Using the Eventbrite API to Get Attendees (with Javascript examples)
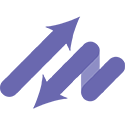
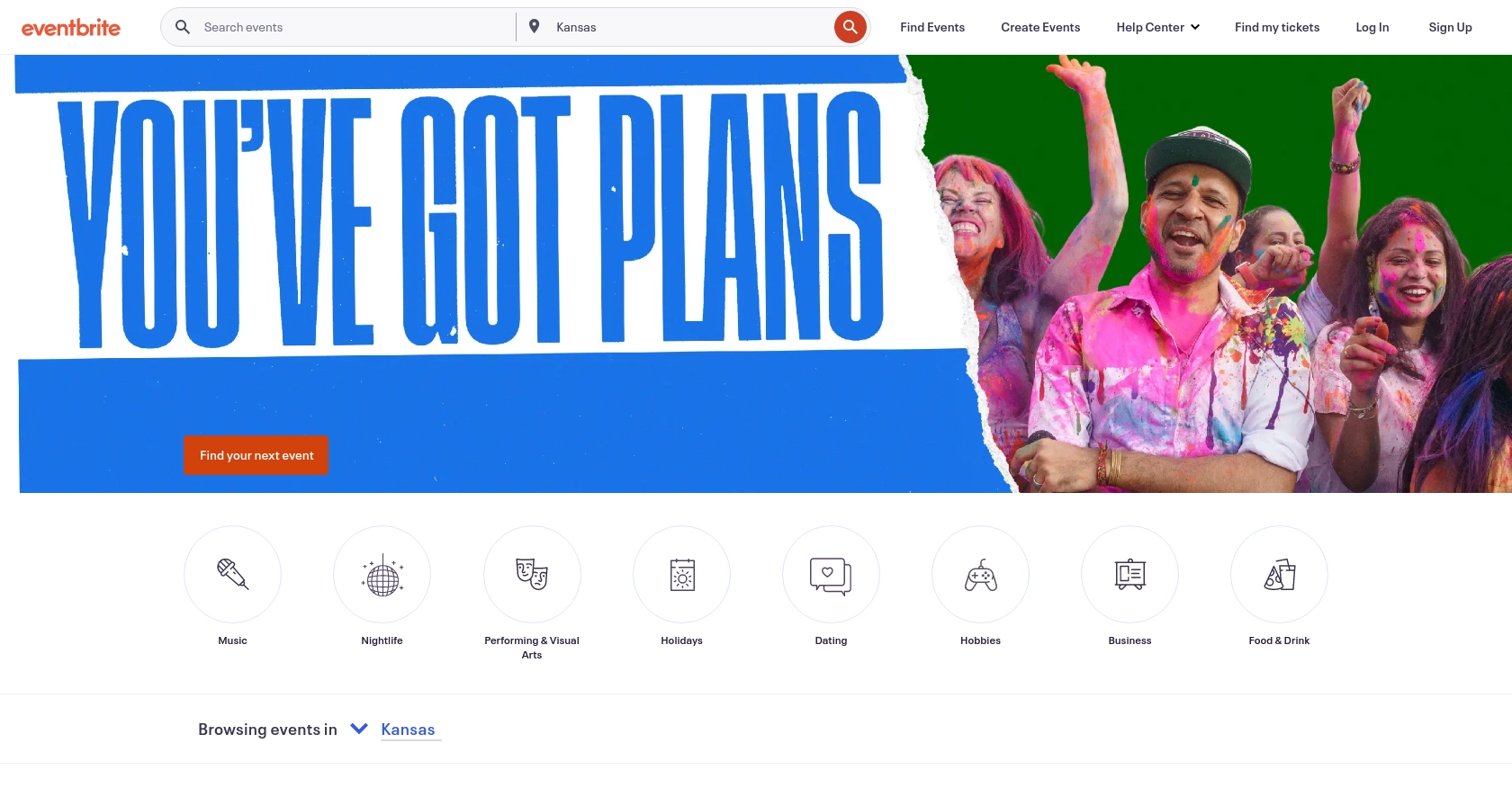
Introduction to Eventbrite API Integration
Eventbrite is a popular event management and ticketing platform that enables users to create, promote, and manage events. It offers a robust API that allows developers to access and interact with event data, making it an essential tool for businesses looking to enhance their event management capabilities.
Integrating with the Eventbrite API can empower developers to automate and streamline various event-related processes. For example, you might want to retrieve a list of attendees for a specific event to personalize communications or analyze attendance patterns. This article will guide you through using JavaScript to interact with the Eventbrite API and efficiently obtain attendee information.
Setting Up Your Eventbrite Developer Account and OAuth Authentication
Before you can start interacting with the Eventbrite API, you'll need to set up a developer account and configure OAuth authentication. This process will allow you to securely access Eventbrite's data and services.
Creating an Eventbrite Developer Account
To begin, you'll need an Eventbrite account. If you don't have one, you can sign up for free on the Eventbrite website. Once you have an account, follow these steps to create a developer account:
- Log in to your Eventbrite account.
- Navigate to the Eventbrite Developer Portal.
- Click on "Create a New App" to start the process of setting up your application.
Configuring OAuth Authentication for Eventbrite API
The Eventbrite API uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to configure OAuth:
- In the Developer Portal, fill out the required fields for your new app, such as the app name and description.
- Set the redirect URI, which is the URL to which users will be redirected after they authorize your app. This should be a secure URL (https).
- Once your app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure, as they are essential for authenticating API requests.
Generating Access Tokens
With your Client ID and Client Secret, you can now generate access tokens to authenticate API requests. Here's a basic outline of the process:
- Direct users to the Eventbrite authorization URL, including your Client ID and redirect URI as parameters.
- After users authorize your app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Eventbrite token endpoint, including your Client ID, Client Secret, and authorization code.
Once you have an access token, you can use it to authenticate your API requests and access Eventbrite data.
For more detailed information, refer to the Eventbrite API documentation.
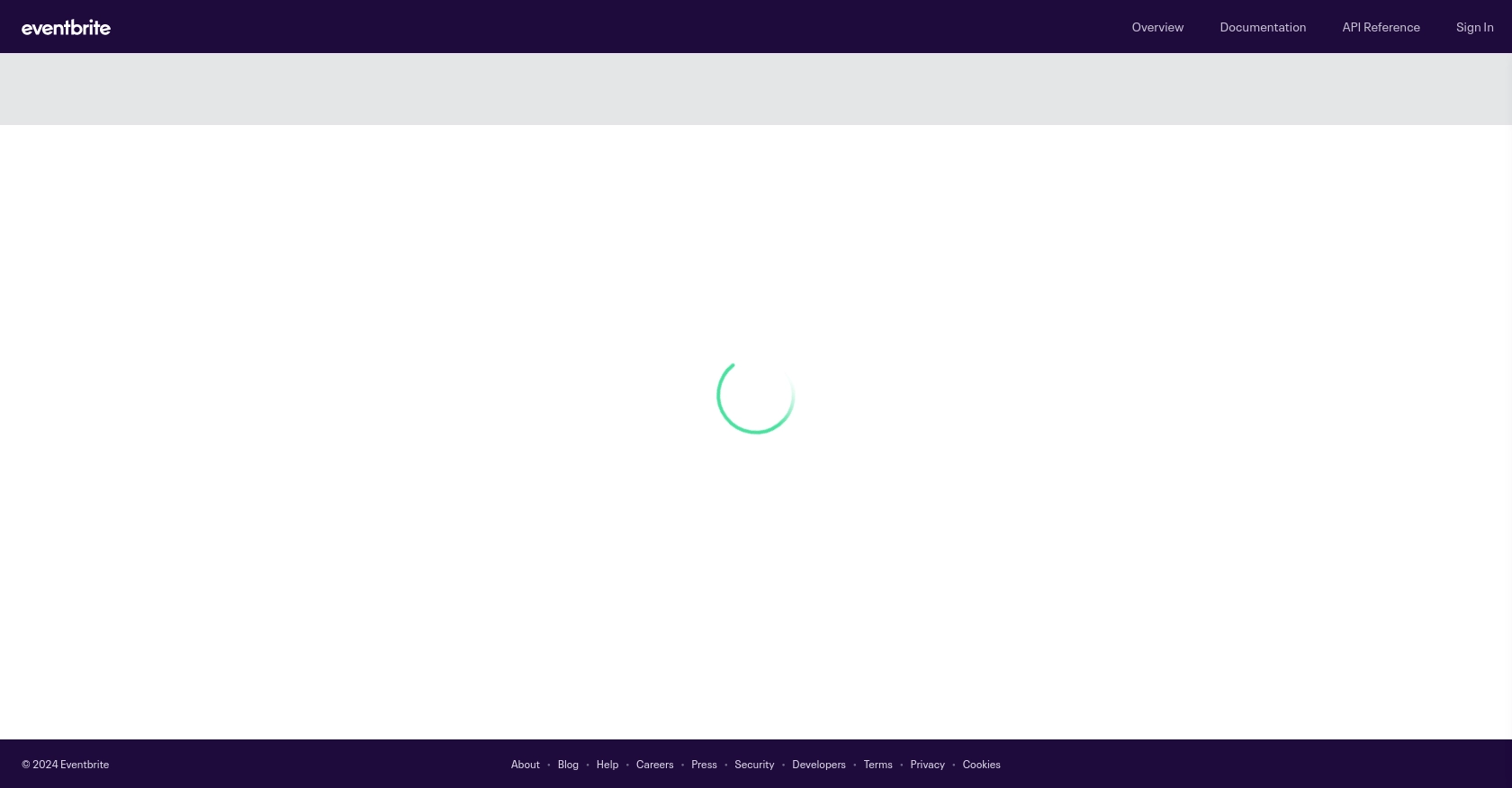
sbb-itb-96038d7
Making API Calls to Retrieve Eventbrite Attendees Using JavaScript
To interact with the Eventbrite API and retrieve attendee information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Eventbrite API Integration
Before making API calls, ensure you have a modern JavaScript environment set up. You can use Node.js or a browser-based environment to execute your code. For this tutorial, we'll focus on using Node.js.
- Ensure you have Node.js installed. You can download it from the official Node.js website.
- Create a new directory for your project and navigate to it in your terminal.
- Initialize a new Node.js project by running
npm init -y
to create apackage.json
file. - Install the
axios
library to simplify making HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Attendees from Eventbrite
With your environment ready, you can now write the JavaScript code to fetch attendees from Eventbrite. Create a new file named getAttendees.js
and add the following code:
const axios = require('axios');
// Replace 'Your_Access_Token' with your actual access token
const accessToken = 'Your_Access_Token';
// Replace 'Your_Event_ID' with the ID of the event you want to fetch attendees for
const eventId = 'Your_Event_ID';
// Set the API endpoint
const endpoint = `https://www.eventbriteapi.com/v3/events/${eventId}/attendees/`;
// Set the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`
};
// Make a GET request to the Eventbrite API
axios.get(endpoint, { headers })
.then(response => {
const attendees = response.data.attendees;
attendees.forEach(attendee => {
console.log(`Name: ${attendee.profile.name}, Email: ${attendee.profile.email}`);
});
})
.catch(error => {
console.error('Error fetching attendees:', error.response ? error.response.data : error.message);
});
In this code, we use the axios
library to make a GET request to the Eventbrite API. Replace Your_Access_Token
with your actual access token and Your_Event_ID
with the ID of the event you wish to retrieve attendees for. The response will include attendee details, which we log to the console.
Verifying Successful API Requests and Handling Errors
After running your script, you should see a list of attendees printed in the console. To verify the request's success, cross-check the output with the attendees listed in your Eventbrite account for the specified event.
Handling errors is crucial for robust API integration. The code includes error handling using a catch
block, which logs any errors encountered during the request. Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters and try again.
- 401 Unauthorized: Authentication failed. Ensure your access token is correct.
- 404 Not Found: The specified event or resource does not exist.
For more detailed error information, refer to the Eventbrite API documentation.
Conclusion and Best Practices for Eventbrite API Integration
Integrating with the Eventbrite API using JavaScript can significantly enhance your event management capabilities by automating attendee data retrieval and analysis. By following the steps outlined in this article, you can efficiently access and manage attendee information, enabling personalized communication and insightful event analytics.
Best Practices for Secure and Efficient Eventbrite API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handling Rate Limits: Be mindful of the API rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully. Refer to the Eventbrite API documentation for specific rate limit details.
- Data Transformation and Standardization: When retrieving attendee data, consider transforming and standardizing fields to match your application's data model. This ensures consistency and simplifies data processing.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Eventbrite can be powerful, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help. By providing a unified API endpoint, Endgrate allows you to connect with multiple platforms, including Eventbrite, through a single integration point.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This streamlined approach enables you to focus on your core product while delivering an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs and enhance your development workflow by visiting Endgrate's website.
Read More
Ready to get started?