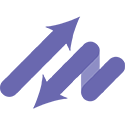
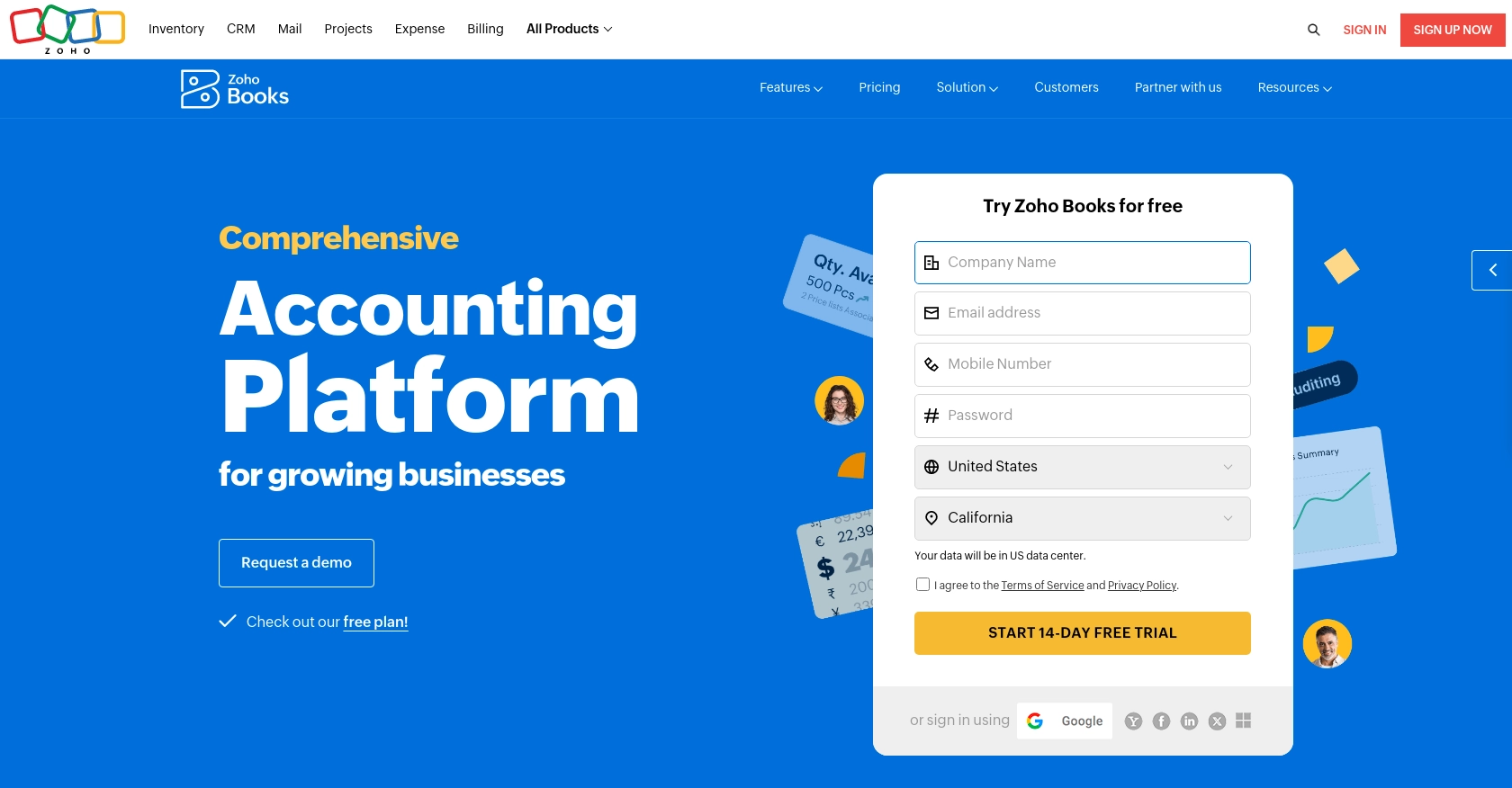
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a suite of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to streamline their financial operations.
For developers, integrating with the Zoho Books API can unlock powerful capabilities to automate and enhance business processes. One such use case is retrieving purchase orders using the Zoho Books API. This can be particularly useful for businesses that need to sync purchase order data with other systems, ensuring seamless operations and up-to-date records.
In this article, we will explore how to interact with the Zoho Books API using PHP to get purchase orders, providing you with the tools and knowledge to efficiently manage your financial data.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start interacting with the Zoho Books API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Zoho Books offers a free trial that you can use to access their API features.
Creating a Zoho Books Account
To begin, visit the Zoho Books website and sign up for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you will have access to the Zoho Books dashboard.
Registering a New Client in Zoho's Developer Console
Zoho Books uses OAuth 2.0 for authentication, which requires you to register your application to obtain a Client ID and Client Secret. Follow these steps:
- Go to the Zoho Developer Console.
- Click on Add Client ID and fill in the required details to register your application.
- Upon successful registration, you will receive your OAuth 2.0 credentials, including the Client ID and Client Secret. Keep these credentials secure.
Generating the OAuth Access Token
With your Client ID and Client Secret, you can now generate an access token to authenticate API requests:
- Redirect users to the following authorization URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified
redirect_uri
with acode
parameter. - Exchange this code for an access token by making a POST request to:
- Store the received access token securely, as it will be used in API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.purchaseorders.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth Documentation.
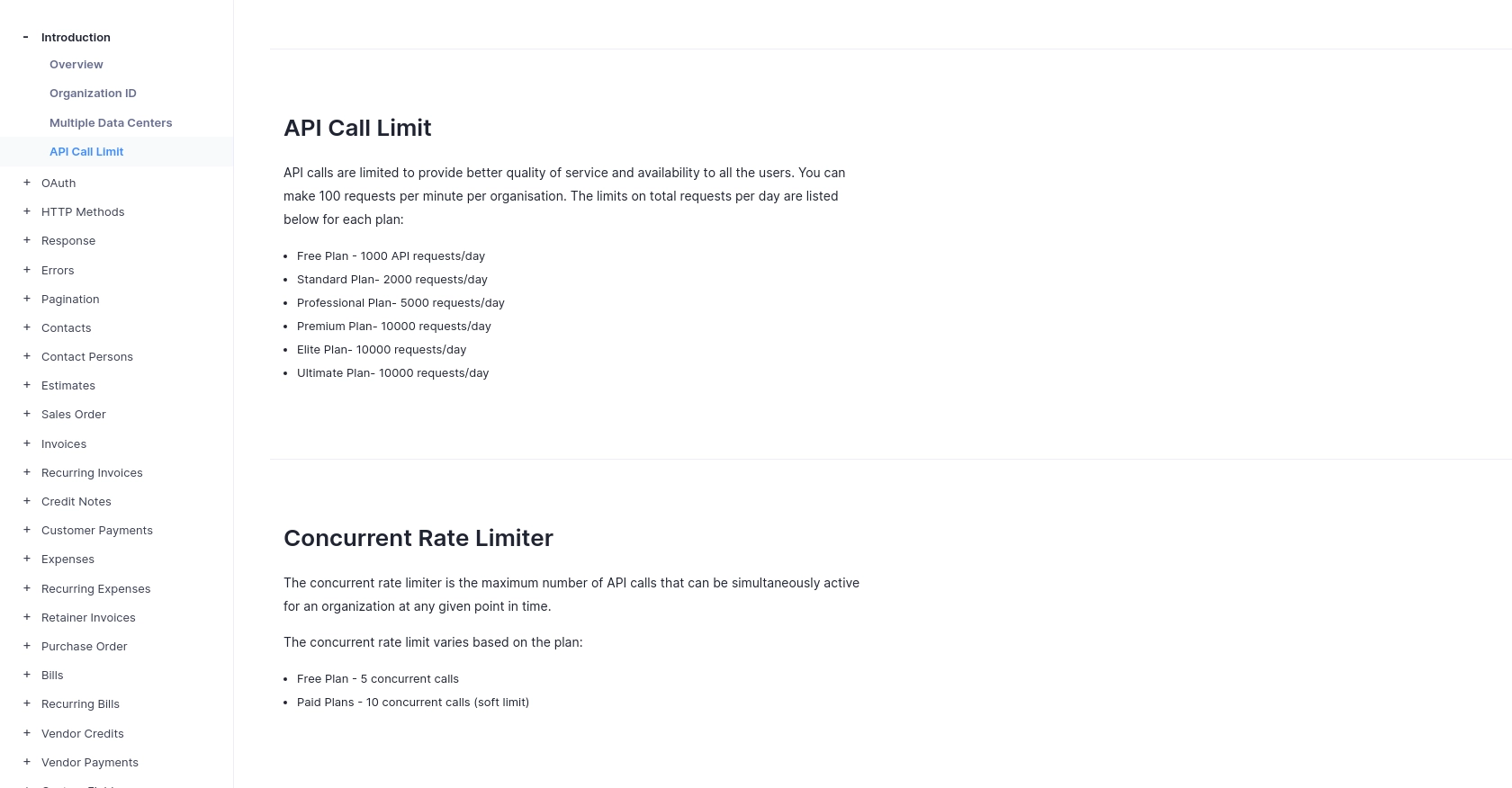
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from Zoho Books Using PHP
To interact with the Zoho Books API and retrieve purchase orders, you'll need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process, including setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled. You can check your PHP version and installed extensions using the following command:
php -v
php -m | grep curl
If cURL is not enabled, you can enable it by editing your php.ini
file and uncommenting the line:
extension=curl
Writing PHP Code to Make API Calls to Zoho Books
With your environment ready, you can now write PHP code to retrieve purchase orders from Zoho Books. Here's a step-by-step guide:
- Create a new PHP file, e.g.,
get_purchase_orders.php
. - Use the following code to set up the API call:
<?php
// Set your access token and organization ID
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
// Set the API endpoint
$url = "https://www.zohoapis.com/books/v3/purchaseorders?organization_id=$organizationId";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Zoho-oauthtoken $accessToken"
]);
// Execute the API call
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display the purchase orders
foreach ($data['purchaseorders'] as $purchaseOrder) {
echo "Purchase Order ID: " . $purchaseOrder['purchaseorder_id'] . "\n";
echo "Vendor Name: " . $purchaseOrder['vendor_name'] . "\n";
echo "Total: " . $purchaseOrder['total'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual Zoho Books access token and organization ID.
Handling API Response and Error Codes
After executing the API call, it's crucial to handle the response correctly. The Zoho Books API uses standard HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid.
- 401 Unauthorized: Authentication failed.
- 404 Not Found: The requested resource was not found.
- 429 Rate Limit Exceeded: Too many requests.
- 500 Internal Server Error: An error occurred on the server.
For more details on error handling, refer to the Zoho Books Error Documentation.
Verifying API Call Success in Zoho Books
To verify that your API call was successful, log in to your Zoho Books account and navigate to the purchase orders section. You should see the purchase orders listed as returned by your API call.
By following these steps, you can efficiently retrieve purchase orders from Zoho Books using PHP, ensuring your financial data is always up-to-date and synchronized with your systems.
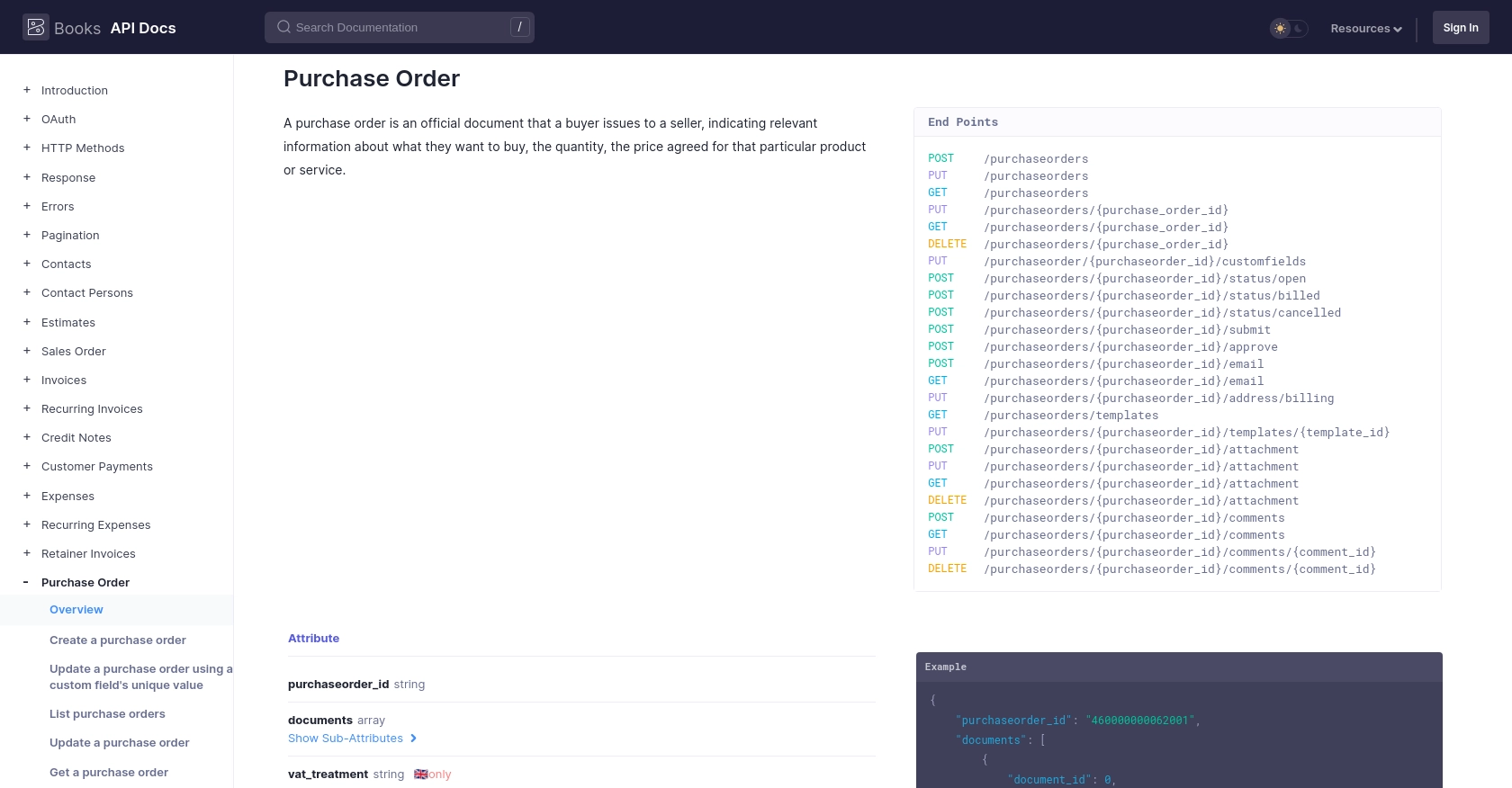
Best Practices for Using Zoho Books API in PHP
When working with the Zoho Books API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the access token and refresh token, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API has rate limits of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the API Call Limit Documentation.
- Implement Error Handling: Properly handle API errors by checking HTTP status codes and response messages. This will help you diagnose issues quickly and maintain a robust integration.
- Optimize Data Usage: Use pagination to manage large datasets efficiently. The Zoho Books API supports pagination, allowing you to retrieve data in chunks. For more information, see the Pagination Documentation.
Conclusion: Streamline Your Integrations with Endgrate
Integrating with the Zoho Books API using PHP can significantly enhance your business operations by automating financial processes and ensuring data consistency across systems. However, building and maintaining multiple integrations can be time-consuming and complex.
Endgrate offers a solution to streamline your integration efforts. By providing a unified API endpoint that connects to multiple platforms, including Zoho Books, Endgrate simplifies the integration process. This allows you to focus on your core product while ensuring a seamless experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/purchase-order/#overview
Ready to get started?