Using the Recruit CRM API to Get Contacts in PHP
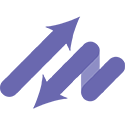
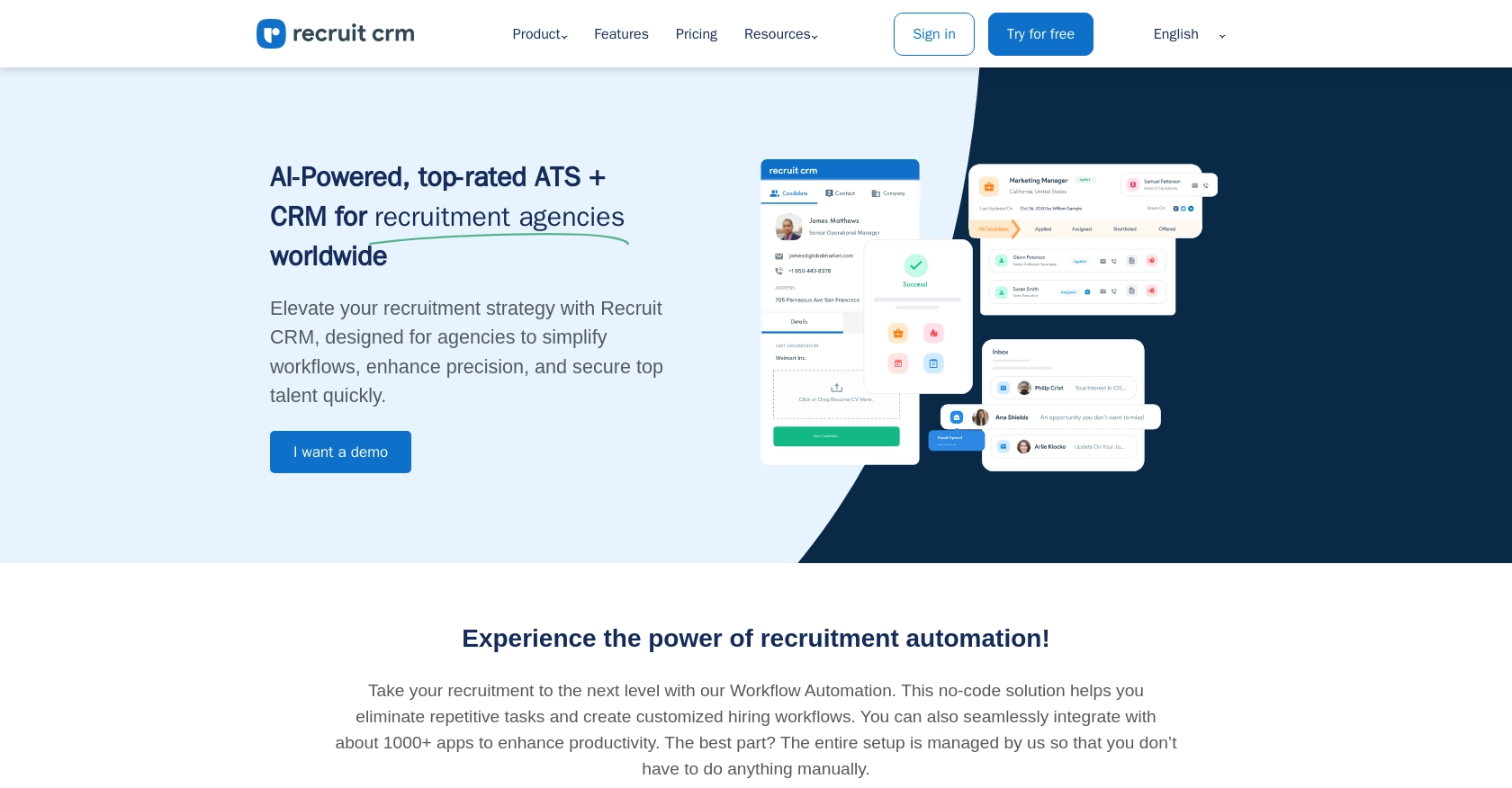
Introduction to Recruit CRM
Recruit CRM is a powerful recruitment software designed to enhance the efficiency of recruitment businesses. It offers a comprehensive suite of tools that streamline the recruitment process, including candidate management, job tracking, and client relationship management.
Integrating with the Recruit CRM API allows developers to customize and optimize recruitment workflows. For example, you can use the API to retrieve contact information, enabling seamless integration with other systems and enhancing data-driven decision-making.
Setting Up Your Recruit CRM Account for API Access
Before you can start using the Recruit CRM API to retrieve contacts, you'll need to set up your account and obtain the necessary API token. This token is essential for authenticating your requests and ensuring secure access to the API.
Creating a Recruit CRM Account
If you don't already have a Recruit CRM account, you can sign up for one by visiting the Recruit CRM website. Follow the instructions to create an account and log in to the platform.
Accessing the API Token in Recruit CRM
Once you have your account set up, follow these steps to obtain your API token:
- Navigate to the Admin Setting section in your Recruit CRM dashboard.
- Select Account Management from the menu.
- Locate the API Token section and generate a new token if you haven't already.
- Copy the API token and store it securely, as it will be used to authenticate your API requests.
Ensure that your API token is kept confidential and not stored in publicly accessible areas, as it carries significant privileges.
Understanding Recruit CRM API Authentication
The Recruit CRM API uses API tokens for authentication. All API requests must be made over HTTPS, and requests without authentication will fail. Make sure to include the API token in the HTTP headers of your requests as shown in the example below:
$ curl https://api.recruitcrm.io/v1/contacts \
-H 'Authorization:Bearer <Your API Token>' \
-H 'Accept:application/json'
Replace <Your API Token>
with the token you obtained earlier.
For more information, you can refer to the Recruit CRM API documentation.
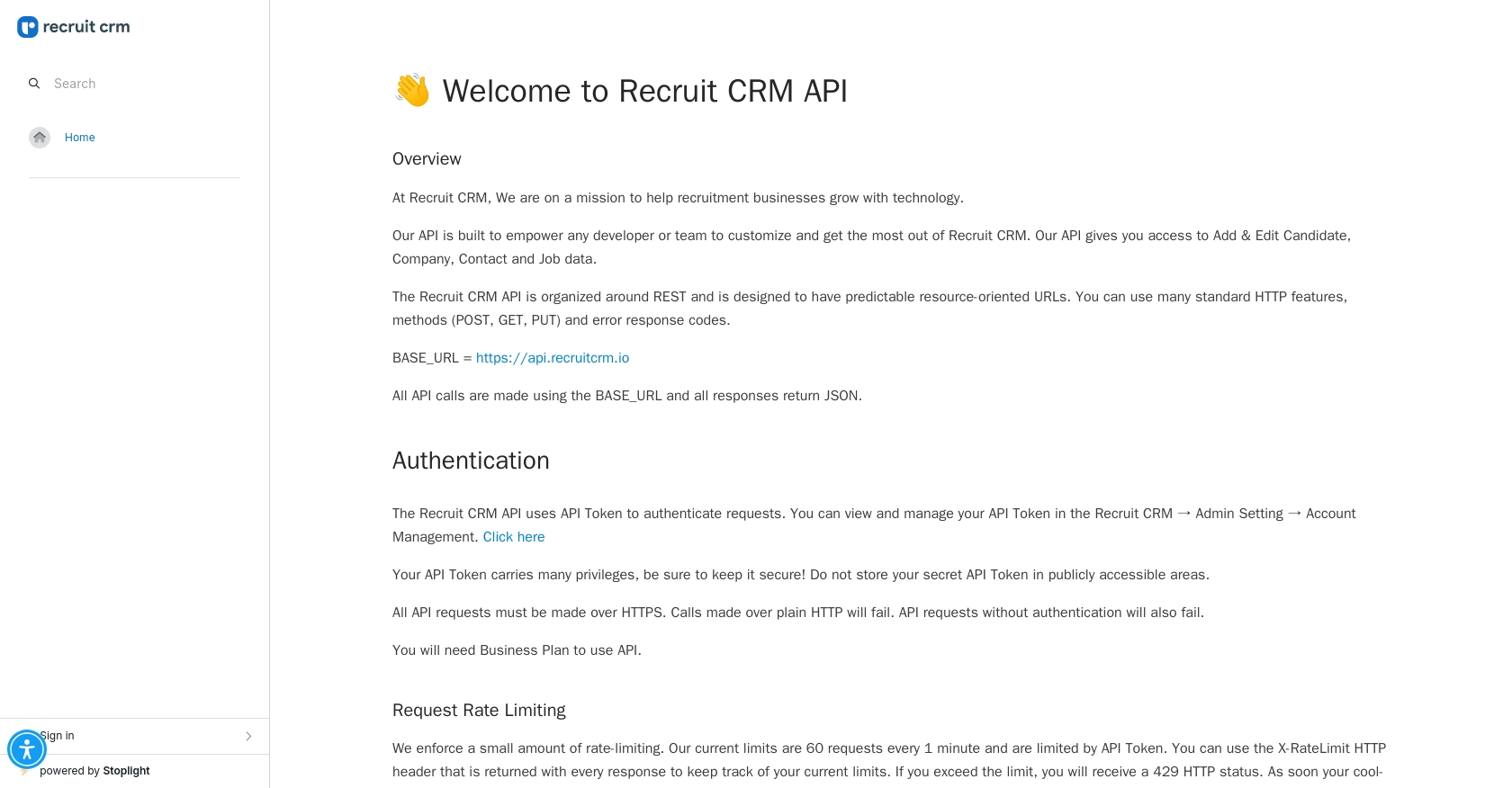
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Recruit CRM Using PHP
To interact with the Recruit CRM API and retrieve contact information, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, installing necessary dependencies, and executing the API call to fetch contacts.
Setting Up Your PHP Environment
Before making API calls, ensure you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need the cURL
extension enabled, which is commonly included in PHP installations.
Installing Required PHP Dependencies
To make HTTP requests in PHP, we'll use the cURL
library. Ensure it's enabled in your php.ini
configuration file. You can verify this by running the following command:
php -m | grep curl
If curl
is listed, you're ready to proceed.
Writing PHP Code to Retrieve Contacts from Recruit CRM
Create a new PHP file named get_recruitcrm_contacts.php
and add the following code to it:
<?php
// Set the API endpoint and headers
$endpoint = "https://api.recruitcrm.io/v1/contacts";
$apiToken = "Your_API_Token"; // Replace with your actual API token
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $apiToken",
"Accept: application/json"
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error: ' . curl_error($ch);
} else {
// Parse the JSON response
$contacts = json_decode($response, true);
// Display the contacts
foreach ($contacts as $contact) {
echo "Name: " . $contact['name'] . "<br>";
echo "Email: " . $contact['email'] . "<br><br>";
}
}
// Close the cURL session
curl_close($ch);
?>
Replace Your_API_Token
with the API token you obtained from Recruit CRM.
Executing the PHP Script and Verifying the Output
Run the PHP script from the command line or a web server:
php get_recruitcrm_contacts.php
You should see a list of contacts displayed, including their names and email addresses.
Handling Errors and Verifying API Request Success
It's crucial to handle potential errors when making API requests. The above script checks for cURL errors and outputs them if any occur. Additionally, ensure that the response contains valid JSON data before attempting to parse it.
To verify the success of your API request, you can cross-check the retrieved contacts with those in your Recruit CRM sandbox account. If the data matches, your integration is working correctly.
For more detailed information on error codes and handling, refer to the Recruit CRM API documentation.
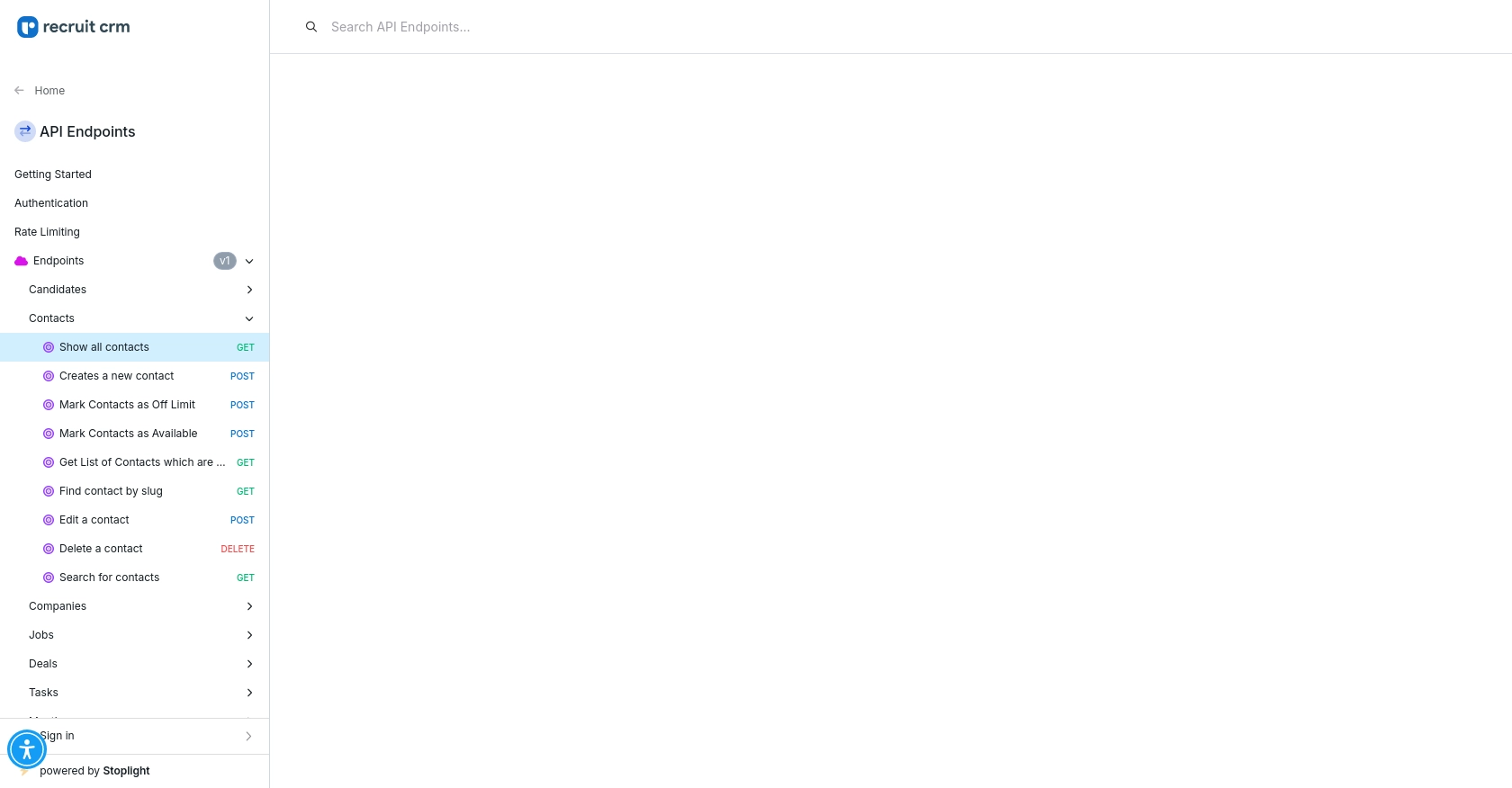
Conclusion and Best Practices for Using Recruit CRM API in PHP
Integrating with the Recruit CRM API using PHP allows developers to efficiently manage and retrieve contact data, enhancing recruitment workflows and enabling seamless integration with other systems. By following the steps outlined in this article, you can set up your environment, authenticate requests, and handle API interactions effectively.
Best Practices for Secure and Efficient API Integration
- Secure API Tokens: Always store your API tokens securely and avoid exposing them in publicly accessible areas. Consider using environment variables or secure vaults to manage sensitive credentials.
- Handle Rate Limiting: Recruit CRM enforces a rate limit of 60 requests per minute. Implement logic to handle HTTP status code 429 and retry requests after the cooldown period. Use the
X-RateLimit
header to monitor your usage. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your systems. This can help maintain data integrity and consistency across platforms.
- Error Handling: Implement robust error handling to manage potential issues with API requests. Check for cURL errors and validate JSON responses to ensure successful data retrieval.
Enhancing Integration Capabilities with Endgrate
While integrating with Recruit CRM API directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Recruit CRM.
By leveraging Endgrate, you can streamline your development efforts, reduce maintenance overhead, and focus on your core product. Endgrate's intuitive integration experience allows you to build once for each use case, providing a seamless experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover the benefits of outsourcing integrations to focus on what truly matters.
Read More
Ready to get started?