How to Create Or Update Company with the Teamleader API in PHP
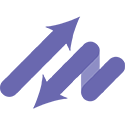
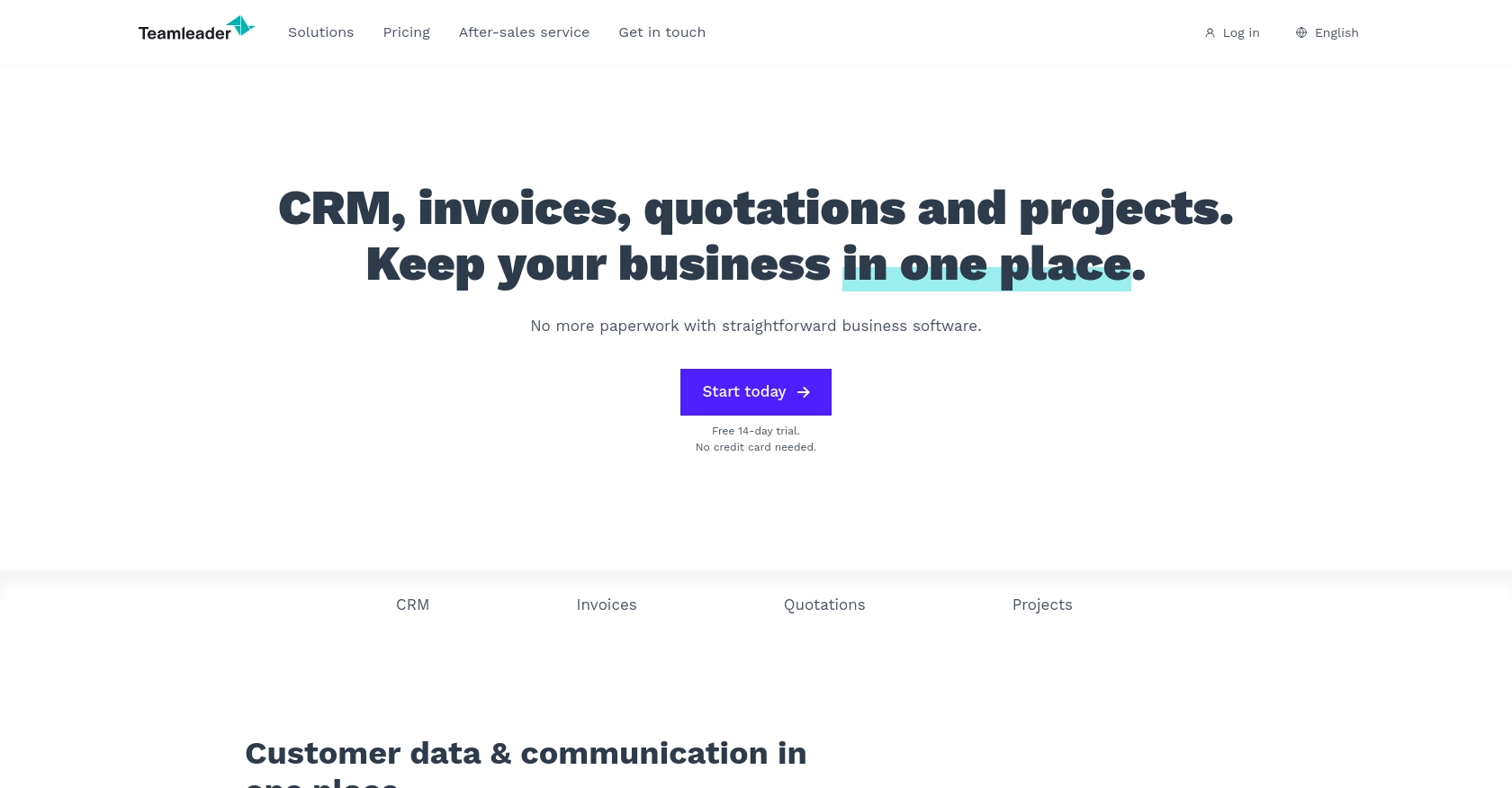
Introduction to Teamleader API
Teamleader is a comprehensive business management tool designed to streamline operations for small to medium-sized enterprises. It offers a suite of features including CRM, project management, and invoicing, all integrated into a single platform. This makes it an ideal choice for businesses looking to enhance their productivity and efficiency.
Developers may want to integrate with the Teamleader API to automate and manage company data effectively. For example, using the Teamleader API, a developer can create or update company information directly from their application, ensuring that the data remains consistent and up-to-date across all platforms.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start integrating with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. Follow the instructions to create your account. Once your account is set up, you'll have access to the Teamleader dashboard where you can manage your test data.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to set up OAuth authentication:
- Log in to your Teamleader account and navigate to the developer section.
- Create a new app by providing the required details such as the app name and description.
- Once the app is created, you'll receive a client ID and client secret. Make sure to store these securely as they will be used to authenticate your API requests.
- Set up the redirect URI, which is the URL where users will be redirected after they authorize your app. This should be a secure URL in your application.
- Request an access token by directing users to the Teamleader authorization URL, including your client ID and redirect URI. After users authorize the app, you'll receive an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Teamleader token endpoint, including your client ID, client secret, and authorization code.
With the access token, you can now make authenticated requests to the Teamleader API.
Generating API Keys for Teamleader
In addition to OAuth, you may need API keys for certain operations. Here's how to generate them:
- Navigate to the API keys section in your Teamleader account settings.
- Click on "Generate New Key" and provide a name for the key to identify its purpose.
- Copy the generated API key and store it securely. This key will be used in your API requests to authenticate and authorize access.
With your Teamleader account and authentication credentials set up, you're ready to start making API calls to create or update company data.
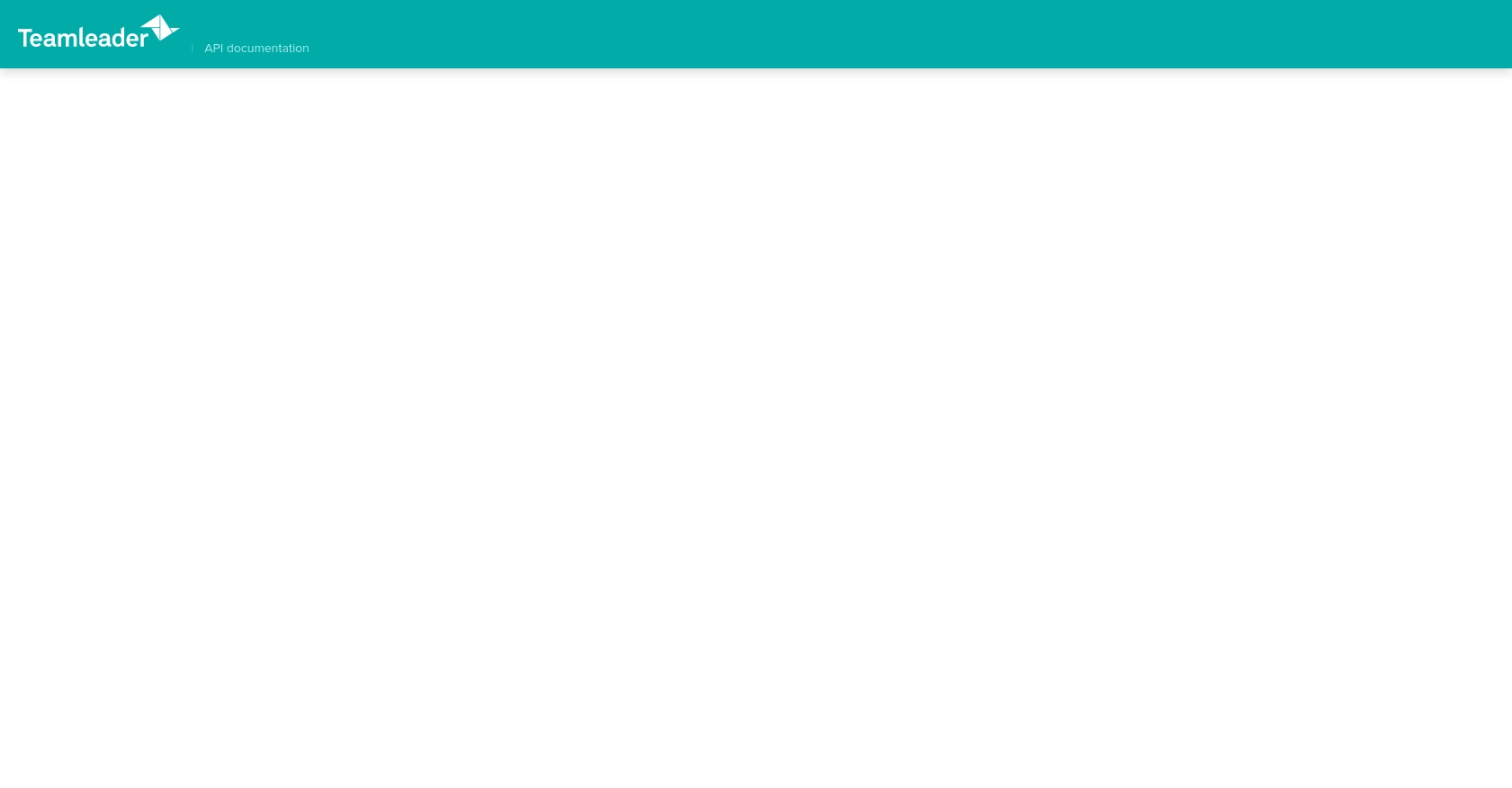
sbb-itb-96038d7
Making API Calls to Create or Update Company with Teamleader API in PHP
To interact with the Teamleader API for creating or updating company data, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Teamleader API Integration
Before diving into the code, ensure that your development environment is ready. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Company in Teamleader
Now that your environment is set up, you can write the PHP code to interact with the Teamleader API. Below is an example script to create or update a company:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
// Define the API endpoint
$url = 'https://api.teamleader.eu/companies.createOrUpdate';
// Set the request headers
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
];
// Define the company data
$companyData = [
'name' => 'Example Company',
'email' => 'contact@example.com',
'telephone' => '123456789'
];
try {
// Make the POST request
$response = $client->post($url, [
'headers' => $headers,
'json' => $companyData
]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
echo "Company created or updated successfully.";
} else {
echo "Failed to create or update company.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during the OAuth setup. This script uses Guzzle to send a POST request to the Teamleader API endpoint for creating or updating a company.
Verifying the API Request and Handling Errors
After running the script, you should verify that the company data has been created or updated in your Teamleader sandbox account. Check the dashboard to confirm the changes.
It's important to handle potential errors gracefully. The code above includes a try-catch block to catch exceptions and display error messages. Refer to the Teamleader API documentation for specific error codes and their meanings.
Conclusion and Best Practices for Using Teamleader API in PHP
Integrating with the Teamleader API to create or update company data in PHP can significantly enhance your application's functionality, ensuring seamless data management and synchronization. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and make API calls to manage company information.
Best Practices for Secure and Efficient API Integration with Teamleader
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to keep these credentials safe from unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Teamleader API to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data you send and receive from the API is properly formatted and standardized to maintain consistency across your application.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the Teamleader API documentation for detailed error codes and their meanings.
Streamline Your Integrations with Endgrate
While integrating with the Teamleader API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Teamleader. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of outsourcing integrations to streamline your development process.
Read More
Ready to get started?