Using the Salesforce API to Create or Update Records in Python
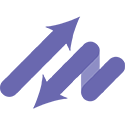
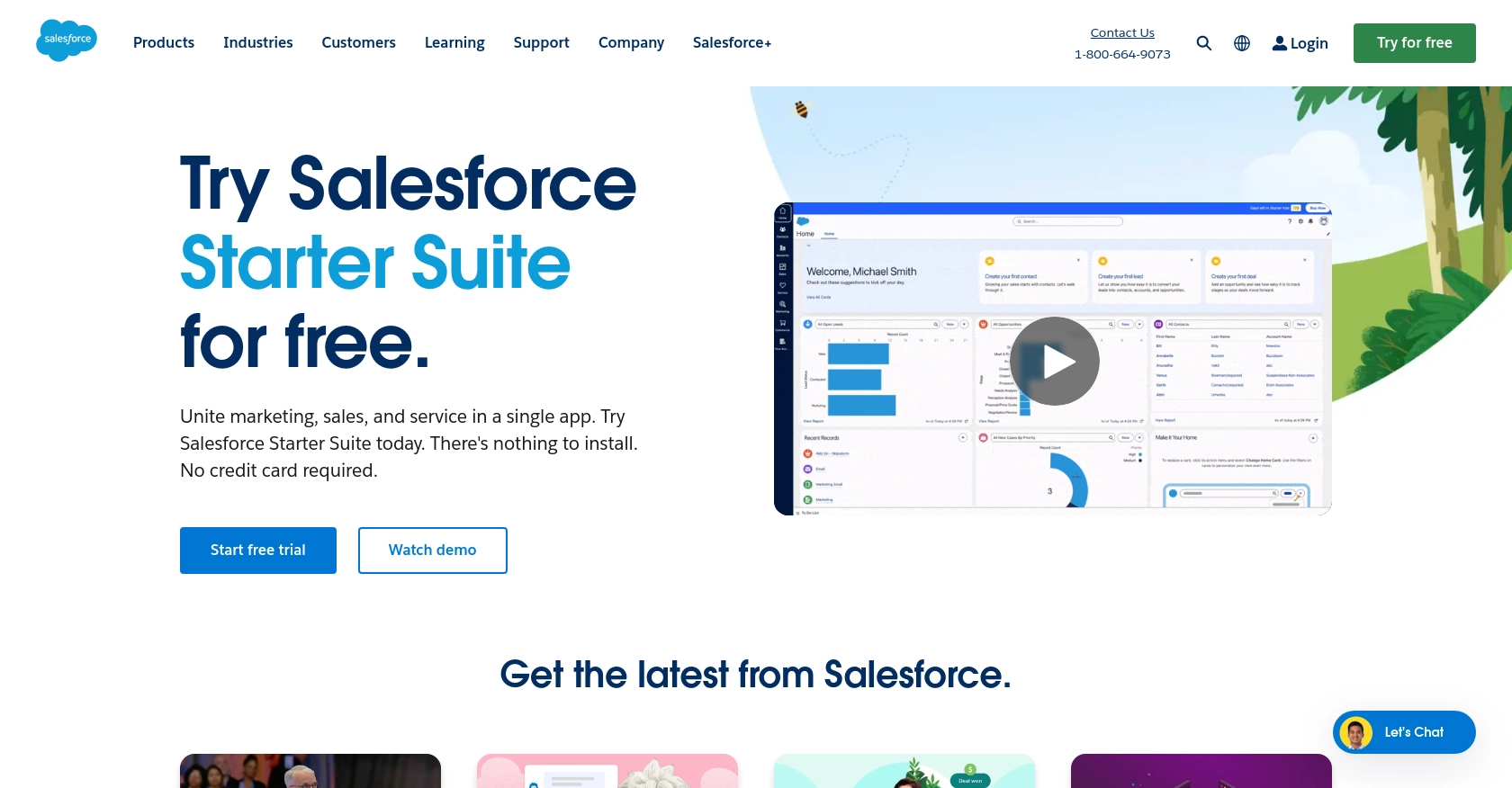
Introduction to Salesforce API Integration
Salesforce is a leading cloud-based customer relationship management (CRM) platform that empowers businesses to manage their sales, service, marketing, and more. Its robust suite of tools and extensive customization options make it a preferred choice for organizations aiming to streamline their operations and enhance customer engagement.
Developers often seek to integrate with Salesforce's API to automate and optimize business processes. By leveraging the Salesforce API, developers can create or update records programmatically, enabling seamless data synchronization between Salesforce and other systems. For example, a developer might use the Salesforce API to automatically update customer records in response to changes in an external database, ensuring that Salesforce always reflects the most current information.
Setting Up Your Salesforce Developer Account and Sandbox Environment
Before you can start integrating with the Salesforce API, you'll need to set up a Salesforce Developer Account and create a sandbox environment. This will allow you to test your API interactions without affecting live data.
Creating a Salesforce Developer Account
To begin, you'll need a Salesforce Developer Account. This account provides access to a free developer environment where you can build and test your applications.
- Visit the Salesforce Developer Signup Page.
- Fill in the required information, including your name, email, and company details.
- Agree to the terms and conditions, then click "Sign Me Up."
- Check your email for a verification link from Salesforce and follow the instructions to activate your account.
Creating a Salesforce Sandbox Environment
Once your developer account is set up, you can create a sandbox environment. This is a separate instance of Salesforce where you can safely test your API interactions.
- Log in to your Salesforce Developer Account.
- Navigate to the "Setup" area by clicking the gear icon in the top right corner.
- In the Quick Find box, type "Sandboxes" and select it from the menu.
- Click "New Sandbox" and choose the type of sandbox you need (e.g., Developer, Developer Pro).
- Enter a name for your sandbox and click "Create."
- Once created, you can access the sandbox by clicking the "Login" link next to your sandbox name.
Setting Up OAuth Authentication for Salesforce API
Salesforce uses OAuth 2.0 for authentication, allowing secure access to its API. Follow these steps to set up OAuth authentication:
- In your Salesforce Developer Account, go to "Setup" and search for "App Manager" in the Quick Find box.
- Click "New Connected App" in the top right corner.
- Fill in the required fields, such as "Connected App Name" and "API Name."
- Under "API (Enable OAuth Settings)," check "Enable OAuth Settings."
- Enter a "Callback URL" (e.g.,
https://localhost/callback
for testing purposes). - Select the OAuth scopes you need, such as "Full access" or "Access and manage your data."
- Click "Save" to create your connected app.
- After saving, you'll receive a "Consumer Key" and "Consumer Secret." Keep these credentials secure as you'll need them for API authentication.
For more detailed information on setting up OAuth, refer to the Salesforce OAuth Documentation.
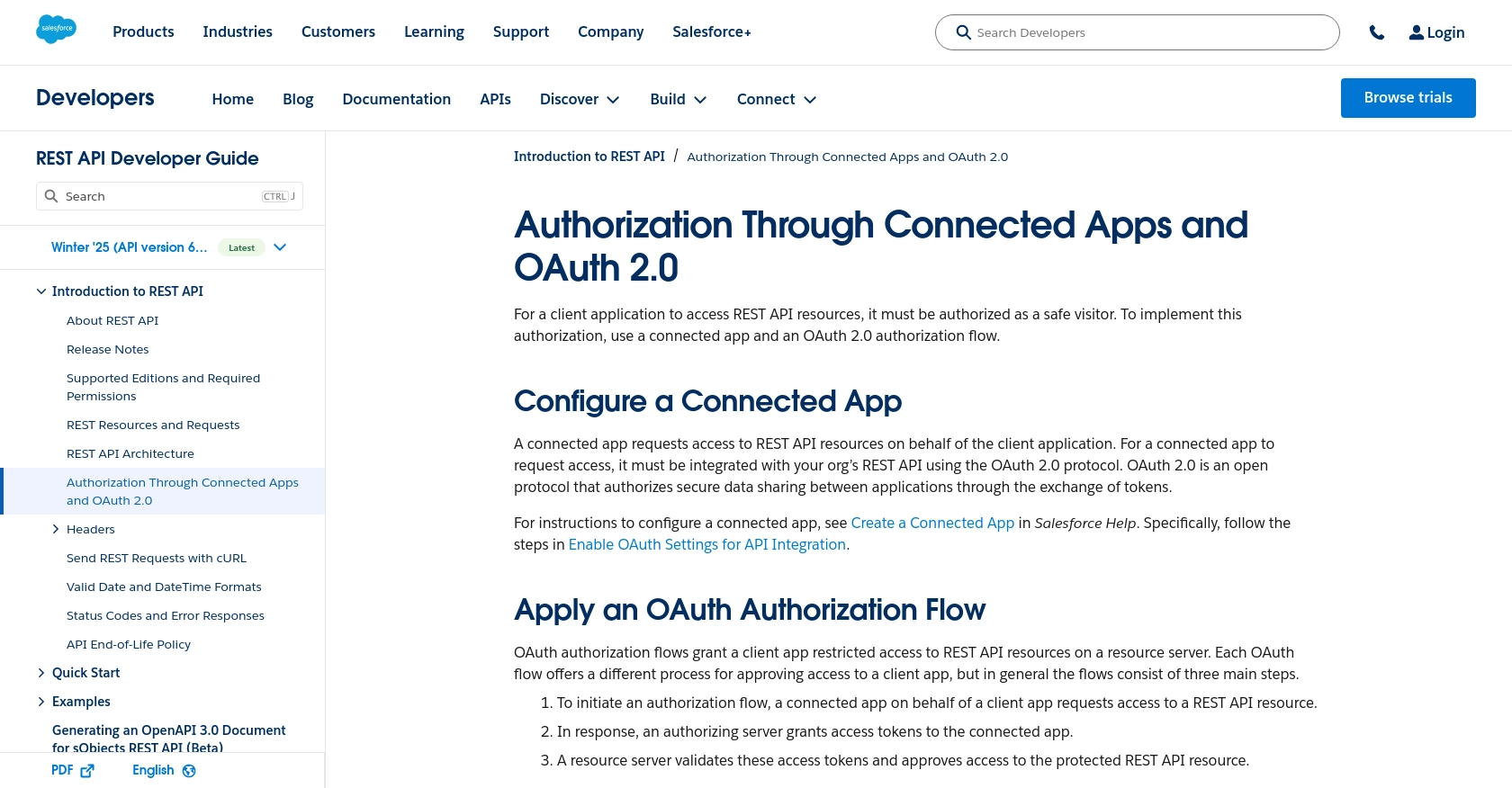
sbb-itb-96038d7
Making API Calls to Salesforce Using Python
To interact with the Salesforce API using Python, you'll need to set up your development environment and write code to create or update records. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and writing the code to perform API operations.
Setting Up Your Python Environment for Salesforce API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests to the Salesforce API:
pip install requests
Creating or Updating Salesforce Records Using Python
With your environment set up, you can now write Python code to create or update records in Salesforce. Below is a sample script that demonstrates how to authenticate and perform these operations.
import requests
# Salesforce OAuth credentials
consumer_key = 'Your_Consumer_Key'
consumer_secret = 'Your_Consumer_Secret'
username = 'Your_Salesforce_Username'
password = 'Your_Salesforce_Password'
security_token = 'Your_Security_Token'
# Salesforce login URL
login_url = 'https://login.salesforce.com/services/oauth2/token'
# Request payload for OAuth
payload = {
'grant_type': 'password',
'client_id': consumer_key,
'client_secret': consumer_secret,
'username': username,
'password': password + security_token
}
# Authenticate and get access token
response = requests.post(login_url, data=payload)
access_token = response.json().get('access_token')
instance_url = response.json().get('instance_url')
# Set headers for subsequent API requests
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
# Example: Create a new Salesforce account
account_data = {
'Name': 'New Account Name',
'Phone': '123-456-7890'
}
# Salesforce API endpoint for creating records
create_url = f'{instance_url}/services/data/v52.0/sobjects/Account/'
# Make the API call to create a record
create_response = requests.post(create_url, headers=headers, json=account_data)
if create_response.status_code == 201:
print('Account created successfully.')
else:
print('Failed to create account:', create_response.json())
# Example: Update an existing Salesforce account
account_id = 'Existing_Account_ID'
update_data = {
'Phone': '098-765-4321'
}
# Salesforce API endpoint for updating records
update_url = f'{instance_url}/services/data/v52.0/sobjects/Account/{account_id}'
# Make the API call to update a record
update_response = requests.patch(update_url, headers=headers, json=update_data)
if update_response.status_code == 204:
print('Account updated successfully.')
else:
print('Failed to update account:', update_response.json())
Replace placeholders like Your_Consumer_Key
, Your_Salesforce_Username
, and Existing_Account_ID
with your actual Salesforce credentials and data.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API calls by checking the Salesforce sandbox environment. If the record creation or update was successful, you should see the changes reflected there.
Handle errors by checking the response status codes and messages. Common error codes include:
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: Authentication failed. Check your credentials.
- 403 Forbidden: You do not have permission to perform this action.
- 404 Not Found: The specified resource could not be found.
For more detailed error handling, refer to the Salesforce API documentation.
Conclusion and Best Practices for Salesforce API Integration
Integrating with the Salesforce API using Python provides a powerful way to automate and streamline your business processes. By following the steps outlined in this guide, you can effectively create and update records in Salesforce, ensuring your data remains synchronized and up-to-date.
Best Practices for Secure and Efficient Salesforce API Usage
- Securely Store Credentials: Always store your Salesforce credentials, such as the Consumer Key and Secret, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Monitor your usage and implement retry logic to handle rate limit errors gracefully. For more information, refer to the Salesforce API documentation.
- Standardize Data Fields: Ensure that data fields are standardized and validated before sending them to Salesforce. This helps prevent errors and maintains data integrity.
- Implement Error Handling: Use robust error handling to manage API response codes and messages. This will help you quickly identify and resolve issues.
Streamline Your Integration Process with Endgrate
While integrating with Salesforce can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Salesforce. By leveraging Endgrate, you can focus on your core product while outsourcing integrations, saving both time and resources.
Explore how Endgrate can enhance your integration experience by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesforce
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?